How Can I Use dplyr Select Without Relying on Column Index?
In the world of data manipulation and analysis, R’s `dplyr` package stands out as a powerful tool that streamlines the process of working with data frames. Among its many functions, the `select()` function is particularly beloved for its ability to help users easily choose specific columns from a dataset. However, while many users are accustomed to selecting columns by their index positions, there exists a more intuitive and versatile approach that allows for selection by column names, patterns, and even logical conditions. This article delves into the art of selecting columns in `dplyr` without relying on their index numbers, unlocking a more readable and maintainable way to handle data.
Understanding how to effectively utilize `dplyr`’s `select()` function can significantly enhance your data manipulation workflow. By focusing on column names rather than indices, you can create code that is not only easier to read but also more robust against changes in your dataset’s structure. This approach is particularly beneficial when working with large datasets or collaborating with others, as it minimizes the risk of errors that can arise from shifting column positions.
Moreover, the flexibility of `select()` extends beyond simple name matching. Users can leverage features like tidy evaluation, helper functions, and even regular expressions to dynamically choose columns based on specific
Using dplyr’s select() without Column Indices
The `select()` function in the `dplyr` package is commonly used to choose specific columns from a data frame. While column indices are often used for this purpose, there are several alternative methods that enhance readability and maintainability of your code.
One effective way to select columns without using indices is by employing column names directly. This allows for more intuitive code, especially when working with data frames that contain many columns or when the structure of the data may change over time.
Selecting Columns by Name
To select columns by name, you can simply pass the column names as arguments to the `select()` function. This method is straightforward and allows for clarity when identifying which columns are being manipulated.
“`R
library(dplyr)
Example data frame
data <- data.frame(
id = 1:5,
name = c("Alice", "Bob", "Charlie", "David", "Eva"),
age = c(25, 30, 35, 40, 45)
)
Selecting specific columns by name
selected_data <- data %>% select(name, age)
“`
Utilizing Helper Functions
`dplyr` also provides helper functions that can be employed within `select()` to make column selection more dynamic. Some useful functions include:
- `starts_with(“prefix”)`: Selects columns starting with a specified prefix.
- `ends_with(“suffix”)`: Selects columns ending with a specified suffix.
- `contains(“string”)`: Selects columns that contain a specific string.
These functions can be especially helpful when dealing with large datasets or when you want to select multiple columns that share a common characteristic.
“`R
Selecting columns that start with ‘a’
selected_data <- data %>% select(starts_with(“a”))
“`
Combining Column Names and Helper Functions
You can also combine direct column names with helper functions for a more versatile selection process. This flexibility allows for both explicit and dynamic column selections in a single call.
“`R
Selecting specific columns and columns that start with ‘a’
selected_data <- data %>% select(name, starts_with(“a”))
“`
Example: Selecting Columns in Practice
Here’s a practical example of how you might use `select()` in a data manipulation scenario.
“`R
Example of selecting and renaming columns
final_data <- data %>%
select(Name = name, Age = age) %>%
mutate(Age = Age + 1) Increment age by 1
“`
Summary Table of Column Selection Methods
Method | Syntax Example | Description |
---|---|---|
Select by name | select(column1, column2) | Directly specify the column names. |
Starts with | select(starts_with(“prefix”)) | Selects columns starting with a specific prefix. |
Ends with | select(ends_with(“suffix”)) | Selects columns ending with a specific suffix. |
Contains | select(contains(“string”)) | Selects columns that contain a specific string. |
By leveraging these various methods of column selection, you can create more readable and maintainable R code, enhancing your data manipulation tasks with `dplyr`.
Using dplyr’s select Function Without Column Index
The `select()` function in the `dplyr` package is typically used to specify columns in a data frame. While many users might rely on column indices to select specific columns, dplyr provides a variety of alternatives that allow for selecting columns by name, enabling more readable and maintainable code.
Column Selection by Name
To select columns by their names, you can pass the column names directly to the `select()` function. This approach is more intuitive and less error-prone compared to using indices, especially in data frames with many columns.
“`R
library(dplyr)
Example data frame
df <- data.frame(
a = 1:5,
b = letters[1:5],
c = rnorm(5)
)
Selecting columns by name
selected_df <- df %>% select(a, b)
“`
Using Helper Functions for Selection
dplyr also includes helper functions that can be utilized within `select()` to enhance the selection process. These functions provide flexibility and reduce the risk of errors.
- starts_with(“prefix”): Selects columns that start with a specific prefix.
- ends_with(“suffix”): Selects columns that end with a specific suffix.
