Why Am I Getting ‘expometroconfig.loadasync is Not a Function’ Error?
In the ever-evolving landscape of web development, encountering errors can be a common yet frustrating experience. One such error that has puzzled many developers is the message: `expometroconfig.loadasync is not a function`. This seemingly cryptic notification can halt progress and lead to hours of debugging, leaving developers questioning their code and the libraries they rely on. Understanding the root causes of this error is crucial for anyone working with JavaScript frameworks, particularly those that integrate with complex configurations and asynchronous operations.
As we delve into the intricacies of this error, we will explore the context in which it typically arises, the potential pitfalls of asynchronous function calls, and the common scenarios that lead to this issue. Whether you are a seasoned developer or a newcomer to the world of coding, grasping the underlying principles of function definitions and library integrations will empower you to troubleshoot effectively. By dissecting the components of `expometroconfig` and its intended functionalities, we aim to equip you with the knowledge needed to resolve this error swiftly and enhance your coding proficiency.
Join us as we unravel the complexities of the `loadasync` function and provide insights into best practices for managing configurations in your projects. With a clearer understanding of this error, you will be better prepared to navigate the challenges
Understanding the Error
The error message `expometroconfig.loadasync is not a function` typically indicates that the JavaScript runtime is unable to locate the `loadasync` function within the `expometroconfig` object. This can stem from several underlying issues, which can be categorized as follows:
- or Null Object: The `expometroconfig` variable might not be initialized or is set to `null` before calling the `loadasync` method.
- Incorrect Library Version: The version of the library or module that is expected to provide `loadasync` may be outdated or incompatible.
- Scope Issues: The function may not be accessible in the current scope due to module encapsulation or improper imports.
- Typographical Errors: There could be a simple spelling error in the function name or the object it is being called on.
Troubleshooting Steps
To address the error effectively, consider the following troubleshooting steps:
- Check Initialization: Ensure that `expometroconfig` is initialized correctly. You can verify this by logging the object to the console.
“`javascript
console.log(expometroconfig);
“`
- Verify Function Existence: Use `typeof` to check whether `loadasync` is defined.
“`javascript
console.log(typeof expometroconfig.loadasync);
“`
- Inspect Library Version: Confirm that you are using the correct version of the library that contains the `loadasync` method. Review the documentation or the changelog for any updates or changes in API.
- Review Imports: Ensure that you have imported the module correctly if you’re using a module system (e.g., ES6 imports, CommonJS).
- Check for Typos: Carefully review your code for any typographical errors in the function name.
Example Debugging Code
Here is an example of how you might structure your code to include checks for the aforementioned issues:
“`javascript
if (expometroconfig && typeof expometroconfig.loadasync === ‘function’) {
expometroconfig.loadasync();
} else {
console.error(“expometroconfig.loadasync is not a function or expometroconfig is .”);
}
“`
Common Causes
Below is a summary table of common causes for the error along with potential fixes:
Cause | Potential Fix |
---|---|
or null object | Ensure `expometroconfig` is properly initialized. |
Incorrect library version | Update to the correct version of the library. |
Scope issues | Check the scope and ensure proper imports. |
Typographical errors | Review function names and object references for typos. |
By carefully examining these aspects, developers can often isolate and resolve the issue leading to the `expometroconfig.loadasync is not a function` error.
Understanding the Error
The error message `expometroconfig.loadasync is not a function` typically indicates that the method `loadasync` is being called on an object that does not possess this function. This can stem from various issues, including but not limited to:
- Incorrect Object Type: The `expometroconfig` may not be initialized correctly or may not be an instance of the expected class that contains `loadasync`.
- Library Version Mismatch: The function may not exist in the version of the library being used. Ensure that the library version matches the documentation.
- Loading Sequence: The function might be called before the library is fully loaded or initialized.
Troubleshooting Steps
To effectively diagnose and resolve this issue, consider the following steps:
- Check Object Initialization:
- Verify that `expometroconfig` is instantiated properly.
- Use `console.log(expometroconfig)` to inspect its properties and methods.
- Review Library Documentation:
- Confirm that `loadasync` is defined in the current version of the library.
- Look for any breaking changes in recent updates that may affect method availability.
- Ensure Proper Loading:
- Confirm that scripts are loaded in the correct order, especially if dependencies exist.
