Why Am I Seeing the Error: Cannot Find Module ‘node:util’?
In the ever-evolving landscape of JavaScript and Node.js, developers frequently encounter a myriad of challenges that can disrupt their workflow. One such issue that has sparked confusion and frustration is the error message: `error: cannot find module ‘node:util’`. This seemingly cryptic notification can halt your project in its tracks, leaving you to navigate through the intricacies of module management and dependencies. Understanding the root causes of this error is essential for any developer looking to maintain a smooth development process and leverage the full power of Node.js.
As you delve deeper into the world of Node.js, you’ll discover that module resolution is a fundamental aspect that can significantly impact your applications. The error in question often arises from misconfigurations, outdated dependencies, or even changes in the Node.js runtime itself. By unpacking the layers of this error, you can not only troubleshoot effectively but also gain insights into best practices for managing modules and dependencies in your projects.
In this article, we will explore the common scenarios that lead to the `cannot find module ‘node:util’` error, providing you with practical solutions and preventative measures. Whether you are a seasoned developer or just starting your journey with Node.js, understanding this error will empower you to create more robust applications and enhance your
Understanding the ‘node:util’ Module
The error message `error: cannot find module ‘node:util’` typically arises when Node.js cannot locate the specified module. The `node:util` module is a built-in module that provides utility functions for various operations, including debugging and formatting. It is essential for developers to ensure that their environment is correctly set up to utilize Node.js modules effectively.
Common Causes of the Error
Several factors may lead to this error. Understanding these can help in troubleshooting:
- Node.js Version: The `node:util` module was introduced in Node.js v14.0.0. If you are using an earlier version, the module will not be available.
- Incorrect Import Statement: The module should be imported correctly using the syntax `import { … } from ‘node:util’;` in ES modules or `const util = require(‘node:util’);` in CommonJS modules.
- Corrupt Installation: A corrupt Node.js installation could prevent the module from being found. This could happen due to interrupted installations or missing files.
Troubleshooting Steps
To resolve the issue, follow these steps:
- Check Node.js Version: Verify that you are using the correct version of Node.js. You can check your version by running:
“`bash
node -v
“`
If the version is below v14.0.0, consider upgrading.
- Update Package Dependencies: Sometimes, outdated packages might reference older module formats. Run:
“`bash
npm update
“`
- Reinstall Node.js: If the problem persists, try reinstalling Node.js. This ensures that all built-in modules are installed correctly.
- Use Correct Import Syntax: Ensure that your import statement aligns with the module system you are using. For example:
“`javascript
// ES Module
import { promisify } from ‘node:util’;
// CommonJS Module
const { promisify } = require(‘node:util’);
“`
Example Use Cases of the ‘node:util’ Module
The `node:util` module contains various utility functions that can simplify development tasks. Below are some common use cases:
Function | Description |
---|---|
`util.promisify` | Converts callback-based functions to return promises. |
`util.inspect` | Provides a string representation of objects, useful for debugging. |
`util.format` | Formats strings with placeholders, similar to `printf`. |
Best Practices
To avoid encountering the `error: cannot find module ‘node:util’`, consider the following best practices:
- Maintain Updated Environments: Regularly update Node.js and your project dependencies to the latest stable versions.
- Utilize Version Control: Implement version control for your projects to track changes and dependencies effectively.
- Read Documentation: Familiarize yourself with the Node.js documentation to understand module usage and best practices.
By adhering to these guidelines, you can minimize the likelihood of running into issues related to module imports and improve your development workflow.
Understanding the Error
The error message `error: cannot find module ‘node:util’` typically indicates that the Node.js runtime is unable to locate the specified module. This can occur due to several factors, including incorrect Node.js version, module path issues, or project configuration problems.
Common Causes
Several reasons might lead to this error:
- Node.js Version: The `node:` prefix for core modules was introduced in Node.js 14. If you are using an older version, the prefix will not be recognized.
- Module Path: If the module is incorrectly referenced, the runtime will not find it.
- Missing Dependencies: The required module might not be installed in the project.
- Corrupted Node Modules: Sometimes, the `node_modules` directory can become corrupted, leading to missing modules.
Resolution Steps
To resolve the `cannot find module ‘node:util’` error, follow these steps:
- Check Node.js Version:
- Run `node -v` in your terminal.
- If the version is below 14, update Node.js to a newer version.
