Why Can’t I Convert ‘java.lang.String’ to JSONObject in Java?
In the world of Java programming, developers often encounter various data types and structures that can pose challenges during application development. One such challenge arises when trying to convert a `String` to a `JSONObject`. This seemingly straightforward task can lead to frustrating errors, particularly the infamous “type java.lang.String cannot be converted to JSONObject.” Understanding the nuances of data types and the intricacies of JSON handling is essential for any Java developer looking to create robust applications. In this article, we will explore the common pitfalls associated with this conversion and provide insights into best practices that can help you navigate these challenges with ease.
When working with JSON in Java, developers frequently utilize libraries such as org.json or Gson to parse and manipulate data. However, the process of converting a `String` representation of JSON into a `JSONObject` is not always as seamless as it appears. One of the primary reasons for conversion errors stems from improperly formatted JSON strings. A valid JSON string must adhere to specific syntax rules, and even a minor deviation can trigger runtime exceptions that halt your application. Understanding these formatting requirements is crucial for successful data manipulation.
Moreover, the error message “type java.lang.String cannot be converted to JSONObject” serves as a reminder of the importance of type compatibility in Java. This error often indicates
Understanding JSON and Java String Conversion
When working with JSON in Java, particularly with libraries like `org.json` or `Gson`, a common issue developers face is the inability to convert a Java `String` directly into a `JSONObject`. This can lead to the error message indicating that a particular type cannot be converted to `JSONObject`.
A `String` in Java represents a sequence of characters, while a `JSONObject` is a structure that holds key-value pairs in a specific format. If a string does not represent a valid JSON object, attempting to convert it directly will result in an exception.
Common Causes of the Conversion Error
Several factors can lead to this conversion issue:
- Invalid JSON Format: The string must be formatted correctly as JSON. For instance, it should have curly braces `{}` for objects and square brackets `[]` for arrays.
- Malformed Data: Missing quotes, commas, or brackets can cause a string to be interpreted as invalid JSON.
- Type Mismatch: Attempting to cast a primitive type or a non-JSON compatible object directly into a `JSONObject`.
Example of Valid vs. Invalid JSON Strings
To clarify, here are examples of valid and invalid JSON strings:
Type | Example | Status |
---|---|---|
Valid JSON | {“name”: “John”, “age”: 30} | Can be converted |
Invalid JSON | {“name”: “John”, “age”:} | Cannot be converted |
Invalid JSON | “name”: “John” | Cannot be converted |
How to Convert a String to JSONObject
To successfully convert a valid JSON string to a `JSONObject`, the following steps can be taken:
- Ensure Valid JSON Format: Validate the string to confirm it is well-formed.
- Use JSON Libraries: Utilize libraries like `org.json` or `Gson` to perform the conversion.
Here’s how you can implement this in Java:
“`java
import org.json.JSONObject;
public class JsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”: \”John\”, \”age\”: 30}”;
// Check if the string is a valid JSON
try {
JSONObject jsonObject = new JSONObject(jsonString);
System.out.println(jsonObject);
} catch (Exception e) {
System.out.println(“Error: ” + e.getMessage());
}
}
}
“`
In this example, if `jsonString` is formatted correctly, it will convert without issues. If the string is malformed, the exception will be caught, and an error message will be printed.
Best Practices for JSON Handling in Java
To avoid conversion issues, consider following these best practices:
- Validate JSON Before Conversion: Use online tools or libraries to check if the JSON format is correct.
- Use Try-Catch Blocks: Implement error handling to manage exceptions gracefully.
- Log Errors: Maintain logs for JSON conversion errors to debug issues effectively.
By adhering to these guidelines, developers can minimize the risk of encountering conversion errors and enhance the robustness of their applications.
Understanding the Error
The error message “type java.lang.String cannot be converted to JSONObject” typically arises when a String variable is expected to be a JSON object. This often occurs in Java applications that manipulate JSON data using libraries like org.json or Gson.
Common scenarios leading to this error include:
- Attempting to parse a plain string that is not in JSON format.
- Passing a string directly to a method that expects a JSONObject or similar data structure.
- Misconfiguration or misunderstanding of the expected input type in API calls.
Common Causes
Several factors can contribute to this error:
- Invalid JSON Format: The string might not be properly formatted as JSON. For example:
- A missing opening or closing brace.
- Incorrect quotation marks or unescaped characters.
- Incorrect Method Usage: Invoking a method that requires a JSONObject but providing a String instead.
- Data Type Mismatch: Misalignments between expected data types in method signatures and actual types provided.
How to Resolve the Issue
To address the error, consider the following approaches:
- Validate the JSON String: Ensure that the string being parsed is valid JSON. You can use online tools or libraries to validate the format.
