How Can You Resolve the ‘Fatal Error: Allowed Memory Size’ Issue in Your Application?
In the world of programming and web development, encountering errors can be a frustrating yet enlightening experience. Among the myriad of issues developers face, the “fatal error: allowed memory size” stands out as a particularly common and perplexing problem. This error typically arises when a script attempts to utilize more memory than what is allocated by the server or environment settings. Understanding this error is crucial for developers who want to optimize their applications, enhance performance, and ensure a seamless user experience. In this article, we will delve into the causes of this error, its implications, and effective strategies to resolve it, empowering you to tackle memory-related challenges head-on.
The “allowed memory size” error is often a result of inefficient coding practices, excessive resource consumption, or simply the limitations set by the hosting environment. When a script exceeds the memory limit, it can lead to abrupt terminations, causing disruptions in service and potentially impacting user satisfaction. Developers must be aware of how their code interacts with memory allocation and the ways in which they can monitor and manage resource usage to prevent such errors from occurring.
In addition to understanding the underlying causes, it is essential to explore the various solutions available for addressing the “allowed memory size” error. From adjusting configuration settings to optimizing code, there are
Understanding PHP’s Memory Limit
When working with PHP, one common issue developers face is the “fatal error: allowed memory size” message. This error indicates that a script has exceeded the memory limit allocated to it by the PHP configuration. The memory limit is a safeguard to prevent poorly written scripts from consuming all available server resources.
The memory limit can be configured in several ways:
- php.ini: This is the main configuration file for PHP. The `memory_limit` directive controls the maximum amount of memory a script is allowed to consume.
- .htaccess: For Apache servers, you can modify the memory limit by adding a line to the .htaccess file:
“`
php_value memory_limit 128M
“`
- ini_set() function: You can also set the memory limit at runtime by using the `ini_set` function within your script:
“`php
ini_set(‘memory_limit’, ‘128M’);
“`
How to Check the Current Memory Limit
To check the current memory limit set for your PHP environment, you can use the following code snippet:
“`php
echo ini_get(‘memory_limit’);
“`
This will return the current limit, which is often set in bytes. Common values include:
- 128M (megabytes)
- 256M
- 512M
Common Causes of Memory Exhaustion
Several factors can lead to memory exhaustion in PHP scripts:
- Inefficient Code: Loops and recursive functions that do not exit properly can consume excessive memory.
- Large Data Sets: Handling large arrays or objects without efficient memory management can quickly lead to memory limits being exceeded.
- Third-Party Libraries: Sometimes, libraries or frameworks may not be optimized for memory usage, leading to unexpected spikes.
Here is a table summarizing common causes of memory issues:
Cause | Description | Potential Solution |
---|---|---|
Inefficient Code | Loops or functions that consume too much memory | Optimize algorithms and refactor code |
Large Data Sets | Handling large arrays/objects | Paginate or chunk data |
Third-Party Libraries | Memory leaks from libraries | Profile and replace inefficient libraries |
Increasing the Memory Limit
If you encounter this error frequently, you may need to increase the memory limit. However, this should be done with caution, as simply raising the limit may not address the underlying issue. Here’s how to increase the limit effectively:
- Evaluate the Script: Before increasing the limit, review the script to identify potential inefficiencies.
- Set a Reasonable Limit: Instead of setting an extremely high limit, consider a moderate increase:
“`php
ini_set(‘memory_limit’, ‘256M’);
“`
- Monitor Memory Usage: Use profiling tools to monitor memory consumption and identify bottlenecks.
By understanding the memory allocation in PHP and taking proactive measures, developers can effectively manage resources and minimize the risk of running into memory exhaustion errors.
Understanding Memory Limits in PHP
The “allowed memory size” error in PHP indicates that a script has exceeded the memory limit allocated for it. This limit is defined in the `php.ini` configuration file and can be adjusted according to the needs of your application.
- Default Memory Limit: The default memory limit in PHP is often set to 128 MB, but this can vary based on the server configuration.
- Configuration Directive: The directive that controls memory limits is `memory_limit`.
To check the current memory limit, you can use the following PHP code snippet:
“`php
echo ini_get(‘memory_limit’);
“`
Common Causes of Memory Exhaustion
Several factors can lead to memory exhaustion in PHP, including:
- Inefficient Code: Poorly optimized loops or recursive functions can consume excessive memory.
- Large Data Sets: Handling large arrays, especially during data processing, can quickly deplete memory resources.
- Third-party Libraries: Some libraries may not manage memory efficiently, leading to higher consumption.
- File Uploads: Large file uploads can exceed memory limits if not handled correctly.
