Why Am I Seeing the Error: ‘Reference to Non-Static Member Function Must Be Called’?
In the world of C++ programming, encountering errors is an inevitable part of the development process. One such error that can leave both novice and seasoned programmers scratching their heads is the message: “error: reference to non-static member function must be called.” This seemingly cryptic notification often arises in the context of object-oriented programming, where the distinction between static and non-static members plays a crucial role. Understanding this error not only helps in debugging but also deepens your grasp of C++’s object-oriented principles.
At its core, this error highlights a fundamental aspect of how member functions are invoked within classes. Non-static member functions are tied to specific instances of a class, meaning they require an object to be called upon. In contrast, static member functions belong to the class itself and do not need an instance. This distinction is vital for effective coding, as misusing these concepts can lead to confusion and frustrating compilation errors.
As we delve deeper into the intricacies of this error, we will explore the underlying principles that govern member function calls, the scenarios in which this error typically arises, and practical strategies to resolve it. By the end of this article, you’ll not only have a clearer understanding of this specific error message but also enhance your overall proficiency in C++
Understanding the Error
The error message “reference to non-static member function must be called” typically occurs in C++ when attempting to use a non-static member function without an instance of the class. Non-static member functions require an object of the class to be invoked, as they can access instance-specific data.
Non-static member functions are associated with an object and can manipulate instance variables. In contrast, static member functions belong to the class itself and do not require an object for invocation. This distinction is crucial in understanding how to correctly call functions in C++.
Common Causes of the Error
Several scenarios can lead to this error, including:
- Calling a Non-Static Function from a Static Context: Attempting to call a non-static member function from a static method or outside any class instance.
- Using the Class Name Instead of an Instance: Trying to call a non-static function using the class name rather than a specific object.
- Improper Pointer or Reference Usage: Dereferencing a pointer to a class or using a reference incorrectly can also result in this error.
Examples of the Error
To illustrate the error and its resolution, consider the following example:
“`cpp
class MyClass {
public:
void display() {
std::cout << "Hello from MyClass!" << std::endl;
}
static void staticDisplay() {
// This line will cause the error
// display(); // Error
}
};
int main() {
MyClass obj;
obj.display(); // Correct usage
MyClass::staticDisplay(); // Correct usage for static function
return 0;
}
```
In the above example, if `display();` is called within `staticDisplay()`, it will trigger the error because `display` is a non-static member function.
How to Resolve the Error
To fix the error, the following approaches can be employed:
- Instantiate the Class: Ensure that the non-static member function is called on an instance of the class.
“`cpp
MyClass obj;
obj.display(); // Correct
“`
- Use `this` Pointer: Inside non-static member functions, use the `this` pointer to refer to the current object.
- Avoid Static Context for Non-Static Calls: Ensure non-static functions are not called from static methods or outside of a class context.
Best Practices
To prevent such errors in your code, consider the following best practices:
- Always maintain clear distinctions between static and non-static member functions.
- Use descriptive names for your methods to avoid confusion regarding their static or non-static nature.
- Use IDE features or compiler warnings to catch these errors early in the development process.
Issue | Resolution |
---|---|
Calling non-static method from static context | Instantiate the class or use a non-static context. |
Incorrect pointer/reference usage | Ensure proper dereferencing and object access. |
Using class name to call non-static method | Use an object instance to call the method. |
Understanding the Error
The error message “reference to non-static member function must be called” typically occurs in C++ when there is an attempt to call a non-static member function without an associated object. This error arises due to the nature of how non-static member functions are designed to operate, requiring an instance of the class to be invoked.
Common Scenarios Leading to the Error
Several common coding patterns can lead to this error:
– **Direct Function Call**: Attempting to call a non-static member function using the class name rather than an object instance.
“`cpp
class MyClass {
public:
void myFunction() {}
};
MyClass::myFunction(); // Error: non-static member function must be called on an object
“`
– **Using Pointers**: Incorrectly using pointers to call non-static member functions without dereferencing the pointer.
“`cpp
MyClass* obj = new MyClass();
obj->myFunction(); // Correct way to call
MyClass::myFunction(); // Error
“`
- Static Context: Attempting to call a non-static function from a static member function or a static context.
