Is ‘Do Nothing’ Python the Secret to Simplifying Your Code?
In the world of programming, efficiency and clarity are paramount. Yet, amidst the hustle of coding, there are moments when the best course of action is to simply pause and do nothing. This might sound counterintuitive, especially in a fast-paced environment where every line of code is expected to perform a task. However, in Python, the concept of “doing nothing” can be an intentional and powerful tool, allowing developers to create cleaner, more maintainable code. In this article, we will explore the nuances of this intriguing concept, revealing how it can enhance your programming practices and lead to more robust applications.
When we talk about “doing nothing” in Python, we are often referring to specific constructs and techniques that allow a program to effectively handle situations where no action is required. This can include the use of placeholders, no-op functions, or even strategic pauses in execution. Understanding when and how to implement these techniques can help developers avoid unnecessary complexity, streamline their code, and improve overall readability.
Moreover, the practice of consciously choosing to do nothing can also play a significant role in error handling and control flow. By recognizing scenarios where no operation is needed, programmers can prevent potential pitfalls and ensure that their applications run smoothly. As we delve deeper into this topic,
Understanding Do Nothing in Python
The concept of “doing nothing” in Python can refer to various scenarios where a block of code is intentionally left empty or where a function is defined but does not perform any action. This is often useful in situations where a placeholder is needed, or when implementing interfaces where certain methods are required but do not need to perform any operations.
In Python, the `pass` statement is commonly used to indicate that no action is to be taken. It serves as a syntactical placeholder and can be employed in several contexts, such as within loops, conditionals, or function definitions.
Usage of Pass Statement
The `pass` statement allows the programmer to create minimalistic structures without triggering errors. Here are some scenarios where `pass` might be used:
- Empty Functions: When a function is defined but not yet implemented.
- Control Flow Structures: When a specific case in an if statement does not require any action.
- Classes: For defining a class with no attributes or methods.
Example code snippets illustrate these usages:
“`python
def empty_function():
pass
if condition:
pass Placeholder for future code
class EmptyClass:
pass
“`
Practical Applications of Doing Nothing
While it may seem counterintuitive, there are practical applications for doing nothing in Python programming:
- Stubs for Future Development: Developers can outline functions or classes to be fleshed out later.
- Conditional Logic: In complex logic flows, certain conditions may not require any action, and `pass` can maintain clarity.
- Maintaining Code Structure: It helps in maintaining a consistent code structure while waiting for further implementation or decisions.
Context | Code Example | Purpose |
---|---|---|
Function Definition | def future_feature(): |
Placeholder for future implementation |
If Statement | if value > 10: |
Do nothing if condition is met |
Class Definition | class Feature: |
Define a class without attributes or methods |
Considerations When Using Do Nothing
While using the `pass` statement can be beneficial, it is essential to consider the following:
- Readability: Excessive use of `pass` can lead to confusion about the code’s intent. It is advisable to comment on the purpose of the placeholder.
- Code Maintenance: Ensure that the placeholders are revisited and implemented to avoid leaving unfinished code in production.
- Performance: While `pass` has negligible performance implications, redundant placeholders can lead to cluttered codebases.
In summary, the ability to “do nothing” in Python, primarily through the use of the `pass` statement, provides flexibility in coding practices, allowing for structured yet incomplete implementations.
Understanding the `do nothing` Concept in Python
In Python, the concept of “doing nothing” can be represented in various contexts, primarily with the use of the `pass` statement. This statement acts as a placeholder in situations where syntactically some action is required but no action is desired.
Using the `pass` Statement
The `pass` statement allows developers to write code that intentionally does nothing. It can be useful in several scenarios, such as:
- Function Definitions: When a function is defined but not yet implemented.
- Control Structures: In `if`, `for`, or `while` statements where a block of code is syntactically necessary but not required.
- Class Definitions: When defining a class that has yet to be filled with properties or methods.
Example of `pass` in a function:
“`python
def my_function():
pass
“`
Example in a control structure:
“`python
for i in range(10):
if i % 2 == 0:
pass Do nothing for even numbers
else:
print(i)
“`
Alternative Ways to ‘Do Nothing’
In addition to `pass`, there are other methods to create a no-operation effect in Python:
- Empty Functions: Just like `pass`, an empty function can be defined, but it is less explicit.
