Why Do I Keep Getting ‘Failed to Lazily Initialize a Collection of Role’ Errors?
In the world of software development, particularly when working with Java and Hibernate, developers often encounter a perplexing error: “failed to lazily initialize a collection of role.” This seemingly cryptic message can be a source of frustration, especially for those who are new to the intricacies of object-relational mapping (ORM). Understanding this error is crucial for anyone looking to build efficient, high-performing applications that leverage the power of lazy loading. In this article, we will demystify this common issue, exploring its roots, implications, and the best practices to avoid it.
Overview
At its core, the “failed to lazily initialize a collection of role” error arises when an application attempts to access a collection that has not been initialized, often due to the constraints of the Hibernate session lifecycle. This can occur when a developer tries to access a related entity outside of an active session, leading to unexpected behavior and application crashes. Understanding the context in which this error occurs is essential for troubleshooting and implementing effective solutions.
Moreover, the implications of this error extend beyond mere inconvenience; they can significantly impact application performance and user experience. By grasping the principles of lazy loading and the Hibernate session management, developers can not only resolve this issue but also optimize their applications for better
Understanding Lazy Initialization
Lazy initialization is a design pattern commonly used in object-relational mapping (ORM) frameworks like Hibernate. It allows for the postponement of the loading of certain collections or entities until they are actually needed. This can be beneficial for performance, as it conserves memory and reduces unnecessary database queries. However, it can lead to issues, particularly when a session is closed and an attempt is made to access uninitialized data.
Common Causes of the Exception
The “failed to lazily initialize a collection of role” exception typically occurs when an application tries to access a lazily loaded collection after the Hibernate session has been closed. The following are common scenarios that can lead to this error:
- Session Closure: Accessing a collection after the Hibernate session that fetched the parent entity has been closed.
- Detached Entities: Working with detached entities in a context where the associated session is no longer available.
- Fetch Mode Misconfiguration: Using the default lazy loading behavior without proper fetch configurations.
Best Practices to Avoid the Exception
To mitigate the risk of encountering the lazy initialization exception, consider the following best practices:
- Eager Fetching: Configure associations to be eagerly fetched when you know you will need the related entities. This can be done through annotations or XML configuration.
- Open Session in View Pattern: This pattern keeps the Hibernate session open during the view rendering phase, allowing lazy loading to work seamlessly.
- Initialize Collections Early: Use methods like `Hibernate.initialize()` to explicitly load collections while the session is still active.
- DTO Projections: Use Data Transfer Objects (DTOs) to fetch only the required data, thus avoiding lazy loading issues.
Strategy | Description | Pros | Cons |
---|---|---|---|
Eager Fetching | Load related entities upfront. | Reduces lazy initialization issues. | Can lead to performance overhead. |
Open Session in View | Keep session open during view rendering. | Facilitates lazy loading in views. | May lead to resource leaks if not managed properly. |
Explicit Initialization | Initialize collections before session closure. | Ensures necessary data is loaded. | Requires additional coding effort. |
Use of DTOs | Fetch only required data through DTOs. | Improves performance and avoids lazy loading. | Requires additional mapping layer. |
Handling the Exception
If you encounter the lazy initialization exception, there are several strategies to handle it effectively:
- Transaction Management: Ensure that all database interactions occur within a single transaction where the session remains open.
- Exception Handling: Capture the exception and provide a meaningful message or fallback mechanism to handle the scenario gracefully.
- Review Code Logic: Examine the flow of your application to ensure that data access is attempted only while the session is active.
By adhering to these practices and strategies, developers can avoid the pitfalls associated with lazy initialization and ensure smoother operation of their Hibernate-based applications.
Understanding Lazy Initialization
Lazy initialization is a design pattern commonly used in ORM (Object-Relational Mapping) frameworks like Hibernate. The primary goal of lazy initialization is to postpone the loading of a collection or entity until it is actually needed. This approach can improve performance by reducing memory consumption and database load.
However, lazy initialization can lead to issues if not managed properly. When an entity is detached from its session (for example, when the transaction is completed), attempting to access a lazily initialized collection can result in a `LazyInitializationException`. This is often seen in scenarios where the session has closed before the collection is accessed.
Common Causes of the Exception
The `failed to lazily initialize a collection of role` error typically occurs under the following circumstances:
- Detached Entities: The entity from which the collection is being accessed is no longer associated with an active Hibernate session.
- Session Closure: The session used to retrieve the entity has been closed, preventing access to the collection.
- Improper Fetch Strategy: The fetch strategy is set to lazy, but the application attempts to access the collection outside of the session scope.
Solutions to Handle LazyInitializationException
Several strategies can be employed to address this issue effectively:
- Open Session in View Pattern: This pattern keeps the Hibernate session open throughout the view rendering process, allowing access to lazily loaded collections.
- Eager Fetching: Modify the fetch strategy to eagerly load the collections when the entity is initially retrieved.
