Does Python Have Interfaces? Exploring the Concept and Its Applications
In the ever-evolving landscape of programming languages, Python stands out for its simplicity and versatility. As developers seek to create robust, maintainable code, the concept of interfaces often emerges as a crucial topic of discussion. But does Python have interfaces in the traditional sense? This question opens up a fascinating exploration of how Python approaches the idea of defining contracts for classes and ensuring that they adhere to specific behaviors.
While Python does not have interfaces in the same way that languages like Java or C# do, it embraces a more flexible approach through the use of abstract base classes (ABCs) and duck typing. This unique philosophy allows developers to focus on what an object can do rather than what it is, promoting a more dynamic and adaptable coding style. The emphasis on readability and simplicity in Python means that the implementation of interfaces is often less rigid, allowing for creativity and innovation in software design.
As we delve deeper into the world of Python interfaces, we will uncover how these concepts can be effectively utilized to create clean, efficient, and scalable code. From understanding the role of abstract classes to exploring the principles of duck typing, we will see how Python’s approach to interfaces not only enhances code quality but also empowers developers to build applications that are both powerful and easy to maintain.
Understanding Interfaces in Python
Python does not have a formal interface construct as found in languages like Java or C#. However, the concept of interfaces can be achieved through abstract base classes (ABCs) and duck typing. An interface can be thought of as a contract that specifies a set of methods that a class must implement, without providing the implementation details.
Abstract Base Classes
Abstract Base Classes allow you to define abstract methods that must be implemented by any subclass. This is accomplished using the `abc` module, which provides infrastructure for defining abstract base classes in Python.
Here’s how you can create an abstract base class:
python
from abc import ABC, abstractmethod
class Animal(ABC):
@abstractmethod
def make_sound(self):
pass
class Dog(Animal):
def make_sound(self):
return “Bark”
class Cat(Animal):
def make_sound(self):
return “Meow”
In the example above, the `Animal` class serves as an interface, and both `Dog` and `Cat` are required to implement the `make_sound` method.
Duck Typing
In Python, duck typing is a programming style that relies on the presence of certain methods and properties, rather than on the actual type of the object. The phrase “If it looks like a duck and quacks like a duck, it must be a duck” encapsulates this concept.
Consider the following example:
python
class Bird:
def fly(self):
return “Flies high!”
class Airplane:
def fly(self):
return “Flying at 30,000 feet!”
def let_it_fly(flyable):
print(flyable.fly())
let_it_fly(Bird())
let_it_fly(Airplane())
In this case, both `Bird` and `Airplane` have a `fly` method, allowing them to be used interchangeably in the `let_it_fly` function, demonstrating duck typing.
Comparison of Abstract Classes and Duck Typing
The following table summarizes the differences between abstract classes and duck typing in Python:
Feature | Abstract Classes | Duck Typing |
---|---|---|
Definition | Formal structure for defining interfaces | Informal, based on method presence |
Enforcement | Enforces implementation at compile time | Checks at runtime |
Flexibility | Less flexible, requires inheritance | More flexible, allows different classes |
Use Case | When you need a strict interface | When you want to prioritize flexibility |
Interfaces in Python
While Python does not have interfaces in the traditional sense, it provides powerful tools such as abstract base classes and duck typing. These features allow developers to create flexible and robust systems that adhere to the principles of polymorphism and encapsulation, aligning with Python’s philosophy of simplicity and readability.
Understanding Interfaces in Python
Python does not have a formal interface construct like some other programming languages such as Java or C#. However, the concept of interfaces can be emulated in Python using abstract base classes (ABCs) and duck typing.
Abstract Base Classes (ABCs)
Abstract Base Classes are part of the `abc` module and provide a way to define abstract methods that must be implemented by any subclass. This allows for a form of interface enforcement.
- Defining an Abstract Base Class:
- Use the `ABC` class as a base class.
- Decorate methods with `@abstractmethod` to indicate they must be implemented.
Example:
python
from abc import ABC, abstractmethod
class MyInterface(ABC):
@abstractmethod
def my_method(self):
pass
- Implementing the Interface:
python
class ConcreteClass(MyInterface):
def my_method(self):
print(“Implementation of my_method”)
Duck Typing
Python’s philosophy of “duck typing” allows for flexibility in how interfaces are defined and used. Instead of enforcing a specific interface, Python checks for the presence of methods and properties at runtime.
