How Can You Rearrange DataFrame Columns to Match a Specific Order of Rows?
In the world of data manipulation and analysis, the ability to rearrange and organize information efficiently is crucial. As datasets grow in complexity, so does the need for effective tools and techniques to streamline data presentation. One common task that analysts often face is rearranging the order of rows in a DataFrame based on a specific list or criteria. This seemingly simple operation can greatly enhance the clarity and usability of data, allowing for more insightful analysis and decision-making.
When working with DataFrames in programming languages like Python, particularly with libraries such as Pandas, the process of rearranging rows can be both intuitive and powerful. By utilizing a predefined list to dictate the order of rows, analysts can ensure that their data is not only organized but also aligned with their analytical goals. This method not only saves time but also reduces the potential for errors that can arise from manual rearrangement.
As we delve deeper into the techniques and functions available for rearranging DataFrame rows, we will explore various approaches, including indexing and sorting methods. Whether you’re a seasoned data analyst or just starting your journey, mastering the art of row rearrangement will undoubtedly enhance your data manipulation skills and empower you to present your findings with greater impact.
Rearranging DataFrame Columns Based on a List
When working with pandas DataFrames in Python, there are times when you may need to rearrange the order of the columns according to a predefined list. This is particularly useful for ensuring consistency in data representation or for meeting specific formatting requirements. The process is straightforward and can be achieved with minimal code.
To rearrange the columns of a DataFrame, you can simply use the list of desired column names to index the DataFrame. Here’s how you can do it:
“`python
import pandas as pd
Example DataFrame
data = {
‘B’: [1, 2, 3],
‘A’: [4, 5, 6],
‘C’: [7, 8, 9]
}
df = pd.DataFrame(data)
Desired order of columns
column_order = [‘A’, ‘B’, ‘C’]
Rearranging columns
df = df[column_order]
“`
In this example, the DataFrame `df` originally has columns in the order of `B`, `A`, `C`. By specifying `column_order`, the DataFrame is rearranged to display columns in the order of `A`, `B`, `C`.
Considerations When Rearranging Columns
While rearranging columns is straightforward, there are several important considerations to keep in mind:
- Column Existence: Ensure that all the column names in your list exist in the DataFrame. If a column does not exist, pandas will raise a `KeyError`.
- Order Sensitivity: The order specified in the list will be strictly followed. Any columns not included in the list will be dropped from the DataFrame.
- Performance: For large DataFrames, rearranging columns can lead to performance overhead. It is advisable to minimize such operations in performance-critical applications.
Example of Rearranging Columns with Missing Values
In scenarios where some columns might be missing from the DataFrame, it is beneficial to filter the list to include only those columns that exist in the DataFrame. This can prevent errors and ensure that your DataFrame remains intact.
“`python
Desired order of columns with potential missing columns
column_order = [‘A’, ‘D’, ‘B’, ‘C’] ‘D’ does not exist
Filter column order based on existing columns in the DataFrame
valid_columns = [col for col in column_order if col in df.columns]
Rearranging columns with valid columns only
df = df[valid_columns]
“`
This approach creates a list of valid columns and rearranges the DataFrame without raising errors.
Table Example of Column Rearrangement
To further illustrate the concept, the following table demonstrates the original and rearranged DataFrames.
Original DataFrame | Rearranged DataFrame |
---|---|
B A C 0 1 4 7 1 2 5 8 2 3 6 9 |
A B C 0 4 1 7 1 5 2 8 2 6 3 9 |
This table clearly shows how the columns are rearranged from their original order to the specified order, enhancing clarity and usability of the data.
Rearranging DataFrame Columns in Python
In Python, particularly using the pandas library, rearranging the order of columns in a DataFrame can be achieved easily by utilizing a list that specifies the desired order. This approach allows for flexibility and clarity in data manipulation.
Steps to Rearrange DataFrame Columns
- Import the pandas library:
Ensure you have the pandas library imported in your script to work with DataFrames.
“`python
import pandas as pd
“`
- Create or load your DataFrame:
You can either create a DataFrame from scratch or load one from a data source.
“`python
data = {
‘A’: [1, 2, 3],
‘B’: [4, 5, 6],
‘C’: [7, 8, 9]
}
df = pd.DataFrame(data)
“`
- Define the desired column order:
Create a list that specifies the order in which you want the columns to appear.
“`python
new_order = [‘C’, ‘A’, ‘B’]
“`
- Rearrange the DataFrame:
Use the list to reorder the columns of the DataFrame.
“`python
df = df[new_order]
“`
- Display the rearranged DataFrame:
You can print or visualize the DataFrame to confirm the changes.
“`python
print(df)
“`
Example of Column Rearrangement
Below is a complete example illustrating how to rearrange the columns in a DataFrame.
