Why Am I Seeing the Error: ‘React Context is Unavailable in Server Components’?
In the rapidly evolving landscape of web development, React has emerged as a powerful library for building user interfaces, particularly with the of server components. However, as developers dive into this innovative approach, they often encounter a perplexing issue: the error message that states, “React context is unavailable in server components.” This seemingly cryptic warning can lead to confusion and frustration, especially for those who rely heavily on React’s context API to manage state across their applications. Understanding the nuances of this error is crucial for developers aiming to harness the full potential of server components while maintaining a seamless user experience.
As we explore the intricacies of this error, we’ll uncover the underlying reasons why React context behaves differently in server components compared to traditional client-side rendering. The context API, designed to facilitate state management and prop drilling, encounters limitations when it comes to the server-rendered environment. This discrepancy can lead to unexpected behaviors and challenges that developers must navigate to ensure their applications run smoothly.
By delving into the core principles of React’s architecture and the role of server components, we will shed light on the best practices for managing state in this new paradigm. Whether you’re a seasoned React developer or just starting your journey, understanding how to effectively handle context in server components will empower you to
Error: React Context is Unavailable in Server Components
React Context is a powerful feature that allows developers to share values between components without having to explicitly pass props down through every level of the component tree. However, when working with React Server Components, developers may encounter the error stating that “React context is unavailable in server components.” This limitation arises from the fundamental differences between server and client components in React.
Server Components are designed to render on the server and send HTML to the client, improving performance and reducing the amount of JavaScript sent over the network. They can fetch data directly from the server but do not support certain client-side features, including React Context, which relies on the client-side lifecycle.
The main reasons for the unavailability of React Context in Server Components include:
- Isolation of Execution: Server Components execute in a different environment than Client Components. The context API is intended for managing state and behavior that is inherently tied to client-side interactivity.
- Data Fetching Patterns: Server Components are optimized for data fetching on the server, meaning they should not depend on context that may change during client-side rendering.
- Lifecycle Methods: Server Components do not have access to lifecycle methods that are essential for context updates, further complicating their interaction with context.
Alternatives to React Context in Server Components
Given these constraints, developers need to consider alternatives for managing state and sharing data in Server Components. Some viable options include:
- Props Drilling: Pass data directly through component props. While this can lead to verbose code, it is a straightforward method to ensure data is available where needed.
- Custom Hooks: Create custom hooks that encapsulate logic and state management, which can be called in both Server and Client Components.
- Global State Management Libraries: Utilize libraries like Redux or Zustand that can be configured to work with both Server and Client Components. These libraries provide a more structured approach to global state management.
Understanding Component Structure
To better illustrate the differences between Server Components and Client Components, consider the following table:
Feature | Server Components | Client Components |
---|---|---|
Rendering Environment | Executed on the server | Executed in the browser |
Access to React Context | No | Yes |
Data Fetching | Direct from the server | Client-side fetching |
Usage of Lifecycle Methods | Limited | Full access |
Understanding the limitations of Server Components with respect to React Context can help developers architect their applications more effectively. By leveraging alternative strategies for state management, developers can still achieve a high level of performance while maintaining the necessary interactivity within their applications.
Error: React Context is Unavailable in Server Components
The error message indicating that “React context is unavailable in server components” arises from the architectural differences between React’s client and server components. Understanding these distinctions is crucial for effectively utilizing context in your applications.
Understanding Server Components
Server components in React are designed to be rendered on the server, allowing developers to fetch data and render HTML before sending it to the client. This approach improves performance and reduces the amount of JavaScript that needs to be sent to the client. Key aspects include:
- Static Rendering: Server components are rendered to static HTML, which can improve loading times.
- Data Fetching: They can directly fetch data from databases or APIs, reducing the need for client-side fetching.
- No Client-side Interactivity: Server components do not maintain state or respond to user interactions, which limits their ability to utilize React’s context API.
Why Context is Unavailable
React’s context API is primarily designed for managing state and passing data through the component tree in client-side applications. The unavailability of context in server components can be attributed to:
- Lifecycle Differences: Server components do not have the same lifecycle as client components, which means they cannot subscribe to context updates or provide context values effectively.
