How to Resolve the ‘Error: NG0205: Injector Has Already Been Destroyed’ in Your Angular Application?
In the ever-evolving world of web development, encountering errors is an inevitable part of the journey. Among the myriad of issues developers face, the `error: ng0205: injector has already been destroyed` stands out as a particularly perplexing challenge for those working with Angular applications. This error not only disrupts the flow of development but can also leave developers scratching their heads in confusion. Understanding the root causes and implications of this error is crucial for maintaining robust and efficient applications. In this article, we will delve into the intricacies of this error, exploring its origins, potential pitfalls, and best practices to prevent it from derailing your project.
As Angular continues to gain traction for its powerful features and flexibility, developers must navigate its complex dependency injection system. The `ng0205` error serves as a reminder of the delicate balance required when managing components and services within this framework. When an injector is destroyed prematurely, it can lead to a cascade of issues that not only affect functionality but also hinder the user experience. By unpacking the circumstances that lead to this error, developers can gain valuable insights into the lifecycle of Angular applications and the importance of proper resource management.
In the following sections, we will explore common scenarios that trigger the `ng0205` error
Understanding the Error
The error message `error: ng0205: injector has already been destroyed` typically occurs in Angular applications when there are attempts to access services or components after the Angular dependency injection system has already disposed of them. This can happen for various reasons, often related to the lifecycle of components or the management of services.
Common Causes
- Component Lifecycle: The error can arise when a component tries to utilize a service after it has been destroyed. This can happen if you have subscriptions or asynchronous operations that are not properly cleaned up in the `ngOnDestroy` lifecycle hook.
- Module Unloading: If a module is unloaded (for instance, through lazy loading or navigating away), any services tied to that module may get destroyed. Attempting to access them afterward can lead to this error.
- Improper Dependency Management: If a service is provided at a component level and that component is destroyed, the service instance is also destroyed, leading to potential errors if other components or services attempt to access it afterward.
Best Practices to Avoid the Error
To prevent the `ng0205` error, consider the following best practices:
- Unsubscribe from Observables: Always unsubscribe from any observable subscriptions in the `ngOnDestroy` method to prevent memory leaks and unexpected behavior.
- Utilize `takeUntil`: Use the `takeUntil` operator in conjunction with a subject to manage the lifecycle of subscriptions effectively.
- Check for Service Availability: Before accessing a service, check if it is available and not destroyed to avoid attempting to access a non-existent instance.
- Proper Use of Services: Ensure that services are provided at the correct level (either root or a specific component) to maintain their lifecycle as needed.
Example Implementation
Here is a simple example demonstrating how to manage subscriptions to avoid the error:
“`typescript
import { Component, OnDestroy } from ‘@angular/core’;
import { Subject } from ‘rxjs’;
import { takeUntil } from ‘rxjs/operators’;
@Component({
selector: ‘app-example’,
templateUrl: ‘./example.component.html’,
})
export class ExampleComponent implements OnDestroy {
private destroy$ = new Subject
constructor(private myService: MyService) {
this.myService.getData()
.pipe(takeUntil(this.destroy$))
.subscribe(data => {
// Handle data
});
}
ngOnDestroy() {
this.destroy$.next();
this.destroy$.complete();
}
}
“`
Summary of Best Practices
Practice | Description |
---|---|
Unsubscribe from Observables | Always unsubscribe in `ngOnDestroy` to avoid memory leaks. |
Use `takeUntil` | Utilize `takeUntil` with a subject for managing subscriptions effectively. |
Service Availability Checks | Ensure the service is available before accessing it. |
Service Provisioning | Provide services at appropriate levels to manage lifecycle correctly. |
By adhering to these practices, developers can significantly reduce the likelihood of encountering the `ng0205` error and enhance the stability and reliability of their Angular applications.
Understanding the Error Message
The error message `error: ng0205: injector has already been destroyed.` typically arises in Angular applications when a component or service attempts to access the dependency injector after it has been destroyed. This situation often indicates a lifecycle management issue within the application.
Common causes of this error include:
- Improper Component Lifecycle Handling: When a component is destroyed but still tries to execute logic that involves dependency injection.
- Observables Not Unsubscribed: If an observable is still active when the component is destroyed, it may attempt to perform actions that require the injector.
- Delayed Operations: Promises or asynchronous tasks that complete after the component is destroyed can lead to this error if they reference the injector.
Debugging the Error
To effectively debug the `ng0205` error, consider the following steps:
- Check Component Lifecycle Hooks:
- Ensure that any logic dependent on the injector is placed in the appropriate lifecycle hooks, such as `ngOnInit` and `ngOnDestroy`.
- Unsubscribe from Observables:
- Always unsubscribe from any subscriptions in the `ngOnDestroy` lifecycle hook to prevent lingering references. Use operators such as `takeUntil` to manage subscriptions efficiently.
- Review Asynchronous Calls:
- For asynchronous operations, ensure that the component is still active before executing any logic that relies on the injector. Utilize flags to check the component’s status.
- Use Angular’s Dependency Injection Properly:
- Ensure services are being injected correctly and that their lifecycle aligns with the components using them.
