Does Python Have a ‘Does Not Equal’ Syntax?
In the world of programming, precision is key, and understanding how to express conditions accurately can make or break your code. One of the fundamental concepts in Python—and indeed in many programming languages—is the ability to compare values. While most people are familiar with the equality operator, the concept of “does not equal” is equally crucial for creating effective conditional statements. Whether you’re debugging a complex algorithm or simply writing a straightforward script, mastering the syntax for “does not equal” in Python is essential for achieving the desired outcomes in your code.
In Python, the “does not equal” operator is a vital tool that allows programmers to filter data, control the flow of their applications, and implement logic that responds dynamically to user input or variable states. Understanding how to properly use this operator can help you avoid common pitfalls and enhance the overall functionality of your programs. As you delve deeper into Python’s syntax and capabilities, you’ll discover that this seemingly simple operator opens the door to more complex comparisons and logical structures.
As we explore the nuances of the “does not equal” syntax in Python, you’ll gain insights into its practical applications, common use cases, and how it fits within the broader context of conditional statements. By the end of this article, you’ll be equipped with the knowledge to confidently implement this operator
Understanding the Not Equal Syntax in Python
In Python, the concept of inequality is expressed using the `!=` operator. This operator is fundamental when evaluating conditions where two values are compared to determine if they are not equal. It is essential to understand how this operator operates within various contexts, such as conditional statements, loops, and functions.
Basic Usage of Not Equal Operator
The `!=` operator can be used with any data types, including integers, strings, lists, and more. When you utilize this operator, Python evaluates the expression and returns a Boolean value—`True` if the values are not equal and “ if they are.
Here is a simple demonstration:
python
# Example of not equal operator
a = 5
b = 10
if a != b:
print(“a and b are not equal”)
In this example, since `5` is not equal to `10`, the output will be “a and b are not equal”.
Complex Comparisons
In more complex scenarios, the `!=` operator can be effectively used in conjunction with other logical operators such as `and` and `or`. This allows for more nuanced conditions to be evaluated.
For example:
python
x = “Hello”
y = “World”
if x != y and len(x) > 3:
print(“x is not equal to y and is longer than 3 characters”)
In this case, since `x` is indeed not equal to `y` and its length is greater than 3, the statement will execute.
Comparing Different Data Types
Python’s `!=` operator also handles comparisons between different data types. However, it is crucial to understand that the results can vary based on the types being compared. Here’s a brief overview:
Data Type 1 | Data Type 2 | Result of `!=` |
---|---|---|
5 | 5.0 | |
“123” | 123 | True |
[1, 2] | (1, 2) | True |
None | 0 | True |
In the above table:
- The first comparison evaluates to “ because Python considers `5` and `5.0` as equal.
- The second comparison returns `True` as a string and an integer are inherently different types.
- The third comparison showcases that a list and a tuple with the same elements are not considered equal.
- Finally, `None` and `0` are also evaluated as different.
Common Pitfalls
While using the `!=` operator, developers should be aware of certain pitfalls:
- Floating Point Comparisons: Due to precision issues, comparing floating-point numbers can yield unexpected results.
- String Comparisons: Ensure that string comparisons account for case sensitivity, as “Hello” is not equal to “hello”.
- Mutable vs Immutable Types: Be cautious when comparing mutable types like lists. Modifying a list after a comparison can lead to confusion.
Understanding the `!=` operator in Python is crucial for effective programming. By applying this operator correctly, developers can create robust and accurate conditional logic, which is essential in various programming scenarios.
Understanding the ‘Does Not Equal’ Syntax in Python
In Python, the syntax for ‘does not equal’ is represented by the `!=` operator. This operator is crucial for comparing two values and determining if they are not equal. It is commonly used in conditional statements, loops, and any situation where a comparison is necessary.
Usage of the ‘!=’ Operator
The `!=` operator can be utilized with various data types, including integers, floats, strings, and even complex data structures like lists and dictionaries. Here are some practical examples:
- Integer Comparison:
python
a = 5
b = 3
if a != b:
print(“a is not equal to b”)
- String Comparison:
python
str1 = “Hello”
str2 = “World”
if str1 != str2:
print(“Strings are not equal”)
- List Comparison:
python
list1 = [1, 2, 3]
list2 = [1, 2, 4]
if list1 != list2:
print(“Lists are not equal”)
Comparing Different Data Types
When using the `!=` operator, Python performs type coercion as needed. However, comparing incompatible types will not raise an error but will simply return `True` or “. Consider the following table that illustrates various comparisons:
Value 1 | Value 2 | Result |
---|---|---|
5 | 5 | |
‘apple’ | ‘banana’ | True |
[1, 2] | [1, 2] | |
(1, 2) | (1, 3) | True |
None | 0 | True |
Using ‘!=’ in Conditional Statements
The `!=` operator is frequently employed in `if` statements to control the flow of a program based on conditions. Here is an example of its usage in a loop:
python
for i in range(5):
if i != 3:
print(f”{i} is not equal to 3″)
This loop will print all numbers from 0 to 4, excluding 3.
