How Can You Delete a Column in a Table Based on Specific Conditions?
In the world of data management, the ability to manipulate tables efficiently is crucial for maintaining clean and relevant datasets. One common task that database administrators and data analysts frequently encounter is the need to delete specific columns from a table based on certain conditions. This process can significantly streamline data analysis, enhance performance, and ensure that only the most pertinent information is retained. Whether you’re working with SQL databases, spreadsheets, or any other structured data format, understanding how to effectively delete columns with conditions can save you time and prevent potential errors.
When it comes to deleting columns conditionally, the approach can vary depending on the platform or programming language you are using. For instance, in SQL, you might leverage the power of conditional statements to identify which columns to remove based on their values or attributes. Similarly, in spreadsheet applications, you can utilize filters and scripts to automate the deletion process. This not only simplifies your workflow but also helps maintain the integrity of your data by ensuring that only relevant columns remain.
As we delve deeper into this topic, we will explore various methods and best practices for deleting columns in tables under specific conditions. From crafting precise SQL queries to employing advanced spreadsheet techniques, you’ll gain insights that will empower you to manage your data more effectively. Whether you’re a seasoned data professional
SQL Command for Deleting a Column
To delete a column from a table in SQL, the `ALTER TABLE` statement is utilized. However, it’s essential to know that SQL does not support conditional column deletion directly. Instead, you can remove a column entirely from a table structure. Here’s the syntax for deleting a column:
“`sql
ALTER TABLE table_name
DROP COLUMN column_name;
“`
This command will permanently remove the specified column from the table, along with all of its data.
Conditional Deletion Logic
While SQL does not allow for conditional deletion of columns directly, you can implement a logic that simulates this behavior by using a temporary table. The process involves:
- Creating a new table without the column you want to remove.
- Copying the data from the original table to the new table, excluding the column in question.
- Dropping the original table.
- Renaming the new table to the original table’s name.
Here’s a structured example:
“`sql
CREATE TABLE new_table AS
SELECT column1, column2 — Exclude column3
FROM original_table;
DROP TABLE original_table;
ALTER TABLE new_table RENAME TO original_table;
“`
Example Scenario
Consider a scenario where you have a table named `employees` with the following structure:
employee_id | first_name | last_name | department | salary |
---|---|---|---|---|
1 | John | Doe | HR | 50000 |
2 | Jane | Smith | IT | 60000 |
3 | Emily | Johnson | IT | 65000 |
If you wish to remove the `department` column, follow these steps.
- Create a new table without the `department` column.
“`sql
CREATE TABLE new_employees AS
SELECT employee_id, first_name, last_name, salary
FROM employees;
“`
- Drop the original `employees` table.
“`sql
DROP TABLE employees;
“`
- Rename the new table to `employees`.
“`sql
ALTER TABLE new_employees RENAME TO employees;
“`
Considerations When Deleting Columns
- Data Loss: Deleting a column will result in the loss of all data stored in that column. Ensure that you have backups if necessary.
- Constraints: Be aware of any constraints or dependencies associated with the column you are deleting. Removing a column that is part of a foreign key or unique constraint can cause errors.
- Database Version: Check the specific SQL syntax for your database management system (DBMS), as there may be variations.
In summary, while SQL does not allow for conditional deletion of columns directly, you can achieve a similar outcome by creating a new table without the unwanted column and transferring the relevant data. Always ensure you have a clear understanding of the implications of such operations on your database schema.
SQL Syntax for Deleting a Column with Conditions
In SQL, the direct deletion of a column based on a condition is not supported. The `ALTER TABLE` command can be used to drop a column, but conditions must be applied to the data itself rather than the structure of the table. However, you can use a workaround to achieve similar outcomes by creating a new table without the unwanted column or by removing data from that column based on certain conditions.
Dropping a Column
To drop a column from a table, use the following SQL syntax:
“`sql
ALTER TABLE table_name
DROP COLUMN column_name;
“`
This command will remove the specified column entirely from the table structure. Note that this operation cannot be undone, and you will lose all data in that column.
