How Can You Execute a PowerShell Script from Another PowerShell Script?
In the realm of Windows automation, PowerShell stands out as a powerful scripting language that empowers users to manage and configure systems with ease. One of the most compelling features of PowerShell is its ability to execute scripts from within other scripts, enabling a modular and organized approach to automation. Whether you’re a seasoned IT professional or a curious newcomer, understanding how to effectively nest scripts can significantly enhance your productivity and streamline complex tasks. This article delves into the nuances of executing a PowerShell script from another PowerShell script, unlocking the potential for more dynamic and efficient workflows.
When you execute a PowerShell script from another script, you open the door to a myriad of possibilities. This technique allows you to break down large, unwieldy scripts into manageable components, making it easier to maintain and troubleshoot your code. By calling external scripts, you can reuse code snippets, share functionality across projects, and create a more organized structure that adheres to best practices in scripting.
Moreover, the process of invoking one script from another can be tailored to meet specific needs, whether you’re passing parameters, handling return values, or managing execution contexts. As you explore the methods and best practices for executing scripts within scripts, you’ll discover how to harness the full power of PowerShell to create robust
Executing a PowerShell Script from Another PowerShell Script
To execute a PowerShell script from another PowerShell script, you can utilize a few straightforward methods. Each method offers different benefits depending on your specific requirements, such as the need for passing parameters or handling outputs.
Using the Call Operator
The call operator, represented by `&`, allows you to run a script by specifying its path. This method is particularly useful when you want to execute a script located in a different directory or when the script requires parameters.
Example:
“`powershell
& “C:\Path\To\YourScript.ps1”
“`
If the script requires parameters, you can pass them directly:
“`powershell
& “C:\Path\To\YourScript.ps1” -Param1 Value1 -Param2 Value2
“`
Using the Dot Sourcing Technique
Dot sourcing is another effective way to run a script within the current scope. This method allows any variables or functions defined in the called script to remain available after the script execution.
Example:
“`powershell
. “C:\Path\To\YourScript.ps1”
“`
This technique is ideal for scenarios where you want to maintain the state or modify global variables.
Using the Invoke-Expression Cmdlet
The `Invoke-Expression` cmdlet can also execute a script but is generally less preferred due to potential security risks, especially with untrusted input. It evaluates a string as a command.
Example:
“`powershell
Invoke-Expression “C:\Path\To\YourScript.ps1”
“`
This method can be useful if you dynamically construct the script path as a string.
Running Scripts with Parameters
When executing a script that requires parameters, it is essential to ensure that the parameters are correctly defined in the called script. Here’s a simple example of a script with parameters:
Script: YourScript.ps1
“`powershell
param (
[string]$Param1,
[int]$Param2
)
Write-Host “Parameter 1 is $Param1 and Parameter 2 is $Param2”
“`
You can call this script from another script as follows:
“`powershell
& “C:\Path\To\YourScript.ps1” -Param1 “Hello” -Param2 10
“`
Best Practices
When executing scripts from other scripts, consider the following best practices:
- Use full paths to avoid issues related to the current working directory.
- Validate input parameters to ensure they meet expected types and values.
- Handle errors gracefully by using try-catch blocks to manage exceptions.
- Document your scripts to clarify any dependencies or expected parameters.
Common Issues and Solutions
Issue | Solution |
---|---|
Script not found | Verify the path and ensure the script exists. |
Parameter errors | Check parameter names and types for accuracy. |
Permissions issues | Run PowerShell as an administrator if necessary. |
Execution policy restrictions | Set the execution policy with `Set-ExecutionPolicy`. |
Utilizing these methods and best practices will enhance your ability to manage PowerShell scripts efficiently and effectively.
Methods to Execute a PowerShell Script from Another PowerShell Script
Executing a PowerShell script from another PowerShell script can be accomplished through several methods. Each method has its own use cases and advantages depending on the specific requirements of the task.
Using the & Call Operator
The simplest way to run a script from another script is to use the `&` (call) operator. This allows you to invoke the script directly.
“`powershell
& “C:\Path\To\YourScript.ps1”
“`
- This method can handle both absolute and relative paths.
- Ensure the script path is enclosed in quotes if it contains spaces.
Using the . (Dot Sourcing) Operator
Dot sourcing allows you to run a script in the current scope. This means any variables or functions defined in the script will be available after execution.
“`powershell
. “C:\Path\To\YourScript.ps1”
“`
- Use this method when you need access to the variables or functions defined in the called script.
- It can lead to variable conflicts if not managed carefully.
Using Start-Process Cmdlet
For situations where you want to run the script in a new process (which may be desirable for isolation), you can use the `Start-Process` cmdlet.
“`powershell
Start-Process “powershell.exe” -ArgumentList “-File C:\Path\To\YourScript.ps1”
“`
- This method runs the script in a separate PowerShell instance.
- It is useful for running scripts that may take a long time or for those that should not interfere with the calling script.
