Why Am I Seeing ‘Failed to Execute ‘postMessage’ on ‘Worker’:?’ and How Can I Fix It?
In the fast-paced world of web development, the seamless interaction between various components is crucial for creating responsive and efficient applications. However, as developers push the boundaries of what is possible, they often encounter a range of challenges that can disrupt the flow of their projects. One such challenge is the error message: “failed to execute ‘postMessage’ on ‘Worker’.” This seemingly cryptic notification can cause confusion and frustration, particularly for those who are new to the intricacies of web workers and message passing. In this article, we will delve into the nuances of this error, exploring its causes, implications, and potential solutions to help you navigate this common pitfall in modern web programming.
Understanding the “failed to execute ‘postMessage’ on ‘Worker'” error requires a grasp of the underlying architecture of web workers and the message-passing mechanism that facilitates communication between threads. Web workers allow developers to run scripts in background threads, enabling the execution of complex tasks without blocking the main user interface. However, when the postMessage method fails, it can stem from various issues such as incorrect data types, security restrictions, or improper worker initialization. By dissecting these factors, developers can gain insight into how to effectively troubleshoot and resolve this error.
As we explore the intricacies
Understanding the PostMessage API
The PostMessage API is a powerful tool that facilitates communication between different browsing contexts, such as iframes and web workers. It allows scripts to send messages to each other, which is particularly useful in web applications that require coordination between multiple components. However, improper use of this API can lead to errors, such as the “failed to execute ‘postMessage’ on ‘Worker'” message.
Key aspects of the PostMessage API include:
- Cross-Origin Communication: The API allows secure messaging across different origins, helping to overcome the limitations imposed by the same-origin policy.
- Event Handling: Messages are received via the `message` event, which must be appropriately handled to ensure the correct processing of received data.
- Data Types: The API supports various data types, including strings, objects, and arrays, enabling versatile communication between contexts.
Common Causes of PostMessage Errors
The “failed to execute ‘postMessage’ on ‘Worker'” error can arise due to several reasons. Understanding these causes can help developers troubleshoot and resolve issues effectively.
- Invalid Target Origin: When sending a message, specifying an incorrect or target origin can lead to this error. The target origin must be an exact match or a wildcard for the message to be sent successfully.
- Closed Worker: If a message is sent to a web worker that has already been terminated, the PostMessage call will fail. This often occurs when the application logic does not correctly manage the worker’s lifecycle.
- Unsupported Data Types: Attempting to send unsupported data types, such as circular references or functions, can trigger an error. The PostMessage API only supports structured cloneable objects.
Error Cause | Description | Resolution |
---|---|---|
Invalid Target Origin | Specified target does not match the receiving context’s origin. | Verify and correct the target origin. |
Closed Worker | Message sent to a terminated worker. | Ensure the worker is active before sending messages. |
Unsupported Data Types | Attempting to send non-cloneable data. | Use structured cloneable objects only. |
Best Practices for Using PostMessage
To ensure reliable communication through the PostMessage API, developers should adhere to several best practices:
- Validate Input: Always validate the data being sent through PostMessage to prevent errors related to unsupported data types.
- Use a Message Protocol: Establish a clear protocol for message types and structures. This helps in maintaining clarity and consistency in communication.
- Error Handling: Implement robust error handling in both the sender and receiver to gracefully manage any issues that arise during message transmission.
- Lifecycle Management: Properly manage the lifecycle of web workers to avoid sending messages to terminated workers. This includes cleaning up and terminating workers when they are no longer needed.
By following these guidelines, developers can mitigate the risk of encountering errors and enhance the reliability of their applications that leverage the PostMessage API.
Understanding the Error
The error message “failed to execute ‘postMessage’ on ‘Worker'” typically arises in JavaScript when attempting to send messages to a Web Worker. This can be due to several factors related to the data being passed or the context of the worker.
Common Causes
Several key issues can lead to this error:
- Invalid Data Type: The postMessage method only accepts certain data types. If you attempt to pass an unsupported type, such as a function or a DOM element, you will encounter this error.
- Cross-Origin Restrictions: Workers have strict cross-origin policies. If you try to send a message from a different origin without proper CORS settings, it will fail.
- Worker Not Initialized: If the worker has not been properly initialized or is terminated, any attempt to send messages will result in this error.
- Message Length Exceeded: There are limitations on the size of the messages being sent. Exceeding these limits can lead to failure in execution.
Data Types Supported by postMessage
When using the postMessage method, ensure that the data types being passed conform to the following list:
Data Type | Description |
---|---|
Primitive Types | Strings, numbers, booleans |
Structured Clones | Arrays, objects (not containing functions or DOM elements) |
Transferable Objects | ArrayBuffers, MessagePorts |
Best Practices for Using postMessage
To avoid encountering the “failed to execute ‘postMessage'” error, consider the following best practices:
- Validate Data Before Sending: Always check the data type before calling postMessage. Use JavaScript’s `typeof` operator for primitive types and `Array.isArray()` for arrays.
