Understanding the Error: What Does ‘Expression Must Be a Modifiable Lvalue’ Mean?
In the realm of programming, encountering errors can often feel like navigating a labyrinth. Among these, the phrase “expression must be a modifiable lvalue” stands out as a common yet perplexing obstacle for developers. This seemingly cryptic message can halt your code in its tracks, leaving you puzzled about what went wrong. Understanding this error is crucial for anyone looking to refine their coding skills and enhance their debugging prowess. In this article, we will unravel the intricacies of modifiable lvalues, explore their significance in programming languages, and equip you with the knowledge to tackle this error head-on.
Overview
At its core, the term “modifiable lvalue” refers to an expression that can be assigned a new value. In programming, not all expressions are created equal; some can be modified, while others are immutable. This distinction is vital for understanding how variables and memory work within a program. When you encounter the error message indicating that an expression must be a modifiable lvalue, it typically signifies that you are attempting to assign a value to something that cannot be changed—such as a constant or a temporary result.
Navigating this error requires a solid grasp of the underlying principles of variable assignment and memory management. By delving into the reasons behind the error
Understanding Modifiable Lvalues
In programming, particularly in languages like C, C++, and others, the term “lvalue” refers to an expression that points to a memory location and allows for modification. An expression must be a modifiable lvalue when the programmer intends to assign a value to it. If the expression isn’t modifiable, the compiler will throw an error indicating that it is not a valid target for assignment.
Common Causes of the Error
The error “expression must be a modifiable lvalue” typically occurs in the following scenarios:
- Using Constants: Attempting to assign a value to a constant or literal. For example:
“`c
5 = x; // Incorrect
“`
- Dereferencing a Non-modifiable Pointer: Trying to modify the value pointed to by a pointer that is declared as `const`.
“`c
const int *ptr = &value;
*ptr = 10; // Incorrect
“`
- Using Temporary Objects: Assigning a value to a temporary object or a result of an expression that does not have a stable memory address.
“`c
(x + y) = 10; // Incorrect
“`
- Function Return Values: Attempting to assign a value to a return from a function that returns a value by value rather than by reference.
“`c
int foo() { return 5; }
foo() = 10; // Incorrect
“`
Examples of Modifiable Lvalues
To clarify the concept further, here are examples of valid modifiable lvalues:
- Variables: Standard variable assignments are valid since they are modifiable lvalues.
“`c
int x;
x = 10; // Correct
“`
- Array Elements: Individual elements of an array can be modified.
“`c
int arr[5];
arr[0] = 1; // Correct
“`
- Dereferencing Pointers: Pointers can be dereferenced to modify the value at the pointed location, provided they are not const.
“`c
int a = 5;
int *p = &a;
*p = 10; // Correct
“`
Resolving the Error
To resolve the “expression must be a modifiable lvalue” error, consider the following approaches:
- Check for Constants: Ensure that the target of the assignment is not a constant or literal.
- Review Pointer Declarations: Confirm that pointers used for modification are not declared as `const`.
- Use References: If working in C++, consider returning by reference to allow modification.
- Re-evaluate Expressions: Ensure the target of the assignment is a valid variable, array element, or dereferenced pointer.
Expression Type | Modifiable | Example |
---|---|---|
Variable | Yes | x = 5; |
Constant | No | 5 = x; |
Array Element | Yes | arr[0] = 10; |
Function Return | No (by value) | foo() = 10; |
Understanding Modifiable Lvalues
In programming, particularly in languages like C and C++, an expression must be a modifiable lvalue when the context requires an assignable target. An lvalue (locator value) refers to an object that occupies some identifiable location in memory (i.e., it has an address). In contrast, an rvalue (read value) is a temporary value that does not have a persistent address.
Examples of Modifiable Lvalues
- Valid Modifiable Lvalues:
- Variables: `int a; a = 10;`
- Array elements: `arr[0] = 5;`
- Dereferenced pointers: `*ptr = 20;`
- Invalid Modifiable Lvalues:
- Constants: `const int b = 15; b = 20;` (Error)
- Temporary results: `(a + b) = 10;` (Error)
- Function calls that return rvalues: `getValue() = 10;` (Error)
Common Scenarios Leading to Errors
The error “expression must be a modifiable lvalue” typically arises in the following situations:
- Attempting to assign a value to a constant or immutable variable.
- Trying to assign a value to the result of an expression instead of a variable.
- Using function calls that return values directly in assignment statements.
