How Can I Effectively Use Find and Replace with Regular Expressions?
In the digital age, where data manipulation and text editing are daily tasks for many professionals, the ability to efficiently find and replace text is a fundamental skill. Enter regular expressions, or regex, a powerful tool that transforms the way we search for and modify text. Whether you’re a programmer, a data analyst, or simply someone who frequently works with text documents, mastering the art of find and replace using regular expressions can significantly enhance your productivity and accuracy. This article will guide you through the intricacies of regex, equipping you with the knowledge to tackle complex text challenges with ease.
At its core, a regular expression is a sequence of characters that forms a search pattern. This pattern can be used to identify specific strings within larger bodies of text, making it an invaluable resource for tasks that require precision. The find and replace functionality allows users to not only locate text but also to modify it according to defined rules, opening up a world of possibilities for text processing. From simple substitutions to more advanced transformations, understanding how to harness the power of regex can streamline your workflow and reduce the likelihood of errors.
As we delve deeper into the realm of find and replace with regular expressions, we will explore the syntax and structure that make regex so versatile. You’ll discover practical applications, tips for crafting effective patterns
Understanding Regular Expressions
Regular expressions (regex) are sequences of characters that define a search pattern. They are widely used in programming, text processing, and data validation. The syntax can vary slightly between different programming languages and tools, but the core principles remain consistent.
Key components of regular expressions include:
- Literals: Characters that match themselves (e.g., `a` matches the letter ‘a’).
- Metacharacters: Special characters that have a unique meaning (e.g., `.` matches any character, `*` matches zero or more occurrences).
- Character classes: Sets of characters enclosed in brackets (e.g., `[abc]` matches ‘a’, ‘b’, or ‘c’).
- Anchors: Define position within the text (e.g., `^` asserts position at the start of a line, `$` asserts position at the end).
Common Find and Replace Patterns
When using regex for find and replace operations, you can leverage the following patterns to enhance your search efficiency:
- Word boundaries: Use `\b` to match a position where a word character is next to a non-word character.
- Groups: Parentheses `()` allow you to create groups, which can be referenced in the replacement string.
- Quantifiers: Specify the number of occurrences (e.g., `+` for one or more, `?` for zero or one).
Here are some examples of find and replace operations using regular expressions:
Find Pattern | Replace Pattern | Description |
---|---|---|
`(\d{3})-(\d{2})-(\d{4})` | `$1.$2.$3` | Formats a social security number from `XXX-XX-XXXX` to `XXX.XX.XXXX` |
`\bcat\b` | `dog` | Replaces whole word ‘cat’ with ‘dog’ |
`(\w+)\s+(\w+)` | `$2, $1` | Swaps first and last names |
Implementation in Various Tools
Different programming languages and text editors offer support for regex find and replace functionality. Below are examples of how to implement regex in some common environments:
Tool/Language | Find Syntax | Replace Syntax |
---|---|---|
JavaScript | /pattern/g | string.replace(/pattern/g, ‘replacement’); |
Python | r’pattern’ | re.sub(r’pattern’, ‘replacement’, string) |
Notepad++ | pattern | replacement (with checkbox for “Regular expression”) |
Testing and Debugging Regular Expressions
Testing regex patterns can often be challenging due to their complexity. There are several online tools and resources available for validating your expressions. Key features of these tools include:
- Live preview: See matches in real-time as you modify your pattern.
- Explanation: Provides a breakdown of your regex to understand its components.
- Sample text: Allows you to test your regex against different text inputs.
Utilizing these tools can significantly streamline the process of crafting effective find and replace operations using regular expressions.
Understanding Regular Expressions
Regular expressions (regex) are sequences of characters that form a search pattern. They are widely used for string searching and manipulation, especially in programming and text processing. Here are some key components of regular expressions:
- Literals: Directly match the characters you specify (e.g., `abc` matches “abc”).
- Meta-characters: Special characters that have specific meanings, such as:
- `.`: Matches any single character.
- `^`: Asserts the position at the start of a line.
- `$`: Asserts the position at the end of a line.
- `*`: Matches zero or more occurrences of the preceding element.
- `+`: Matches one or more occurrences of the preceding element.
- `?`: Matches zero or one occurrence of the preceding element.
- Character Classes: Define a set of characters to match, enclosed in square brackets (e.g., `[abc]` matches “a”, “b”, or “c”).
