Why Am I Getting ‘django.core.exceptions.AppRegistryNotReady: Apps Aren’t Loaded Yet’ Error in Django?
In the dynamic world of web development, Django stands out as a powerful framework that streamlines the creation of robust applications. However, even seasoned developers can encounter perplexing errors that disrupt their workflow. One such error, `django.core.exceptions.AppRegistryNotReady: Apps aren’t loaded yet.`, can leave many scratching their heads in confusion. This error serves as a reminder of the intricacies involved in Django’s app loading mechanism and the importance of understanding its lifecycle. In this article, we will delve into the causes and implications of this error, providing clarity and guidance for developers navigating the Django landscape.
At its core, the `AppRegistryNotReady` error signifies that Django’s application registry is not fully initialized when certain operations are attempted. This can occur during various stages of development, particularly when dealing with model imports or signals before the app registry is ready. Understanding the timing and context in which Django initializes its apps is crucial for preventing this error from surfacing.
Moreover, the implications of encountering this error extend beyond mere annoyance; they can lead to significant delays in development and debugging processes. By exploring the common scenarios that trigger this issue and the best practices for avoiding it, developers can enhance their proficiency with Django and ensure smoother project execution. Join us as we unravel the complexities of
Understanding the Error
The error message `django.core.exceptions.AppRegistryNotReady: Apps aren’t loaded yet.` typically indicates that there is an attempt to use Django applications before the application registry has been fully initialized. This can occur in various situations, most commonly during the startup of the Django server or when executing management commands.
A few key scenarios that can lead to this error include:
- Accessing models or database queries too early in the application lifecycle.
- Importing models in a module that is executed before the Django app registry is ready.
- Misconfigured settings or improper import statements in the project.
Common Causes
Several factors can contribute to this error. Understanding these causes can help in troubleshooting and resolving the issue effectively. Here are some common causes:
- Improper Imports: Importing models or Django-specific components in files that are executed on application load can lead to this error.
- Management Commands: If a custom management command tries to access models before the apps are loaded, this error will occur.
- Signals: Connecting signals in a module that loads before the app registry is ready can trigger this error.
- Circular Imports: Circular dependencies between modules can lead to situations where the app registry isn’t ready when you try to access models.
Troubleshooting Steps
To resolve the `AppRegistryNotReady` error, consider the following troubleshooting steps:
- Check Import Locations: Ensure that model imports are done after Django has fully loaded. Avoid importing models at the top level of modules that are executed at startup.
- Use AppConfig: If you’re working with signals, consider connecting them in the `ready()` method of your `AppConfig` class. This ensures that the application is fully loaded before signals are connected.
- Management Command Adjustments: If you’re writing custom management commands, ensure you are using `django.setup()` correctly to initialize the Django environment.
- Review Settings: Check the `INSTALLED_APPS` setting in your Django settings file to ensure all apps are correctly listed and configured.
Here’s a small table summarizing common causes and their solutions:
Cause | Solution |
---|---|
Improper Imports | Delay imports until after the application has started. |
Management Commands | Use `django.setup()` in your management command to ensure apps are loaded. |
Signals | Connect signals in the `ready()` method of `AppConfig`. |
Circular Imports | Refactor code to eliminate circular dependencies. |
Best Practices
To prevent the `AppRegistryNotReady` error from occurring in the first place, adhering to best practices during development is crucial. Here are some recommended practices:
- Organize Code Structure: Keep imports organized and import models only where necessary, ideally within functions or methods rather than at the module level.
- Use Django’s AppConfig: Always use Django’s `AppConfig` to define application configurations, which can help manage the lifecycle of your application better.
- Testing and Debugging: Use Django’s testing framework to catch these issues early. Writing tests that access models can help you identify import-related problems.
By following these guidelines and understanding the intricacies of Django’s application loading process, developers can minimize the chances of encountering the `AppRegistryNotReady` error in their projects.
Understanding the Error
The error message `django.core.exceptions.AppRegistryNotReady: Apps aren’t loaded yet.` typically occurs when you attempt to access models or perform database operations before Django has fully initialized its application registry. This situation often arises during startup, particularly when importing models or executing code that requires access to the ORM (Object-Relational Mapping) layer.
Key points to understand about this error include:
- Timing of Execution: The error signifies that the Django application is not yet ready to handle database operations. This can happen if you run code in the global scope of a module that imports models or interacts with the database.
- Application Registry: Django’s application registry is responsible for keeping track of all installed apps and their models. Accessing any part of this registry before it is fully prepared will trigger the error.
Common Causes
Several common scenarios can lead to this error:
- Improper Imports:
- Importing models at the top level of a module instead of within functions or methods.
- Signal Handlers:
- Defining signal handlers that attempt to access models during the startup phase can cause this error.
- Management Commands:
- Writing custom management commands that access the database without ensuring the app registry is ready.
- Testing Environment:
- Running tests that rely on the database before the test environment is fully set up.
