What is a Do While Loop in JavaScript and How Does It Work?
### Introduction
In the realm of programming, loops are the lifeblood of efficient code, allowing developers to execute repetitive tasks with ease. Among the various looping constructs available in JavaScript, the `do while` loop stands out for its unique structure and functionality. Unlike its counterparts, the `do while` loop guarantees that the code block will run at least once, making it an invaluable tool for scenarios where initial execution is critical. Whether you’re a seasoned developer or just beginning your journey into the world of JavaScript, understanding the nuances of the `do while` loop can enhance your coding prowess and streamline your programming tasks.
The `do while` loop operates on a simple yet powerful principle: it executes a block of code and then evaluates a condition to determine whether to repeat the process. This characteristic distinguishes it from other loops, such as the `for` and `while` loops, which check conditions before executing their code blocks. As a result, the `do while` loop is particularly useful in situations where user input is required, or when you need to ensure that a specific operation occurs at least once before any conditions are applied.
As we delve deeper into the mechanics of the `do while` loop, we will explore its syntax, practical applications, and best practices
Understanding the Structure of a do…while Loop
The `do…while` loop in JavaScript provides a way to execute a block of code at least once, regardless of the condition specified. Its structure ensures that the loop’s body is executed before evaluating the condition, which distinguishes it from the traditional `while` loop.
The syntax of a `do…while` loop is as follows:
javascript
do {
// code block to be executed
} while (condition);
In this structure:
- The code block inside the `do` section runs once before the condition is checked.
- After executing the block, the `while` condition is evaluated.
- If the condition evaluates to `true`, the loop continues to execute; if “, the loop terminates.
Example of a do…while Loop
Here is a simple example demonstrating the use of a `do…while` loop:
javascript
let count = 0;
do {
console.log(“Count is: ” + count);
count++;
} while (count < 5);
In this example, the loop will print the count from 0 to 4. The output will be:
Count is: 0
Count is: 1
Count is: 2
Count is: 3
Count is: 4
This illustrates that the loop executes the console log statement before checking if `count` is less than 5.
Key Characteristics of do…while Loops
- Guaranteed Execution: The loop body runs at least once even if the condition is from the start.
- Post-Condition Evaluation: The condition is evaluated after the code block execution, which can be advantageous in scenarios where an initial operation is required.
Comparative Analysis of Loop Structures
To further understand the `do…while` loop, it can be useful to compare it to other loop constructs like `while` and `for` loops.
Loop Type | Execution Guarantee | Condition Check Timing |
---|---|---|
do…while | At least once | After loop body execution |
while | Zero or more times | Before loop body execution |
for | Zero or more times | Before loop body execution |
This table highlights the differences in execution guarantees and timing of condition checks between the various loop types, emphasizing the unique nature of `do…while`.
Common Use Cases for do…while Loops
The `do…while` loop is particularly useful in scenarios where the initial execution of the loop is necessary, regardless of the condition. Some common use cases include:
- User Input Validation: Prompting users for input until valid data is provided.
- Menu-Driven Programs: Displaying a menu and executing the selection until the user opts to exit.
- Repetitive Tasks: Performing a task that must be executed at least once, such as loading a resource or initializing a value.
Utilizing the `do…while` loop in these situations can lead to clearer and more concise code, enhancing readability and maintainability.
Understanding the Syntax of a Do While Loop
The `do while` loop in JavaScript is a control flow statement that executes a block of code at least once before checking a condition at the end of the loop. The basic syntax is as follows:
javascript
do {
// Code to be executed
} while (condition);
### Key Components:
- do: This keyword indicates the start of the loop.
- Code Block: The statements that you want to execute.
- while (condition): The condition is evaluated after the code block is executed. If true, the loop will repeat.
Example of a Do While Loop
Consider the following example, which prompts the user to input a number until they enter a number greater than 10:
javascript
let number;
do {
number = prompt(“Enter a number greater than 10:”);
} while (number <= 10);
In this example:
- The user will be prompted at least once to enter a number.
- The loop continues until the user enters a number greater than 10.