Passing Parameters to the Called Script
When executing a script, you may need to pass parameters. Each of the methods described supports parameter passing.
- Using & Call Operator:
“`powershell
& “C:\Path\To\YourScript.ps1” -Param1 Value1 -Param2 Value2
“`
- Using Dot Sourcing:
“`powershell
. “C:\Path\To\YourScript.ps1” -Param1 Value1 -Param2 Value2
“`
- Using Start-Process:
“`powershell
Start-Process “powershell.exe” -ArgumentList “-File C:\Path\To\YourScript.ps1 -Param1 Value1 -Param2 Value2”
“`
Handling Execution Policy
Execution policies may restrict the execution of scripts. If you encounter issues, you might need to adjust the policy:
- Temporarily change the execution policy for the session:
“`powershell
Set-ExecutionPolicy -ExecutionPolicy Bypass -Scope Process
“`
- Use this command with caution and ensure your environment’s security policies are upheld.
Example Scenario
Here is an example scenario that illustrates the use of these methods:
Method | Example Command | Use Case |
---|---|---|
Call Operator | `& “C:\Scripts\MainScript.ps1″` | Simple execution without scope change |
Dot Sourcing | `. “C:\Scripts\MainScript.ps1″` | Need access to variables/functions |
Start-Process | `Start-Process “powershell.exe” -ArgumentList “-File C:\Scripts\MainScript.ps1″` | Isolate execution in a new process |
These methods provide flexibility in how you manage the execution of PowerShell scripts, allowing for a tailored approach based on your scripting needs.
Executing PowerShell Scripts: Expert Insights
Dr. Emily Carter (Senior Systems Administrator, Tech Solutions Inc.). “To execute a PowerShell script from another PowerShell script, one can utilize the ‘Invoke-Expression’ cmdlet or simply call the script directly by its path. This method allows for modular script design, enhancing maintainability and reusability in complex automation tasks.”
Mark Thompson (PowerShell Automation Specialist, Cloud Innovators). “Using the ‘&’ call operator is a straightforward approach for executing one script from another. This operator provides a clear syntax and helps avoid common pitfalls related to script execution policies, ensuring that scripts run smoothly in various environments.”
Linda Garcia (IT Security Consultant, SecureTech Advisory). “When executing scripts from other scripts, it is crucial to consider the execution policy settings in PowerShell. Implementing ‘Set-ExecutionPolicy’ appropriately can prevent security risks while allowing necessary scripts to run, thus balancing functionality with security compliance.”
Frequently Asked Questions (FAQs)
How can I execute a PowerShell script from another PowerShell script?
You can execute a PowerShell script from another script by using the `&` call operator followed by the path to the script. For example: `& “C:\Path\To\YourScript.ps1″`.
What is the difference between using the call operator and dot-sourcing?
The call operator (`&`) runs the script in a separate scope, meaning variables defined in the called script do not affect the calling script. Dot-sourcing (`. “C:\Path\To\YourScript.ps1″`) runs the script in the current scope, allowing variables and functions to persist in the calling script.
Can I pass parameters to a PowerShell script when executing it from another script?
Yes, you can pass parameters by including them after the script path. For example: `& “C:\Path\To\YourScript.ps1” -Param1 Value1 -Param2 Value2`.
What should I do if the script I want to execute requires administrative privileges?
If the script requires administrative privileges, you should run the calling script as an administrator. You can also use the `Start-Process` cmdlet with the `-Verb RunAs` parameter to elevate privileges when executing the script.
How can I handle errors when executing a script from another script?
You can use `try` and `catch` blocks to handle errors effectively. Wrap the script execution command in a `try` block and handle any exceptions in the `catch` block to manage errors gracefully.
Is there a way to check if the script executed successfully?
Yes, you can check the `$LASTEXITCODE` variable immediately after executing the script. A value of `0` indicates success, while any other value indicates an error occurred during execution.
Executing a PowerShell script from another PowerShell script is a common practice that enhances modularity and reusability in scripting. By using the `&` call operator or the `Invoke-Expression` cmdlet, users can seamlessly run external scripts, allowing for complex automation tasks to be broken down into manageable components. This method not only simplifies the management of code but also promotes better organization by enabling the separation of different functionalities into distinct scripts.
Additionally, it is essential to consider the context in which the scripts are executed. Scripts can be run in different scopes, which may affect variable accessibility and execution flow. Understanding how to manage parameters and return values between scripts is crucial for ensuring that they interact correctly. Utilizing techniques like passing arguments or returning output can significantly enhance the effectiveness of script execution.
mastering the execution of PowerShell scripts from one another is a valuable skill for any PowerShell user. It allows for the creation of sophisticated automation solutions while maintaining clarity and organization in code. By leveraging the appropriate methods and understanding the implications of script execution contexts, users can optimize their PowerShell scripting practices and improve overall productivity.
Author Profile
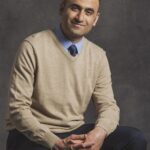
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?