Does Python Have a Switch Case Statement? Exploring Alternatives!
### Introduction
As programming languages continue to evolve, developers often seek efficient ways to manage complex decision-making structures within their code. One common question that arises among Python enthusiasts is whether the language supports a traditional switch-case statement, a staple in many other programming languages like C, Java, and JavaScript. While Python is celebrated for its simplicity and readability, the absence of a built-in switch-case construct has led to intriguing discussions about alternative approaches to handle multiple conditional branches. In this article, we will explore the nuances of Python’s decision-making capabilities and uncover how developers can achieve similar functionality without a dedicated switch-case statement.
In Python, the absence of a switch-case construct does not imply a lack of options for managing multiple conditions. Instead, Python offers a range of alternatives, such as if-elif-else chains, dictionaries, and even the newly introduced match-case statement in Python 3.10. Each of these methods presents unique advantages and use cases, allowing developers to choose the most suitable approach for their specific scenarios. By understanding these alternatives, programmers can maintain clarity and efficiency in their code while effectively handling diverse conditions.
As we delve deeper into the topic, we will examine the traditional switch-case functionality found in other languages and how Python’s design philosophy influences its approach to conditional logic
Understanding Python’s Control Flow
Python does not have a traditional switch-case statement as found in many other programming languages. Instead, it utilizes a series of conditional statements, primarily `if`, `elif`, and `else`, to achieve similar functionality. This approach provides flexibility and readability, aligning with Python’s design philosophy.
Implementing Switch-Case Functionality
While Python lacks a built-in switch-case structure, developers can mimic this functionality through various methods. Below are some of the most common techniques:
- Using Dictionaries: A popular way to simulate switch-case behavior in Python is by using dictionaries to map keys to functions or values.
- Using `if-elif-else` Chains: This is the most straightforward method, where multiple conditions are checked sequentially.
- Using Functions: Defining functions that correspond to different cases can make the code cleaner and more modular.
Example of Dictionary-Based Switch Case
Here’s an example using a dictionary to simulate a switch-case:
python
def case_one():
return “This is case one.”
def case_two():
return “This is case two.”
def case_default():
return “This is the default case.”
switch = {
1: case_one,
2: case_two
}
def switch_case(argument):
return switch.get(argument, case_default)()
# Usage
result = switch_case(1) # Output: This is case one.
This example demonstrates how a dictionary can map keys (case values) to corresponding functions, providing a clear and efficient way to implement switch-case-like behavior.
Comparison of Control Flow Methods
To better understand the advantages and disadvantages of each method, consider the following table:
Method | Advantages | Disadvantages |
---|---|---|
Dictionary-based | Clean, efficient, easy to maintain. | Requires function definitions and may be less intuitive for beginners. |
If-elif-else | Simple and straightforward; easy to understand. | Can become lengthy and hard to read with many cases. |
Function-based | Encourages modular programming and code reuse. | May introduce overhead if not managed properly. |
Switch-Case Alternatives
While Python does not have a native switch-case statement, the available alternatives provide robust means to handle multiple conditions effectively. By utilizing dictionaries, if-elif-else chains, or functions, developers can create clear and maintainable code that achieves the desired functionality.
Understanding Python’s Control Flow
Python does not have a traditional switch-case statement as seen in many other programming languages like C or Java. Instead, Python utilizes if-elif-else constructs to achieve similar functionality. This approach provides flexibility and clarity, allowing developers to handle multiple conditions effectively.
Alternatives to Switch-Case in Python
While Python lacks a built-in switch-case statement, there are several effective alternatives to implement similar behavior:
- If-Elif-Else Chains: This is the most straightforward method.
python
def example(value):
if value == 1:
return “One”
elif value == 2:
return “Two”
elif value == 3:
return “Three”
else:
return “Other”
- Dictionaries as Switch Cases: Python dictionaries can be used to map keys to functions or values, mimicking switch-case behavior.
python
def case_one():
return “One”
def case_two():
return “Two”
def case_three():
return “Three”
switch = {
1: case_one,
2: case_two,
3: case_three
}
def example(value):
return switch.get(value, lambda: “Other”)()
- Using Match Statements (Python 3.10 and later): Python introduced the `match` statement, which functions similarly to a switch-case.
