Why Does My ESP32 FreeRTOS Task Keep Timing Out?
In the world of embedded systems, the ESP32 microcontroller has emerged as a favorite among developers for its versatility and robust capabilities. When paired with FreeRTOS, a real-time operating system, the ESP32 can handle multiple tasks efficiently, making it ideal for complex applications. However, as with any powerful tool, challenges can arise—one of the most common being task timeouts. Understanding how to manage and troubleshoot task timeouts in FreeRTOS is crucial for ensuring your applications run smoothly and reliably. In this article, we will explore the intricacies of task management on the ESP32, shedding light on the causes of timeouts and offering strategies to prevent them.
Task timeouts can occur for various reasons, often linked to resource contention, improper task prioritization, or misconfigured timeouts. When a task exceeds its allocated time, it can lead to performance bottlenecks, unresponsive systems, or even crashes. Developers need to be aware of how FreeRTOS manages task scheduling and how to configure their tasks to avoid these pitfalls. By understanding the underlying mechanics of task execution and timeout handling, you can enhance the reliability of your ESP32 applications.
Moreover, effective debugging and monitoring techniques can significantly aid in identifying and resolving timeout issues. Whether you’re a seasoned
Understanding Task Timeout in FreeRTOS
In FreeRTOS, a task timeout occurs when a task fails to complete its operation within a specified time period. This situation can arise due to various factors such as resource contention, improper task priority management, or waiting for events that do not occur. Understanding how to effectively manage task timeouts is crucial for maintaining system reliability and responsiveness.
Key factors influencing task timeouts include:
- Task Priorities: FreeRTOS allows for the assignment of different priorities to tasks. A lower-priority task may be preempted by higher-priority tasks, potentially leading to a timeout if the lower-priority task is unable to execute in a timely manner.
- Resource Availability: Tasks often wait for resources (e.g., queues, semaphores) to become available. If these resources are not released in time, the tasks may experience timeouts.
- Blocking Calls: Functions that block a task until an event occurs can lead to timeouts if the expected event never happens.
Configuring Timeouts
When creating tasks, it is essential to configure timeouts appropriately to ensure that your application behaves as expected under various conditions. FreeRTOS provides several mechanisms to handle timeouts effectively.
Timeouts can be configured for:
- Queue Operations: When sending or receiving data from a queue, you can specify a timeout period. If the operation cannot complete within this period, it will return an error code.
- Semaphore Operations: Similarly, when attempting to take a semaphore, you can specify a timeout to prevent indefinite blocking.
- Task Notifications: FreeRTOS also allows for task notifications, which can include timeouts to manage asynchronous events.
Example of configuring a timeout for a queue receive operation:
“`c
if (xQueueReceive(myQueue, &data, pdMS_TO_TICKS(1000)) == pd) {
// Handle timeout
}
“`
Handling Timeouts
When a timeout occurs, it is critical to have a robust handling mechanism in place. Here are some strategies:
- Error Handling: Implement error handling routines to manage timeout scenarios, such as retrying the operation or logging the event for debugging purposes.
- State Management: Use state machines to manage the different states of your task, allowing for a clear response to timeouts.
- Dynamic Adjustment: Consider dynamically adjusting timeouts based on system load or task execution time to enhance responsiveness.
Timeout Management Table
Operation | Timeout Configuration | Handling Strategy |
---|---|---|
Queue Receive | pdMS_TO_TICKS(milliseconds) | Retry or log error |
Semaphore Take | pdMS_TO_TICKS(milliseconds) | Use state machine to handle timeout |
Task Notification | Configure timeout in notification wait | Dynamic adjustment based on previous execution times |
By implementing these practices, developers can effectively manage task timeouts in FreeRTOS, ensuring that the system remains responsive and reliable even under challenging conditions.
Understanding Task Timeouts in FreeRTOS on ESP32
FreeRTOS allows developers to manage tasks efficiently, but sometimes tasks may time out due to various reasons. Understanding these timeouts is crucial for effective task management and system reliability.
Common Causes of Task Timeouts
Several factors can lead to tasks timing out in FreeRTOS:
- Blocking Operations: If a task is waiting for a resource (like a semaphore or mutex) that is not available, it can cause a timeout.
- Priority Inversion: Lower priority tasks holding resources needed by higher priority tasks can lead to delays and potential timeouts.
- Insufficient Stack Size: A task running out of stack space can disrupt normal operation, leading to unexpected behavior and timeouts.
- Long-Running Tasks: Tasks that take too long to execute without yielding can prevent other tasks from running, leading to timeouts.
- Configuration Issues: Incorrect FreeRTOS configurations, such as tick frequency or time slice allocation, can also contribute to task timeouts.
Debugging Task Timeouts
To diagnose and resolve timeout issues, consider the following steps:
- Increase Task Stack Size: Ensure that each task has sufficient stack space allocated.
- Review Blocking Calls: Analyze the usage of semaphores, queues, and other blocking calls to identify potential deadlocks.
- Check Task Priorities: Assess and adjust task priorities to prevent priority inversion issues.
- Use Trace Tools: Employ FreeRTOS trace tools to monitor task execution and identify bottlenecks.
- Log Execution Times: Implement logging for task execution times to pinpoint delays.