- contains(“string”): Selects columns that contain a specific string.
- matches(“regex”): Selects columns that match a regular expression.
- everything(): Selects all columns.
Example of using helper functions:
“`R
Selecting columns that start with ‘a’
selected_df <- df %>% select(starts_with(“a”))
Selecting columns that contain ‘b’
selected_df <- df %>% select(contains(“b”))
“`
Combining Selection Methods
You can combine multiple selection methods to create more complex queries. This allows for a tailored approach to selecting columns based on various criteria.
“`R
Selecting columns that start with ‘a’ and contain ‘r’
selected_df <- df %>% select(starts_with(“a”), contains(“r”))
“`
Excluding Columns
In addition to selecting columns, you may want to exclude certain columns from your selection. This can be achieved using the `-` operator in conjunction with the column names.
“`R
Excluding column ‘b’
selected_df <- df %>% select(-b)
“`
Renaming Selected Columns
When selecting columns, you can also rename them on the fly using the `rename()` function alongside `select()`. This is particularly useful for improving clarity in your data frame.
“`R
Renaming selected columns
selected_df <- df %>% select(a, b) %>% rename(A_Column = a, B_Column = b)
“`
Conclusion on Selecting Columns
Using named selections and helper functions in dplyr enhances the efficiency and readability of your data manipulation tasks. This method allows for more dynamic and clear code, making it easier to understand and maintain.
Expert Insights on Using `dplyr` Select Without Column Index
Dr. Emily Carter (Data Scientist, Analytics Innovations). “Using `dplyr`’s `select()` function without relying on column indices enhances code readability and maintainability. By referring to column names directly, data analysts can avoid confusion that arises from changes in the dataset structure, making their scripts more robust against future modifications.”
Michael Chen (Senior R Programmer, Data Solutions Inc.). “Selecting columns by name rather than index in `dplyr` not only improves clarity but also reduces the risk of errors. When working with large datasets, it’s easy to lose track of index positions, whereas named selections make the intention of the code immediately clear to anyone reviewing it.”
Sarah Johnson (R Language Consultant, StatTech Advisors). “Incorporating named selections in `dplyr` promotes better coding practices. It allows for easier debugging and collaboration among team members, as everyone can quickly understand which variables are being manipulated without having to reference the dataset directly.”
Frequently Asked Questions (FAQs)
What is the purpose of the `select` function in `dplyr`?
The `select` function in `dplyr` is used to choose specific columns from a data frame or tibble. It allows users to manipulate datasets by retaining only the relevant columns needed for analysis.
Can I select columns in `dplyr` without using their index?
Yes, you can select columns by their names rather than their indices. You can specify column names directly within the `select` function, making it easier to understand and maintain your code.
How do I select multiple columns by name in `dplyr`?
To select multiple columns by name, list the column names separated by commas within the `select` function. For example, `select(data, column1, column2, column3)` will retain only the specified columns.
Is it possible to exclude certain columns when using `select`?
Yes, you can exclude columns by using the `-` operator before the column name. For instance, `select(data, -column1)` will return all columns except `column1`.
Can I use regular expressions to select columns in `dplyr`?
Yes, `dplyr` allows the use of regular expressions with the `matches()` function within `select`. This enables you to select columns that match a specific pattern, enhancing flexibility in data manipulation.
What are some common functions used in conjunction with `select`?
Common functions used alongside `select` include `filter()` for subsetting rows, `mutate()` for creating new columns, and `arrange()` for sorting the data. These functions can be combined in a pipeline for efficient data processing.
The `dplyr` package in R is a powerful tool for data manipulation, and its `select()` function is particularly useful for subsetting data frames. While many users often rely on column indices to specify which columns to select, there are several advantages to using column names instead. Selecting columns by name enhances code readability and maintainability, making it easier for others (or even oneself at a later date) to understand the intent behind the code. Additionally, using column names helps to avoid errors that can arise from changes in the data frame’s structure, such as when columns are added or removed.
Another key benefit of using column names in the `select()` function is the ability to leverage tidy evaluation features. This allows for more dynamic programming capabilities, such as selecting columns based on certain conditions or patterns. For example, one can use functions like `starts_with()`, `ends_with()`, or `contains()` to select multiple columns that meet specific criteria without needing to reference their indices explicitly. This not only streamlines the code but also makes it more flexible and adaptable to changes in the data.
while using column indices in the `dplyr` select function may seem convenient, opting for column names provides significant advantages
Author Profile
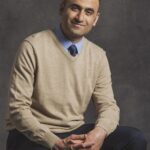
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?