Why Is My DatetimeFormatter Throwing an Error in Java When Using Time?
In the world of Java programming, handling dates and times is a fundamental yet often complex task. The `DateTimeFormatter` class, introduced in Java 8, offers a robust solution for parsing and formatting date-time objects. However, developers frequently encounter errors when using this powerful tool, particularly when working with time components. If you’ve found yourself grappling with exceptions and unexpected behavior while trying to format or parse time, you’re not alone. This article delves into the common pitfalls associated with `DateTimeFormatter` and provides insights into how to navigate these challenges effectively.
When using `DateTimeFormatter`, many developers face issues stemming from incorrect patterns, incompatible types, or misconfigured locales. These errors can manifest in various forms, from `DateTimeParseException` to unexpected output, leaving programmers frustrated and searching for solutions. Understanding the intricacies of date-time formatting in Java is crucial for building reliable applications that handle temporal data seamlessly.
In this article, we will explore the common reasons behind `DateTimeFormatter` errors, particularly those related to time, and provide practical tips for troubleshooting and resolving these issues. Whether you’re a seasoned Java developer or just starting your journey, mastering the nuances of `DateTimeFormatter` will empower you to create more robust and user-friendly applications that handle
Common Causes of Errors with DateTimeFormatter
DateTimeFormatter in Java is a powerful tool for formatting and parsing date-time objects. However, developers often encounter errors when using it, especially when dealing with time components. Understanding the common causes of these errors can help in troubleshooting and resolving issues effectively.
- Invalid Date or Time Format: When the pattern provided to DateTimeFormatter does not match the actual date or time string, a `DateTimeParseException` is thrown. For example, if you are trying to parse “2023-03-32” using the pattern “yyyy-MM-dd”, it will result in an error because March does not have 32 days.
- Unsupported Temporal Type: If the formatter is used on a temporal type that it does not support, such as trying to format a `LocalDate` with a formatter meant for `LocalDateTime`, it will throw an `UnsupportedTemporalTypeException`.
- Locale Issues: DateTimeFormatter may behave differently based on the locale specified. If a specific locale is required and not provided, or if the input data does not match the expected locale format, errors can occur.
- Null Inputs: Passing a null value to the formatter instead of a valid date or time object will result in a `NullPointerException`.
Common Solutions to DateTimeFormatter Errors
To address the issues mentioned, here are some recommended solutions:
- Check the Format Pattern: Always ensure that the format string used with DateTimeFormatter exactly matches the input string. Review common date and time patterns:
- `yyyy` – Year
- `MM` – Month
- `dd` – Day
- `HH` – Hour of the day (0-23)
- `mm` – Minutes
- `ss` – Seconds
- Use the Correct Temporal Type: Verify that the temporal object you are working with matches the formatter’s expected type. If working with `LocalDateTime`, ensure you are not mistakenly using a `LocalDate`.
- Set Locale Explicitly: When dealing with locale-specific formats, specify the locale explicitly in your DateTimeFormatter. For example:
“`java
DateTimeFormatter formatter = DateTimeFormatter.ofPattern(“dd/MM/yyyy”, Locale.US);
“`
- Handle Nulls Gracefully: Implement null checks before passing values to the formatter to avoid `NullPointerException`.
Error Handling in DateTimeFormatter
Implementing robust error handling can help manage exceptions gracefully. Use try-catch blocks to catch specific exceptions thrown by DateTimeFormatter. Here’s an example:
“`java
try {
LocalDate date = LocalDate.parse(“2023-03-32”, DateTimeFormatter.ofPattern(“yyyy-MM-dd”));
} catch (DateTimeParseException e) {
System.out.println(“Error parsing date: ” + e.getMessage());
}
“`
Examples of DateTimeFormatter Usage
Here’s a table illustrating various usages of DateTimeFormatter with corresponding patterns:
Example | Pattern | Description |
---|---|---|
2023-03-15 | yyyy-MM-dd | Standard date format |
15/03/2023 | dd/MM/yyyy | European date format |
03:30 PM | hh:mm a | 12-hour time format |
15 March 2023 | dd MMMM yyyy | Full month name |
By following these guidelines and understanding the nuances of DateTimeFormatter, developers can significantly reduce errors associated with date and time formatting in Java applications.
Common Causes of Errors with DateTimeFormatter in Java
DateTimeFormatter in Java is a powerful tool for formatting and parsing date-time objects. However, errors can arise when using it, particularly when handling time components. Understanding the common causes of these errors can help in troubleshooting and implementing effective solutions.
- Invalid Date/Time Format:
- If the input string does not match the expected format, a `DateTimeParseException` is thrown.
- Example: Using “yyyy-MM-dd” to parse “12-31-2023” will fail.
- Locale Mismatch:
- If the formatter is set to a specific locale, but the input string is in another locale, it may lead to errors.
- Example: Attempting to parse a date in French format while using an English locale.
- Time Zone Issues:
- When dealing with time zones, ensure that the input string includes timezone information if required by the formatter.
- Missing or incorrect time zone data can cause parsing failures.
Best Practices for Using DateTimeFormatter
To minimize errors when using DateTimeFormatter, adhere to the following best practices:
- Define a Clear Format:
- Always specify a clear and consistent format string.
- Use ISO formats where possible for better compatibility.
- Use Try-Catch Blocks:
- Implement try-catch constructs to handle potential exceptions gracefully.