“`python
def empty_function():
return
“`
- Using `None`: Assigning `None` to a variable or returning `None` from a function can imply “no operation” in certain logical flows.
- Commented Out Code: Sometimes, developers use comments to indicate that certain code is intentionally not executed. However, this should be used judiciously to avoid clutter.
Performance Considerations
While using `pass` or other no-op patterns, it’s essential to consider performance implications in larger applications. The overhead of executing a no-op is negligible, but frequent use of empty constructs can lead to less readable and maintainable code.
Statement Type | Example | Purpose |
---|---|---|
`pass` | `if condition: pass` | Placeholder in control flow |
Empty Function | `def noop(): pass` | Function defined with no action |
Return `None` | `return None` | Implicit no operation |
Best Practices
When implementing the `do nothing` concept in Python, adhere to the following best practices:
- Clarity: Use `pass` for clarity when a block is intentionally left empty.
- Comments: Utilize comments to explain why a no-op is in place to enhance code readability.
- Limit Usage: Avoid overusing empty constructs which could lead to confusion in understanding code flow.
Applying these best practices will ensure that the intention behind “doing nothing” in code remains clear and comprehensible to all developers who may work with it in the future.
Understanding the Implications of “Do Nothing” in Python Programming
Dr. Emily Carter (Senior Software Engineer, Python Development Group). “The concept of ‘doing nothing’ in Python, often represented by the ‘pass’ statement, is crucial for maintaining code structure. It allows developers to outline functions or control structures without implementing logic immediately, facilitating incremental development.”
Mark Thompson (Lead Python Instructor, Code Academy). “In educational settings, teaching the ‘do nothing’ approach helps beginners understand the flow of control in programming. It emphasizes the importance of placeholders in code, which can be filled in later, thus promoting better coding practices.”
Linda Zhao (Software Architect, Tech Innovations Inc.). “Using ‘do nothing’ statements effectively can enhance readability and maintainability of code. It signals to other developers that a section is intentionally left blank for future implementation, thereby improving collaboration in team environments.”
Frequently Asked Questions (FAQs)
What does “do nothing” mean in Python?
“Do nothing” in Python typically refers to a situation where a function or block of code is defined but does not perform any actions. This can be achieved using the `pass` statement, which acts as a placeholder.
How can I create a “do nothing” function in Python?
You can create a “do nothing” function by defining a function that contains only the `pass` statement. For example:
“`python
def do_nothing():
pass
“`
When would I use a “do nothing” function?
A “do nothing” function can be useful during the development process when you want to define a function’s interface without implementing its functionality immediately. It helps in maintaining code structure.
Is there a built-in way to represent a “do nothing” action in Python?
Yes, the `pass` statement is the built-in way to represent a “do nothing” action in Python. It allows you to write syntactically correct code without executing any operation.
Can “do nothing” be used in exception handling?
Yes, “do nothing” can be used in exception handling by catching exceptions and using `pass` in the except block. This will suppress the error without any action taken. However, it is generally advisable to handle exceptions appropriately.
Are there performance implications of using “do nothing” in Python?
Using “do nothing” constructs like `pass` has negligible performance implications. However, excessive use of such constructs without proper handling may lead to code that is difficult to maintain or understand.
In the realm of Python programming, the concept of “doing nothing” can be represented through various constructs, such as the `pass` statement, the `None` object, and the use of empty functions or classes. The `pass` statement serves as a placeholder in situations where syntactically some code is required, but no action is necessary. This allows developers to maintain the structure of their code without implementing functionality at that moment, which can be particularly useful during the initial stages of development or when outlining a program’s architecture.
Additionally, the `None` object plays a crucial role in Python as it signifies the absence of a value or a null state. This can be particularly useful in function definitions where a return value is not required, or in cases where a variable may not yet hold a meaningful value. Understanding how to effectively utilize these constructs can lead to clearer, more maintainable code, as it allows developers to explicitly indicate their intentions within the codebase.
Moreover, employing “do nothing” techniques can enhance debugging and testing processes. By using placeholders and null values, developers can focus on the broader structure of their applications without being bogged down by the implementation details. This approach not only streamlines the development workflow but also
Author Profile
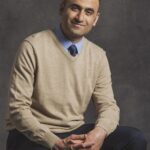
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?