Deleting Data from a Column Based on Conditions
If the goal is to remove or nullify data from a specific column based on certain criteria, you can use the `UPDATE` statement to set the values to `NULL` or a default value. The following example illustrates this approach:
“`sql
UPDATE table_name
SET column_name = NULL
WHERE condition;
“`
Example:
Assuming you have a `users` table and you want to nullify the `email` column for users who are inactive, the query would look like:
“`sql
UPDATE users
SET email = NULL
WHERE status = ‘inactive’;
“`
Creating a New Table Without the Column
If you need to eliminate a column and keep the rest of the data intact, consider creating a new table that mirrors the existing table minus the unwanted column. Here is a step-by-step approach:
- Create a new table with the desired structure:
“`sql
CREATE TABLE new_table_name AS
SELECT column1, column2, column3 — exclude the column you want to delete
FROM existing_table_name;
“`
- Drop the old table if necessary:
“`sql
DROP TABLE existing_table_name;
“`
- Rename the new table to the original table name:
“`sql
ALTER TABLE new_table_name RENAME TO existing_table_name;
“`
Note:
- Ensure you have backups of your data before performing structural changes.
- This method can be beneficial for large tables, reducing downtime and enabling selective data migration.
Considerations When Deleting Columns
When contemplating the deletion of a column or data within a column, consider the following:
- Data Integrity: Ensure that removing the column does not violate any constraints or relationships in your database schema.
- Backups: Always have a backup strategy in place before making changes to your database structure.
- Performance: Dropping columns from very large tables can affect performance; plan to execute such operations during off-peak hours.
- Dependencies: Check for any dependencies such as views, stored procedures, or application code that may reference the column before removal.
Expert Insights on Deleting Columns in Tables with Conditions
Dr. Emily Chen (Data Management Specialist, Tech Innovations Inc.). “When considering the deletion of a column in a table based on specific conditions, it is crucial to assess the impact on data integrity and relationships within the database. A systematic approach, including backups and thorough testing, should be employed to prevent data loss.”
Michael Thompson (Database Administrator, Cloud Solutions Group). “Implementing conditional column deletions requires a clear understanding of SQL commands and their implications. Utilizing the ‘ALTER TABLE’ statement with a ‘WHERE’ clause can streamline the process, but one must ensure that the conditions are accurately defined to avoid unintended deletions.”
Sarah Patel (Software Engineer, Data Integrity Corp.). “In practice, deleting a column conditionally can lead to complications, especially in larger datasets. I recommend using a staging table to validate the conditions before executing the deletion in the main table, thereby safeguarding against potential errors.”
Frequently Asked Questions (FAQs)
How can I delete a column in a table with a specific condition?
To delete a column in a table based on a condition, you typically need to use a combination of SQL commands. First, identify the rows that meet your condition using a `DELETE` statement, and then use `ALTER TABLE` to drop the column if necessary.
Is it possible to delete a column without affecting other data in the table?
Yes, deleting a column using the `ALTER TABLE` statement will remove that specific column without affecting the remaining data in the table. However, ensure that you back up your data before performing this operation.
What SQL command is used to delete a column from a table?
The SQL command used to delete a column from a table is `ALTER TABLE table_name DROP COLUMN column_name;`. This command will remove the specified column from the table structure.
Can I delete multiple columns at once based on a condition?
You cannot delete multiple columns based on a condition directly in a single command. You must first delete the rows that meet your condition and then drop the columns separately using multiple `ALTER TABLE` commands.
What happens to the data in a column when it is deleted?
When a column is deleted, all data contained within that column is permanently removed from the table. This action cannot be undone unless a backup is available.
Are there any risks associated with deleting a column in a database?
Yes, risks include losing important data, breaking existing queries or applications that rely on the column, and potential data integrity issues. Always ensure proper backups and review dependencies before proceeding with the deletion.
In the realm of database management, the ability to delete a column in a table based on specific conditions is a crucial operation. This process typically involves the use of SQL commands, particularly the `ALTER TABLE` statement combined with a `WHERE` clause to specify the conditions under which the column should be removed. Understanding the syntax and implications of this operation is essential for maintaining data integrity and ensuring that the database functions as intended.
Key insights into this process highlight the importance of careful planning before executing such commands. Deleting a column can lead to loss of data, and therefore, it is advisable to back up the database prior to making any alterations. Additionally, one must consider the dependencies and relationships that the column may have with other tables or applications to avoid unintended consequences.
Moreover, it is important to note that not all database systems support conditional deletion of columns in the same way. Some may require additional steps or different syntax to achieve the desired outcome. Therefore, familiarity with the specific database management system in use is vital for executing these operations effectively and safely.
Author Profile
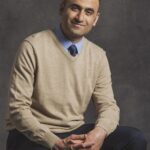
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?