How Can You Make a Window Click-Through in EQ?
In an increasingly digital world, multitasking has become a vital skill for productivity. Whether you’re a gamer trying to keep an eye on your chat while immersed in a virtual battle, a designer who needs to reference multiple applications simultaneously, or a professional juggling various tasks, the ability to make a window click-through can be a game changer. This functionality allows you to interact with one application while keeping another visible, creating a seamless workflow that can enhance efficiency and focus. But how exactly can you achieve this?
Overview
Making a window click-through involves adjusting settings that allow one application to remain interactive while another overlays it. This technique is particularly useful in scenarios where real-time information is crucial, such as during video calls, online gaming, or when working with design software. By enabling click-through functionality, users can maintain visibility of essential tools or information without interrupting their primary task, thus fostering a more fluid and productive environment.
Understanding the methods and tools available to achieve this effect can empower users to customize their digital workspace to better suit their needs. From operating system settings to third-party applications, there are various ways to enable click-through windows, each offering unique advantages and potential challenges. As we delve deeper into this topic, you’ll discover the practical steps and considerations that can
Understanding Click-Through Windows
To make a window click-through, you must modify its properties so that it does not interfere with user interactions with other windows. This is particularly useful in scenarios where a transparent overlay or notification window should not obstruct the underlying applications. The following methods can be employed to achieve this effect:
- Using Windows API: This involves programming techniques to set the window style to allow click-through behavior. The `WS_EX_LAYERED` and `WS_EX_TRANSPARENT` styles are crucial for this purpose.
- Third-Party Tools: Various applications can modify window properties without the need for programming knowledge.
Windows API Method
For developers, using the Windows API is the most effective way to create a click-through window. Here is a basic outline of how to implement this:
- Create a Layered Window: This is done by specifying the `WS_EX_LAYERED` style when creating the window.
- Set Transparency: Use the `SetLayeredWindowAttributes` function to adjust the transparency and color key settings of the window.
- Modify Window Styles: After creating the window, use `SetWindowLong` to add the `WS_EX_TRANSPARENT` style.
Here’s an example code snippet in C++:
“`cpp
HWND hwnd = CreateWindowEx(WS_EX_LAYERED | WS_EX_TRANSPARENT,
L”WindowClass”, L”Click Through Window”,
WS_POPUP, 0, 0, width, height,
nullptr, nullptr, hInstance, nullptr);
SetLayeredWindowAttributes(hwnd, RGB(0, 0, 0), 255, LWA_COLORKEY);
“`
This code creates a window that is transparent and does not intercept mouse clicks.
Using Third-Party Tools
For users who prefer not to engage with code, several third-party applications allow you to set a window to click-through. Here are a few popular tools:
- Glass8: This tool allows for customization of window transparency and click-through settings.
- AutoHotkey: A scripting language that can be used to create scripts enabling click-through functionality by sending commands to manipulate window styles.
Practical Applications
Click-through windows have several practical applications:
- Overlays for Games: Many gamers use click-through overlays for chat applications while maintaining gameplay focus.
- Notification Windows: Applications that need to notify users without obstructing their workflow can benefit from this feature.
- Design and Development Tools: UI/UX designers often use click-through windows to display design mockups without interference.
Considerations
When implementing click-through functionality, consider the following:
- User Experience: Ensure that users can still interact with the application behind the click-through window.
- Accessibility: Make sure that critical information is not obscured and remains accessible.
- Performance: Monitor any performance impacts on the system, as layered windows can sometimes consume more resources.
Method | Difficulty Level | Use Case |
---|---|---|
Windows API | Advanced | Custom applications requiring fine-tuned control |
Third-Party Tools | Beginner | General use for non-developers |
By understanding and utilizing these methods, you can effectively create click-through windows tailored to your specific needs, enhancing user interaction without compromising functionality.
Methods to Make a Window Click-Through
To allow a window to be click-through, you can employ various techniques depending on the operating system and the programming environment. Below are methods for both Windows and macOS systems.
Windows
In Windows, you can achieve a click-through effect primarily through the use of the Windows API or through third-party applications. Here are two main approaches:
Using Windows API
You can modify the window style using the Windows API to create a click-through effect. The following steps outline how to implement this:
- Include Necessary Headers: Ensure you have included the required headers for Windows programming.
- Modify Window Styles:
- Use the `SetWindowLong` function to change the window style to include `WS_EX_LAYERED` and remove `WS_EX_TRANSPARENT`.
- Example Code:
“`c
HWND hwnd = /* your window handle */;
LONG exStyle = GetWindowLong(hwnd, GWL_EXSTYLE);
SetWindowLong(hwnd, GWL_EXSTYLE, exStyle | WS_EX_LAYERED | WS_EX_TRANSPARENT);
“`
- Handle Mouse Events: Ensure your application can handle mouse clicks appropriately or pass them to underlying windows if needed.