Example Code Fixes
Here are some practical code snippets to illustrate how to resolve this error:
Proper Subscription Management:
“`typescript
import { Subject } from ‘rxjs’;
export class MyComponent implements OnInit, OnDestroy {
private destroy$ = new Subject
constructor(private myService: MyService) {}
ngOnInit() {
this.myService.getData()
.pipe(takeUntil(this.destroy$))
.subscribe(data => {
// Handle data
});
}
ngOnDestroy() {
this.destroy$.next();
this.destroy$.complete();
}
}
“`
**Handling Promises Safely**:
“`typescript
export class AnotherComponent implements OnInit, OnDestroy {
private isAlive = true;
ngOnInit() {
this.myService.fetchData().then(data => {
if (this.isAlive) {
// Use data
}
});
}
ngOnDestroy() {
this.isAlive = ;
}
}
“`
Best Practices to Avoid the Error
To reduce the likelihood of encountering the `ng0205` error, adhere to these best practices:
- Use Angular’s Lifecycle Hooks: Familiarize yourself with Angular’s lifecycle hooks to manage resource allocation and cleanup effectively.
- Leverage `async` Pipes: Utilize `async` pipes in templates to manage observable subscriptions automatically.
- Service Scope Management: Be mindful of the scope of services. Avoid using singleton services unnecessarily within components that may be destroyed.
- Monitor Component States: Implement checks to ascertain the state of the component before performing operations that require the injector.
By following these strategies, you can effectively mitigate the occurrence of the `ng0205` error in Angular applications and ensure robust component lifecycle management.
Understanding the `ng0205: injector has already been destroyed` Error
Dr. Emily Carter (Senior Software Engineer, Angular Development Team). “The `ng0205` error typically arises when an Angular component or service attempts to access an injector after it has been destroyed. This often occurs in scenarios involving asynchronous operations or subscriptions that outlive their parent components. Properly managing the lifecycle of components and ensuring that all subscriptions are cleaned up can help mitigate this issue.”
Michael Chen (Lead Architect, Frontend Solutions Inc.). “In my experience, the `ng0205` error can be particularly frustrating during unit testing. It is essential to ensure that all dependencies are correctly mocked and that the component’s lifecycle is respected. Failing to do so can lead to attempts to access destroyed injectors, which results in this error. Implementing robust testing strategies can significantly reduce the occurrence of such issues.”
Sarah Patel (Angular Consultant, Tech Innovations Group). “Addressing the `ng0205` error requires a thorough understanding of Angular’s dependency injection system. Developers should be vigilant about the timing of service instantiation and destruction. Utilizing the `ngOnDestroy` lifecycle hook effectively to unsubscribe from observables and detach event listeners can prevent this error from surfacing in production environments.”
Frequently Asked Questions (FAQs)
What does the error “ng0205: injector has already been destroyed” indicate?
This error indicates that the Angular dependency injector has been destroyed, but an attempt is being made to access or inject a service or component that relies on it.
What are common causes of the “ng0205” error?
Common causes include navigating away from a component while asynchronous operations are still pending, or improperly managing the lifecycle of services and components, especially when using Observables or Promises.
How can I resolve the “ng0205: injector has already been destroyed” error?
To resolve this error, ensure that you unsubscribe from Observables and cancel Promises when a component is destroyed. Additionally, check the component lifecycle to avoid accessing services after the injector has been destroyed.
Can this error occur in lazy-loaded modules?
Yes, this error can occur in lazy-loaded modules if the injector for those modules is destroyed before all asynchronous operations complete. Proper lifecycle management is essential in these scenarios.
Is there a way to prevent the “ng0205” error from occurring?
Preventing this error involves implementing proper cleanup logic in the `ngOnDestroy` lifecycle hook. Always ensure that subscriptions are managed and that services are not accessed post-destruction of the component.
What debugging steps can I take when encountering this error?
Debugging steps include reviewing the component lifecycle, checking for active subscriptions, and ensuring that no services are being accessed after the component is destroyed. Use Angular’s debugging tools to trace the lifecycle events for better insights.
The error message “ng0205: injector has already been destroyed” typically arises in Angular applications when there is an attempt to access a dependency injection system after it has been disposed of. This situation often occurs due to improper lifecycle management of components or services, particularly when asynchronous operations are involved. Developers may encounter this error when navigating away from a component while still awaiting a response from an observable or promise that relies on the destroyed injector.
To mitigate this issue, it is crucial to ensure that all subscriptions to observables are properly managed and unsubscribed when a component is destroyed. Utilizing Angular’s lifecycle hooks, such as `ngOnDestroy`, allows developers to clean up resources effectively. Additionally, employing strategies like the `takeUntil` operator can help manage subscriptions and prevent memory leaks, thereby reducing the likelihood of encountering the ng0205 error.
Another important consideration is to review the overall architecture of the application to ensure that services are not being accessed after their respective components have been destroyed. This can involve implementing guards or checks that validate the state of the component before proceeding with operations that require the injector. By adhering to best practices in component and service management, developers can enhance the stability of their Angular applications and minimize the occurrence of such errors.
Author Profile
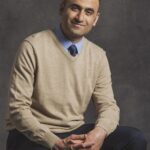
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?