What Does It Mean When the Exception of Type ‘System.OutOfMemoryException’ Is Thrown?
In the intricate world of software development, encountering errors is an inevitable part of the journey. Among the myriad of exceptions that developers may face, the `System.OutOfMemoryException` stands out as a particularly daunting challenge. This exception signals a critical issue: the application has exhausted the available memory resources, leading to a halt in operations. Understanding the nuances of this exception is vital for developers, as it not only affects application performance but can also impact user experience and system stability. In this article, we will delve into the causes, implications, and strategies for effectively managing and preventing this formidable exception.
Overview
The `System.OutOfMemoryException` typically arises when an application attempts to allocate more memory than is available in the system, whether due to memory leaks, inefficient resource management, or simply a high demand for resources. This exception can occur in various environments, from desktop applications to large-scale web services, and can manifest in numerous ways, often leaving developers scrambling to identify the root cause. As applications grow in complexity and data volume, the risk of encountering this exception increases, making it imperative for developers to be well-versed in its implications.
Addressing the `System.OutOfMemoryException` requires a multifaceted approach that encompasses both preventative measures and effective troubleshooting
Understanding OutOfMemoryException
OutOfMemoryException is a common runtime error that occurs in applications when the common language runtime (CLR) cannot allocate enough memory for an object. This exception is particularly prevalent in applications that manage large datasets or utilize extensive resources. In the context of .NET applications, it signals that the system has exhausted its available memory, which can stem from various factors.
Common Causes
Several conditions can lead to an OutOfMemoryException:
- Memory Leaks: Unmanaged resources are not released properly, causing memory to be consumed over time without being freed.
- Large Object Allocations: Attempting to allocate large arrays or collections that exceed the available heap size.
- Fragmentation: The heap can become fragmented, leading to a situation where large contiguous blocks of memory are unavailable, even if the total free memory exceeds the size of the allocation.
- Excessive Parallel Processing: Running too many tasks simultaneously can consume available memory rapidly.
Impact of OutOfMemoryException
The ramifications of encountering an OutOfMemoryException can be significant:
- Application Crashes: The immediate effect often results in the abrupt termination of the application.
- Degraded Performance: If the system is continually trying to allocate memory, performance issues may arise even before a crash occurs.
- Data Loss: In cases where the application does not handle the exception gracefully, user data may be lost or corrupted.
Prevention Strategies
To prevent OutOfMemoryException from occurring, consider implementing the following strategies:
- Optimize Memory Usage: Regularly review and optimize your code to minimize memory usage.
- Dispose Unmanaged Resources: Always dispose of unmanaged resources appropriately using `IDisposable` to ensure memory is freed.
- Use Memory Profiling Tools: Utilize tools to analyze memory usage and identify memory leaks or inefficiencies.
- Limit Parallel Tasks: Manage the number of parallel tasks to avoid overwhelming system memory.
Handling OutOfMemoryException
In cases where an OutOfMemoryException cannot be avoided, robust error handling mechanisms should be in place. The following approaches can be employed:
- Try-Catch Blocks: Implement try-catch blocks to catch the exception and log the error.
- Graceful Degradation: Design the application to continue functioning at a reduced capacity rather than crashing entirely.
- User Notifications: Inform users of the issue and provide options for saving work or retrying operations.
Strategy | Description | Benefits |
---|---|---|
Memory Optimization | Reduce memory footprint through efficient coding practices. | Improved performance and reduced risk of exceptions. |
Memory Profiling | Analyze memory usage to detect leaks and inefficiencies. | Identifies problem areas before they cause exceptions. |
Error Handling | Implement structured error handling to manage exceptions. | Enhances user experience and application reliability. |
Understanding the ‘System.OutOfMemoryException’
The `System.OutOfMemoryException` is a common error in .NET applications, indicating that the application has exhausted the available memory resources. This exception is typically thrown when an application tries to allocate memory for an object, but the Common Language Runtime (CLR) cannot fulfill the request due to insufficient memory.
Common Causes
Several factors can lead to an `OutOfMemoryException`, including:
- Memory Leaks: Unreleased resources or references to objects that are no longer needed can accumulate, leading to increased memory usage over time.
- Large Object Allocation: Attempting to allocate large arrays or collections may exceed available memory limits.
- Excessive Parallelism: Running too many threads concurrently can lead to higher memory usage, especially if each thread holds large objects.
- Fragmentation: Over time, memory fragmentation can occur, making it difficult for the runtime to find contiguous blocks of memory.
Impact of the Exception
When this exception occurs, the following impacts can be observed:
- Application Crashes: The application may terminate unexpectedly, leading to a loss of unsaved data.
- Degraded Performance: The application may slow down significantly before the exception is thrown, affecting user experience.
- Resource Exhaustion: Other applications running on the same system may also suffer from resource constraints.