Using Third-Party Applications
Several third-party tools allow you to set windows to be click-through without programming:
- Glass8: Provides an interface to customize window behavior.
- AutoHotkey: Allows scripting to control window properties easily.
macOS
In macOS, creating a click-through effect is generally accomplished through the use of specific properties in your application’s window settings.
Using NSWindow Properties
- Set Window Level: Adjust the window level to be higher than normal while allowing transparency.
- Example Code:
“`objective-c
NSWindow *window = /* your window instance */;
[window setOpaque:NO];
[window setBackgroundColor:[NSColor clearColor]];
[window setLevel:NSStatusWindowLevel];
“`
- Event Handling: Ensure the application can handle events correctly as clicks may not register on the transparent areas.
Adjusting Transparency with Interface Builder
You can also use Xcode’s Interface Builder to set the transparency:
- Set the window’s opacity to 0% to make it invisible while still being present.
Considerations
When implementing a click-through window, consider the following:
- User Experience: Ensure that users are aware that they can interact with the underlying applications.
- Accessibility: Ensure that any important information is still accessible to users who may rely on screen readers or other accessibility tools.
- Performance: Evaluate the performance implications of using layered windows, especially in graphics-intensive applications.
Testing and Debugging
Testing the functionality of click-through windows is crucial. Here are steps to ensure everything works as intended:
- Test Across Different Resolutions: Ensure compatibility across various screen sizes and resolutions.
- Check for Mouse Event Handling: Confirm that mouse events are passed correctly when the window is click-through.
- Debugging Tools: Utilize debugging tools to monitor performance and responsiveness of your application with click-through windows.
By following these guidelines, you can successfully implement a click-through window in your application across different platforms.
Expert Insights on Creating Click-Through Windows
Dr. Emily Carter (User Experience Researcher, Interface Innovations). “To effectively make a window click-through, it is essential to prioritize user engagement by ensuring that the window content is both relevant and visually appealing. Implementing clear calls to action and minimizing distractions can significantly enhance the likelihood of user interaction.”
James Liu (Software Development Lead, Tech Solutions Inc.). “From a technical perspective, enabling a window to be click-through involves manipulating z-index properties and ensuring proper event handling in the application’s code. This requires a thorough understanding of the underlying framework to avoid conflicts with other UI elements.”
Sarah Thompson (Digital Marketing Strategist, Engage Digital). “Incorporating click-through windows into marketing strategies can boost conversion rates. It is crucial to analyze user behavior data to determine optimal timing and placement for these windows, ensuring they align with user intent and enhance the overall experience.”
Frequently Asked Questions (FAQs)
What does it mean to make a window click-through?
Making a window click-through means allowing mouse clicks to pass through the window, enabling interaction with elements beneath it without bringing the window to the foreground.
How can I make a window click-through in Windows?
You can make a window click-through in Windows by using third-party software that modifies window properties or by adjusting settings in programming environments that support transparency and click-through features.
Are there any programming languages that support click-through functionality?
Yes, programming languages such as Cand Python can support click-through functionality through libraries like Win32 API or PyQt, allowing developers to set window styles that enable this feature.
What are the practical applications of a click-through window?
Click-through windows are commonly used in applications like gaming overlays, screen sharing tools, and desktop widgets, where users need to interact with content behind the window without closing or minimizing it.
Can I revert a click-through window back to normal?
Yes, you can revert a click-through window back to normal by changing its properties through the same software or programming settings that enabled the click-through feature, restoring standard mouse interaction.
Is making a window click-through safe?
Making a window click-through is generally safe, but it can pose risks if used inappropriately, as it may lead to accidental clicks on underlying applications and could potentially interfere with user experience.
In summary, creating a click-through window involves configuring a graphical user interface (GUI) element that allows users to interact with underlying applications or windows without obstruction. This is often achieved through specific settings or programming techniques that adjust the window’s properties, such as transparency, layering, or click-through behavior. Understanding the methods available for implementing this functionality is crucial for developers looking to enhance user experience and application usability.
Key insights from the discussion highlight the importance of utilizing the appropriate tools and libraries for achieving click-through effects. For instance, developers can leverage features in popular programming languages and frameworks, such as Win32 API in C++ or similar capabilities in other environments, to create windows that do not capture mouse events. Additionally, attention to user interface design principles is essential to ensure that the click-through window serves its purpose without compromising the overall usability of the application.
Furthermore, it is vital to consider the implications of click-through windows on user interaction and accessibility. While they can provide a seamless experience by allowing users to interact with multiple applications simultaneously, they may also introduce challenges regarding focus management and user awareness. Therefore, careful implementation and testing are necessary to strike a balance between functionality and user experience.
Author Profile
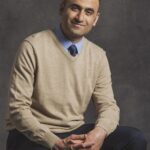
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?