Configuration Parameters Related to Timeouts
The following FreeRTOS configuration parameters can impact task timeout behavior:
Parameter | Description |
---|---|
`configUSE_PREEMPTION` | Determines if preemptive scheduling is enabled. |
`configTICK_RATE_HZ` | Sets the tick rate, affecting how time is managed. |
`configMAX_PRIORITIES` | Defines the maximum number of task priorities available. |
`configMINIMAL_STACK_SIZE` | Sets the minimum stack size for tasks, crucial for avoiding overflow. |
Best Practices to Prevent Task Timeouts
Implementing best practices can significantly reduce the risk of task timeouts:
- Design for Responsiveness: Break long tasks into smaller chunks to allow other tasks to execute in between.
- Use Event Groups: Instead of blocking calls, utilize event groups to signal task readiness.
- Regularly Audit Task Code: Periodically review and refactor task code to improve efficiency and responsiveness.
- Monitor Resource Usage: Track resource allocation and usage patterns to prevent starvation and bottlenecks.
Handling Timeouts Gracefully
In cases where timeouts are inevitable, ensuring graceful handling is essential:
- Implement Timeout Callbacks: Define callback functions that execute when a timeout occurs, allowing for recovery or resource release.
- Retry Mechanisms: Use retry strategies for tasks that can recover from a timeout, thereby enhancing system robustness.
- Fallback Procedures: Establish fallback mechanisms for critical tasks to prevent complete system failure.
While task timeouts in FreeRTOS on ESP32 can pose challenges, understanding their causes and implementing effective strategies can mitigate these issues. Through careful design and diligent monitoring, developers can enhance the reliability and performance of their applications.
Understanding ESP32 FreeRTOS Task Timeout Challenges
Dr. Emily Carter (Embedded Systems Engineer, Tech Innovations Inc.). “In my experience, task timeouts in FreeRTOS on the ESP32 often stem from improper task prioritization. Ensuring that higher priority tasks are not starved of CPU time is crucial to prevent unexpected timeouts.”
Mark Thompson (IoT Solutions Architect, Smart Devices Co.). “Monitoring task execution time and implementing watchdog timers can significantly mitigate timeout issues. It’s essential to analyze task execution patterns to identify bottlenecks that could lead to timeouts.”
Lisa Chen (Firmware Developer, Open Source Hardware Group). “The configuration of the FreeRTOS scheduler plays a vital role in task management. Developers should ensure that the stack size and task delay parameters are set appropriately to avoid unintended timeouts.”
Frequently Asked Questions (FAQs)
What causes an ESP32 FreeRTOS task to time out?
An ESP32 FreeRTOS task may time out due to various reasons, including blocking operations that exceed the specified timeout duration, insufficient stack size, or high CPU load that prevents the task from executing in a timely manner.
How can I increase the timeout duration for a FreeRTOS task?
You can increase the timeout duration by adjusting the parameters in the task creation function, such as `vTaskDelay()` or `xSemaphoreTake()`, where you specify the timeout value in ticks.
What is the default timeout for FreeRTOS tasks on ESP32?
The default timeout for FreeRTOS tasks on ESP32 is not fixed; it depends on the specific API function being used. For example, `xSemaphoreTake()` has a default timeout of `portMAX_DELAY` if not specified otherwise.
How can I debug a FreeRTOS task timeout issue on ESP32?
To debug a FreeRTOS task timeout issue, use logging functions like `ESP_LOGI()` to track task execution and resource availability. Additionally, utilize FreeRTOS’s built-in trace tools and task status functions to analyze task states and timing.
What are the implications of a task timing out in FreeRTOS on ESP32?
When a task times out in FreeRTOS, it may lead to resource leaks, unresponsive behavior, or missed deadlines, which can affect overall system performance and reliability.
Can task priority affect timeout behavior in FreeRTOS on ESP32?
Yes, task priority can significantly affect timeout behavior. Higher priority tasks may preempt lower priority ones, causing delays in execution and potentially leading to timeouts if lower priority tasks are not scheduled in a timely manner.
The ESP32, a popular microcontroller, is often used in projects requiring multitasking capabilities. When utilizing FreeRTOS, developers may encounter task timeouts, which can occur due to various reasons such as insufficient stack size, priority issues, or improper synchronization mechanisms. Understanding these factors is crucial for effective task management and ensuring that applications run smoothly without unexpected delays or failures.
One of the primary causes of task timeouts in FreeRTOS is the stack size allocated to each task. If the stack size is too small, it can lead to stack overflow, causing the task to hang or terminate unexpectedly. Developers should carefully assess the stack requirements of their tasks and adjust the configuration accordingly to prevent such issues. Additionally, task priorities play a significant role; tasks with lower priority may not get CPU time if higher priority tasks are consistently running, leading to perceived timeouts.
Another important aspect to consider is the synchronization between tasks. Using mechanisms such as semaphores or mutexes can help manage shared resources effectively. However, improper use of these synchronization tools can lead to deadlocks or priority inversion, which can also manifest as timeouts. Therefore, it is essential to implement robust error handling and monitoring to diagnose and resolve these issues promptly.
Author Profile
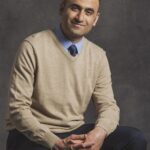
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?