How Does the ‘Do While’ Loop Work in Python 3?
### Introduction
In the world of programming, control flow is a fundamental concept that dictates how a program executes its instructions. Among the various control flow structures, loops play a crucial role in enabling repetitive tasks with ease and efficiency. While many languages offer a straightforward way to create loops, Python takes a slightly different approach. If you’ve ever found yourself wondering how to implement a `do while` loop in Python 3, you’re not alone. This article will unravel the intricacies of looping in Python, exploring alternatives and best practices that can help you achieve the same functionality without the traditional `do while` syntax.
When it comes to looping in Python, the absence of a native `do while` construct might seem like a limitation at first glance. However, Python’s flexibility and the power of its existing loop structures, such as `while` and `for`, allow developers to replicate the behavior of a `do while` loop effectively. The key lies in understanding the unique characteristics of these loops and how they can be combined to meet your programming needs.
In the following sections, we will delve into the mechanics of loops in Python, examining how to create conditions that ensure a block of code runs at least once before checking for further iterations. By the end of this exploration, you’ll be
Understanding the Do-While Loop in Python
In Python, there is no built-in `do while` loop construct like in some other programming languages such as C or Java. However, the functionality of a `do while` loop can be emulated using a `while` loop. The key characteristic of a `do while` loop is that it executes the loop’s body at least once before checking the loop’s condition.
To replicate this behavior in Python, you can use a `while` loop with a specific structure. Here’s how you can achieve it:
- Execute the code block.
- Evaluate the condition.
- If the condition is true, repeat the process.
Here is a basic example:
python
condition = True
while True:
# Code to execute
print(“This will print at least once.”)
# Check condition
if not condition:
break
In this example, the loop will run indefinitely until the condition becomes . The key point is that the loop body is executed before the condition is checked, ensuring at least one execution.
Examples of Emulating Do-While Loops
To illustrate how to emulate a `do while` loop, consider the following scenarios:
- **User Input Validation**: You want to keep asking the user for input until they provide a valid response.
- **Game Loop**: A game continues until the player decides to quit.
### Example: User Input Validation
python
user_input = “”
while True:
user_input = input(“Enter a positive number: “)
if user_input.isdigit() and int(user_input) > 0:
print(f”You entered: {user_input}”)
break
else:
print(“Invalid input, please try again.”)
In this example, the program prompts the user at least once, ensuring they have the opportunity to enter a valid number.
### Example: Game Loop
python
play_again = “yes”
while True:
# Code for the game here
print(“Playing the game…”)
play_again = input(“Do you want to play again? (yes/no): “)
if play_again.lower() != “yes”:
break
This implementation allows the game to be played at least once, with the option to play again afterward.
Benefits of Using Emulated Do-While Loops
Emulating a `do while` loop in Python offers several advantages:
- Flexibility: You can control the execution flow precisely by placing conditions at the end of your loop.
- Clear Logic: The structure can make certain types of logic clearer, especially when an action must occur before a decision is made.
- User-Friendly: It is particularly useful in scenarios requiring user input, as it guarantees that prompts are issued at least once.
Feature | Do-While Loop | While Loop (Emulation) |
---|---|---|
Execution Guarantee | At least once | At least once |
Syntax | Built-in | Custom structure |
Common Usage | Input validation, menu selections | General looping |
By understanding how to implement a `do while` loop using a `while` loop in Python, developers can enhance their programming capabilities and create more robust applications.
Understanding the `do…while` Loop Concept in Python
Python does not have a built-in `do…while` loop structure as seen in other programming languages like C, C++, or Java. However, you can simulate the behavior of a `do…while` loop using a `while` loop with a specific structure.
The essence of a `do…while` loop is that it executes the block of code at least once before checking the condition. This can be accomplished in Python by using a `while` loop with a condition that is checked at the end of the loop.
Simulating a `do…while` Loop in Python
To create a `do…while` loop in Python, you can follow this pattern:
python
while True:
# Code block to execute
# …
if not condition:
break
In this structure:
- The loop starts with `while True`, ensuring that it runs indefinitely until explicitly broken.
- The code block inside the loop is executed first.
- After executing the block, the condition is checked, and if it evaluates to “, the `break` statement is executed to exit the loop.