- Correct Module Usage:
- Ensure you are using the correct syntax to import the module:
“`javascript
const util = require(‘node:util’);
“`
- Install Missing Dependencies:
- If your project requires additional packages, run:
“`bash
npm install
“`
- Reinstall Node Modules:
- Sometimes, simply reinstalling the modules resolves the issue:
“`bash
rm -rf node_modules
npm install
“`
Example of Correct Implementation
Here is a simple example demonstrating the correct usage of the `node:util` module:
“`javascript
const util = require(‘node:util’);
function formatString(str) {
return util.format(‘Formatted string: %s’, str);
}
console.log(formatString(‘Hello, World!’));
“`
Testing Your Environment
To ensure your environment is properly set up, consider executing the following commands:
Command | Purpose |
---|---|
`node -v` | Check the Node.js version. |
`npm list` | List installed packages in your project. |
`npm outdated` | Check for outdated packages. |
`npm audit` | Identify vulnerabilities in your dependencies. |
Further Troubleshooting
If the error persists after following the above steps, consider the following troubleshooting techniques:
- Check for Global vs Local Installation: Ensure that the module is installed in the correct scope.
- Verify Environment Variables: Ensure that the `NODE_PATH` environment variable is set correctly if you are using custom module paths.
- Look for Typos: Simple typographical errors in the module name can lead to this issue.
- Consult Documentation: Refer to the official Node.js documentation for any updates regarding module usage.
By following these guidelines, you should be able to resolve the `error: cannot find module ‘node:util’` effectively.
Understanding the ‘Cannot Find Module’ Error in Node.js
Dr. Emily Carter (Senior Software Engineer, NodeJS Solutions Inc.). “The error ‘cannot find module ‘node:util’?’ typically arises when the Node.js environment is unable to locate the specified module. This can occur due to several reasons, including incorrect module paths or using a version of Node.js that does not support the module. It is crucial to ensure that your Node.js version is up to date and that the module is correctly referenced in your code.”
Michael Chen (Lead Developer, Tech Innovations Group). “When encountering the ‘cannot find module ‘node:util’?’ error, developers should first verify the installation of the required Node.js modules. This issue often stems from missing dependencies or an improperly configured package.json file. Running ‘npm install’ can resolve many of these issues by ensuring all dependencies are correctly installed.”
Sarah Patel (Technical Consultant, CodeCraft Consulting). “This error can also indicate that the module in question is not available in the current context. For instance, if you are using a version of Node.js that does not support the ‘node:’ prefix for core modules, you may need to adjust your import statements. Always check the Node.js documentation for the version you are using to understand the available modules and their correct usage.”
Frequently Asked Questions (FAQs)
What does the error “cannot find module ‘node:util'” mean?
This error indicates that the Node.js runtime is unable to locate the specified module, which is typically due to incorrect import statements or an unsupported version of Node.js.
How can I resolve the “cannot find module ‘node:util'” error?
To resolve this error, ensure that you are using a compatible version of Node.js that supports the ‘node:’ prefix for built-in modules, or modify your import statement to use the traditional syntax, such as `require(‘util’)`.
Is the ‘node:util’ module available in all Node.js versions?
No, the ‘node:util’ module is available starting from Node.js version 16.0.0. Ensure that your Node.js version is up to date to utilize this module with the ‘node:’ prefix.
What should I do if I am using an older version of Node.js?
If you are using an older version of Node.js, consider upgrading to a newer version that supports the ‘node:’ prefix. Alternatively, revert to using the standard import syntax without the prefix.
Can this error occur in a specific environment or framework?
Yes, this error can occur in environments or frameworks that do not support the latest Node.js features. It is advisable to check the compatibility of your environment with the Node.js version being used.
Are there any tools to help diagnose this module error?
Yes, tools such as Node.js package managers (npm, yarn) can help diagnose module-related issues. Additionally, using linters or IDEs with built-in error detection can assist in identifying import problems.
The error message “cannot find module ‘node:util'” typically indicates that the Node.js runtime environment is unable to locate the specified module. This issue often arises due to incorrect module import syntax, outdated Node.js versions, or misconfigured project settings. As Node.js evolves, certain modules may be restructured or renamed, leading to potential discrepancies in module resolution.
One of the primary reasons for encountering this error is the use of the newer module resolution syntax introduced in recent Node.js versions. It is essential to ensure that the Node.js version in use supports the ‘node:’ prefix for core modules. Users should verify their Node.js installation and consider upgrading to a more recent version if necessary. Additionally, checking the project’s dependencies and ensuring that all required modules are properly installed can help mitigate this issue.
Another valuable insight is the importance of adhering to best practices when managing Node.js modules. Utilizing package managers like npm or yarn effectively can help maintain a clean and organized project structure. Furthermore, developers should familiarize themselves with the Node.js documentation to understand changes and updates to module management, which can prevent similar errors in the future.
Author Profile
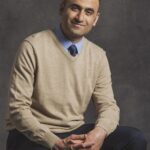
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?