How Do I Resolve the ‘Error: Cannot Find Module ‘discord.js” Issue?
In the dynamic world of Discord bot development, encountering errors is an inevitable part of the journey. One of the most common hurdles developers face is the dreaded message: “error: cannot find module ‘discord.js’.” This seemingly simple error can halt your progress and leave you scratching your head, especially if you’re eager to bring your bot to life. Understanding the root causes of this issue is crucial for both novice and experienced developers alike, as it can save you time and frustration in the long run.
In this article, we will delve into the intricacies behind this error message, exploring its common triggers and the steps you can take to resolve it. Whether you’re working on your first bot or refining an existing project, recognizing how to navigate module dependencies in Node.js is essential. We’ll also highlight best practices for managing your project’s environment, ensuring that you can focus on building engaging and interactive experiences for your Discord community without getting bogged down by technical setbacks.
Join us as we unravel the mysteries of module management in Discord bot development, equipping you with the knowledge and tools to overcome this error and enhance your coding journey. With the right insights, you’ll be well on your way to creating seamless and functional bots that captivate users and elevate your programming skills.
Common Causes of the ‘Cannot Find Module’ Error
The error message “cannot find module ‘discord.js'” typically indicates that the Node.js runtime is unable to locate the specified module. This can arise from several common issues:
- Module Not Installed: The `discord.js` module may not be installed in your project. This is often the first point of troubleshooting.
- Incorrect Path: The import path may be incorrect. Ensure that the path you are using to require or import the module is correct.
- Node Modules Missing: Sometimes, the `node_modules` folder can be incomplete or corrupted, leading to missing modules.
- Version Compatibility: The installed version of `discord.js` might not be compatible with your version of Node.js.
- Global vs. Local Installation: If you installed the module globally but are trying to use it in a local project, Node.js may not find it.
Steps to Resolve the Error
To address the “cannot find module” error, follow these steps:
- Check if the Module is Installed:
Run the following command in your terminal to verify that `discord.js` is listed among your dependencies:
“`bash
npm list discord.js
“`
- Install the Module:
If it is not installed, you can add it to your project by executing:
“`bash
npm install discord.js
“`
- Verify the Import Statement:
Ensure that your import or require statement is correct. It should look something like:
“`javascript
const Discord = require(‘discord.js’);
“`
- Reinstall Node Modules:
If you suspect the `node_modules` folder is corrupted, delete it and reinstall:
“`bash
rm -rf node_modules
npm install
“`
- Check Node.js Version:
Ensure your Node.js version is compatible with the version of `discord.js` you are trying to use. You can check your Node.js version with:
“`bash
node -v
“`
- Use Correct Node Environment:
If you are using a version manager like `nvm`, ensure you are using the correct Node.js version for your project.
Module Installation Table
Here’s a brief overview of the commands for module installation and verification:
Action | Command |
---|---|
Check Installed Modules | npm list discord.js |
Install discord.js | npm install discord.js |
Remove Node Modules | rm -rf node_modules |
Reinstall Node Modules | npm install |
By following these steps and utilizing the table above, you should be able to effectively resolve the “cannot find module ‘discord.js'” error and continue developing your application seamlessly.
Common Causes of the ‘Cannot Find Module’ Error
The ‘error: cannot find module ‘discord.js” typically arises from several common issues related to the setup and configuration of your Node.js project. Understanding these causes can help you troubleshoot effectively.
- Module Not Installed: The most straightforward reason for this error is that the `discord.js` package has not been installed in your project.
- Incorrect Installation Location: If `discord.js` was installed globally but your project is looking for a local module, this discrepancy can trigger the error.
- Node Modules Folder Missing: If the `node_modules` directory is missing from your project, it indicates that dependencies have not been installed correctly.
- Corrupted Installation: Sometimes, the installation of the package may become corrupted, leading to issues where the module cannot be found.
- Package.json Misconfiguration: If the `package.json` file does not list `discord.js` as a dependency, Node.js will not be able to locate it.
Resolving the Error
To resolve the ‘cannot find module ‘discord.js” error, follow these steps:
- Install `discord.js`:
- Open your terminal and navigate to your project directory.
- Run the command:
“`bash
npm install discord.js
“`
- Check Installation Location:
- Confirm that you are installing `discord.js` in the correct directory. Use:
“`bash
npm list discord.js
“`
- This command will show if the module is installed and where it is located.
