How Can I Delete All Empty Folders Using PowerShell?
In the digital age, our file systems can quickly become cluttered with unnecessary data, and one of the most common culprits of this disarray is empty folders. While they may seem harmless, these vacant spaces can accumulate over time, making it difficult to navigate your directories efficiently. If you’re looking to streamline your file management process and reclaim valuable storage space, learning how to delete all empty folders using PowerShell can be a game-changer. This powerful scripting language offers a swift and effective solution for tidying up your system, allowing you to focus on what truly matters.
PowerShell is a versatile tool that empowers users to automate tasks and manage system resources with ease. When it comes to empty folders, the traditional method of manually sifting through directories can be tedious and time-consuming. Fortunately, PowerShell provides a straightforward approach to identify and eliminate these superfluous folders in bulk. By harnessing the power of commands and scripts, you can efficiently clean up your file structure, enhancing both organization and performance.
In this article, we will explore the various methods available in PowerShell for deleting empty folders, from simple one-liners to more complex scripts. Whether you are a seasoned IT professional or a casual user, understanding how to leverage PowerShell for this task can
Understanding PowerShell Commands for Deleting Empty Folders
PowerShell provides a powerful way to manage files and folders within the Windows environment. When dealing with empty folders, it can be beneficial to automate their deletion to keep your directory structures clean and organized. Below are the key concepts and commands to achieve this.
Basic PowerShell Command Structure
To delete all empty folders using PowerShell, the `Get-ChildItem` cmdlet can be employed in conjunction with `Where-Object` and `Remove-Item`. The basic structure of the command is as follows:
“`powershell
Get-ChildItem -Path “C:\Your\Directory\Path” -Recurse -Directory | Where-Object { $_.GetFileSystemInfos().Count -eq 0 } | Remove-Item
“`
In this command:
- `Get-ChildItem`: Lists the items in the specified path.
- `-Path`: Specifies the directory to search within.
- `-Recurse`: Searches through all subdirectories.
- `-Directory`: Filters to include only directories.
- `Where-Object`: Filters out only those directories that are empty.
- `Remove-Item`: Deletes the empty directories.
Detailed Breakdown of the Command
To understand how the command works, let’s break it down further:
- Get-ChildItem: This command retrieves all items, including files and folders, from the specified directory. The use of `-Recurse` allows it to traverse through all levels of subdirectories.
- Filtering for Empty Folders: The `Where-Object` cmdlet checks each folder to see if it contains any files or subfolders. The condition `$_ .GetFileSystemInfos().Count -eq 0` ensures that only folders with no contents are considered.
- Removing Empty Folders: Finally, `Remove-Item` is executed on the filtered list to delete the empty directories.
Considerations Before Executing the Command
Before running the command, consider the following points:
- Backup Important Data: Ensure that no critical data resides in the folders being targeted.
- Test in a Safe Environment: Run the command in a controlled environment to validate its functionality.
- Permissions: Ensure you have the necessary permissions to delete folders in the specified directory.
Example Scenario
To illustrate, consider a directory structure like this:
“`
C:\Test\
│
├── Folder1
│ ├── Subfolder1
│ └── Subfolder2
│
├── Folder2
│
└── Folder3
├── Subfolder3
“`
If `Folder2` and `Subfolder2` were empty, running the command would result in the deletion of these folders.
Alternative Approach Using a Script
For users who frequently need to delete empty folders, creating a script can streamline the process. Below is an example of a simple PowerShell script:
“`powershell
$path = “C:\Your\Directory\Path”
Get-ChildItem -Path $path -Recurse -Directory | Where-Object { $_.GetFileSystemInfos().Count -eq 0 } | Remove-Item -Force
“`
This script can be saved as a `.ps1` file and executed whenever needed, automating the task of cleaning up empty directories.
Using PowerShell to delete empty folders is an efficient way to maintain a tidy file system. By understanding the command structure and considerations, users can effectively manage their directories and reduce clutter without manual intervention.
Using PowerShell to Delete All Empty Folders
To delete all empty folders in a specified directory using PowerShell, you can utilize a straightforward command. This command will search through the directory and remove any folders that do not contain files or subfolders.
PowerShell Command to Delete Empty Folders
You can execute the following command in PowerShell to remove empty directories:
“`powershell
Get-ChildItem -Path “C:\Your\Directory\Path” -Directory -Recurse | Where-Object { $_.GetFileSystemInfos().Count -eq 0 } | Remove-Item -Force
“`
Explanation of the Command:
- `Get-ChildItem`: This cmdlet retrieves the files and folders in the specified path.
- `-Path “C:\Your\Directory\Path”`: Replace this with the path to the directory you want to clean.
- `-Directory`: Ensures that only directories are considered.
- `-Recurse`: Searches through all subdirectories.
- `Where-Object { $_.GetFileSystemInfos().Count -eq 0 }`: Filters the results to only include empty folders.
- `Remove-Item -Force`: Deletes the identified empty folders without prompting for confirmation.
Considerations When Deleting Empty Folders
- Backup Important Data: Always ensure you have backups of your important data before executing deletion commands.
- Run as Administrator: Some folders may require administrative privileges to delete. Run PowerShell as an administrator if necessary.
- Confirm Deletion: It may be wise to initially run the command without the `Remove-Item` portion to confirm which folders will be deleted.