- Convert String to JSONObject: Use appropriate methods to convert the string into a JSONObject. For example, using org.json:
“`java
String jsonString = “{\”key\”:\”value\”}”;
JSONObject jsonObject = new JSONObject(jsonString);
“`
- Check API Documentation: Ensure that the method you are calling is intended to accept a JSONObject. Refer to the library documentation for correct usage.
Example Code Snippet
Here’s a simple example demonstrating correct conversion of a String to a JSONObject:
“`java
import org.json.JSONObject;
public class JsonExample {
public static void main(String[] args) {
String jsonString = “{\”name\”:\”John\”, \”age\”:30}”;
try {
// Creating a JSONObject from the string
JSONObject jsonObject = new JSONObject(jsonString);
System.out.println(“Name: ” + jsonObject.getString(“name”));
System.out.println(“Age: ” + jsonObject.getInt(“age”));
} catch (Exception e) {
System.out.println(“Error: ” + e.getMessage());
}
}
}
“`
Additional Considerations
When working with JSON in Java, keep the following in mind:
- Library Selection: Choose the right library for your needs. Common libraries include:
- org.json
- Gson
- Jackson
- Error Handling: Implement robust error handling to manage JSON parsing exceptions effectively.
- Performance Implications: Be aware of the performance impacts of parsing large JSON strings and consider streaming options if necessary.
- Data Structure Awareness: Understand the structure of the JSON being parsed to avoid runtime exceptions related to missing keys or incorrect types.
By following these guidelines, developers can effectively address the “type java.lang.String cannot be converted to JSONObject” error, leading to smoother JSON data handling in their Java applications.
Understanding JSON Conversion Issues in Java
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Corp). “The error ‘f type java.lang.string cannot be converted to jsonobject’ typically arises when attempting to parse a string that does not conform to the expected JSON format. It is crucial to ensure that the string being converted is a valid JSON object, which requires proper syntax including braces, quotes, and key-value pairs.”
Michael Chen (Lead Java Developer, CodeCraft Solutions). “In Java, when you encounter this error, it often indicates that the string you are trying to convert is either malformed or not a JSON object at all. Debugging the string content before conversion can help identify if it is a valid JSON format or if it needs to be constructed correctly.”
Sarah Thompson (Java Programming Instructor, University of Technology). “To resolve the ‘f type java.lang.string cannot be converted to jsonobject’ error, developers should utilize libraries like Gson or Jackson which provide robust methods for parsing strings into JSON objects. Additionally, validating the JSON structure using online validators can save time and prevent common mistakes.”
Frequently Asked Questions (FAQs)
What does the error “f type java.lang.string cannot be converted to jsonobject” mean?
This error indicates that a string is being passed where a JSONObject is expected. The JSON library cannot convert a plain string into a JSON object without proper formatting.
How can I resolve the “f type java.lang.string cannot be converted to jsonobject” error?
To resolve this error, ensure that you are passing a valid JSON string to the method expecting a JSONObject. The string should be properly formatted as JSON, such as `{“key”:”value”}`.
What are common causes of this error in Java applications?
Common causes include attempting to parse a string that does not represent a valid JSON object, or mistakenly passing a string variable instead of a JSONObject instance to a method.
Can this error occur due to incorrect JSON formatting?
Yes, incorrect JSON formatting can lead to this error. Ensure that the JSON string adheres to the correct syntax, including proper use of braces, quotes, and commas.
How can I check if a string is a valid JSON before converting it to a JSONObject?
You can use a JSON parsing library, such as `org.json`, to attempt parsing the string. If parsing fails, it indicates that the string is not valid JSON.
Is there a way to debug this issue effectively?
Debugging can be done by logging the string being passed and checking its format. Additionally, using try-catch blocks around the parsing code can help identify the exact point of failure.
The error message “f type java.lang.String cannot be converted to JSONObject” typically arises in Java applications when there is an attempt to convert a string that does not conform to the JSON format into a JSONObject. This situation often occurs when developers mistakenly assume that a plain string can be processed as JSON without proper parsing or validation. Understanding the nature of JSON and the requirements for its structure is crucial for resolving this issue.
To successfully convert a string to a JSONObject, the string must be a valid JSON representation. This means it should be properly formatted, including correct use of braces, brackets, and key-value pairs. If the string is not formatted correctly, the conversion will fail, resulting in the aforementioned error. Developers should utilize libraries such as org.json or Gson, which provide methods to parse strings into JSON objects, ensuring that the input is validated before conversion.
Key takeaways from this discussion include the importance of validating input data before attempting to convert it into a JSONObject. Developers should implement error handling to catch exceptions that may arise during conversion attempts. Additionally, familiarizing oneself with JSON structure and utilizing robust libraries can significantly reduce the likelihood of encountering conversion errors. Adopting these best practices will enhance the reliability and stability of Java applications that interact with
Author Profile
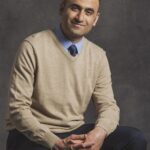
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?