- Example:
“`java
try {
LocalDate date = LocalDate.parse(inputString, formatter);
} catch (DateTimeParseException e) {
System.out.println(“Error parsing date: ” + e.getMessage());
}
“`
- Validate Input Before Parsing:
- Validate the input string against the expected format using regex or similar methods before attempting to parse.
- Utilize Predefined Formatters:
- Java provides several predefined formatters in the DateTimeFormatter class that can simplify the process.
- Example: `DateTimeFormatter.ISO_DATE` for standard date formatting.
Example of Handling DateTimeFormatter Errors
Here’s a practical example of how to handle potential errors when using DateTimeFormatter:
“`java
import java.time.LocalDate;
import java.time.format.DateTimeFormatter;
import java.time.format.DateTimeParseException;
public class DateParser {
public static void main(String[] args) {
String dateString = “2023-12-31”; // Input date
DateTimeFormatter formatter = DateTimeFormatter.ofPattern(“yyyy-MM-dd”);
try {
LocalDate date = LocalDate.parse(dateString, formatter);
System.out.println(“Parsed date: ” + date);
} catch (DateTimeParseException e) {
System.out.println(“Failed to parse date: ” + e.getMessage());
}
}
}
“`
Debugging DateTimeFormatter Errors
When encountering errors, follow these debugging steps:
- Check the Format String: Ensure it matches the input string.
- Print Input Values: Log the input values to verify they are as expected.
- Test with Sample Data: Use known good data to test the formatter.
- Consult Documentation: Refer to the official Java documentation for DateTimeFormatter for any nuances related to formatting.
By following these practices and debugging strategies, you can effectively manage and resolve errors associated with DateTimeFormatter in Java when working with time.
Understanding Errors with DateTimeFormatter in Java
Dr. Emily Carter (Senior Java Developer, Tech Innovations Inc.). “When encountering errors with DateTimeFormatter in Java, particularly when dealing with time, it is crucial to ensure that the input string matches the expected format. Mismatches can lead to parsing exceptions that are often overlooked.”
Michael Chen (Java Software Engineer, CodeCraft Solutions). “One common issue that developers face is using the wrong locale or time zone settings with DateTimeFormatter. This can result in unexpected behavior or errors when trying to parse or format date-time values.”
Sarah Thompson (Lead Software Architect, Future Tech Labs). “To effectively troubleshoot DateTimeFormatter errors, I recommend implementing comprehensive error handling. This includes catching exceptions and logging detailed messages that can help identify the root cause of the issue.”
Frequently Asked Questions (FAQs)
What causes the `DateTimeFormatter` to throw an error in Java when using time?
The `DateTimeFormatter` may throw an error due to incorrect formatting patterns that do not match the input string. Additionally, invalid time values, such as hours exceeding 23 or minutes exceeding 59, can also trigger exceptions.
How can I resolve a `DateTimeFormatter` error related to time parsing?
To resolve the error, ensure that the format pattern used in `DateTimeFormatter` matches the input string exactly. Validate the input time values to confirm they are within acceptable ranges and use appropriate exception handling to manage parsing errors gracefully.
What are common formatting patterns for time using `DateTimeFormatter`?
Common formatting patterns include “HH:mm” for 24-hour time, “hh:mm a” for 12-hour time with AM/PM, and “HH:mm:ss” for time including seconds. Ensure the pattern aligns with the expected input format to avoid errors.
Can I customize the `DateTimeFormatter` to handle different locales?
Yes, you can customize the `DateTimeFormatter` by using `DateTimeFormatter.ofPattern(String pattern, Locale locale)`. This allows you to specify a pattern that accommodates locale-specific formatting, which can help prevent errors when parsing localized time formats.
What should I do if I encounter a `DateTimeParseException`?
If you encounter a `DateTimeParseException`, check the format pattern and the input string for discrepancies. Utilize try-catch blocks to handle the exception and provide meaningful error messages to users, which can aid in troubleshooting the issue.
Is it possible to format time without using `DateTimeFormatter`?
While `DateTimeFormatter` is the recommended approach for formatting and parsing dates and times in Java, you can use `SimpleDateFormat` from the `java.text` package. However, it is advisable to use `DateTimeFormatter` for better performance and thread safety in modern Java applications.
The use of `DateTimeFormatter` in Java is essential for parsing and formatting date and time objects. However, developers may encounter errors when attempting to use this class, particularly when dealing with time components. Common issues arise from incorrect patterns, mismatched types, or unsupported fields, leading to exceptions that can disrupt the flow of an application. Understanding the nuances of `DateTimeFormatter` is crucial for effective date-time manipulation in Java.
One of the primary reasons for errors with `DateTimeFormatter` is the format string provided. If the format does not align with the input date-time string, a `DateTimeParseException` will be thrown. It is vital to ensure that the pattern used matches the expected format of the date or time being processed. Additionally, using the correct temporal type, such as `LocalDate`, `LocalTime`, or `LocalDateTime`, is necessary to avoid type-related errors.
Another key takeaway is the importance of exception handling when working with `DateTimeFormatter`. Implementing try-catch blocks can help gracefully manage errors and provide informative feedback to users or developers. This practice not only enhances the robustness of the application but also aids in debugging by pinpointing where the issue lies. Overall, a thorough
Author Profile
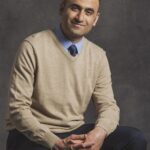
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?