Why Am I Encountering EOF When Reading a Line?
In the world of programming and data processing, encountering the phrase “EOF when reading a line” can send shivers down the spine of even the most seasoned developers. This seemingly cryptic message often signals an unexpected end of file condition, leaving programmers grappling with the implications of incomplete data and the potential for bugs in their code. As we delve into this topic, we’ll unravel the mysteries behind EOF errors, explore their causes, and discuss effective strategies for handling them. Whether you’re a novice coder or a veteran in the field, understanding EOF conditions is crucial for building robust applications that can gracefully manage input and output operations.
EOF, or End Of File, is a term that signifies the termination of a data stream. When reading a line from a file or input stream, encountering an EOF unexpectedly can lead to various issues, such as incomplete data processing or runtime errors. This situation often arises in programming languages that rely on stream-based input, where the program expects more data to be available but instead finds that the stream has concluded. Understanding how and why EOF errors occur is essential for debugging and ensuring that your applications can handle data input smoothly.
The implications of an EOF error extend beyond mere inconvenience; they can affect the integrity of data processing workflows and lead to significant disruptions
Understanding EOF in Line Reading
When working with file input in programming, encountering an “EOF” (End of File) condition while reading a line indicates that the end of the file has been reached. This situation can occur in various programming languages when attempting to read data that is no longer available.
EOF is crucial in file handling because it helps in managing data flow and ensuring that programs do not attempt to read beyond the available data. Handling EOF properly is essential for robust programming, especially in applications that require file I/O operations.
Common Causes of EOF When Reading a Line
Several factors can lead to an EOF condition when reading a line from a file:
- File Completeness: The file being read has no more data available to read. This is the most straightforward cause of EOF.
- Improper Handling of Input Streams: In some cases, the input stream may not be correctly managed, leading to premature EOF detection.
- Incorrect Loop Structures: While reading lines in a loop, an error in the loop condition can cause the program to attempt reading beyond the last line.
Handling EOF Gracefully
To handle EOF gracefully, programmers can implement certain strategies in their code:
- Use exception handling to catch EOF exceptions and handle them appropriately.
- Check for EOF explicitly before reading a new line.
- Implement loop structures that properly check for the end of the file.
Example of handling EOF in Python:
“`python
try:
with open(‘file.txt’, ‘r’) as file:
for line in file:
process(line.strip())
except EOFError:
print(“Reached end of file.”)
“`
Best Practices for EOF Handling
Adhering to best practices can help avoid issues related to EOF:
- Always validate file accessibility before reading.
- Employ context managers where available to ensure files are closed properly after their use.
- Utilize built-in functions or methods that automatically handle EOF conditions.
EOF in Different Programming Languages
Different programming languages have unique ways of handling EOF. Below is a comparison of how EOF is managed in several popular languages:
Language | EOF Handling Method |
---|---|
Python | Uses `EOFError` exception; iterates through files directly. |
Java | Utilizes `Scanner` class or `BufferedReader`, returning `null` at EOF. |
C | Uses `feof()` function to check for EOF after reading. |
JavaScript | File reading through `FileReader` API with event listeners for completion. |
Understanding and managing EOF conditions is essential for creating efficient and error-free file handling routines in programming. By following best practices and utilizing language-specific features, developers can ensure their applications function reliably, even in the presence of EOF situations.
Understanding EOF in Reading Lines
EOF, or End of File, is a condition in computer programming that indicates no more data can be read from a data source. In the context of reading lines from a file or input stream, encountering an EOF condition means that the program has reached the end of the data available for reading.
When a program attempts to read a line and encounters EOF unexpectedly, it can lead to various issues depending on the programming language and the method used for reading. Here are some key points to consider:
- EOF Handling: Different programming languages have distinct ways to handle EOF. For instance:
- In Python, reading a line from a file using `file.readline()` will return an empty string when EOF is reached.
- In C, functions like `fgets()` return `NULL` when EOF is encountered.
- Error Handling: It is crucial to implement error handling when reading lines:
- Check for EOF before processing data to prevent runtime errors.
- Use try-except blocks in languages like Python to gracefully handle EOF exceptions.
- Common Causes of EOF Issues:
- Attempting to read beyond the available data in the input stream.
- File corruption or misformatted data that alters the expected flow of reading.
- Misconfigured file pointers that do not point to the correct position in the file.
