How Can I Resolve the ‘Error Converting Data Type Varchar to Bigint’ Issue?
In the world of database management and programming, encountering errors is an inevitable part of the journey. One common yet perplexing issue that many developers face is the “error converting data type varchar to bigint.” This error can halt your progress and leave you scratching your head, especially when it appears unexpectedly during data manipulation or querying. Understanding the nuances behind this error is crucial for anyone working with SQL databases, as it can significantly impact the integrity and performance of your applications. In this article, we will delve into the root causes of this error, explore its implications, and provide practical solutions to overcome it.
Overview
At its core, the “error converting data type varchar to bigint” occurs when a database engine attempts to convert a string (varchar) representation of a number into a larger integer type (bigint) but fails to do so due to incompatible data formats. This issue often arises in scenarios where data types are not explicitly defined or when unexpected characters are present in the data. Developers may encounter this error during data imports, transformations, or when executing queries that involve type conversions.
Understanding the context in which this error arises is essential for effective troubleshooting. Factors such as data integrity, schema design, and input validation play a significant role in preventing such errors. By examining
Common Causes of the Error
The error “converting data type varchar to bigint” typically arises in SQL databases when there is an attempt to convert a string (varchar) that cannot be appropriately interpreted as a numeric value (bigint). This issue can originate from various sources, including:
- Invalid Data Format: The varchar string may contain non-numeric characters, such as letters or symbols.
- Leading or Trailing Spaces: Extra spaces in the string can prevent successful conversion.
- Empty Strings: Attempting to convert an empty string will also result in an error.
- Inconsistent Data Types: Mixing data types in operations, such as attempting arithmetic on varchar and bigint types, can lead to conversion errors.
Solutions to Resolve the Error
To fix the “error converting data type varchar to bigint,” several strategies can be employed:
- Data Cleaning: Ensure that the varchar data consists solely of valid numeric characters. This might involve:
- Removing non-numeric characters
- Trimming spaces from the strings
- Replacing empty strings with valid numeric values (e.g., `0`)
- Using TRY_CAST or TRY_CONVERT: These functions return `NULL` instead of raising an error when conversion fails. For example:
“`sql
SELECT TRY_CAST(your_column AS BIGINT) FROM your_table;
“`
- Conditional Checks: Implement checks to filter out non-convertible values before attempting to convert. This can be done using a WHERE clause:
“`sql
SELECT your_column
FROM your_table
WHERE ISNUMERIC(your_column) = 1;
“`
Example of Error Handling
Consider a scenario where you are attempting to aggregate data from a table containing both varchar and bigint types. A common method to handle potential conversion errors is by using a query that first verifies the data type.
Here’s a sample SQL query that demonstrates this approach:
“`sql
SELECT SUM(TRY_CAST(your_column AS BIGINT)) AS Total
FROM your_table
WHERE your_column IS NOT NULL AND your_column <> ” AND ISNUMERIC(your_column) = 1;
“`
This query ensures that only valid numeric entries are summed, thereby preventing the conversion error.
Data Type Conversion Table
The following table outlines the common SQL data types and their characteristics, which can help in understanding potential conversion issues.
Data Type | Description | Typical Size |
---|---|---|
VARCHAR(n) | Variable-length string | n bytes (up to 8000) |
BIGINT | Integer data type | 8 bytes |
INT | Standard integer data type | 4 bytes |
DECIMAL(p,s) | Fixed-point number with precision p and scale s | Varies |
Understanding the differences between these data types can aid in diagnosing and resolving conversion issues effectively.
Error Overview
The error “converting data type varchar to bigint” typically occurs in SQL Server when an attempt is made to convert a string (varchar) to a numeric type (bigint) but the string contains non-numeric characters or is improperly formatted. This error can lead to failed queries and data integrity issues.
Common Causes
Several factors can trigger this error, including:
- Non-numeric Characters: The varchar field contains letters, special characters, or spaces.
- Empty Strings: An empty string is encountered during the conversion.
- Improper Formatting: The string representation of the number may contain commas or other formatting characters not recognized by bigint.
- Data Type Mismatch: The column or variable being converted does not match the expected data type.
Identifying the Issue
To identify the exact cause of the error, consider the following approaches:
- Query Inspection: Review the SQL query for potential type mismatches.
- Data Validation: Use the `TRY_CAST` or `TRY_CONVERT` functions to check for convertible values:
“`sql
SELECT column_name
FROM table_name
WHERE TRY_CONVERT(bigint, column_name) IS NULL;
“`
- Data Profiling: Examine the data within the varchar column to identify any problematic values.
