Why Am I Encountering the ‘Error Converting Data Type NVARCHAR to Numeric’ in My Database Queries?
In the realm of data management and database operations, encountering errors is an inevitable part of the journey. One common issue that many developers and database administrators face is the perplexing error message: “error converting data type nvarchar to numeric.” This seemingly innocuous phrase can halt your progress and lead to frustration, especially when you’re deep into data processing or analysis. Understanding the nuances behind this error is crucial for anyone working with databases, as it can impact data integrity and application performance. In this article, we will delve into the causes of this error, explore its implications, and provide insights on how to effectively troubleshoot and resolve it.
When working with databases, data types play a pivotal role in ensuring that information is stored and manipulated correctly. The nvarchar data type, designed for storing variable-length Unicode strings, is often used to accommodate diverse datasets. However, when operations require conversion to numeric formats, mismatches can occur, leading to conversion errors. This situation can arise from a variety of scenarios, such as improper data entry, unexpected characters in string fields, or even misconfigured database schemas.
Understanding the root causes of the “error converting data type nvarchar to numeric” is essential for maintaining the integrity of your data and ensuring smooth operations. By identifying the
Understanding the Error
The error message “error converting data type nvarchar to numeric” typically arises in SQL Server when there is an attempt to convert a string (nvarchar) that cannot be interpreted as a number. This often occurs during data operations such as inserts, updates, or when performing calculations on numeric fields.
Key reasons for this error include:
- Non-numeric Characters: The nvarchar field contains characters that are not digits, such as letters or symbols (e.g., ‘abc’, ‘123abc’).
- Incorrect Formatting: The numeric representation in the nvarchar field may not conform to the expected numeric format (e.g., commas, currency symbols).
- Empty Strings or NULL Values: Attempts to convert empty strings or NULL values into numeric data types can also lead to this error.
Common Scenarios
This error can occur in various scenarios, including but not limited to:
- Insert Statements: When inserting nvarchar data into a numeric column without proper conversion or validation.
- Update Statements: Attempting to update a numeric field with nvarchar data that is improperly formatted.
- Arithmetic Operations: Performing calculations that involve nvarchar columns without ensuring they contain valid numeric data.
Preventive Measures
To prevent this error, consider the following practices:
- Data Validation: Always validate data before attempting conversion. Use conditional statements to check for numeric values.
- Try-Catch Blocks: Implement error handling in your SQL queries using TRY…CATCH to gracefully manage conversion errors.
- Data Cleansing: Before inserting or updating data, cleanse your nvarchar fields to remove non-numeric characters.
Example of Handling the Error
Here is an example illustrating how to handle the error using a TRY…CATCH block:
“`sql
BEGIN TRY
INSERT INTO YourTable (NumericColumn)
SELECT CAST(NvarcharColumn AS NUMERIC)
FROM SourceTable
WHERE ISNUMERIC(NvarcharColumn) = 1;
END TRY
BEGIN CATCH
PRINT ‘An error occurred: ‘ + ERROR_MESSAGE();
END CATCH;
“`
In this example, the query attempts to insert values only if they are numeric, thus reducing the likelihood of encountering the conversion error.
Data Type Conversion Table
The following table outlines common SQL Server data types and their conversion compatibility:
Nvarchar | Numeric | Conversion Method |
---|---|---|
Valid Numeric String | Int, Decimal, Float | CAST() or CONVERT() |
Non-Numeric String | Int, Decimal, Float | Error |
Empty String | Int, Decimal, Float | Error |
Numeric with Commas | Int, Decimal, Float | Error unless cleaned |
Understanding the intricacies of data type conversion is essential for maintaining data integrity and ensuring smooth database operations. By implementing proper validation and error handling, you can mitigate the risks associated with converting nvarchar to numeric data types.
Understanding the Error
The error message “error converting data type nvarchar to numeric” typically occurs in SQL Server when there is an attempt to convert a string (nvarchar) that cannot be interpreted as a numeric value. This can happen in various scenarios, such as during data insertion, updates, or when performing calculations.
Common causes of this error include:
- Non-numeric characters: The string contains letters, symbols, or spaces that are not valid in a numeric context.
- Improper formatting: The numeric values might be formatted in a way that SQL Server does not recognize, such as using commas as thousand separators.
- NULL or empty strings: Attempting to convert NULL values or empty strings can lead to this error if the database expects a numeric value.
Troubleshooting Steps
To resolve the error, follow these troubleshooting steps:
- Identify the problematic data: Use queries to isolate the rows that cause the error.
- Check for non-numeric characters: Utilize string functions to find non-numeric characters in your data.
- Validate data types: Ensure that the data types in your table schema match the data being inserted or updated.
- Convert or cast data appropriately: Use SQL functions to convert data safely.
