Understanding ‘Does Not Equal’ in JavaScript: What You Need to Know?
In the world of programming, precision is key, and understanding how to compare values effectively is fundamental to writing robust and error-free code. In JavaScript, one of the most common tasks developers encounter is determining whether two values are not equal. While this may seem straightforward, the nuances of equality and inequality can lead to unexpected behavior if not handled correctly. This article delves into the concept of “does not equal” in JavaScript, exploring the various operators and methods available for performing these comparisons.
At the heart of JavaScript’s comparison mechanics are two primary operators: `!=` (loose inequality) and `!==` (strict inequality). Each serves a distinct purpose, with the former allowing type coercion and the latter enforcing strict type checks. Understanding the differences between these operators is crucial for developers who want to avoid pitfalls that can arise from unintended type conversions. Moreover, the implications of using one operator over the other can significantly affect the logic of your code, leading to either successful outcomes or frustrating bugs.
As we dive deeper into the intricacies of these operators, we will also examine practical examples, common use cases, and best practices for implementing inequality checks in JavaScript. Whether you’re a seasoned developer or just starting your coding journey, mastering the concept of “does not equal
Understanding the Comparison Operators
In JavaScript, comparison operators are crucial for evaluating expressions. The operator `!=` is used to determine if two values are not equal. However, it performs a type coercion, meaning it converts the operands to the same type before making the comparison. This can lead to unexpected results.
To avoid such issues, JavaScript also offers the strict inequality operator `!==`, which checks both the value and type of the operands without performing type coercion. This is considered best practice as it provides more predictable outcomes.
Examples of Not Equal Operators
Here are some examples illustrating how both `!=` and `!==` work:
javascript
console.log(5 != ‘5’); // (type coercion occurs)
console.log(5 !== ‘5’); // true (different types)
console.log(null != undefined); // (considered equal with type coercion)
console.log(null !== undefined); // true (different types)
The first example demonstrates how `5 != ‘5’` evaluates to “ due to type coercion, while `5 !== ‘5’` evaluates to `true` since the types differ.
When to Use Not Equal Operators
Choosing between `!=` and `!==` depends on the specific requirements of the comparison:
- Use `!=` when:
- You are certain that type coercion will not lead to misleading results.
- You are comparing values from different sources where type consistency is guaranteed.
- Use `!==` when:
- You want to avoid type coercion and ensure both value and type match.
- You are dealing with user input or data from external sources where type inconsistencies may arise.
Common Pitfalls
Utilizing the not equal operators can lead to common pitfalls, especially for those new to JavaScript:
- Comparing different types without considering type coercion can yield negatives.
- Relying on `!=` can introduce bugs in your code, especially in conditional statements and loops.
Comparison Table
The following table summarizes the differences between the two not equal operators:
Operator | Description | Type Coercion | Example |
---|---|---|---|
!= | Not equal, allows type coercion | Yes | 5 != ‘5’ → |
!== | Strict not equal, no type coercion | No | 5 !== ‘5’ → true |
By understanding these distinctions, developers can make informed decisions when performing comparisons in JavaScript, leading to more robust and error-free code.
Understanding the “Not Equal” Operator in JavaScript
In JavaScript, to evaluate whether two values are not equal, you can use the `!=` operator. This operator compares two values for inequality, performing type coercion if necessary.
Types of Not Equal Operators
JavaScript provides two primary not equal operators:
- Loose Not Equal (`!=`): This operator checks for inequality with type coercion.
- Strict Not Equal (`!==`): This operator checks for inequality without type coercion, meaning both the value and type must differ.
Usage Examples
Here are some examples to illustrate the difference between the loose and strict not equal operators:
Expression | Result (Loose) | Result (Strict) |
---|---|---|
5 != ‘5’ | true | |
5 !== ‘5’ | true | |
null != undefined | true | |
null !== undefined | true | |
‘hello’ != ‘world’ | true | true |
‘5’ != 5 | true |
Best Practices
When using the not equal operators in JavaScript, consider the following best practices:
- Use Strict Not Equal: Prefer `!==` over `!=` to avoid unexpected results due to type coercion.
