Why Does the Error ‘Does Not Exist on Type ‘AutolinkNode’?’ Keep Popping Up?
In the world of programming, encountering errors can often feel like navigating a labyrinth—each twist and turn revealing new challenges to overcome. One such common yet perplexing error that developers frequently face is the message that something “does not exist on type ‘autolinknode’.” This seemingly cryptic notification can halt progress and lead to frustration, especially for those who are new to the intricacies of type systems in languages like TypeScript. Understanding the roots of this error and how to effectively address it is essential for any developer looking to enhance their coding skills and streamline their workflow.
This article delves into the nuances of type errors, particularly focusing on the ‘autolinknode’ type. We will explore the underlying principles of type safety and how these concepts manifest in real-world coding scenarios. By breaking down the components of this error, we aim to equip you with the knowledge needed to troubleshoot and resolve similar issues, empowering you to write cleaner, more efficient code.
As we navigate through the complexities of type definitions and their implications on your projects, we’ll also highlight best practices that can help prevent such errors from surfacing in the first place. Whether you are a seasoned developer or just starting your coding journey, understanding these concepts will not only enhance your problem-solving skills but
Understanding the Error
When encountering the error message `does not exist on type ‘autolinknode’`, it typically indicates that TypeScript is unable to recognize a property or method being called on an instance of the `autolinknode` type. This can arise from various factors including misconfigurations, type mismatches, or the absence of expected properties in the defined type.
Common reasons for this error include:
- Typographical Errors: Ensure that the property name is spelled correctly and matches the definition in the type.
- Type Definitions: The type `autolinknode` may not include the property or method you are attempting to access.
- Incorrect Type Assertions: If using type assertions, ensure that the assertion is valid and accurately reflects the actual structure of the object.
To address the issue, one must first investigate the type definitions and ensure that the property in question is included.
Resolving the Issue
Here are steps to troubleshoot and resolve the error:
- Check Type Definitions: Review the definition of the `autolinknode` type to confirm the property exists.
- Update TypeScript Configurations: Sometimes, issues arise from incorrect TypeScript configurations. Make sure your `tsconfig.json` is set up correctly.
- Use Type Guards: Implement type guards to ensure that the object is of the expected type before accessing its properties.
Example type guard:
“`typescript
function isAutolinkNode(node: any): node is AutolinkNode {
return ‘expectedProperty’ in node;
}
“`
- Type Assertion: If you are confident that the property exists, you can use type assertions, though this should be done cautiously.
Example of type assertion:
“`typescript
const node = someNode as AutolinkNode;
console.log(node.expectedProperty);
“`
Example of Type Definitions
Understanding the structure of the `autolinknode` type is crucial. Below is an example definition:
“`typescript
interface AutolinkNode {
id: string;
url: string;
title?: string; // Optional property
}
“`
In this example, `title` is optional. Attempting to access it without checking may lead to the error if it is not defined on a particular instance.
Common Properties of AutolinkNode
The following table outlines typical properties associated with the `autolinknode` type:
Property | Type | Description |
---|---|---|
id | string | Unique identifier for the node |
url | string | Link associated with the node |
title | string (optional) | Title or description for the link |
By understanding the structure and properties of the `autolinknode` type, developers can better troubleshoot and resolve the error. Always ensure that your code respects the defined types and structures to avoid such issues.
Understanding the Error Message
The error message `does not exist on type ‘autolinknode’` typically occurs in TypeScript when you attempt to access a property or method that is not defined in the specified type. This is a common issue developers face when working with typed languages, where strict type definitions prevent the use of properties.
Key Causes of the Error
- Incorrect Type Definition: The property or method you are trying to access may not be defined in the `autolinknode` type.
- Type Assertion Issues: If you are using type assertions, they may not align with the actual structure of the object.
- Library Updates: Changes in third-party libraries can lead to discrepancies between the expected and actual types.
Troubleshooting Steps
- Check Type Definitions: Review the definition of `autolinknode` to confirm if the property exists. This can often be found in the documentation or type definition files.
- Use Type Guards: Implement type guards to ensure that you are accessing properties of the correct type. For example:
“`typescript
if (‘desiredProperty’ in autolinknode) {
// Safe to access desiredProperty
}
“`
- Update TypeScript Configuration: Ensure your `tsconfig.json` is set up to include all necessary type definitions and libraries.
Example of Type Definition
An example of how an `autolinknode` type might be defined is illustrated below. This can help clarify what properties are accessible.