“`cpp
class MyClass {
public:
void myFunction() {}
static void staticFunction() {
myFunction(); // Error: cannot call non-static function
}
};
“`
Resolution Strategies
To resolve this error, ensure that non-static member functions are called on an instance of the class. Here are key strategies:
– **Instantiate the Class**: Always create an object of the class before calling its non-static member functions.
“`cpp
MyClass obj;
obj.myFunction(); // Correct usage
“`
– **Use Pointers Properly**: If using pointers, make sure to dereference the pointer or use the arrow operator.
“`cpp
MyClass* objPtr = new MyClass();
objPtr->myFunction(); // Correct usage
“`
- Avoid Static Context for Non-static Calls: Ensure that non-static member functions are not called in static contexts unless an object is available.
Example Code for Clarity
Consider the following example to illustrate the correct usage of non-static member functions:
“`cpp
include
class Example {
public:
void display() {
std::cout << "Hello, World!" << std::endl;
}
static void staticDisplay() {
// display(); // This would cause an error
Example ex; // Create an instance
ex.display(); // Call the non-static member function using an instance
}
};
int main() {
Example example;
example.display(); // Correct usage
Example::staticDisplay(); // Correct usage
return 0;
}
```
By adhering to these guidelines and understanding the context in which non-static member functions can be called, developers can effectively avoid the "reference to non-static member function must be called" error in their C++ code. Proper object instantiation and method invocation practices are key to ensuring smooth functionality within class structures.
Understanding the ‘Non-Static Member Function’ Error in C++
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “The error ‘reference to non-static member function must be called’ typically arises when a developer attempts to reference a non-static member function without an object context. This is a common pitfall in C++ programming, especially for those transitioning from languages that allow more flexible function calls.”
James Liu (C++ Programming Instructor, Tech University). “Understanding the distinction between static and non-static member functions is crucial. Non-static member functions require an instance of the class to be invoked, while static functions do not. Failing to recognize this can lead to confusion and compilation errors.”
Sarah Thompson (Lead Developer, NextGen Software). “To resolve the ‘reference to non-static member function must be called’ error, ensure that you are calling the function on an instance of the class. Utilizing pointers or references to the class instance can also help clarify the context in which the function is being called.”
Frequently Asked Questions (FAQs)
What does the error “reference to non-static member function must be called” mean?
This error indicates that you are attempting to reference a non-static member function without an instance of the class. Non-static member functions require an object of the class to be called.
How can I resolve this error in my code?
To resolve this error, ensure that you are calling the non-static member function on an instance of the class. For example, use `objectName.functionName()` instead of just `functionName()`.
Can I call a non-static member function from a static context?
No, you cannot directly call a non-static member function from a static context because static functions do not have access to instance-specific data. You must create an instance of the class to call the non-static function.
What is the difference between static and non-static member functions?
Static member functions belong to the class itself and can be called without an instance. Non-static member functions require an instance of the class to access instance-specific data and behavior.
Are there any scenarios where this error might not be applicable?
This error is specifically related to C++ and similar languages. In languages that support different paradigms, such as Python or JavaScript, the rules for calling methods may differ, and this specific error may not occur.
Can I use pointers or references to call non-static member functions?
Yes, you can use pointers or references to call non-static member functions. Ensure that the pointer or reference points to a valid instance of the class before invoking the function.
The error message “reference to non-static member function must be called” typically arises in C++ programming when a developer attempts to reference a non-static member function without an instance of the class. Non-static member functions are designed to operate on instances of a class, which means they require an object to be invoked. This error often indicates a misunderstanding of the distinction between static and non-static functions within the object-oriented paradigm of C++.
To resolve this error, it is essential to ensure that non-static member functions are called on an instance of the class. Developers should create an object of the class and then use that object to call the desired function. This reinforces the concept that non-static functions are inherently tied to the state of a specific object, and thus, cannot be invoked without one. Understanding this principle is crucial for effective C++ programming and for avoiding similar errors in the future.
In summary, the “reference to non-static member function must be called” error serves as a reminder of the importance of object-oriented principles in C++. By ensuring that non-static member functions are invoked on class instances, developers can not only eliminate this error but also enhance their understanding of class behavior and object interactions. This knowledge is foundational for writing robust and efficient
Author Profile
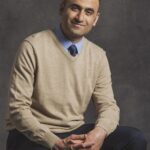
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?