How Can I Execute a PowerShell Script from a Batch File?
In the world of system administration and automation, the ability to seamlessly integrate different scripting languages can significantly enhance productivity and efficiency. One common scenario that many IT professionals encounter is the need to execute a PowerShell script from a batch file. This powerful combination allows users to leverage the strengths of both scripting environments, enabling complex tasks to be automated with ease. Whether you’re looking to streamline routine processes, manage system configurations, or perform bulk operations, understanding how to execute PowerShell scripts from a batch file can unlock a new level of versatility in your workflow.
At its core, executing a PowerShell script from a batch file involves creating a simple command that bridges the two scripting languages. This method not only simplifies the execution of PowerShell commands but also allows for the incorporation of legacy batch scripts into modern automation strategies. By using a batch file as a launching point, users can harness the advanced capabilities of PowerShell while maintaining the familiarity of traditional batch processing.
As you delve deeper into this topic, you’ll discover the various techniques and best practices for executing PowerShell scripts from batch files. From command-line parameters to error handling, each aspect plays a crucial role in ensuring that your scripts run smoothly and effectively. Prepare to explore the nuances of this integration, and equip yourself with the knowledge to enhance your scripting toolkit
Executing a PowerShell Script from a Batch File
To execute a PowerShell script from a batch file, you need to call the PowerShell executable and provide the path to the script. This can be accomplished using the `powershell.exe` command within the batch file.
Here’s a basic syntax for the command:
“`batch
powershell.exe -ExecutionPolicy Bypass -File “C:\path\to\your\script.ps1”
“`
Components of the Command
- powershell.exe: This is the executable file for PowerShell.
- -ExecutionPolicy Bypass: This parameter allows you to bypass the execution policy for the script, ensuring it runs without restrictions. It is advisable to understand the security implications of this option.
- -File: This parameter indicates that you are specifying a script file to execute.
- “C:\path\to\your\script.ps1”: This is the full path to your PowerShell script. Ensure the path is enclosed in quotes, especially if it contains spaces.
Example Batch File
Here’s a simple example of a batch file that executes a PowerShell script:
“`batch
@echo off
echo Starting PowerShell script…
powershell.exe -ExecutionPolicy Bypass -File “C:\Scripts\MyScript.ps1”
echo PowerShell script executed.
pause
“`
Considerations
- Path Considerations: Ensure that the path to your PowerShell script is correct. If the script is in the same directory as the batch file, you can use a relative path.
- Execution Policy: The default execution policy may prevent scripts from running. You can change the policy temporarily using the `-ExecutionPolicy Bypass` option, but it is recommended to configure the policy appropriately for your environment.
- Error Handling: It is good practice to include error handling in your PowerShell script to manage any issues that arise during execution.
Common Issues
Issue | Description | Solution |
---|---|---|
Script Not Found | The specified script path is incorrect. | Double-check the script path and filename. |
Execution Policy Restriction | The script is not allowed to run due to policy settings. | Use `-ExecutionPolicy Bypass` or set a policy that allows script execution. |
Permissions Issues | The user running the batch file does not have the necessary permissions. | Ensure the user has permissions to execute the script. |
Additional Parameters
You can also pass parameters to your PowerShell script from the batch file. This is done by appending them after the script name:
“`batch
powershell.exe -ExecutionPolicy Bypass -File “C:\Scripts\MyScript.ps1” -Param1 Value1 -Param2 Value2
“`
In the PowerShell script, you can access these parameters as follows:
“`powershell
param (
[string]$Param1,
[string]$Param2
)
“`
Incorporating these practices will enable you to effectively run PowerShell scripts from a batch file while managing permissions and execution policies safely.
Executing a PowerShell Script from a Batch File
To execute a PowerShell script from a batch file, you can use the `powershell` command with the appropriate parameters. Below are the key steps and considerations involved in this process.
Basic Command Structure
The basic command structure for running a PowerShell script within a batch file is as follows:
“`batch
powershell -ExecutionPolicy Bypass -File “C:\Path\To\YourScript.ps1”
“`
Explanation of Components:
- powershell: This invokes the PowerShell executable.
- -ExecutionPolicy Bypass: This parameter allows the script to run without being blocked by execution policies. It’s useful if your system restricts script execution.
- -File: This flag specifies the path to the PowerShell script that you want to execute.
- “C:\Path\To\YourScript.ps1”: Replace this with the actual path to your PowerShell script.
Creating the Batch File
To create a batch file that executes a PowerShell script, follow these steps:
- Open Notepad or any text editor.
- Enter the PowerShell command as described above.
- Save the file with a `.bat` extension, for example, `RunPowerShellScript.bat`.
Example Batch File Content:
“`batch
@echo off
powershell -ExecutionPolicy Bypass -File “C:\Scripts\MyScript.ps1”
pause
“`
- @echo off: This command prevents the batch file from displaying each command in the console.