Best Practices to Avoid the Error
To mitigate the risk of encountering the `AppRegistryNotReady` error, consider the following best practices:
- Delay Model Imports:
- Always import models within functions or methods rather than at the module level. This ensures that imports occur only after the application registry is loaded.
“`python
def my_function():
from myapp.models import MyModel
Proceed with database operations
“`
- Use AppConfig:
- If you need to execute code when an app is ready, utilize the `ready()` method of your app’s `AppConfig` class.
“`python
from django.apps import AppConfig
class MyAppConfig(AppConfig):
name = ‘myapp’
def ready(self):
from . import signals Import signals here
“`
- Check Database Access:
- Ensure that any database access happens after Django has fully initialized, especially in scripts and management commands.
Debugging Steps
If you encounter this error, follow these debugging steps:
- Review Import Statements: Check your import statements and ensure no models are imported at the module level.
- Check Signal Definitions: Inspect signal handlers for any model access that might occur during startup.
- Utilize Django Shell: Use the Django shell to test model access. If the error occurs here, it indicates issues with your project setup.
- Examine Settings: Ensure that all apps are correctly listed in the `INSTALLED_APPS` setting.
- Run Migrations: Make sure all migrations are applied and the database is in sync with the models.
Example Scenarios
Scenario | Description |
---|---|
Importing Models Globally | Accessing model classes at the module level leads to premature access before the app registry loads. |
Signal Handling During Initialization | Signals that execute database queries too early can trigger the error. |
Custom Management Command Execution | If a management command interacts with models before the app is ready, it will raise the error. |
Testing Without Proper Setup | Running tests that interact with models before Django initializes can lead to the error. |
Understanding the Django App Registry Issue
Dr. Emily Chen (Senior Software Engineer, Django Development Team). “The error ‘django.core.exceptions.AppRegistryNotReady: Apps aren’t loaded yet’ typically arises when you attempt to access models or other components before the Django application is fully initialized. It’s crucial to ensure that your code is executed at the right time in the application lifecycle.”
Mark Thompson (Lead Architect, Web Framework Solutions). “This error often indicates a misconfiguration in your Django settings or an issue with the import order of your applications. Developers should be cautious about where they place import statements to avoid premature access to models.”
Lisa Patel (Django Consultant, CodeCrafters Inc.). “To resolve the ‘Apps aren’t loaded yet’ error, I recommend checking the initialization sequence of your apps and ensuring that any code dependent on models is encapsulated within functions or classes that are called after Django has completed its startup process.”
Frequently Asked Questions (FAQs)
What does the error “django.core.exceptions.AppRegistryNotReady: Apps aren’t loaded yet” mean?
This error indicates that Django is attempting to access application models or other components before the application registry has been fully initialized. This typically occurs when code that relies on models is executed too early in the startup process.
What are common causes for encountering this error in Django?
Common causes include importing models or accessing the database in the global scope of a module, running code in the wrong lifecycle method, or misconfigurations in the Django settings that prevent proper app loading.
How can I resolve the “Apps aren’t loaded yet” error?
To resolve this error, ensure that any code that interacts with models is executed after Django has fully initialized. This can be done by placing such code inside functions or classes that are called after the application is ready, such as using the `ready()` method in an AppConfig class.
What is the role of the AppConfig class in Django?
The AppConfig class is responsible for configuring application-specific settings and behavior. It allows you to define initialization code that runs when the application starts, ensuring that models and other components are loaded correctly.
Can this error occur during testing in Django?
Yes, this error can occur during testing if test cases attempt to access models or database functionality before the Django test framework has fully initialized the application. Properly structuring test case setup methods can help avoid this issue.
Is there a way to prevent this error from happening in the future?
To prevent this error, adhere to best practices by avoiding model imports at the module level and using Django’s signal framework or the `ready()` method in AppConfig for any initialization code that requires access to models.
The error message “django.core.exceptions.AppRegistryNotReady: Apps aren’t loaded yet” typically arises in Django when there is an attempt to access application models or perform database queries before the Django application is fully initialized. This situation often occurs in scenarios such as running scripts, importing models prematurely, or executing code in the wrong order during the startup phase of the application. Understanding the lifecycle of a Django application is crucial to avoid this issue.
One of the primary reasons for encountering this error is the improper placement of import statements. When models are imported at the module level in a script or a management command, it can lead to this error if the application registry is not yet ready. It is essential to ensure that any code interacting with models is executed after the Django application has been fully loaded, typically within the context of a function or within the `ready` method of an application configuration class.
Another key takeaway is the importance of using Django’s built-in mechanisms for managing application readiness. Utilizing signals, particularly the `ready` method in the `AppConfig` class, allows developers to run code only after all applications are loaded. Additionally, developers should be cautious about circular imports, as they can also contribute to this error. Proper structuring of imports
Author Profile
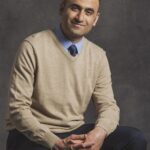
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?