Why Does Every Derived Table Need Its Own Alias in SQL?
In the world of SQL, the intricacies of query writing can often lead to confusion, especially when it comes to the nuances of derived tables. One common pitfall that many developers encounter is the requirement that “every derived table must have its own alias.” This seemingly straightforward rule can trip up even seasoned SQL practitioners, leading to errors that can derail an otherwise well-structured query. Understanding this concept is crucial for anyone looking to master SQL and enhance their database querying skills.
Derived tables, which are essentially temporary result sets created within a SQL query, play a pivotal role in simplifying complex data retrieval tasks. However, without proper aliasing, these derived tables can create ambiguity in your SQL statements, making it difficult for the database engine to interpret your intentions. This requirement for aliasing not only helps in maintaining clarity but also ensures that your queries are more readable and maintainable. As we delve deeper into this topic, we’ll explore the reasons behind this rule, its implications on query performance, and best practices for effectively using derived tables in your SQL code.
By grasping the importance of aliasing derived tables, you’ll be better equipped to write efficient and error-free SQL queries. Whether you’re a novice eager to learn the ropes or an experienced developer looking to refine your skills, understanding this fundamental
Understanding Derived Tables
Derived tables are temporary result sets generated from a subquery within a SQL statement. They provide a way to simplify complex queries by breaking them down into manageable pieces. However, one critical rule in SQL is that every derived table must have its own alias. This requirement ensures that the derived table can be referenced later in the query.
When you create a derived table, you define it in the `FROM` clause of your SQL statement. For example:
“`sql
SELECT *
FROM (SELECT column1, column2 FROM your_table WHERE condition) AS derived_table_alias;
“`
In this example, `derived_table_alias` serves as the alias for the derived table. Without this alias, SQL will return an error, as it cannot identify the temporary result set.
Importance of Aliasing Derived Tables
Using aliases for derived tables provides several benefits:
- Readability: Aliases clarify the purpose of derived tables, making queries easier to understand.
- Avoiding Errors: Aliasing prevents ambiguity when referencing columns from the derived table.
- Reusability: You can refer to the alias multiple times within the same query, enhancing efficiency.
Common Errors Related to Aliasing
Failing to provide an alias for a derived table will result in an error, typically something akin to “every derived table must have its own alias.” It is essential to be aware of the common pitfalls:
Error Type | Description |
---|---|
Missing Alias | Forgetting to provide an alias results in a syntax error. |
Incorrect Alias Usage | Misusing the alias in subsequent references can lead to confusion. |
Best Practices for Using Derived Tables
To effectively use derived tables and avoid common errors, consider the following best practices:
- Always provide a clear and concise alias for every derived table.
- Use meaningful aliases that reflect the content or purpose of the derived table.
- Keep your queries organized by formatting them properly, separating subqueries from main queries.
By adhering to these practices, you can enhance the clarity and maintainability of your SQL queries.
Understanding the Requirement for Aliases in Derived Tables
In SQL, a derived table is a temporary result set that you can use within a `SELECT` statement. Derived tables are often used to simplify complex queries by breaking them down into more manageable parts. However, one essential rule when using derived tables is that each must have its own alias.
Why Aliases are Mandatory
The requirement for aliases serves several purposes:
- Clarity: Aliases provide a clear name for the derived table, making it easier to reference in the query.
- Scope Management: Aliases help to define the scope of the derived table, preventing potential conflicts with other tables or columns in the query.
- Readability: By giving a descriptive alias, the SQL query becomes more understandable to others who may read or maintain it.
Syntax for Using Derived Tables with Aliases
The syntax for defining a derived table with an alias is straightforward. Here’s a basic structure:
“`sql
SELECT column_name(s)
FROM (
SELECT column_name(s)
FROM table_name
WHERE condition
) AS alias_name
WHERE another_condition;
“`
Example:
“`sql
SELECT employee_name, total_sales
FROM (
SELECT employee_id, SUM(sales_amount) AS total_sales
FROM sales
GROUP BY employee_id
) AS sales_summary
WHERE total_sales > 10000;
“`
In this example, `sales_summary` is the alias for the derived table, which aggregates sales data per employee.
