Does Python Have a Switch Statement? Exploring Alternatives and Solutions
In the world of programming, the ability to make decisions based on varying conditions is a fundamental skill that shapes how we write efficient and readable code. Many popular programming languages, such as C, Java, and JavaScript, offer a built-in switch statement, allowing developers to streamline their conditional logic. However, Python, known for its simplicity and readability, takes a different approach. This raises an intriguing question: does Python have a switch statement? In this article, we will explore Python’s unique handling of conditional statements, delve into alternative structures, and uncover the reasoning behind Python’s design choices.
While Python does not include a traditional switch statement, it provides several powerful tools to achieve similar functionality. The language emphasizes clarity and explicitness, which leads to the use of if-elif-else chains as a primary means of handling multiple conditions. This approach, while straightforward, can sometimes lead to lengthy and less maintainable code when dealing with numerous cases.
To address this, Python developers have devised creative alternatives, such as using dictionaries to map keys to functions or values, effectively mimicking the behavior of a switch statement. Additionally, the of structural pattern matching in Python 3.10 offers a more sophisticated way to handle complex conditional logic. As we dive deeper
Understanding Python’s Conditional Constructs
Python does not have a traditional switch statement like some other programming languages such as C or Java. Instead, Python relies on a combination of if-elif-else constructs and dictionary mappings to achieve similar functionality. This approach provides flexibility and clarity, allowing developers to implement conditional logic effectively.
If-Elif-Else Constructs
The most straightforward way to handle multiple conditions in Python is through if-elif-else statements. This structure allows for checking multiple expressions and executing corresponding blocks of code.
Example:
“`python
def switch_example(value):
if value == 1:
return “One”
elif value == 2:
return “Two”
elif value == 3:
return “Three”
else:
return “Invalid value”
“`
In this example, the function `switch_example` checks the value against different cases and returns a corresponding string. While this method is clear and easy to understand, it can become cumbersome with many conditions.
Using Dictionaries for Switch-Like Behavior
Another efficient way to simulate a switch statement is by using dictionaries to map keys to functions or values. This method simplifies the code and enhances readability.
Example:
“`python
def case_one():
return “One”
def case_two():
return “Two”
def case_three():
return “Three”
def default_case():
return “Invalid value”
switch_dict = {
1: case_one,
2: case_two,
3: case_three
}
def switch_example(value):
return switch_dict.get(value, default_case)()
“`
In this code, `switch_dict` serves as a mapping from case values to functions. The `get` method is used to retrieve the function associated with a key, returning the `default_case` function if the key does not exist.
Comparison of Methods
Feature | If-Elif-Else | Dictionary Mapping |
---|---|---|
Syntax Clarity | Simple and clear | Requires more setup but concise |
Performance | Slower with many conditions | Faster for large conditions |
Flexibility | Limited to static conditions | Dynamic with functions |
Readability | Straightforward for few cases | Cleaner for many cases |
In summary, Python’s lack of a traditional switch statement is compensated by powerful alternatives like if-elif-else constructs and dictionary-based mappings. Understanding when to use each method can enhance the readability and efficiency of your code.
Python’s Alternative to Switch Statements
Python does not have a built-in switch statement as seen in other programming languages like C, Java, or JavaScript. However, there are several alternative approaches that can achieve similar functionality.
Using Dictionaries for Switch-like Behavior
One common method to simulate switch statements in Python is by utilizing dictionaries. This approach allows you to map keys to functions or values, enabling a cleaner and more efficient way to handle multiple cases.
“`python
def case_one():
return “This is case one.”
def case_two():
return “This is case two.”
def case_default():
return “This is the default case.”
switch_dict = {
1: case_one,
2: case_two
}
result = switch_dict.get(case_number, case_default)()
“`
In this example, `case_number` is the variable being switched on. The `get` method retrieves the function associated with the provided key or defaults to `case_default` if the key is not found.
Using If-Elif-Else Statements
Another straightforward approach involves using a series of if-elif-else statements. This method is simple and effective, especially for a small number of cases.
“`python
if case_number == 1:
result = “This is case one.”
elif case_number == 2:
result = “This is case two.”
else:
result = “This is the default case.”