Example of a Simulated `do…while` Loop
Consider the following example, which prompts a user for input until they provide a valid response:
python
user_input = “”
while True:
user_input = input(“Please enter a number greater than 0: “)
if user_input.isdigit() and int(user_input) > 0:
print(f”You entered: {user_input}”)
break
else:
print(“Invalid input. Try again.”)
In this case:
- The loop continues to prompt the user until they input a valid number.
- The input is checked after the prompt, ensuring the code inside the loop runs at least once.
Advantages of Simulating `do…while` in Python
- Simplicity: It provides a straightforward way to ensure that code executes at least once.
- Flexibility: This method can adapt to various conditions and scenarios, allowing for complex logic to be implemented easily.
- Readability: When used correctly, it can maintain clarity in the code structure, making it evident that the loop will run at least once.
Comparison with Standard `while` Loops
Feature | `do…while` Loop | `while` Loop |
---|---|---|
Execution Guarantee | Executes at least once | Executes only if condition is true |
Condition Check | After executing the block | Before executing the block |
Use Case | Useful for user input prompts | General looping scenarios |
By understanding this simulation technique, Python developers can implement similar functionality to a `do…while` loop, ensuring their code remains effective and user-friendly.
Understanding the Use of Do While Loops in Python 3
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Python 3 does not have a built-in do while loop structure like some other programming languages. However, developers can mimic this functionality using a while loop combined with a boolean condition that is initially set to True.”
Michael Chen (Lead Python Developer, CodeCraft Solutions). “While Python lacks a native do while loop, the flexibility of its while loop allows for similar behavior. By placing the loop body before the condition check, you can effectively create a do while loop simulation.”
Sarah Thompson (Computer Science Professor, University of Technology). “The absence of a do while loop in Python 3 encourages developers to think critically about their loop structures. This can lead to cleaner, more readable code, as it often requires a reevaluation of the logic being implemented.”
Frequently Asked Questions (FAQs)
What is a do-while loop in Python 3?
A do-while loop is a control flow statement that executes a block of code at least once before checking a condition to determine if it should continue executing. Python does not have a built-in do-while loop syntax, but similar functionality can be achieved using a while loop.
How can I implement a do-while loop in Python 3?
To simulate a do-while loop in Python, you can use a while loop with a break statement. First, execute the code block, then check the condition at the end of the block. If the condition is true, the loop continues; otherwise, it breaks.
Can you provide an example of a do-while loop simulation in Python 3?
Certainly. Here is an example:
python
while True:
# Code block to execute
print(“This will run at least once.”)
# Condition to continue or break
if not condition:
break
What are the advantages of using a do-while loop?
The main advantage of a do-while loop is that it guarantees the execution of the code block at least once, regardless of the condition. This is particularly useful when the initial execution is necessary before any condition can be evaluated.
Are there any limitations to using a do-while loop in Python 3?
Since Python does not have a native do-while loop, implementing it requires a workaround, which may lead to less readable code. Additionally, improper use can lead to infinite loops if the condition is not handled correctly.
When should I use a do-while loop instead of a regular while loop in Python 3?
You should use a do-while loop when you need to ensure that a block of code runs at least once before any condition is checked. This is particularly useful in scenarios where the initial execution is critical for subsequent logic or validation.
In Python 3, the traditional “do while” loop structure, commonly found in other programming languages, does not exist as a built-in construct. However, the functionality of a “do while” loop can be emulated using a combination of a “while” loop and a conditional statement. This approach allows developers to execute a block of code at least once before checking a condition at the end of the loop, thus achieving the same effect as a “do while” loop.
To implement this in Python, one can use a “while True” loop along with a break statement that evaluates the condition at the end of the loop. This method provides flexibility and maintains clarity in the code structure. Additionally, it aligns with Python’s design philosophy that emphasizes readability and simplicity, making it easier for developers to understand the flow of the program.
In summary, while Python 3 does not have a native “do while” loop, developers can effectively simulate its behavior through alternative looping constructs. This adaptability showcases Python’s versatility and encourages programmers to think creatively when faced with language limitations. Understanding these alternatives is crucial for writing efficient and maintainable code in Python.
Author Profile
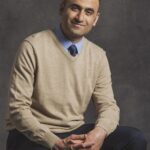
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?