Common Mistakes When Using Derived Tables
When working with derived tables, several common mistakes can occur:
- Omitting the Alias: Failing to provide an alias will result in a syntax error.
- Using Invalid Characters: Aliases should not contain spaces or special characters, which may lead to confusion or errors.
- Referencing Non-Existent Columns: Ensure that the columns you refer to in the outer query exist in the derived table.
Best Practices for Naming Aliases
To enhance the quality of your SQL queries, consider the following best practices for naming derived table aliases:
- Descriptive Names: Use names that clearly describe the purpose of the derived table, such as `monthly_sales` or `top_customers`.
- Consistency: Maintain a consistent naming convention throughout your queries for easier readability.
- Avoid Abbreviations: While brevity can be beneficial, avoid excessive abbreviations that may confuse others.
The Importance of Aliases in Derived Tables
Adhering to the requirement of providing an alias for every derived table is crucial for writing effective SQL queries. This practice not only enhances the clarity and maintainability of your code but also ensures that your queries execute without errors. By following the outlined guidelines and best practices, you can create SQL statements that are both efficient and easy to understand.
Understanding the Necessity of Aliases for Derived Tables
Dr. Emily Carter (Database Architect, Tech Solutions Inc.). “In SQL, every derived table must have its own alias to ensure clarity and avoid ambiguity in queries. This practice enhances readability and allows for easier debugging, especially in complex joins.”
Michael Chen (Senior Data Analyst, Insight Analytics). “Using aliases for derived tables is not merely a requirement; it is a best practice that promotes maintainability. When working with nested queries, having distinct aliases prevents confusion and helps in understanding the data flow.”
Laura Patel (SQL Trainer and Consultant, DataWise Academy). “The rule that every derived table must have its own alias is crucial for SQL syntax compliance. Without an alias, the query will fail, which underscores the importance of this convention in writing effective SQL statements.”
Frequently Asked Questions (FAQs)
What does it mean that every derived table must have its own alias?
Every derived table in SQL, which is essentially a subquery used in the FROM clause, must be assigned a unique alias. This is necessary for the database engine to reference the derived table correctly in subsequent parts of the query.
Why is an alias required for derived tables?
An alias is required for derived tables to provide a clear reference point within the query. Without an alias, the SQL engine cannot identify the derived table, leading to syntax errors and preventing the execution of the query.
Can I use the same alias for multiple derived tables in a single query?
No, each derived table must have a unique alias within the same query. Using the same alias for multiple derived tables will result in ambiguity, causing the SQL engine to throw an error.
How do I create an alias for a derived table?
To create an alias for a derived table, you simply follow the derived table definition with the keyword AS, followed by the desired alias name. For example: `(SELECT column1, column2 FROM table_name) AS alias_name`.
What happens if I forget to assign an alias to a derived table?
If you forget to assign an alias to a derived table, the SQL query will fail to execute, and an error message will indicate that the derived table requires an alias. This is a mandatory requirement in SQL syntax.
Are there any exceptions to the alias requirement for derived tables?
There are no exceptions to the alias requirement for derived tables in SQL. Every derived table, regardless of its complexity or purpose, must have a unique alias to ensure proper identification and functionality within the query.
In SQL, a derived table is a temporary result set that is generated within a query. It is crucial to note that every derived table must have its own alias. This requirement stems from the need for clarity and organization in SQL queries, enabling the database engine to reference the derived table effectively. Without an alias, the SQL syntax becomes ambiguous, leading to potential errors during execution.
Furthermore, the use of aliases enhances the readability of the SQL code. By assigning a meaningful name to a derived table, developers can make their queries more understandable for others who may read or maintain the code in the future. This practice is especially important in complex queries involving multiple derived tables, as it helps to distinguish between different result sets and their purposes.
In summary, the necessity for every derived table to have its own alias is a fundamental aspect of SQL query construction. It not only ensures proper execution but also promotes better code organization and clarity. Adhering to this rule is essential for anyone looking to write efficient and maintainable SQL queries.
Author Profile
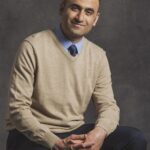
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?