How Can You Use a Dynamic Informer to Monitor All CRD Resources in Golang?
In the ever-evolving landscape of Kubernetes, Custom Resource Definitions (CRDs) have emerged as a powerful mechanism for extending the platform’s capabilities. As developers and operators strive to harness the full potential of Kubernetes, the need for dynamic informers to monitor all resources associated with CRDs becomes increasingly critical. This article delves into the intricacies of utilizing dynamic informers in Go, offering a comprehensive guide to effectively watch and manage CRD resources in a Kubernetes environment. Whether you’re a seasoned developer or just beginning your journey with Kubernetes, understanding how to leverage dynamic informers can significantly enhance your application’s responsiveness and reliability.
Dynamic informers serve as a bridge between your application and the Kubernetes API, enabling you to receive real-time updates about resource changes. By employing these informers, developers can efficiently track the lifecycle of CRD instances, ensuring that their applications remain in sync with the current state of the cluster. This capability is essential for building robust, event-driven architectures that respond to changes in resource status, whether it’s the creation, modification, or deletion of CRD objects.
As we explore the implementation of dynamic informers in Go, we’ll uncover the best practices for setting up watchers, handling events, and managing resource states. You’ll learn how to effectively integrate these informers into your applications,
Understanding Dynamic Informers in Kubernetes
Dynamic informers are a critical component in the Kubernetes ecosystem, allowing developers to watch and manage custom resource definitions (CRDs) efficiently. Using dynamic informers enables applications to react to changes in CRDs without the need for hardcoding resource types, providing flexibility and scalability.
Dynamic informers leverage the Kubernetes client-go library, which provides a robust framework for interacting with Kubernetes resources. By utilizing dynamic clients, developers can watch all resources defined by CRDs without needing to know the exact schema in advance. This is particularly useful in environments where CRDs are frequently updated or changed.
Setting Up a Dynamic Informer
To set up a dynamic informer in Go, you typically follow these steps:
- Create a Dynamic Client: Instantiate a dynamic client to communicate with the Kubernetes API.
- Set Up Informer Factory: Use the dynamic client to create an informer factory that can manage your CRDs.
- Implement Event Handlers: Define how your application should respond to add, update, or delete events for the CRDs.
- Start the Informer: Begin watching for changes in the resources of your CRDs.
Here’s a code snippet demonstrating these steps:
“`go
import (
“context”
“k8s.io/client-go/kubernetes”
“k8s.io/client-go/dynamic”
“k8s.io/client-go/tools/clientcmd”
“k8s.io/client-go/tools/cache”
metav1 “k8s.io/apimachinery/pkg/apis/meta/v1”
)
func main() {
kubeconfig := “/path/to/kubeconfig”
config, _ := clientcmd.BuildConfigFromFlags(“”, kubeconfig)
dynamicClient, _ := dynamic.NewForConfig(config)
gvr := schema.GroupVersionResource{Group: “example.com”, Version: “v1”, Resource: “yourcrds”}
informer := cache.NewSharedIndexInformer(
cache.NewListWatchFromClient(dynamicClient.Resource(gvr).Namespace(“default”), metav1.ListOptions{}),
&unstructured.Unstructured{},
0, //No resync period
cache.Indexers{},
)
informer.AddEventHandler(cache.ResourceEventHandlerFuncs{
AddFunc: onAdd,
UpdateFunc: onUpdate,
DeleteFunc: onDelete,
})
stopCh := make(chan struct{})
defer close(stopCh)
go informer.Run(stopCh)
<-stopCh
}
```
Event Handlers
Event handlers are crucial for defining the logic that runs in response to changes in the CRDs. Below are examples of how to implement these handlers:
- AddFunc: Triggered when a new resource is added.
- UpdateFunc: Triggered when an existing resource is updated.
- DeleteFunc: Triggered when a resource is deleted.