“`
While this method lacks the elegance of a switch statement, it remains a clear and direct way to handle multiple conditions.
Leveraging the match Statement (Python 3.10 and Later)
Starting with Python 3.10, a new structural pattern matching feature was introduced, which provides a more sophisticated alternative to traditional switch statements. The `match` statement allows for more complex patterns and can match types, sequences, and other structures.
“`python
match case_number:
case 1:
result = “This is case one.”
case 2:
result = “This is case two.”
case _:
result = “This is the default case.”
“`
This syntax is clearer and can handle more complex scenarios, making it a powerful tool for developers familiar with the latest Python features.
Comparative Summary of Approaches
Approach | Pros | Cons |
---|---|---|
Dictionaries | Clean, efficient, extensible | Requires functions for each case |
If-Elif-Else | Simple and intuitive | Can become unwieldy with many cases |
Match Statement (3.10+) | Powerful pattern matching capabilities | Requires Python 3.10 or newer |
Each method has its own advantages and may be more suitable depending on the specific use case and the complexity of the conditions being evaluated.
Understanding Python’s Control Flow: The Absence of a Switch Statement
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Python does not include a traditional switch statement like some other programming languages. Instead, Python encourages the use of if-elif-else chains or dictionary mappings to achieve similar functionality, promoting more readable and maintainable code.”
Michael Chen (Programming Language Researcher, CodeLab Institute). “The absence of a switch statement in Python is a design choice that aligns with the language’s philosophy of simplicity and clarity. Developers often find that using dictionaries or functions as values can effectively replace switch-case logic while enhancing flexibility.”
Sarah Thompson (Lead Python Developer, Open Source Solutions). “Although Python lacks a built-in switch statement, the of structural pattern matching in Python 3.10 offers a powerful alternative. This feature allows for more complex conditional logic that can mimic switch-case behavior while adhering to Python’s syntax and style.”
Frequently Asked Questions (FAQs)
Does Python have a switch statement?
Python does not have a built-in switch statement like some other programming languages. However, similar functionality can be achieved using dictionaries or if-elif-else statements.
What is the alternative to a switch statement in Python?
The alternative to a switch statement in Python is to use a dictionary to map keys to functions or values, or to use a series of if-elif-else statements to handle multiple conditions.
How can I implement a switch-like behavior in Python?
You can implement switch-like behavior by defining a dictionary where keys represent cases and values are functions or results. You can then call the dictionary with the desired key.
Are there any libraries that provide switch statement functionality in Python?
While there are no standard libraries that provide a switch statement, third-party libraries like `PySwitch` can be used to mimic switch statement behavior.
Why doesn’t Python have a switch statement?
Python emphasizes readability and simplicity. The design philosophy favors using dictionaries or if-elif-else constructs, which can be more flexible and easier to understand in many scenarios.
Is there a proposal to add a switch statement in future Python versions?
As of now, there are no official proposals to add a switch statement to Python. The community generally prefers existing alternatives for their clarity and effectiveness.
Python does not have a built-in switch statement like many other programming languages such as C, Java, or JavaScript. Instead, Python developers typically use alternative constructs to achieve similar functionality. The most common approaches include using if-elif-else chains, dictionaries for mapping cases to functions or values, and the more recent match statement introduced in Python 3.10, which provides pattern matching capabilities that can mimic switch-like behavior.
The if-elif-else construct is straightforward and widely understood, making it a go-to solution for many developers. However, it can become cumbersome and less readable when dealing with numerous cases. On the other hand, dictionaries can provide a cleaner and more efficient way to handle multiple cases, especially when the cases are associated with specific actions or values. This method enhances code maintainability and readability by clearly defining case mappings.
The of the match statement in Python 3.10 represents a significant advancement in the language’s capabilities. This feature allows for more expressive and versatile case handling, enabling developers to match patterns and destructure data in a way that is both powerful and concise. As such, while Python lacks a traditional switch statement, it offers several robust alternatives that can fulfill the same purpose, each with its own advantages
Author Profile
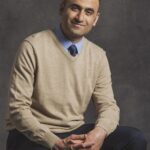
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?