Command for Previewing Empty Folders
To preview the empty folders before deletion, you can modify the command as follows:
“`powershell
Get-ChildItem -Path “C:\Your\Directory\Path” -Directory -Recurse | Where-Object { $_.GetFileSystemInfos().Count -eq 0 }
“`
This command will list all empty folders without deleting them, allowing you to review the results.
Additional Options for Folder Deletion
If you want to restrict the deletion process to specific types of directories or add additional filters, consider the following options:
- Filter by Name: You can filter folders by specific names or patterns.
- Exclude Certain Paths: Use additional conditions in the `Where-Object` cmdlet to exclude certain directories from deletion.
Example with Name Filter
“`powershell
Get-ChildItem -Path “C:\Your\Directory\Path” -Directory -Recurse | Where-Object { $_.GetFileSystemInfos().Count -eq 0 -and $_.Name -ne “ExcludeThisFolder” } | Remove-Item -Force
“`
This command will delete all empty folders except those named “ExcludeThisFolder.”
Scheduling the Cleanup Task
To automate the process of deleting empty folders, you can schedule a PowerShell script to run at regular intervals using Task Scheduler.
- Create a PowerShell Script: Save the commands in a `.ps1` file.
- Use Task Scheduler: Set up a new task that triggers the script based on your preferred schedule.
Steps to Schedule the Script
- Open Task Scheduler.
- Create a new task and set up the trigger (e.g., daily, weekly).
- In the action, select “Start a program” and point it to `powershell.exe`.
- In the “Add arguments” field, enter `-File “C:\Path\To\YourScript.ps1″`.
By using these methods, you can efficiently manage and remove empty folders within your file system using PowerShell.
Expert Insights on Deleting Empty Folders with PowerShell
Jessica Lin (Senior Systems Administrator, Tech Solutions Inc.). “Using PowerShell to delete all empty folders is an efficient way to maintain a clean file system. The command ‘Get-ChildItem -Path
-Recurse | Where-Object { $_.PSIsContainer -and -not (Get-ChildItem -Path $_.FullName) } | Remove-Item’ effectively identifies and removes these folders without affecting any files.”
Michael Thompson (IT Security Consultant, CyberSafe Group). “While automating the deletion of empty folders with PowerShell can save time, it is crucial to ensure that no important directories are mistakenly classified as empty. Always conduct a review of the folders before executing the removal command to prevent data loss.”
Linda Garcia (Cloud Infrastructure Engineer, CloudTech Innovations). “PowerShell provides a robust solution for managing file systems, including the removal of empty folders. Implementing this in a scheduled task can help keep your directories organized without manual intervention, thus enhancing overall productivity.”
Frequently Asked Questions (FAQs)
How can I delete all empty folders using PowerShell?
You can delete all empty folders in a specified directory by using the command `Get-ChildItem -Path “C:\YourDirectory” -Directory -Recurse | Where-Object { $_.GetFileSystemInfos().Count -eq 0 } | Remove-Item`. Replace `”C:\YourDirectory”` with the path of your target directory.
Is it safe to delete empty folders with PowerShell?
Yes, deleting empty folders is generally safe as they do not contain any files. However, ensure that the folders are not required for any applications or system processes before deletion.
Can I preview the empty folders before deleting them?
Yes, you can preview the empty folders by running the command `Get-ChildItem -Path “C:\YourDirectory” -Directory -Recurse | Where-Object { $_.GetFileSystemInfos().Count -eq 0 }`. This will list all empty folders without deleting them.
What happens if I try to delete non-empty folders using this command?
The command provided will only target and delete folders that are empty. Non-empty folders will be unaffected and remain intact.
Can I delete empty folders in multiple directories at once?
Yes, you can delete empty folders in multiple directories by specifying multiple paths in the command. For example: `Get-ChildItem -Path “C:\Dir1”, “C:\Dir2” -Directory -Recurse | Where-Object { $_.GetFileSystemInfos().Count -eq 0 } | Remove-Item`.
Is there a way to confirm the deletion of empty folders in PowerShell?
You can add the `-WhatIf` parameter to the `Remove-Item` command, like this: `Remove-Item -WhatIf`. This will simulate the deletion process and show which folders would be deleted without actually removing them.
In summary, using PowerShell to delete all empty folders is a straightforward yet powerful task that can significantly streamline file management processes. By employing specific cmdlets such as `Get-ChildItem` and `Remove-Item`, users can effectively identify and remove empty directories within a specified path. This capability is particularly useful for maintaining organized file systems, especially in environments where numerous folders may be created and left unused over time.
Key takeaways from the discussion include the importance of understanding the command structure and the potential impact of using these commands. Users should always ensure they are targeting the correct directories to avoid unintentional data loss. Additionally, utilizing the `-Recurse` parameter allows for a comprehensive search through nested folders, enhancing the effectiveness of the cleanup process.
Furthermore, it is advisable to implement safety measures, such as running a test command that lists empty folders before executing the deletion command. This practice helps verify the results and prevents accidental removal of important directories. Overall, mastering these PowerShell commands can lead to more efficient file management and a cleaner digital workspace.
Author Profile
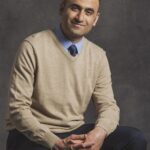
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?