“`typescript
interface AutoLinkNode {
id: string;
url: string;
title?: string; // Optional property
}
“`
Accessing Properties Safely
When accessing properties, especially optional ones, it is crucial to handle cases where they may not be defined:
- Use optional chaining:
“`typescript
const title = autolinknode.title ?? “Default Title”; // Fallback to default if title is not present
“`
- Utilize a type assertion if you are certain of the type:
“`typescript
const node = autolinknode as AutoLinkNode;
console.log(node.url); // Safe access if you are sure of the type
“`
Best Practices to Avoid Errors
Adhering to best practices can significantly reduce the occurrence of type-related errors:
- Consistent Type Definitions: Ensure all types are consistently defined across your application.
- Regular Updates: Keep your libraries and TypeScript updated to the latest versions to avoid compatibility issues.
- Thorough Testing: Employ unit tests to verify that your types and properties behave as expected, especially after changes.
Example Table of Common Type Errors
Error Message | Possible Cause | Solution |
---|---|---|
`does not exist on type ‘autolinknode’` | Accessing a non-existent property | Verify property existence in type |
`Type ” is not assignable to type ‘string’` | Missing optional property handling | Use optional chaining or default values |
`Argument of type ‘X’ is not assignable to parameter of type ‘Y’` | Mismatched types in function calls | Ensure parameter types match expected types |
Conclusion on Type Safety
Maintaining type safety is essential in TypeScript development. By understanding the implications of type definitions, utilizing appropriate checks, and following best practices, developers can effectively mitigate the risks associated with type-related errors.
Understanding Type Errors in TypeScript: Expert Insights
Dr. Emily Chen (Senior Software Engineer, TypeScript Development Team). “The error message ‘does not exist on type ‘autolinknode’?’ typically indicates that the property or method you are trying to access is not defined within the specified type. It is crucial to ensure that your type definitions are correctly set up and that you are referencing the right types in your code.”
Mark Thompson (Lead TypeScript Instructor, Code Academy). “When encountering the error ‘does not exist on type ‘autolinknode’?’, developers should consider checking their interfaces and type declarations. Often, this error arises from a mismatch between expected and actual type structures, which can be resolved by reviewing the type definitions.”
Sarah Patel (Technical Consultant, Software Solutions Inc.). “To effectively troubleshoot the ‘does not exist on type ‘autolinknode’?’ error, I recommend using TypeScript’s type assertion features. This allows developers to explicitly define the expected type, which can help in clarifying the intended structure and resolving the error.”
Frequently Asked Questions (FAQs)
What does the error “does not exist on type ‘autolinknode'” mean?
This error indicates that you are attempting to access a property or method that is not defined on the ‘autolinknode’ type in your TypeScript code. It usually arises from type mismatches or incorrect assumptions about the structure of the object.
How can I resolve the “does not exist on type ‘autolinknode'” error?
To resolve this error, ensure that the property or method you are trying to access is correctly defined in the ‘autolinknode’ type. You may need to check your type definitions or update them to include the necessary properties.
What are common causes of the “does not exist on type ‘autolinknode'” error?
Common causes include using outdated type definitions, attempting to access properties that are not part of the type, or misconfigurations in your TypeScript setup that lead to incorrect type inference.
Can I use type assertions to bypass the “does not exist on type ‘autolinknode'” error?
Yes, you can use type assertions to bypass the error, but this approach should be used cautiously. It may lead to runtime errors if the asserted type does not match the actual structure of the object.
Is it advisable to ignore TypeScript errors like “does not exist on type ‘autolinknode’?”
Ignoring TypeScript errors is generally not advisable, as it undermines the benefits of type safety. It is better to address the root cause of the error to maintain code quality and prevent potential issues.
How can I check the structure of ‘autolinknode’ to avoid this error?
You can check the structure of ‘autolinknode’ by reviewing its type definition in your codebase or using TypeScript’s built-in tools such as the TypeScript Language Service, which can provide insights into the types and their properties.
The phrase “does not exist on type ‘autolinknode'” typically arises in the context of programming, particularly when dealing with TypeScript or similar typed languages. This error indicates that a specific property or method being referenced is not defined within the type ‘autolinknode’. Understanding the implications of this error is crucial for developers, as it can hinder the functionality of the code and lead to runtime issues if not addressed properly.
One of the primary reasons for encountering this error is the mismatch between the expected structure of an object and its actual implementation. Developers must ensure that the properties and methods they are trying to access are correctly defined in the type’s interface. This often requires a thorough review of the type definitions and the objects being manipulated within the codebase. Proper type annotations and adherence to the defined structures can significantly reduce the occurrence of such errors.
Furthermore, resolving the “does not exist on type ‘autolinknode'” error often involves leveraging TypeScript’s features, such as type assertions or defining more precise types. Developers should familiarize themselves with the type system and use tools like TypeScript’s compiler options to catch these errors during the development phase rather than at runtime. This proactive approach not only improves code reliability but also enhances overall
Author Profile
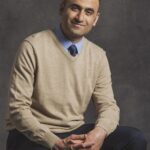
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?