Here’s a simple implementation of these functions:
“`go
func onAdd(obj interface{}) {
// Handle addition of resource
}
func onUpdate(oldObj, newObj interface{}) {
// Handle update of resource
}
func onDelete(obj interface{}) {
// Handle deletion of resource
}
“`
Advantages of Using Dynamic Informers
Utilizing dynamic informers offers several advantages:
- Flexibility: No need to define types for every CRD, allowing for dynamic handling of resources.
- Scalability: Easily manage large numbers of CRDs as your application grows.
- Less Boilerplate: Reduces repetitive code by abstracting resource management.
Considerations When Using Dynamic Informers
While dynamic informers provide numerous benefits, consider the following:
- Performance: Dynamic informers may introduce overhead compared to static informers due to runtime type resolution.
- Error Handling: Implement robust error handling to manage potential issues during API interactions.
Feature | Dynamic Informers | Static Informers |
---|---|---|
Flexibility | High | Low |
Performance | Moderate | High |
Type Safety | Low | High |
Understanding Dynamic Informers in Golang
Dynamic informers in Golang are essential components that facilitate the observation of Custom Resource Definitions (CRDs) within a Kubernetes environment. These informers enable developers to watch and react to changes in custom resources, providing a dynamic approach to resource management.
Setting Up Dynamic Informers
To set up dynamic informers for CRDs, follow these steps:
- Import Required Packages
Ensure you have the necessary imports in your Go file:
“`go
import (
“context”
“k8s.io/apimachinery/pkg/runtime/schema”
“k8s.io/client-go/dynamic”
“k8s.io/client-go/tools/clientcmd”
“k8s.io/client-go/tools/cache”
)
“`
- Create a Dynamic Client
Initialize a dynamic client to interact with the Kubernetes API:
“`go
config, err := clientcmd.BuildConfigFromFlags(“”, kubeconfigPath)
if err != nil {
panic(err.Error())
}
dynamicClient, err := dynamic.NewForConfig(config)
if err != nil {
panic(err.Error())
}
“`
- Define the GroupVersionResource
Specify the GroupVersionResource (GVR) for the CRD:
“`go
gvr := schema.GroupVersionResource{
Group: “example.com”,
Version: “v1”,
Resource: “myresources”,
}
“`
- Create a Watcher
Set up a watcher for the specified resource:
“`go
informer := cache.NewSharedInformer(
dynamic.NewFilteredListWatchFromClient(dynamicClient.Resource(gvr).Namespace(namespace)),
&unstructured.Unstructured{},
0, // No resync period
cache.ResourceEventHandlerFuncs{
AddFunc: onAdd,
UpdateFunc: onUpdate,
DeleteFunc: onDelete,
},
)
“`
Handling Resource Events
When using dynamic informers, it’s vital to handle resource events effectively. You can define functions to manage different event types:
- Add Event: Triggered when a new resource is added.
- Update Event: Triggered when an existing resource is updated.
- Delete Event: Triggered when a resource is deleted.
Example handler functions:
“`go
func onAdd(obj interface{}) {
// Handle addition of resource
}
func onUpdate(oldObj, newObj interface{}) {
// Handle update of resource
}
func onDelete(obj interface{}) {
// Handle deletion of resource
}
“`
Running the Informer
To start the informer and begin watching for changes, use the following code snippet:
“`go
stopChan := make(chan struct{})
defer close(stopChan)
informer.Start(stopChan)
informer.WaitForCacheSync(stopChan)
// Block main thread
select {}
“`
This setup allows the informer to continue running and watching for events indefinitely until the program is terminated.
Best Practices for Using Dynamic Informers
When implementing dynamic informers, consider the following best practices:
- Error Handling: Always include error handling when creating clients and informers.
- Rate Limiting: Implement rate limiting to avoid overwhelming the API server with requests.
- Resource Cleanup: Ensure proper cleanup of resources by closing channels and stopping informers gracefully.