python
def example(value):
match value:
case 1:
return “One”
case 2:
return “Two”
case 3:
return “Three”
case _:
return “Other”
Performance Considerations
When comparing the alternatives for control flow, consider the following performance aspects:
Method | Performance | Readability | Flexibility |
---|---|---|---|
If-Elif-Else Chains | Moderate | High | Moderate |
Dictionary Mapping | High | Moderate | High |
Match Statements | High | High | High |
- If-Elif-Else Chains are simple and intuitive but can become unwieldy with many conditions.
- Dictionaries allow for a clean and efficient lookup but require more setup.
- Match Statements offer a more structured and readable approach while maintaining performance, especially with complex patterns.
In summary, while Python does not have a traditional switch-case statement, it offers several effective alternatives. Developers can choose based on their specific needs for readability, performance, and flexibility, making Python’s control flow robust and adaptable to various scenarios.
Understanding Python’s Approach to Control Flow: The Absence of Switch Case
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “Python does not include a traditional switch-case statement as seen in other programming languages. Instead, Python encourages the use of if-elif-else constructs, which provide greater flexibility and readability in handling multiple conditions.”
Mark Thompson (Programming Language Researcher, CodeLab Institute). “While Python lacks a built-in switch-case statement, developers can achieve similar functionality using dictionaries to map keys to functions or values. This approach not only mimics switch-case behavior but also leverages Python’s strengths in data structures.”
Lisa Nguyen (Python Educator and Author, LearnPythonToday). “The absence of a switch-case statement in Python is a design choice that aligns with the language’s philosophy of simplicity and explicitness. For those transitioning from languages with switch-case, adapting to Python’s control flow can enhance their coding skills and understanding of the language.”
Frequently Asked Questions (FAQs)
Does Python have a built-in switch case statement?
No, Python does not have a built-in switch case statement like some other programming languages. Instead, Python uses if-elif-else statements to achieve similar functionality.
How can I implement switch case functionality in Python?
You can implement switch case functionality in Python using dictionaries to map keys to functions or values. This approach allows for cleaner and more efficient code compared to multiple if-elif statements.
Are there any libraries that provide switch case functionality in Python?
Yes, there are third-party libraries, such as `PySwitch` or `Switch`, that can provide switch case-like functionality. However, using standard Python constructs is generally recommended for clarity and maintainability.
What are the alternatives to switch case in Python?
Alternatives include using if-elif-else chains, dictionaries for function mapping, or using the match statement introduced in Python 3.10, which provides pattern matching capabilities similar to switch case.
Is the match statement in Python 3.10 similar to a switch case?
Yes, the match statement in Python 3.10 offers functionality that resembles switch case statements, allowing for more complex pattern matching and destructuring of data types in a concise manner.
Why is there no switch case in Python?
The absence of a switch case statement in Python is a design choice by the language’s creators, emphasizing readability and simplicity. The existing constructs, such as if-elif-else and dictionaries, are deemed sufficient for most use cases.
Python does not have a built-in switch-case statement like some other programming languages such as C or Java. Instead, Python developers typically use alternative constructs to achieve similar functionality. The most common approaches include using if-elif-else statements, dictionaries for mapping values to functions, and the match-case statement introduced in Python 3.10, which offers pattern matching capabilities that can mimic switch-case behavior.
The introduction of the match-case statement in Python 3.10 represents a significant enhancement to the language, allowing for more expressive and readable code when handling multiple conditions. This feature enables developers to match complex data structures and patterns, providing a powerful tool for decision-making in code. However, for those using earlier versions of Python, the traditional if-elif-else structure remains a widely accepted method for branching logic.
In summary, while Python does not have a traditional switch-case construct, it provides several alternatives that can effectively fulfill the same purpose. Developers should choose the approach that best fits their specific use case, considering factors such as code readability, maintainability, and the version of Python being used. Understanding these alternatives is crucial for writing efficient and clear Python code.
Author Profile
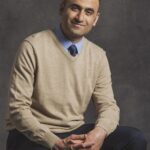
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?