Here’s an example of how to check for non-numeric characters:
“`sql
SELECT *
FROM YourTable
WHERE TRY_CAST(YourColumn AS NUMERIC) IS NULL AND YourColumn IS NOT NULL;
“`
Preventing the Error
To prevent future occurrences of the error, consider implementing the following strategies:
- Data validation: Enforce validation rules on input data to ensure it adheres to expected formats.
- Use TRY_CAST or TRY_CONVERT: These functions return NULL instead of an error when conversion fails, allowing for safer data handling.
“`sql
SELECT TRY_CAST(YourColumn AS NUMERIC) AS NumericValue
FROM YourTable;
“`
- Regular expressions: Use regex in application code to validate numeric input before it reaches the database.
- Schema design: Ensure appropriate data types are used in your table structures to minimize conversion issues.
Example Scenarios
Here are a few scenarios that can lead to this error, along with possible resolutions:
Scenario | Error Cause | Resolution |
---|---|---|
Inserting user input directly | Non-numeric characters in input | Validate and sanitize input |
Aggregating data | NULL or invalid formatted strings | Use TRY_CAST to filter invalid data |
Importing data from external sources | Improperly formatted numeric values | Preprocess data to correct formats |
Performing calculations | Mixed data types in calculations | Ensure all operands are of numeric types |
Best Practices
Adopt the following best practices to minimize the risk of encountering this error:
- Consistent data entry: Ensure that users enter data in a consistent format.
- Error logging: Implement error logging to capture details of failed conversions for future analysis.
- Regular audits: Periodically review data in the database for compliance with expected formats and types.
- Training: Educate users on the importance of data integrity and the impact of incorrect data types.
By following these guidelines, you can significantly reduce the likelihood of encountering the “error converting data type nvarchar to numeric” and ensure smoother database operations.
Understanding the Challenges of Data Type Conversion in SQL
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “The error of converting data type nvarchar to numeric often arises from the presence of non-numeric characters in the nvarchar field. It is crucial to validate and clean the data before attempting conversion to prevent such errors.”
Michael Chen (Database Administrator, Reliable Systems Corp.). “In my experience, this error frequently occurs when the database contains unexpected nulls or empty strings. Implementing rigorous data validation rules can significantly reduce the incidence of this error.”
Lisa Patel (SQL Developer, Data Solutions Group). “To effectively handle the nvarchar to numeric conversion error, I recommend using TRY_CONVERT or TRY_CAST functions in SQL Server. These functions can gracefully handle conversion failures by returning NULL instead of throwing an error.”
Frequently Asked Questions (FAQs)
What does the error “error converting data type nvarchar to numeric” mean?
This error indicates that there is an attempt to convert a string (nvarchar) value into a numeric type, but the string contains non-numeric characters or is formatted incorrectly.
What are common causes of this error in SQL Server?
Common causes include trying to convert strings that contain letters, special characters, or empty values into numeric types, as well as mismatched data types in expressions or calculations.
How can I identify which value is causing the error?
You can identify the problematic value by using the `TRY_CAST` or `TRY_CONVERT` functions, which return NULL for non-convertible values, allowing you to isolate and examine those entries.
What steps can I take to resolve this error?
To resolve this error, ensure that all nvarchar values intended for conversion to numeric types are clean, containing only valid numeric characters, and handle any potential NULL or empty values appropriately.
Can I prevent this error from occurring in the future?
Yes, you can prevent this error by validating and sanitizing input data before performing conversions, using constraints to enforce data integrity, and implementing error handling in your SQL queries.
Is this error specific to SQL Server or can it occur in other databases?
While the phrasing of the error may vary, similar conversion errors can occur in other database systems when attempting to convert non-numeric strings to numeric types. Each database has its own methods for handling such conversions.
The error message “error converting data type nvarchar to numeric” typically occurs in database operations when there is an attempt to convert a string (nvarchar) value into a numeric type. This issue often arises in SQL Server or other relational database management systems when the data being processed contains non-numeric characters or is improperly formatted. Identifying the root cause of this error is crucial for ensuring data integrity and successful query execution.
One of the primary reasons for this error is the presence of invalid characters in the nvarchar data, such as letters, special symbols, or whitespace. It is essential to validate and sanitize the data before performing conversions. Additionally, ensuring that the data types in the database schema align with the expected input types can prevent such errors. Utilizing functions like TRY_CAST or TRY_CONVERT can also help manage conversion attempts more gracefully by returning NULL for failed conversions instead of generating an error.
To resolve this issue effectively, developers and database administrators should implement thorough data validation processes and consider using error handling strategies in their SQL queries. Regularly reviewing and cleaning the data can help maintain its integrity and prevent similar errors in the future. By understanding the underlying causes and implementing best practices, organizations can minimize the occurrence of the “error converting data type nv
Author Profile
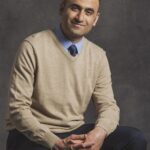
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?