Comparison with Other Loop Types
Feature | Do While Loop | While Loop | For Loop |
---|---|---|---|
Minimum Executions | At least once | Zero or more | Zero or more |
Condition Check Location | End of the loop | Beginning of the loop | Beginning of the loop |
Syntax Complexity | Simple | Moderate | Moderate |
### Advantages of Do While Loops:
- Guarantees that the code block runs at least once.
- Useful when the initial condition is not required to execute the code.
### Disadvantages:
- Potential for infinite loops if the condition is not updated correctly.
- Less commonly used than `for` or `while` loops, which may affect readability in some cases.
Common Use Cases
Do while loops are particularly useful in scenarios where:
- User input is needed, and you want to ensure at least one prompt.
- You need to validate conditions after executing a block of code.
- You are implementing retry mechanisms, such as asking users to re-enter values upon invalid input.
Best Practices
- Ensure the condition in the `while` statement eventually becomes to avoid infinite loops.
- Use descriptive variable names to enhance code readability.
- Comment your code to clarify the purpose of the loop and its exit condition.
By adhering to these best practices, developers can leverage `do while` loops effectively within their JavaScript code, ensuring both functionality and maintainability.
Expert Insights on the Do While Loop in JavaScript
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The do while loop in JavaScript is particularly useful when you need to ensure that a block of code runs at least once, regardless of the condition. This characteristic makes it ideal for scenarios such as user input validation, where you want to prompt the user until valid input is received.”
Michael Chen (JavaScript Framework Developer, CodeCraft Solutions). “One of the advantages of the do while loop is its simplicity in syntax and structure. It allows developers to write cleaner code when the initial execution of the loop is necessary. However, it is crucial to manage the loop’s exit condition carefully to avoid infinite loops.”
Sarah Thompson (Lead JavaScript Instructor, Web Development Academy). “In teaching JavaScript, I emphasize the importance of understanding the difference between the do while loop and other looping constructs. The do while loop’s behavior can lead to unexpected results for beginners if they do not grasp how and when the condition is evaluated.”
Frequently Asked Questions (FAQs)
What is a do while loop in JavaScript?
A do while loop in JavaScript is a control structure that executes a block of code at least once and then repeatedly executes the block as long as a specified condition evaluates to true.
How does a do while loop differ from a while loop?
The primary difference is that a do while loop guarantees at least one execution of the loop’s body, whereas a while loop checks the condition before executing the loop, which may result in zero executions if the condition is initially.
What is the syntax of a do while loop in JavaScript?
The syntax is as follows:
javascript
do {
// code to be executed
} while (condition);
The code inside the do block executes once before the condition is evaluated.
Can you provide an example of a do while loop?
Certainly. Here is an example:
javascript
let count = 0;
do {
console.log(count);
count++;
} while (count < 5);
This code will output numbers 0 through 4 to the console.
When should I use a do while loop instead of other loops?
Use a do while loop when you need to ensure that the loop body is executed at least once, such as when prompting a user for input or performing an action that must occur before any condition is checked.
Are there any potential pitfalls when using a do while loop?
Yes, a common pitfall is creating an infinite loop if the condition never evaluates to . It is crucial to ensure that the loop’s condition will eventually become to avoid unintentional infinite execution.
The “do while” loop in JavaScript is a control flow statement that allows for the execution of a block of code at least once, followed by repeated execution as long as a specified condition remains true. This structure is particularly useful when the initial execution of the code block is necessary before checking the condition, making it distinct from the standard “while” loop, which evaluates the condition before executing the block. The syntax is straightforward, consisting of the “do” keyword, the code block enclosed in curly braces, followed by the “while” keyword and the condition in parentheses.
One of the key advantages of using a “do while” loop is its guarantee of at least one execution of the code block, which can be critical in scenarios where initial data processing or user input is required. This feature makes it ideal for applications such as form validation or interactive prompts where user engagement is necessary before proceeding. Moreover, the loop’s structure facilitates easy readability and maintenance, allowing developers to clearly define the execution flow.
In summary, the “do while” loop is a powerful tool in JavaScript programming that enhances control over code execution. By ensuring that the code block runs at least once, it provides flexibility in handling user input and other scenarios requiring immediate
Author Profile
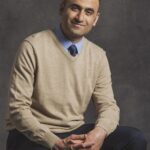
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?