Common Pitfalls
While using the `!=` operator, developers should be mindful of the following common pitfalls:
- Floating Point Precision Issues: Comparing floating-point numbers for equality or inequality can lead to unexpected results due to precision errors.
python
a = 0.1 + 0.2
b = 0.3
print(a != b) # This may return True due to floating-point precision
- Mutable vs Immutable Types: When comparing mutable types like lists or dictionaries, ensure that you are aware of their contents, as two lists with identical contents are equal, while two lists that are different objects in memory (even if they contain the same elements) may not be.
- None Type Comparisons: Be cautious when comparing `None` with other data types. In Python, `None` is considered distinct from all other values.
python
value = None
if value != 0:
print(“Value is not zero”)
This will print “Value is not zero” since `None` is not equal to `0`.
Understanding the `!=` operator is essential for effective programming in Python. Its correct application in various scenarios enhances the logic and control flow of your code.
Understanding the ‘Does Not Equal’ Syntax in Python
Dr. Emily Carter (Senior Software Engineer, Python Development Institute). “In Python, the ‘does not equal’ syntax is represented by ‘!=’. This operator is essential for conditional statements, allowing developers to compare values effectively and execute code based on inequality.”
James Lin (Lead Python Instructor, Code Academy). “Many beginners often confuse the ‘!=’ operator with other languages’ syntax for inequality. It is crucial to understand that in Python, ‘!=’ is the standard way to denote that two values are not equal, which is fundamental in programming logic.”
Dr. Sarah Thompson (Computer Science Professor, Tech University). “The ‘does not equal’ operator, ‘!=’, is not just a syntactical choice in Python; it embodies the language’s philosophy of simplicity and readability, making it easier for newcomers to grasp fundamental programming concepts.”
Frequently Asked Questions (FAQs)
What is the syntax for “does not equal” in Python?
In Python, the syntax for “does not equal” is represented by the operator `!=`. This operator is used to compare two values, returning `True` if they are not equal and “ if they are equal.
Are there any alternatives to the “does not equal” operator in Python?
While `!=` is the standard operator for “does not equal,” you can also use the `is not` keyword for comparing object identities, which checks if two references point to different objects.
Can “does not equal” be used in conditional statements in Python?
Yes, the `!=` operator can be used in conditional statements, such as `if` statements, to execute code based on whether two values are not equal.
How does “does not equal” work with different data types in Python?
The `!=` operator works with various data types, including integers, strings, and lists. It compares the values and types, returning `True` if they are not equal in value or type.
What happens if I use “does not equal” on incompatible types in Python?
When using the `!=` operator on incompatible types, Python will return `True` since the values are inherently different. For example, comparing a string and an integer will yield `True`.
Is there a case-sensitive aspect to “does not equal” in Python?
Yes, string comparisons using `!=` are case-sensitive in Python. For example, `”Hello” != “hello”` will return `True` because the casing differs.
In Python, the syntax for expressing inequality is represented by the `!=` operator. This operator allows developers to compare two values or expressions, returning `True` if they are not equal and “ if they are equal. This fundamental aspect of Python’s syntax is crucial for control flow statements, such as `if` conditions, where decisions are made based on the comparison of values.
It is important to note that Python also supports other comparison operators, such as `<`, `>`, `<=`, and `>=`, which can be used alongside `!=` to create complex logical conditions. Understanding how to effectively utilize these operators enhances the ability to write robust and efficient code. Moreover, Python’s dynamic typing system allows for comparisons between different data types, although caution should be exercised to avoid unintended results.
In summary, the `!=` operator is an essential component of Python’s syntax for expressing inequality. Mastery of this operator, along with its counterparts, is vital for effective programming in Python. By leveraging these tools, developers can create more dynamic and responsive applications that accurately reflect the conditions of their logic.
Author Profile
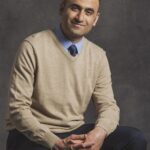
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?