Best Practices to Avoid OutOfMemoryException
Implementing best practices can help mitigate the risk of encountering an `OutOfMemoryException`:
- Monitor Memory Usage: Use profiling tools to track memory consumption and identify potential leaks.
- Optimize Data Structures: Choose appropriate data structures based on use-case requirements to minimize memory footprint.
- Dispose of Resources: Implement the IDisposable interface to properly release unmanaged resources.
- Limit Large Object Allocations: Break large arrays or collections into smaller manageable chunks.
- Use Weak References: For objects that can be recreated, consider using weak references to allow the garbage collector to reclaim memory.
Handling OutOfMemoryException
When an `OutOfMemoryException` is encountered, consider the following strategies:
- Catch and Log: Implement try-catch blocks to gracefully handle the exception and log relevant details for further analysis.
- Graceful Degradation: Allow the application to continue running with reduced functionality, if applicable.
- User Notifications: Inform users about the issue and suggest actions to mitigate the impact, such as freeing up memory.
- Automated Recovery: Implement logic to restart specific operations or the application itself in case of an exception.
Debugging Techniques
To effectively debug and resolve `OutOfMemoryException`, employ the following techniques:
Technique | Description |
---|---|
Memory Profiling | Use profiling tools (e.g., Visual Studio Diagnostic Tools) to monitor memory usage patterns. |
Dump Analysis | Analyze memory dumps using tools like WinDbg to identify memory allocations and leaks. |
Code Review | Conduct thorough code reviews focused on memory management practices. |
Garbage Collection Logs | Enable and review garbage collection logs to understand memory allocation and reclamation behavior. |
By applying these strategies and understanding the implications of `System.OutOfMemoryException`, developers can enhance the robustness and reliability of their applications.
Understanding the ‘System.OutOfMemoryException’ in Software Development
Dr. Emily Carter (Senior Software Architect, Tech Innovations Inc.). “The ‘System.OutOfMemoryException’ is a critical error that indicates the application has exhausted the available memory resources. Developers must implement efficient memory management techniques and monitor memory usage to prevent this exception from disrupting application performance.”
Michael Chen (Lead Systems Engineer, Cloud Solutions Corp.). “When encountering a ‘System.OutOfMemoryException’, it is essential to analyze the application’s memory allocation patterns. Tools such as memory profilers can help identify memory leaks or excessive memory consumption, leading to more robust and scalable applications.”
Sarah Johnson (Principal Software Developer, Agile Development Group). “Addressing the ‘System.OutOfMemoryException’ requires a proactive approach. Implementing strategies like caching, optimizing data structures, and using asynchronous programming can significantly reduce memory footprint and enhance application stability.”
Frequently Asked Questions (FAQs)
What does the error ‘system.outofmemoryexception’ indicate?
The ‘system.outofmemoryexception’ indicates that the application has exhausted the available memory resources, preventing it from allocating additional memory for operations.
What are common causes of a ‘system.outofmemoryexception’?
Common causes include memory leaks, excessive data processing, large object allocations, or insufficient system memory. Inefficient algorithms that require large data structures can also contribute.
How can I troubleshoot a ‘system.outofmemoryexception’?
To troubleshoot, analyze memory usage patterns, optimize code to reduce memory consumption, use profiling tools to identify memory leaks, and consider increasing the system’s physical memory or adjusting application settings.
Can this exception occur in both desktop and server applications?
Yes, the ‘system.outofmemoryexception’ can occur in both desktop and server applications, as both types can encounter memory limitations based on their configurations and workloads.
Is there a way to prevent ‘system.outofmemoryexception’?
Preventive measures include optimizing memory usage by managing data efficiently, implementing garbage collection practices, and ensuring that the application is designed to handle large datasets appropriately.
What should I do if I encounter this exception in production?
If encountered in production, log the error details, analyze the application’s memory usage, and implement immediate fixes such as optimizing code or increasing memory resources. Long-term, consider refactoring the application to improve memory management.
The exception of type ‘System.OutOfMemoryException’ is a critical error encountered in .NET applications, indicating that the system has run out of memory to allocate for new objects. This exception can occur due to various reasons, including memory leaks, excessive memory consumption, or inefficient resource management within an application. Understanding the causes and implications of this exception is essential for developers to ensure the stability and performance of their applications.
One of the key takeaways is the importance of memory management in software development. Developers should implement best practices such as using memory profiling tools, optimizing data structures, and releasing unused resources to mitigate the risk of encountering this exception. Additionally, proper exception handling strategies should be in place to gracefully manage situations when memory limits are reached, thereby improving user experience and application reliability.
Furthermore, it is crucial to monitor application performance and memory usage over time. Regularly reviewing memory consumption patterns can help identify potential bottlenecks and areas for improvement. By proactively addressing these issues, developers can reduce the likelihood of ‘System.OutOfMemoryException’ occurrences and enhance the overall efficiency of their applications.
Author Profile
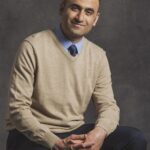
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?