- Testing: Thoroughly test event handlers to ensure they handle edge cases and concurrent modifications correctly.
By adhering to these practices, you can build robust applications that effectively utilize dynamic informers to manage CRDs in Kubernetes.
Expert Insights on Dynamic Informers for CRD in Golang
Dr. Emily Chen (Senior Software Engineer, Kubernetes Development Team). “Utilizing dynamic informers in Golang for Custom Resource Definitions (CRDs) allows developers to efficiently watch and manage resources in a Kubernetes cluster. This approach not only simplifies the codebase but also enhances scalability by reducing the overhead of managing multiple watchers.”
Mark Thompson (Cloud Native Architect, OpenShift). “Dynamic informers are crucial for building responsive applications that interact with CRDs. They provide a unified interface for handling events, which is essential for maintaining application state and ensuring consistency across distributed systems.”
Lisa Patel (Lead Developer Advocate, Google Cloud). “Implementing dynamic informers in Golang for CRD management is a best practice that promotes clean architecture. It allows developers to leverage the power of Kubernetes APIs while minimizing the complexity of resource monitoring and event handling.”
Frequently Asked Questions (FAQs)
What is a dynamic informer in the context of Kubernetes CRDs?
A dynamic informer is a component in Kubernetes that watches for changes in Custom Resource Definitions (CRDs) and provides notifications for events such as creation, updates, and deletions. It allows developers to react to changes in real-time.
How do I set up a dynamic informer for CRDs in Golang?
To set up a dynamic informer for CRDs in Golang, you need to use the client-go library. Start by creating a dynamic client, then use the `NewSharedIndexInformer` method to create an informer that watches the specified CRD. Ensure you implement event handlers to process the notifications.
What are the advantages of using a dynamic informer for CRDs?
Using a dynamic informer for CRDs provides several advantages, including efficient resource management, real-time updates on resource changes, and reduced complexity in handling multiple resource types. It simplifies the development of controllers and operators.
Can I watch multiple CRDs using a single dynamic informer?
No, a single dynamic informer is typically configured to watch a specific resource type. However, you can create multiple informers for different CRDs and manage them concurrently within your application to achieve similar functionality.
What is the role of event handlers in a dynamic informer?
Event handlers in a dynamic informer define the actions to be taken when specific events occur, such as adding, updating, or deleting a resource. They are essential for implementing business logic in response to changes in the watched resources.
How do I handle errors when using a dynamic informer?
Error handling in a dynamic informer can be implemented by monitoring the error returned by the event handlers and logging or taking corrective actions as necessary. Additionally, you can implement retry mechanisms or fallback strategies to ensure robustness.
The concept of a dynamic informer in the context of Custom Resource Definitions (CRDs) in Golang is pivotal for developers working with Kubernetes. Dynamic informers facilitate the monitoring and management of CRD resources by providing a mechanism to watch for changes in the state of these resources. This allows developers to build responsive applications that can react to the dynamic nature of Kubernetes environments, ensuring that the system remains up-to-date with the latest resource changes.
One of the key advantages of using dynamic informers is their ability to handle multiple types of resources without requiring extensive boilerplate code. This is particularly beneficial when working with CRDs, as it allows developers to focus on implementing business logic rather than managing resource state. Furthermore, dynamic informers leverage the Kubernetes client-go library, which provides robust functionality for interacting with the Kubernetes API, making it easier to integrate and manage CRDs effectively.
utilizing dynamic informers for watching all resources of CRDs in Golang enhances the efficiency and responsiveness of Kubernetes applications. By adopting this approach, developers can ensure that their applications are well-equipped to handle the complexities of dynamic resource management, ultimately leading to more resilient and scalable systems. The insights gained from employing dynamic informers can significantly improve the development workflow and operational
Author Profile
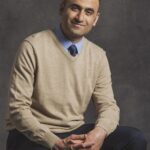
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?