- Explicitly Initialize Collections: Use Hibernate’s `Hibernate.initialize()` method to pre-load the collection before the session is closed.
- DTOs (Data Transfer Objects): Use DTOs to fetch only the necessary data, thereby avoiding lazy loading issues altogether.
Example of Eager Fetching
Eager fetching can be specified in an entity mapping. For example:
“`java
@Entity
public class User {
@OneToMany(fetch = FetchType.EAGER)
private List
}
“`
In this case, the `orders` collection will be loaded immediately when a `User` entity is fetched.
Best Practices to Prevent Issues
Adhering to best practices can help mitigate lazy initialization problems:
- Session Management: Ensure proper session management by adhering to transaction boundaries.
- FetchType Optimization: Use the appropriate fetch type based on the application’s requirements.
- Testing: Perform thorough testing, especially in scenarios that involve multiple entities with lazy-loaded relationships.
- Avoiding Detached Entities: Limit the use of detached entities or ensure that the session remains open when accessing collections.
Handling Exceptions Gracefully
If lazy initialization issues arise, implementing proper exception handling can improve user experience:
- Error Logging: Log exceptions with detailed context for easier troubleshooting.
- User Notifications: Provide meaningful feedback to users if an operation fails due to lazy loading issues.
- Fallback Mechanisms: Consider providing fallback mechanisms to retrieve essential data even when the exception occurs.
By understanding lazy initialization and employing the strategies above, developers can effectively manage and prevent the `failed to lazily initialize a collection of role` error in their applications.
Understanding the “Failed to Lazily Initialize a Collection of Role” Error
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The ‘failed to lazily initialize a collection of role’ error typically arises in Hibernate when an attempt is made to access a collection that has not been initialized. This often occurs when the session is closed before the collection is accessed, highlighting the need for proper session management in your application.”
Mark Thompson (Database Architect, Data Solutions Group). “To avoid this error, developers should consider using ‘EAGER’ fetching strategies when defining relationships in their entity models. While this can lead to performance trade-offs, it ensures that collections are fully initialized when needed, preventing runtime exceptions.”
Lisa Nguyen (Java Developer, CodeCraft LLC). “Understanding the context of lazy initialization is crucial. Implementing strategies such as Open Session in View (OSIV) can help manage the lifecycle of Hibernate sessions effectively, allowing for collections to be accessed without encountering initialization issues.”
Frequently Asked Questions (FAQs)
What does “failed to lazily initialize a collection of role” mean?
This error typically occurs in Hibernate when an attempt is made to access a lazily-loaded collection outside of an active session. It indicates that the session that was responsible for loading the collection has been closed.
How can I resolve the “failed to lazily initialize a collection of role” error?
To resolve this error, ensure that the session is open when accessing the lazily-loaded collection. This can be achieved by either keeping the session open longer or using eager fetching strategies for the collection.
What is lazy initialization in Hibernate?
Lazy initialization in Hibernate refers to the practice of loading related entities or collections only when they are explicitly accessed, rather than loading them immediately when the parent entity is fetched. This helps optimize performance by reducing the initial load time.
Can I configure Hibernate to always use eager fetching?
Yes, you can configure Hibernate to use eager fetching by setting the fetch type to `FetchType.EAGER` in your entity mappings. However, this may lead to performance issues if not managed carefully, especially with large datasets.
What are the implications of using lazy loading?
Using lazy loading can improve application performance by minimizing the amount of data loaded from the database at once. However, it requires careful management of session boundaries to avoid “lazy initialization” exceptions.
Are there alternatives to lazy loading in Hibernate?
Yes, alternatives include using DTOs (Data Transfer Objects) to fetch only the required data, utilizing batch fetching to load collections in batches, or employing Hibernate’s `@BatchSize` annotation to optimize loading of collections.
The error message “failed to lazily initialize a collection of role” typically occurs in Hibernate, a popular object-relational mapping framework in Java. This issue arises when an application attempts to access a lazily loaded collection outside of an active Hibernate session. In Hibernate, collections can be configured to load data lazily, meaning that the data is only retrieved from the database when it is explicitly accessed. If the session is closed before this access occurs, the framework cannot fetch the data, leading to this error.
To address this issue, developers can adopt several strategies. One common approach is to ensure that the session remains open while accessing the lazily loaded collections. This can be achieved by using techniques such as Open Session in View (OSIV) or by eagerly fetching the collections when the parent entity is retrieved. Another solution is to explicitly initialize the collection before the session is closed, using methods like `Hibernate.initialize()` or by using a query that fetches the necessary data upfront.
In summary, understanding the lazy loading mechanism in Hibernate and the lifecycle of sessions is crucial for preventing the “failed to lazily initialize a collection of role” error. By employing appropriate strategies to manage session states and data fetching, developers can enhance the robustness of their
Author Profile
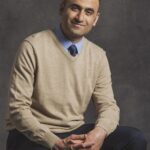
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?