- pause: This keeps the console window open after the script execution, allowing you to see any output or errors.
Handling Spaces in File Paths
If your script path contains spaces, ensure you enclose the path in double quotes. For example:
“`batch
powershell -ExecutionPolicy Bypass -File “C:\My Scripts\MyScript.ps1”
“`
Running the Batch File
To execute the batch file:
- Navigate to the directory where the batch file is saved.
- Double-click the batch file or run it from the command prompt by typing its name.
Common Issues and Troubleshooting
- Execution Policy Errors: If you encounter execution policy errors, ensure you are using `-ExecutionPolicy Bypass` correctly.
- Script Not Found: Verify that the path to your PowerShell script is correct.
- Permissions: Ensure that you have the necessary permissions to execute the script and access the specified path.
Alternative Method Using PowerShell Command
You can also run PowerShell commands directly from the batch file without referencing a script file. For example:
“`batch
@echo off
powershell -ExecutionPolicy Bypass -Command “Get-Process | Out-File C:\ProcessList.txt”
pause
“`
Benefits of This Approach:
- Directly execute commands without creating separate script files.
- Useful for quick tasks or automation.
By following the outlined steps and guidelines, you can effectively execute PowerShell scripts from batch files, enhancing your automation capabilities in Windows environments.
Executing PowerShell Scripts from Batch Files: Expert Insights
Dr. Emily Carter (Senior Systems Engineer, Tech Innovations Inc.). “Executing PowerShell scripts from batch files is a powerful method to automate complex tasks. By using the ‘powershell.exe’ command followed by the script path, users can seamlessly integrate PowerShell functionality within their batch processes, enhancing efficiency and control.”
Michael Chen (IT Automation Specialist, FutureTech Solutions). “When embedding PowerShell scripts in batch files, it is crucial to handle the execution policy appropriately. Utilizing the ‘-ExecutionPolicy Bypass’ flag allows scripts to run without restrictions, which is particularly useful in environments where security settings may otherwise hinder execution.”
Lisa Tran (Cybersecurity Analyst, SecureNet Labs). “While executing PowerShell scripts from batch files can streamline operations, it is essential to consider security implications. Ensure that scripts are sourced from trusted locations and implement logging to monitor script execution, thereby mitigating potential risks associated with automation.”
Frequently Asked Questions (FAQs)
How can I execute a PowerShell script from a batch file?
You can execute a PowerShell script from a batch file by using the following command: `powershell -ExecutionPolicy Bypass -File “C:\path\to\your\script.ps1″`. This command allows the script to run without being blocked by execution policies.
What is the purpose of the ExecutionPolicy parameter?
The ExecutionPolicy parameter controls the conditions under which PowerShell loads configuration files and runs scripts. Using `Bypass` allows the script to run without restrictions, which is useful for automation tasks.
Can I pass parameters to a PowerShell script from a batch file?
Yes, you can pass parameters by appending them to the script call. For example: `powershell -ExecutionPolicy Bypass -File “C:\path\to\your\script.ps1” -Param1 Value1 -Param2 Value2`.
What should I do if my PowerShell script requires administrator privileges?
If your script requires elevated privileges, you can create a shortcut to the batch file and set it to run as an administrator. Alternatively, you can use the `Start-Process` cmdlet in PowerShell with the `-Verb RunAs` parameter.
Are there any security concerns when executing PowerShell scripts from a batch file?
Yes, executing scripts from a batch file can pose security risks, especially if the script source is untrusted. Ensure that you review and validate the script’s content before execution to avoid potential security vulnerabilities.
What should I do if the PowerShell script does not execute as expected?
If the script does not execute, check for syntax errors, ensure the correct file path is specified, and verify that the PowerShell execution policy allows script execution. Additionally, you can run the script directly in PowerShell to troubleshoot any issues.
Executing a PowerShell script from a batch file is a straightforward process that can enhance automation and streamline workflows in various computing environments. By utilizing the command line, users can easily call PowerShell scripts from within a batch file, allowing for the integration of complex PowerShell functionalities into simpler batch file operations. This capability is particularly useful for system administrators and users who wish to leverage the power of PowerShell while maintaining compatibility with legacy batch processes.
There are several methods to execute a PowerShell script from a batch file, including using the ‘powershell.exe’ command followed by the script path. It is essential to ensure that the execution policy is set appropriately to allow the script to run. Additionally, users can choose to run the script in a new PowerShell window or in the background, depending on their specific needs. Proper error handling and logging can also be implemented to monitor the execution of the script effectively.
the ability to execute PowerShell scripts from batch files not only enhances automation capabilities but also allows users to harness the advanced features of PowerShell in a familiar batch environment. Understanding the syntax and execution policies involved is crucial for successful implementation. This integration can lead to increased efficiency and improved management of tasks across different systems.
Author Profile
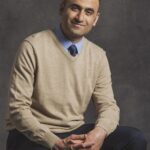
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?