Code Snippets Illustrating Errors
“`c
// Example of an error due to assigning to a constant
const int max = 100;
max = 200; // Error: expression must be a modifiable lvalue
// Example of an error due to assigning to an expression
int x = 5, y = 10;
(x + y) = 15; // Error: expression must be a modifiable lvalue
// Example of an error with a function returning an rvalue
int getValue() { return 42; }
getValue() = 10; // Error: expression must be a modifiable lvalue
“`
Resolution Strategies
To resolve this error, consider the following strategies:
- Ensure that you are assigning values to variables that are not declared as `const`.
- When working with expressions, store the result in a variable before attempting to modify it.
- Verify that function calls used in assignment statements return references or pointers if modification is intended.
Summary of Key Points
Aspect | Modifiable Lvalue | Non-modifiable Lvalue |
---|---|---|
Definition | Can be assigned a value | Cannot be assigned a value |
Examples | Variables, array elements | Constants, temporary results |
Common Errors | Assigning to constants | Assigning to expressions |
Resolution Strategies | Use variables for assignment | Store results before modifying |
By adhering to these guidelines and understanding the nature of lvalues and rvalues, you can effectively avoid the pitfalls that lead to the “expression must be a modifiable lvalue” error in your coding practices.
Understanding the Concept of Modifiable Lvalues in Programming
Dr. Emily Carter (Senior Software Engineer, CodeCraft Solutions). “The term ‘modifiable lvalue’ refers to an expression that can be assigned a new value. In programming languages like C and C++, understanding this concept is crucial as it dictates how variables can be manipulated and ensures that only valid expressions are assigned new values.”
Michael Chen (Lead Compiler Developer, Tech Innovations Inc.). “When a compiler encounters an expression that must be a modifiable lvalue, it checks whether the expression can indeed be modified. This is essential for maintaining the integrity of data and preventing runtime errors, particularly in languages that enforce strict type checking.”
Sarah Thompson (Professor of Computer Science, University of Techland). “In many programming paradigms, the distinction between lvalues and rvalues is fundamental. A modifiable lvalue allows for direct manipulation of memory locations, which is not only efficient but also necessary for implementing certain algorithms and data structures effectively.”
Frequently Asked Questions (FAQs)
What does “expression must be a modifiable lvalue” mean?
This error indicates that the expression you are trying to modify is not a modifiable lvalue. An lvalue refers to an object that occupies identifiable location in memory (i.e., it can be assigned a value), while an rvalue is a temporary value that does not have a persistent memory location.
When does this error typically occur in programming?
This error commonly occurs when attempting to assign a value to a constant, literal, or an expression that does not represent a variable. For example, trying to modify a literal like `5 = x` will trigger this error.
How can I resolve the “expression must be a modifiable lvalue” error?
To resolve this error, ensure that you are assigning values to valid variables or objects that can be modified. Check your code for any constants or expressions that should be variables instead.
Are there specific programming languages where this error is more prevalent?
This error is particularly common in languages like C, C++, and Java, where the distinction between lvalues and rvalues is critical for memory management and variable assignment.
Can you provide an example of code that triggers this error?
Certainly. The following code will trigger this error:
“`c
int main() {
int x;
10 = x; // Error: 10 is not a modifiable lvalue
return 0;
}
“`
What should I consider when designing functions to avoid this error?
When designing functions, ensure that parameters intended to be modified are passed by reference or pointer, allowing the function to modify the original variable rather than a copy. This practice helps avoid situations where non-modifiable lvalues are mistakenly used.
The phrase “expression must be a modifiable lvalue” typically arises in programming languages such as C and C++. It indicates that an expression must refer to a location in memory that can be modified. In programming, an lvalue (locator value) represents an object that occupies identifiable location in memory, allowing it to be assigned a new value. Conversely, an rvalue (read value) is an expression that does not have a persistent memory location and cannot be assigned a new value. Understanding the distinction between lvalues and rvalues is crucial for writing correct and efficient code.
When a programmer encounters an error stating that an expression must be a modifiable lvalue, it often signifies that they are attempting to assign a value to something that cannot be modified. Common examples include attempting to assign a value to a literal constant or the result of a function that returns an rvalue. This error emphasizes the importance of ensuring that the target of an assignment is indeed a modifiable lvalue, which can lead to more robust and error-free code.
Key takeaways from this discussion include the necessity of understanding the rules governing lvalues and rvalues in programming languages. Developers should be mindful of the contexts in which they are working with expressions, particularly when
Author Profile
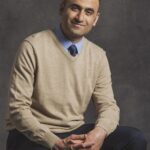
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?