“`python
import pandas as pd
Create a sample DataFrame
data = {
‘A’: [1, 2, 3],
‘B’: [4, 5, 6],
‘C’: [7, 8, 9]
}
df = pd.DataFrame(data)
Define the new order of columns
new_order = [‘C’, ‘A’, ‘B’]
Rearrange the DataFrame
df = df[new_order]
Output the rearranged DataFrame
print(df)
“`
The output will show the DataFrame with columns rearranged as follows:
“`
C A B
0 7 1 4
1 8 2 5
2 9 3 6
“`
Rearranging Rows Based on a List
To rearrange the order of rows in a DataFrame based on a specific list, the process is similar but focuses on the index rather than columns.
- Define the desired row order:
Create a list that specifies the desired row order based on their index.
“`python
new_row_order = [2, 0, 1] New order of indices
“`
- Rearrange the DataFrame rows:
Use the list to reorder the rows of the DataFrame.
“`python
df = df.iloc[new_row_order]
“`
- Display the rearranged DataFrame:
Print or visualize the DataFrame to confirm the changes.
“`python
Rearrange the DataFrame rows
df = df.iloc[new_row_order]
Output the rearranged DataFrame
print(df)
“`
The output will display the DataFrame with rows rearranged according to the specified order.
Rearranging columns and rows in pandas DataFrames is straightforward and can significantly enhance data organization and analysis efficiency. By following the steps outlined above, users can quickly manipulate their DataFrames to suit their analytical needs.
Expert Insights on Rearranging DataFrame Columns in Python
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “Rearranging DataFrame columns based on a specific order can significantly enhance data readability and analysis. Utilizing the `reindex` method in pandas allows for a straightforward approach to achieve this, ensuring that the DataFrame aligns with the desired structure.”
Michael Chen (Senior Software Engineer, Data Solutions Group). “When working with large datasets, maintaining an organized column order is crucial for efficient data manipulation. I recommend creating a list of the desired column order and applying it directly to the DataFrame to streamline subsequent operations.”
Lisa Patel (Machine Learning Specialist, AI Analytics Corp.). “Rearranging DataFrame columns not only improves clarity but also aids in feature selection for machine learning models. It is essential to ensure that the order reflects the importance of the features in relation to the target variable.”
Frequently Asked Questions (FAQs)
How can I rearrange the order of DataFrame columns in Python?
To rearrange the order of DataFrame columns in Python, you can use the indexing method by passing a list of the desired column order to the DataFrame. For example, `df = df[[‘column1’, ‘column3’, ‘column2’]]` will rearrange the columns accordingly.
Is it possible to reorder rows in a DataFrame based on a specific list?
Yes, you can reorder rows in a DataFrame based on a specific list by using the `loc` indexer. For instance, if you have a list of indices, you can select the rows with `df = df.loc[your_list]`, where `your_list` contains the desired order of row indices.
What method can I use to sort a DataFrame by multiple columns?
To sort a DataFrame by multiple columns, you can use the `sort_values()` method. For example, `df.sort_values(by=[‘column1’, ‘column2’], ascending=[True, ])` will sort the DataFrame first by `column1` in ascending order and then by `column2` in descending order.
Can I rearrange both rows and columns simultaneously in a DataFrame?
Yes, you can rearrange both rows and columns simultaneously by first reordering the columns and then applying the row reordering. For example, you can perform `df = df.loc[your_row_list, [‘column1’, ‘column2’]]` to achieve this.
What happens if the list used for reordering rows contains indices that do not exist in the DataFrame?
If the list used for reordering rows contains indices that do not exist in the DataFrame, a `KeyError` will be raised. It is important to ensure that all indices in the list are valid and present in the DataFrame.
Can I use a condition to filter and rearrange rows in a DataFrame?
Yes, you can use conditions to filter and rearrange rows in a DataFrame. By applying boolean indexing, you can create a new DataFrame that meets specific criteria and then reorder it as needed. For example, `df_filtered = df[df[‘column’] > value]` followed by reordering the filtered DataFrame.
Rearranging the order of rows in a DataFrame based on a specified list of column values is a common task in data manipulation. This process allows users to organize their data in a manner that aligns with specific analytical needs or presentation requirements. By leveraging libraries such as pandas in Python, users can efficiently reorder rows to match a predefined sequence, enhancing data readability and interpretability.
To achieve this, one can utilize the `pd.Categorical` method to create a categorical type based on the desired order. This method ensures that the DataFrame recognizes the specified order when sorting. Additionally, the `sort_values` function can be employed to rearrange the DataFrame rows according to the categorical order, resulting in a structured dataset that reflects the intended hierarchy or sequence.
Key takeaways from this discussion include the importance of understanding the underlying data structure and the tools available for data manipulation. Mastery of these techniques not only streamlines data analysis but also enriches the quality of insights derived from the data. As data-driven decision-making continues to grow in significance, the ability to customize data presentation through row rearrangement becomes an invaluable skill for analysts and data scientists alike.
Author Profile
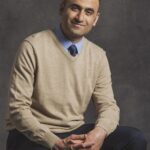
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?