- Example of Duck Typing:
python
class Duck:
def quack(self):
print(“Quack!”)
class Dog:
def quack(self):
print(“I’m not a duck, but I can quack!”)
def make_it_quack(thing):
thing.quack()
# Using the function
duck = Duck()
dog = Dog()
make_it_quack(duck) # Outputs: Quack!
make_it_quack(dog) # Outputs: I’m not a duck, but I can quack!
Benefits of Using Interfaces
Using interfaces (via ABCs or duck typing) in Python provides several advantages:
- Encapsulation: Interfaces help encapsulate functionality and define clear contracts.
- Flexibility: Duck typing allows for greater flexibility and less rigid design.
- Code Maintenance: Encourages modular design, making code easier to maintain and extend.
Key Considerations
When designing with interfaces in Python, consider the following:
Aspect | Abstract Base Classes | Duck Typing |
---|---|---|
Enforced Structure | Yes | No |
Runtime Checks | Yes | Yes (method presence) |
Error Detection | At instantiation | At runtime |
Use Cases | When strict adherence is required | When flexibility is desired |
Utilizing either approach can enhance the design of Python applications, allowing for clearer architecture and improved code quality.
Understanding Interfaces in Python: Expert Perspectives
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “While Python does not have interfaces in the same way that languages like Java do, it supports a form of interface through abstract base classes (ABCs) in the `abc` module. This allows developers to define methods that must be created within any subclass, promoting a form of contract programming.”
Mark Thompson (Lead Python Developer, Open Source Solutions). “In Python, the concept of interfaces is often implemented using duck typing, where the focus is on whether an object can perform a certain action rather than whether it is of a specific type. This flexibility allows for a more dynamic approach to coding, although it may lead to less strict type checking.”
Lisa Chen (Professor of Computer Science, University of Technology). “Python’s approach to interfaces emphasizes readability and simplicity. By using protocols, particularly with the introduction of type hints in Python 3.5 and later, developers can create clear interfaces that enhance code maintainability while still adhering to Python’s dynamic nature.”
Frequently Asked Questions (FAQs)
Does Python have interfaces?
Python does not have a formal concept of interfaces like some other programming languages (e.g., Java). However, it supports the creation of interfaces through abstract base classes (ABCs) in the `abc` module.
How can I define an interface in Python?
You can define an interface in Python by creating an abstract base class that includes one or more abstract methods. Subclasses must implement these methods to be instantiated.
What is the purpose of using interfaces in Python?
Interfaces in Python provide a way to define a contract for classes, ensuring that they implement certain methods. This promotes code consistency and enables polymorphism.
Can you provide an example of an interface in Python?
Certainly. Here’s a simple example using the `abc` module:
python
from abc import ABC, abstractmethod
class MyInterface(ABC):
@abstractmethod
def my_method(self):
pass
class MyClass(MyInterface):
def my_method(self):
print(“Implementation of my_method”)
Are interfaces mandatory in Python?
Interfaces are not mandatory in Python. They are a design choice that can enhance code readability and maintainability, but Python’s dynamic typing allows for flexibility without strict interface enforcement.
How do interfaces relate to duck typing in Python?
Duck typing is a concept in Python where the type or class of an object is less important than the methods it defines. Interfaces can complement duck typing by providing a clear structure for expected behaviors, while still allowing for flexibility in implementation.
Python does not have interfaces in the traditional sense as seen in languages like Java or C#. Instead, it employs a more flexible approach through the use of abstract base classes (ABCs) and duck typing, which allows for a more dynamic and less rigid structure. ABCs can be defined using the `abc` module, enabling developers to create a blueprint for classes that must implement specific methods. This approach provides a way to enforce certain behaviors while still allowing for polymorphism.
Moreover, Python’s philosophy emphasizes readability and simplicity, which is reflected in its handling of interfaces. The concept of “duck typing” suggests that if an object behaves like a particular type (i.e., it has the necessary methods and properties), it can be treated as that type, regardless of its actual class. This flexibility allows developers to focus on the functionality of their code rather than adhering strictly to predefined interfaces.
In summary, while Python does not implement interfaces in a conventional manner, it offers powerful alternatives through abstract base classes and duck typing. These features enable developers to create robust and maintainable code while adhering to Python’s core principles of simplicity and readability.
Key takeaways include the understanding that Python’s approach to interfaces emphasizes flexibility and dynamic typing
Author Profile
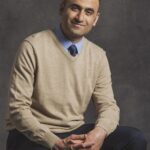
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?