Examples of EOF Handling
Below are examples of how EOF is handled in different programming languages:
Language | Code Example | Description |
---|---|---|
Python | “`python with open(‘file.txt’, ‘r’) as f: line = f.readline() while line: print(line) line = f.readline()“` |
Reads lines until EOF is reached, printing each line. |
C | “`c include int main() { FILE *file = fopen(“file.txt”, “r”); char buffer[256]; while (fgets(buffer, sizeof(buffer), file) != NULL) { printf(“%s”, buffer); } fclose(file); } “` |
Reads and prints lines until EOF is encountered. |
Java | “`java import java.io.*; public class ReadFile { public static void main(String[] args) throws IOException { BufferedReader reader = new BufferedReader(new FileReader(“file.txt”)); String line; while ((line = reader.readLine()) != null) { System.out.println(line); } reader.close(); } } “` |
Uses a BufferedReader to read lines until EOF. |
Best Practices for EOF Management
To effectively manage EOF when reading lines, consider implementing the following best practices:
- Use Buffered Reading: Buffered reading can improve performance and handle EOF conditions more efficiently.
- Validate Input Sources: Ensure that the input files or streams are correctly formatted and accessible.
- Graceful Exit: Implement logic to exit loops gracefully when EOF is encountered, avoiding infinite loops or crashes.
- Logging: Maintain logs for debugging purposes, particularly when reading large files or streams, to trace EOF encounters.
By adhering to these practices, developers can minimize issues related to EOF when reading lines and ensure robust handling of data inputs.
Understanding EOF Issues in Line Reading
Dr. Emily Carter (Computer Science Professor, Tech University). “EOF, or End of File, is a critical concept in file handling. When reading a line, encountering EOF indicates that there are no more data to read, which can lead to unexpected behavior if not properly handled in the code.”
Michael Chen (Software Engineer, CodeCraft Solutions). “Handling EOF correctly is essential in programming. It is important to implement checks to ensure that your program can gracefully terminate or handle the absence of data without crashing.”
Linda Gomez (Data Analyst, Insight Analytics). “In data processing, EOF errors can disrupt workflows. It is advisable to implement robust error handling mechanisms to manage EOF scenarios, ensuring that data integrity is maintained throughout the reading process.”
Frequently Asked Questions (FAQs)
What does “EOF” stand for in programming?
EOF stands for “End of File.” It is a condition in a computer operating system that indicates no more data can be read from a data source, such as a file or input stream.
Why does an “EOF when reading a line” error occur?
This error occurs when a program attempts to read a line of input but reaches the end of the input stream unexpectedly, indicating that there is no more data to read.
How can I handle EOF errors in my code?
You can handle EOF errors by implementing error-checking mechanisms, such as using try-except blocks in Python or checking return values in languages like C or Java, to gracefully manage situations where input is not available.
What are common scenarios that lead to EOF errors?
Common scenarios include reading from an empty file, reaching the end of user input in a console application, or improperly closing file streams before all data has been read.
Can EOF errors be prevented during file reading?
Yes, EOF errors can be minimized by ensuring that files are properly formatted, validating input before reading, and using appropriate file handling techniques to check for the end of the stream.
Is EOF handling the same across different programming languages?
While the concept of EOF is universal, the methods to handle EOF conditions can vary significantly between programming languages, including syntax and available functions for reading input.
The term “EOF when reading a line” refers to the End Of File (EOF) condition encountered while attempting to read input from a file or standard input stream. This situation typically arises in programming contexts where a program expects more data to be available for processing but instead reaches the end of the input source. Understanding EOF is crucial for effective error handling and ensuring that programs can gracefully manage unexpected input scenarios.
One of the primary considerations when dealing with EOF is the implementation of robust input validation and error handling mechanisms. Developers must anticipate the possibility of reaching EOF and implement logic to handle this condition appropriately. This includes checking for EOF before attempting to read data, which can prevent runtime errors and enhance the stability of the application.
Additionally, EOF handling is essential for various programming languages and environments, as the way EOF is detected and managed can vary. Familiarity with the specific functions and methods used to read input in a given programming language is vital. This knowledge allows developers to write more efficient and error-resistant code, ultimately leading to better user experiences and more reliable applications.
Author Profile
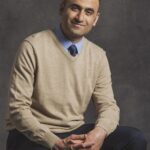
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?