Adjusting Memory Limits
If you encounter a memory limit error, you can adjust the memory limit using several methods:
- php.ini File: Modify the `php.ini` file directly.
“`ini
memory_limit = 256M
“`
- .htaccess File: If you do not have access to `php.ini`, you can set the limit in an `.htaccess` file.
“`apache
php_value memory_limit 256M
“`
- Runtime Configuration: You can also set the memory limit at runtime using the following PHP code:
“`php
ini_set(‘memory_limit’, ‘256M’);
“`
Monitoring Memory Usage
Monitoring memory usage is crucial for maintaining application performance. You can use built-in PHP functions to analyze memory consumption:
- memory_get_usage(): Returns the amount of memory allocated to your PHP script.
- memory_get_peak_usage(): Returns the peak memory allocated during the script execution.
Example code to monitor memory usage:
“`php
echo ‘Current memory usage: ‘ . memory_get_usage() . ‘ bytes’;
echo ‘Peak memory usage: ‘ . memory_get_peak_usage() . ‘ bytes’;
“`
Best Practices for Memory Management
To prevent memory exhaustion and optimize your PHP applications, consider the following best practices:
- Optimize Algorithms: Review and optimize your algorithms to reduce memory footprint.
- Limit Data Scope: Load only the necessary data into memory when processing.
- Utilize Generators: Use generators instead of arrays for handling large datasets to save memory.
- Unset Variables: Explicitly unset variables that are no longer needed to free up memory.
Managing memory effectively is essential for the performance and reliability of PHP applications. By understanding memory limits, monitoring usage, and implementing best practices, developers can minimize the risk of encountering memory-related errors.
Understanding Memory Limitations in Programming
Dr. Emily Carter (Senior Software Architect, Tech Innovations Inc.). “The ‘fatal error: allowed memory size’ message typically indicates that a script is attempting to use more memory than what is allocated in the PHP configuration. This can often be resolved by optimizing the code or increasing the memory limit in the php.ini file.”
Mark Thompson (Lead Developer, CodeCraft Solutions). “When encountering a ‘fatal error: allowed memory size’, it is crucial to analyze memory usage patterns within your application. Implementing memory profiling tools can help identify memory leaks or inefficient code segments that contribute to excessive memory consumption.”
Linda Chen (Performance Engineer, Web Optimization Group). “In many cases, the default memory limit set in PHP is insufficient for large applications. Developers should consider setting a higher memory limit, but this should be done cautiously, ensuring that the underlying code is also optimized to prevent similar issues in the future.”
Frequently Asked Questions (FAQs)
What does the error “fatal error: allowed memory size” mean?
This error indicates that a PHP script has exceeded the memory limit set in the PHP configuration, preventing further execution of the script.
How can I increase the allowed memory size in PHP?
You can increase the allowed memory size by modifying the `php.ini` file, using the `ini_set()` function within your script, or by adding a directive in your `.htaccess` file, depending on your server configuration.
What is the default memory limit for PHP?
The default memory limit for PHP is typically set to 128MB, but it can vary based on the server configuration and PHP version.
What are the consequences of setting a very high memory limit?
Setting a very high memory limit can lead to server performance issues, increased resource consumption, and potential crashes if multiple scripts run simultaneously, consuming excessive memory.
How can I check the current memory limit in PHP?
You can check the current memory limit by using the `phpinfo()` function, which displays the PHP configuration settings, including the `memory_limit` directive.
What should I do if increasing the memory limit does not resolve the error?
If increasing the memory limit does not resolve the error, consider optimizing your code to use less memory, reviewing third-party libraries for efficiency, or debugging to identify memory leaks within your application.
The “fatal error: allowed memory size” message is a common issue encountered in programming, particularly in PHP applications. This error indicates that a script has attempted to use more memory than is allocated by the PHP configuration settings. The default memory limit can often be insufficient for resource-intensive applications, leading to this error. Developers must understand the implications of memory limits and how to manage them effectively to ensure their applications run smoothly.
To resolve this error, developers can take several approaches. One common solution is to increase the memory limit in the PHP configuration file (php.ini) by modifying the `memory_limit` directive. Alternatively, memory limits can be adjusted on a per-script basis using the `ini_set` function within the script itself. It is also essential to identify and optimize any inefficient code that may be consuming excessive memory, as this can provide a more sustainable long-term solution.
Monitoring memory usage during development and testing phases is crucial. Tools and techniques such as profiling and debugging can help identify memory leaks or bottlenecks that contribute to high memory consumption. By implementing best practices in coding and resource management, developers can minimize the risk of encountering memory-related errors and enhance the overall performance of their applications.
Author Profile
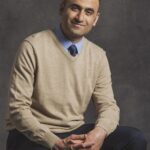
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?