- Verify `node_modules` Folder:
- Ensure that the `node_modules` folder exists in your project directory. If it does not, run:
“`bash
npm install
“`
- Clear npm Cache:
- If the installation seems corrupt, clearing the npm cache may help:
“`bash
npm cache clean –force
“`
- Reinstall Dependencies:
- If issues persist, you can reinstall all project dependencies:
“`bash
rm -rf node_modules
npm install
“`
Best Practices for Managing Dependencies
To avoid encountering module-related errors in the future, adhere to the following best practices:
- Use a Package Manager: Always use npm or yarn for managing your Node.js packages. This ensures proper installation and dependency management.
- Keep Dependencies Updated:
- Regularly update your dependencies to avoid compatibility issues. Use:
“`bash
npm update
“`
- Check Compatibility: When using multiple libraries, check for compatibility between versions, especially major updates.
- Use a `.gitignore` File: Include `node_modules` in your `.gitignore` file to prevent it from being tracked by version control systems like Git.
- Run Scripts from the Project Root: Ensure you run commands from the root of your project where `package.json` is located to maintain the correct context.
Troubleshooting Tips
If you continue to experience issues even after following the above steps, consider the following troubleshooting tips:
Issue | Suggested Action |
---|---|
Error persists after installation | Double-check if your project structure is correct. |
Version mismatch | Check compatibility between Node.js and `discord.js`. |
Running in the wrong environment | Ensure you are in the correct environment (e.g., local vs. production). |
Utilizing these strategies will aid in effectively resolving the ‘cannot find module ‘discord.js” error and ensuring a smoother development experience with Node.js and Discord bots.
Resolving the ‘Cannot Find Module’ Error in Discord.js
Dr. Emily Carter (Senior Software Engineer, Node.js Foundation). “The error ‘cannot find module ‘discord.js” typically indicates that the module is not installed in your project. Ensure that you have run ‘npm install discord.js’ in your project directory. Additionally, check your package.json file to confirm that the dependency is listed.”
James Liu (Full Stack Developer, Tech Innovations Inc.). “This error can also arise if there are issues with the Node.js environment. Make sure that your Node.js version is compatible with the version of Discord.js you are trying to use. Sometimes, clearing the npm cache with ‘npm cache clean –force’ can resolve hidden issues.”
Sarah Thompson (Lead Developer, Open Source Community). “If you are working in a monorepo or using workspaces, ensure that the module is installed in the correct location. You may need to navigate to the specific package directory and run the installation command there. Always verify your import paths as well to avoid misconfigurations.”
Frequently Asked Questions (FAQs)
What does the error “cannot find module ‘discord.js'” mean?
This error indicates that the Node.js runtime cannot locate the ‘discord.js’ library in your project. It typically occurs when the module is not installed or is improperly referenced in your code.
How can I resolve the “cannot find module ‘discord.js'” error?
To resolve this error, ensure that you have installed the ‘discord.js’ package by running `npm install discord.js` in your project directory. Verify that the installation completes without errors.
What should I check if I still encounter the error after installation?
If the error persists, check your project’s `node_modules` directory to confirm that ‘discord.js’ is present. Additionally, ensure that your `package.json` file lists ‘discord.js’ under dependencies.
Could the error occur due to incorrect file paths?
Yes, incorrect file paths can lead to this error. Ensure that you are using the correct path when requiring the module in your code, such as `require(‘discord.js’)`.
Does this error occur with other Node.js modules as well?
Yes, similar errors can occur with other Node.js modules if they are not installed or if there are issues with the module’s path or version. Always ensure proper installation and referencing.
What version of Node.js is required for ‘discord.js’?
‘discord.js’ requires Node.js version 16.9.0 or higher. Ensure your Node.js version meets this requirement to avoid compatibility issues.
The error message “cannot find module ‘discord.js'” typically indicates that the Node.js environment is unable to locate the Discord.js library, which is essential for developing Discord bots and applications. This issue often arises due to a few common reasons, including the library not being installed, incorrect file paths, or issues with the Node.js environment itself. Developers encountering this error should first verify that Discord.js is correctly installed in their project directory and that they are using the appropriate version of Node.js that is compatible with the library.
To resolve this error, one of the primary steps is to ensure that the Discord.js package is installed using npm (Node Package Manager). Running the command `npm install discord.js` in the terminal will install the library if it is not already present. Additionally, checking the project’s `package.json` file can provide insight into whether the library is listed as a dependency. If the library is indeed installed but the error persists, it may be necessary to check the import statements in the code to confirm that they are correctly referencing the installed module.
Furthermore, developers should consider the possibility of issues related to the Node.js environment itself, such as version mismatches or corrupted installations. In such cases, updating Node.js or reinstalling the
Author Profile
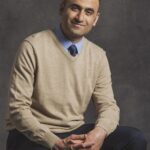
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?