- Separation of Concerns: Context is intended for client-side state management. Since server components do not handle client-side interactions, providing context in this way is unnecessary and could lead to confusion.
Workarounds for Using Context with Server Components
While context cannot be directly used in server components, there are alternative strategies to achieve similar functionality:
- Lift State Up: Move state management to a parent client component where context can be provided, allowing server components to receive props instead.
- Use Props: Instead of using context, pass the required data as props from a client component to server components. This maintains the data flow without the need for context.
- Combine Client and Server Components: Structure your application by combining client components with server components to utilize context where it is applicable.
Example Implementation
Below is an example of how to manage state and props between components:
“`javascript
// Parent Component (Client)
const ParentComponent = () => {
const [value, setValue] = useState(“Hello World”);
return (
);
};
// Server Component
const ServerComponent = () => {
return
;
};
// Client Component
const ClientComponent = () => {
const { value } = useContext(Context);
return
;
};
“`
In this example, the `ParentComponent` provides context to `ClientComponent`, while `ServerComponent` simply renders without context access. This pattern allows for effective data management while adhering to React’s architectural principles.
Understanding the Limitations of React Context in Server Components
Dr. Emily Chen (Senior Software Engineer, React Development Team). “The limitation of React context in server components stems from the fundamental architecture of server-side rendering. Context relies on the React component tree, which is not fully established in server components, leading to the error message regarding its unavailability.”
Mark Thompson (Lead Frontend Architect, Innovative Web Solutions). “When working with server components, developers must be aware that context providers cannot be utilized as they would in client components. This necessitates alternative state management strategies that are compatible with server-rendered environments.”
Lisa Patel (React Consultant, Web Performance Experts). “The error indicating that React context is unavailable in server components highlights a critical aspect of React’s design. It is essential for developers to adjust their approach when integrating context APIs, especially in hybrid applications that leverage both server and client components.”
Frequently Asked Questions (FAQs)
What does the error “react context is unavailable in server components” mean?
This error indicates that you are attempting to use React Context in a server component where it is not supported. Server components are designed to render on the server without access to the React Context API.
Why can’t I use React Context in server components?
React Context is primarily intended for client-side state management. Server components render on the server and do not have access to the client-side React lifecycle, which is necessary for context to function properly.
How can I resolve the “react context is unavailable in server components” error?
To resolve this error, ensure that you are using React Context only within client components. If you need to share data between server and client components, consider passing props directly or using a different state management solution.
Can I use React Context in a hybrid component?
Yes, you can use React Context in hybrid components, but only within the client-side part of the component. Ensure that any context-related logic is encapsulated within the client portion to avoid this error.
Are there alternatives to React Context for state management in server components?
Yes, alternatives include using props to pass data down from server components to client components or utilizing libraries like Redux or Zustand that can manage state across both server and client components effectively.
Is this error specific to certain versions of React?
The error is primarily associated with React 18 and later versions, where the distinction between server and client components was introduced. Ensure you are following the guidelines specific to the version you are using.
The error message “react context is unavailable in server components” typically arises in React applications that utilize server components. This issue highlights the limitations of using React Context API within server-rendered components, as the context is designed primarily for client-side rendering. Understanding the distinction between server and client components is crucial for developers working with React’s latest features, particularly in frameworks like Next.js.
One of the key takeaways is the importance of correctly managing state and context in a React application that employs server components. Developers should consider alternative strategies for state management that do not rely on context when working in a server environment. This may involve using props to pass data down to components or leveraging other state management libraries that are compatible with server-side rendering.
Additionally, it is essential to stay informed about updates and best practices in React development. As the framework evolves, new features and methodologies may emerge that could address current limitations, including the handling of context in server components. Keeping abreast of the React documentation and community discussions can provide valuable insights and solutions to common challenges.
Author Profile
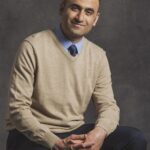
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?