- Be Aware of Type Coercion: Understand how JavaScript handles different types, especially when comparing numbers and strings.
- Consistent Type Checking: If your application logic relies on type consistency, ensure that you are comparing like types whenever possible.
Common Pitfalls
When working with not equal comparisons, be mindful of common pitfalls:
- Comparing objects and arrays using `!=` or `!==` will always return `true` unless they reference the same instance.
- Be cautious with falsy values (e.g., `0`, `”`, `null`, `undefined`, `NaN`). These can lead to unexpected results when using the loose not equal operator.
Usage
Understanding the nuances between `!=` and `!==` can help avoid bugs in your JavaScript code. By adhering to strict type checking whenever feasible, you can ensure your comparisons are both reliable and predictable.
Understanding the Concept of Inequality in JavaScript
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “In JavaScript, the operator ‘!=’ is used to determine if two values are not equal, while ‘!==’, the strict inequality operator, checks both value and type. This distinction is crucial for avoiding unexpected behavior in applications.”
Michael Chen (JavaScript Framework Developer, CodeCraft Solutions). “When using ‘!=’ in JavaScript, it’s important to remember that it performs type coercion, which can lead to confusing results. For more predictable outcomes, developers should prefer ‘!==’, which enforces strict equality.”
Sarah Patel (JavaScript Educator, Web Dev Academy). “Understanding the difference between ‘!=’ and ‘!==’, as well as their implications, is essential for writing robust JavaScript code. Misusing these operators can introduce subtle bugs that are difficult to trace.”
Frequently Asked Questions (FAQs)
What does “does not equal” mean in JavaScript?
The “does not equal” operator in JavaScript is represented by `!=`. It checks if two values are not equal, returning `true` if they are different and “ if they are the same.
What is the difference between != and !== in JavaScript?
The `!=` operator performs type coercion, meaning it converts the values to the same type before comparison. In contrast, `!==` checks both value and type without coercion, ensuring that both are strictly not equal.
When should I use != instead of !==?
You should use `!=` when you want to allow type coercion and compare values regardless of their types. However, it is generally recommended to use `!==` for strict comparisons to avoid unexpected results due to type conversion.
Can you provide an example of != in JavaScript?
Certainly. For example, `5 != ‘5’` evaluates to “ because the values are considered equal after type coercion, while `5 !== ‘5’` evaluates to `true` since they are of different types.
Is it a best practice to use != in JavaScript?
It is not considered a best practice to use `!=` due to potential confusion from type coercion. Using `!==` is preferred for clarity and to prevent unintended behavior in comparisons.
How can I check if two values are not equal in JavaScript without type coercion?
To check if two values are not equal without type coercion, use the `!==` operator. For example, `value1 !== value2` will return `true` only if `value1` and `value2` are not equal in both value and type.
In JavaScript, the concept of “does not equal” is primarily represented by the operator `!=` and its strict counterpart `!==`. The `!=` operator checks for inequality between two values, performing type coercion if necessary, while `!==` checks for both value and type, ensuring that no type conversion occurs. This distinction is crucial for developers to understand, as it can lead to different outcomes depending on the operator used.
Using `!=` may lead to unexpected results due to its coercive nature, particularly when comparing different data types. For example, `0 != ‘0’` evaluates to because the string ‘0’ is coerced into a number. In contrast, `!==` would yield true in this case, as the types are different. Therefore, it is advisable to use `!==` for comparisons where type integrity is essential, thereby avoiding potential bugs in the code.
Moreover, developers should be aware of the implications of using these operators in conditional statements and loops. Misunderstanding the behavior of equality operators can lead to logical errors, which may be difficult to debug. As a best practice, it is recommended to consistently use strict equality (`===` and `!==`) to ensure that both value
Author Profile
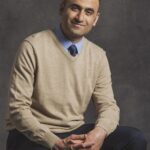
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?