- Use Structured Cloning: Ensure that only data that can be structured cloned is sent. Avoid sending complex types like functions or DOM nodes.
- Error Handling: Implement try-catch blocks around your postMessage calls to gracefully handle any exceptions that may arise.
- Cross-Origin Handling: If you are working with multiple origins, ensure that your server is configured to handle CORS properly.
Debugging Steps
If you encounter the error, follow these debugging steps:
- Check Worker Initialization: Ensure that the worker is correctly instantiated and running.
- Inspect Message Data: Log the data you are attempting to send to verify that it conforms to the supported types.
- Review Console Logs: Look for additional error messages in the console that may provide more context about the failure.
- Test in Different Browsers: Occasionally, browser-specific issues can affect worker behavior. Testing across multiple environments can help identify such problems.
Example Code Snippet
Here is an example demonstrating proper use of postMessage in a Web Worker context:
“`javascript
// main.js
const worker = new Worker(‘worker.js’);
worker.onmessage = function(event) {
console.log(‘Message from worker:’, event.data);
};
const messageData = { type: ‘greeting’, content: ‘Hello, Worker!’ };
try {
worker.postMessage(messageData); // Ensure messageData is a valid structured clone
} catch (error) {
console.error(‘Failed to post message:’, error);
}
// worker.js
self.onmessage = function(event) {
console.log(‘Message received from main script:’, event.data);
self.postMessage(‘Message processed’);
};
“`
Understanding the context and constraints of Web Workers and postMessage is crucial for effective web application development. By adhering to best practices and thoroughly debugging, you can mitigate issues associated with this error.
Understanding the ‘postMessage’ Error in Web Workers
Dr. Emily Carter (Web Development Specialist, Tech Innovations Inc.). “The error ‘failed to execute ‘postMessage’ on ‘worker” typically arises when there is an issue with the message format or the worker’s state. Developers must ensure that the data being sent is serializable and that the worker has been properly initialized before sending messages.”
Mark Thompson (Senior Software Engineer, Cloud Solutions Group). “This error can also occur if the worker script is not correctly loaded or if there are cross-origin restrictions in place. It is crucial to verify that the worker’s URL is accessible and adheres to the same-origin policy to prevent such issues.”
Lisa Chen (Front-End Architect, Digital Experience Agency). “In my experience, debugging this error involves checking the browser’s console for additional error messages. Often, the root cause can be traced back to a misconfiguration in the web worker setup or improper handling of asynchronous operations.”
Frequently Asked Questions (FAQs)
What does the error “failed to execute ‘postmessage’ on ‘worker'” mean?
This error indicates that a message could not be sent to a Web Worker using the `postMessage` method, often due to issues such as incorrect target origin or the worker being terminated.
What are common causes of the “failed to execute ‘postmessage’ on ‘worker'” error?
Common causes include attempting to post a message to a worker that is not active, using an unsupported data type, or passing an incorrect reference to the worker.
How can I troubleshoot the “failed to execute ‘postmessage’ on ‘worker'” error?
To troubleshoot, check if the worker is properly instantiated and running. Ensure that the message being sent is serializable and that the correct target origin is specified.
Can this error occur in all browsers?
Yes, this error can occur in any modern browser that supports Web Workers. However, the specifics may vary slightly depending on the browser’s implementation.
Is there a way to prevent the “failed to execute ‘postmessage’ on ‘worker'” error?
To prevent this error, ensure that you validate the worker’s state before sending messages, use appropriate data types, and handle any potential exceptions during message passing.
What should I do if the error persists despite troubleshooting?
If the error persists, consider reviewing the worker’s lifecycle management in your code, checking for any potential memory issues, or consulting the browser’s developer console for more detailed error logs.
The error message “failed to execute ‘postMessage’ on ‘Worker'” typically arises in web development when there is an issue with the communication between the main thread and a web worker. This error indicates that the message being sent is either malformed or that the worker is not in a state to receive messages. Understanding the context in which this error occurs is crucial for developers aiming to implement effective multithreading in their applications.
One of the primary reasons for encountering this error is the violation of the structured clone algorithm, which is used to serialize messages sent to web workers. If the data being sent includes non-serializable objects, such as DOM elements or functions, the postMessage call will fail. It is essential for developers to ensure that only valid data types, such as strings, numbers, and objects that can be cloned, are sent to the worker.
Another common cause is attempting to send messages to a worker that has already terminated or is in the process of being terminated. Developers should implement proper checks to ensure that the worker is alive and ready to receive messages before attempting to communicate. Additionally, error handling mechanisms should be in place to gracefully manage such failures and provide meaningful feedback to the user or developer.
In summary
Author Profile
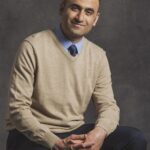
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?