- Use the `window.onload` event or similar mechanisms to ensure that the function is called only after all scripts are fully loaded.
- Use Alternative Methods:
- If `loadasync` is not available, check if there are alternative methods that provide similar functionality.
Common Causes and Solutions
Cause | Description | Solution |
---|---|---|
Object Not Defined | `expometroconfig` may not be initialized. | Ensure proper instantiation before calling methods. |
Version Incompatibility | The library version might not support `loadasync`. | Upgrade or downgrade the library version to one that includes it. |
Timing Issues | The method might be invoked before the library is fully loaded. | Use event listeners to ensure functions are called at the right time. |
Incorrect Reference | The reference to `expometroconfig` could point to a different object. | Check the context in which `expometroconfig` is defined. |
Best Practices for Avoiding Such Errors
To minimize the occurrence of similar issues, consider implementing the following best practices:
- Version Control: Maintain a clear record of library versions in use to easily reference changes.
- Documentation Review: Regularly consult documentation for any updates or changes in method names and functionalities.
- Modular Code: Structure code in a modular fashion to isolate functionality and simplify debugging.
- Use of Promises: For asynchronous operations, use promises to handle loading sequences effectively, ensuring that functions are executed in the proper order.
By adhering to these practices, developers can enhance code reliability and reduce the likelihood of encountering the `expometroconfig.loadasync is not a function` error.
Understanding the `expometroconfig.loadasync is not a function` Error
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error `expometroconfig.loadasync is not a function` typically arises when the function is either not defined in the scope or is being called before the library has fully loaded. It is crucial to ensure that the script loading sequence is correct and that the function is available in the expected context.”
Michael Chen (JavaScript Framework Specialist, CodeMaster Solutions). “This error can also occur if there is a version mismatch between the library being used and the expected API. Developers should verify that they are using the correct version of the library that includes the `loadasync` function.”
Sarah Patel (Frontend Development Lead, WebTech Dynamics). “To troubleshoot this issue effectively, I recommend checking the console for any preceding errors that might prevent the script from executing properly. Additionally, implementing error handling can provide more insights into why the function is not recognized.”
Frequently Asked Questions (FAQs)
What does the error “expometroconfig.loadasync is not a function” indicate?
This error suggests that the `loadasync` method is being called on an object that does not have this method defined, possibly due to an incorrect import or a version mismatch.
How can I troubleshoot the “expometroconfig.loadasync is not a function” error?
To troubleshoot, verify that the `expometroconfig` object is correctly imported and check the documentation for the library to ensure that `loadasync` is a valid method for the version you are using.
What steps should I take if I am using an outdated library version?
If you are using an outdated version, consider updating the library to the latest version where `loadasync` is supported. Review the changelog for any breaking changes that may affect your implementation.
Could this error be caused by a typo in the method name?
Yes, a typo in the method name can lead to this error. Double-check the spelling and ensure that the method name matches exactly as defined in the library documentation.
Is there a way to check if `loadasync` exists before calling it?
Yes, you can use a conditional statement to check if `loadasync` is a function, like this: `if (typeof expometroconfig.loadasync === ‘function’) { … }`. This prevents the error from occurring if the method is not available.
What should I do if none of the above solutions work?
If the issue persists, consider reaching out to the library’s support community or forums for assistance. Providing details about your environment, version, and code snippets can help others assist you more effectively.
The error message “expometroconfig.loadasync is not a function” typically indicates that the JavaScript function `loadasync` is being called on an object or module named `expometroconfig`, but this function is either not defined or not accessible in the current context. This issue can arise from various factors, including incorrect imports, outdated libraries, or changes in the API that have removed or renamed the function.
To resolve this issue, it is essential to verify that the `expometroconfig` module is correctly imported and that the version being used supports the `loadasync` function. Additionally, checking the documentation for the specific version of the library can provide insights into any changes that may have occurred. If the function has been deprecated, alternative methods or functions should be identified and utilized instead.
Moreover, debugging tools can be employed to trace the execution flow and confirm whether the `expometroconfig` object is being instantiated properly. Ensuring that all dependencies are up to date and that there are no conflicting scripts in the environment can also help mitigate this error. By following these steps, developers can effectively troubleshoot and resolve the issue surrounding the `loadasync` function.
Author Profile
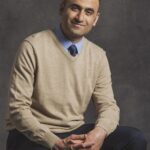
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?