Resolving the Error
To resolve the conversion error, implement one or more of the following strategies:
- Data Cleaning: Ensure the varchar data contains only numeric characters. This may include:
- Removing non-numeric characters
- Trimming whitespace
- Replacing commas or currency symbols
- Using TRY_CONVERT: Utilize the `TRY_CONVERT` function to safely attempt conversion and handle errors gracefully:
“`sql
SELECT TRY_CONVERT(bigint, column_name) AS ConvertedValue
FROM table_name;
“`
- Filtering Invalid Data: Exclude non-convertible records by applying a WHERE clause:
“`sql
SELECT column_name
FROM table_name
WHERE column_name NOT LIKE ‘%[^0-9]%’;
“`
- Changing Data Types: If applicable, change the data type of the column in the database schema to better accommodate the data:
“`sql
ALTER TABLE table_name
ALTER COLUMN column_name BIGINT;
“`
Best Practices
To prevent encountering this error in the future, consider the following best practices:
- Data Validation: Implement validation rules at the application level to ensure only appropriate values are entered.
- Consistent Data Types: Maintain consistent data types across your database schema to avoid conversion issues.
- Regular Audits: Conduct regular audits of your data to identify and rectify any anomalies.
- Error Handling: Incorporate robust error handling in your SQL scripts to manage conversion errors efficiently.
Example Scenario
Consider a scenario where you have a table `EmployeeData` with a varchar column `EmployeeID` that occasionally contains non-numeric characters. To address the conversion error, you could execute the following SQL:
“`sql
SELECT EmployeeID
FROM EmployeeData
WHERE TRY_CONVERT(bigint, EmployeeID) IS NULL;
“`
This query will return all records that cannot be converted, allowing you to investigate and correct the underlying data issues effectively.
Understanding the Challenges of Data Type Conversion in Databases
Dr. Emily Carter (Database Architect, Tech Solutions Inc.). “The error ‘converting data type varchar to bigint’ typically arises when the database engine encounters non-numeric characters in a varchar field that it attempts to convert to a bigint. It is crucial to validate and cleanse your data before performing such conversions to avoid runtime exceptions.”
James Liu (Data Analyst, Insight Analytics Group). “In many cases, this error can be mitigated by using explicit conversion functions and error handling in SQL queries. Implementing a try-catch block can help manage unexpected data types and ensure that the application remains robust.”
Sarah Thompson (Senior Software Engineer, CloudTech Solutions). “When dealing with legacy systems, it is common to encounter this error due to inconsistent data entry practices. Regular audits and data normalization processes should be established to maintain data integrity and prevent such conversion errors.”
Frequently Asked Questions (FAQs)
What does the error “converting data type varchar to bigint” mean?
This error occurs when there is an attempt to convert a string (varchar) that cannot be interpreted as a numeric value (bigint) in a database operation, such as a query or data insertion.
What are common causes of this error?
Common causes include non-numeric characters in the varchar field, empty strings, or values that exceed the range of the bigint data type, which can lead to conversion failures.
How can I troubleshoot this error?
To troubleshoot, check the data in the varchar column for any non-numeric characters or invalid formats. Use functions like TRY_CAST or TRY_CONVERT to safely attempt conversions without throwing errors.
What is the difference between varchar and bigint data types?
Varchar is a variable-length string data type used for storing text, while bigint is a numeric data type that stores large integers. Each serves different purposes in data storage and manipulation.
Can I prevent this error from occurring in the future?
Yes, implementing data validation rules before inserting or updating records can help prevent this error. Additionally, using appropriate data types in your database schema can reduce the likelihood of conversion issues.
What should I do if I encounter this error in a stored procedure?
Review the stored procedure for any data type mismatches. Ensure that the parameters and variables are defined with compatible data types and consider adding error handling to manage conversion exceptions gracefully.
The error message “error converting data type varchar to bigint” typically arises in database systems, particularly when dealing with SQL queries. This error indicates that the system is attempting to convert a string (varchar) into a numeric type (bigint), but the string does not contain a valid numeric representation. This can occur during operations such as data insertion, updates, or when performing calculations that involve type conversion.
Common scenarios that lead to this error include attempting to perform mathematical operations on varchar fields that contain non-numeric characters, or when a varchar field is expected to hold numeric data but contains invalid entries. To resolve this issue, it is essential to ensure that the data being processed is in the correct format. This may involve validating input data, cleaning up existing data, or using conversion functions that handle errors gracefully.
Key takeaways from addressing this error include the importance of data validation and type consistency within databases. Implementing strict data entry protocols and using appropriate data types can significantly reduce the occurrence of such errors. Additionally, employing error handling mechanisms within SQL queries can provide more informative feedback and facilitate troubleshooting when issues arise.
Author Profile
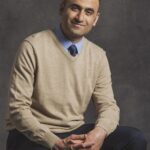
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?