- Quantifiers: Specify how many instances of a character or group must be present for a match to occur:
- `{n}`: Exactly n times.
- `{n,}`: At least n times.
- `{n,m}`: Between n and m times.
Using Regular Expressions for Find and Replace
The find and replace functionality in text editors and programming languages often utilizes regular expressions to perform more complex search-and-replace operations. Here’s how it generally works:
- Find: Use a regex pattern to identify the text you want to replace.
- Replace: Specify a replacement string, which can include references to captured groups from the regex pattern.
Example Usage
Operation | Regex Pattern | Description | Replacement | |
---|---|---|---|---|
Find email | `\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z | a-z]{2,}\b` | Matches standard email addresses | `[email protected]` |
Find phone number | `\(\d{3}\) \d{3}-\d{4}` | Matches phone numbers in (123) 456-7890 format | `123-456-7890` | |
Find dates | `\b\d{1,2}/\d{1,2}/\d{2,4}\b` | Matches dates in MM/DD/YYYY or M/D/YY format | `YYYY-MM-DD` |
Implementation in Different Languages
Various programming languages have their own syntax and methods for performing find and replace operations with regular expressions. Below are examples from popular languages:
Python
“`python
import re
text = “Contact us at [email protected]”
result = re.sub(r’\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b’, ‘[email protected]’, text)
“`
JavaScript
“`javascript
let text = “Visit us at [email protected]”;
let result = text.replace(/\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b/g, ‘[email protected]’);
“`
Java
“`java
import java.util.regex.*;
String text = “Reach out at [email protected]”;
String result = text.replaceAll(“\\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\\.[A-Z|a-z]{2,}\\b”, “[email protected]”);
“`
Common Pitfalls
When using regular expressions for find and replace, several common issues may arise:
- Greediness: By default, quantifiers are greedy, meaning they will match as much text as possible. To make them non-greedy, append a `?` (e.g., `.*?`).
- Incorrect Escaping: Characters with special meanings in regex (like `.` or `*`) need to be escaped (e.g., `\.`).
- Performance: Complex patterns can lead to performance issues, especially with large datasets. Optimize your regex to improve efficiency.
By understanding the structure and common practices of regular expressions, users can effectively implement find and replace operations in various applications.
Expert Insights on Effective Use of Find and Replace Regular Expressions
Dr. Emily Carter (Data Scientist, Tech Innovations Inc.). “Regular expressions are a powerful tool for text manipulation, especially when using find and replace functions. Mastering regex can significantly enhance data cleaning processes, allowing for efficient pattern matching and replacement across large datasets.”
Mark Thompson (Software Engineer, CodeCraft Solutions). “When implementing find and replace with regular expressions, it is crucial to understand the syntax and structure of regex patterns. This knowledge enables developers to perform complex replacements accurately, minimizing errors and improving code quality.”
Linda Patel (Technical Writer, Regex Resources). “For anyone looking to streamline their text editing tasks, utilizing find and replace with regular expressions can save time and effort. It is essential to test your regex patterns thoroughly to ensure they perform as expected before applying them to larger projects.”
Frequently Asked Questions (FAQs)
What is a regular expression?
A regular expression, often abbreviated as regex, is a sequence of characters that defines a search pattern. It is commonly used for string matching and manipulation in programming and text processing.
How do I perform a find and replace using regular expressions?
To perform a find and replace using regular expressions, you typically use a programming language or text editor that supports regex. You define the search pattern and specify the replacement string. The tool then scans the text, identifies matches, and replaces them accordingly.
What are some common use cases for find and replace with regex?
Common use cases include data validation, formatting text (such as changing date formats), removing unwanted characters, and transforming strings based on specific patterns.
Are there any limitations to using regular expressions for find and replace?
Yes, regular expressions can become complex and difficult to read, especially for intricate patterns. Additionally, they may not be supported in all text editors or programming environments, and performance can degrade with very large datasets.
What are some examples of regular expressions for find and replace?
Examples include replacing all email addresses in a text with a placeholder using the pattern `\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,7}\b`, or changing all occurrences of a specific word with `\bword\b` to ensure whole word matches.
How can I test my regular expressions before using them?
You can test regular expressions using online regex testers or tools integrated into text editors. These platforms allow you to input your regex pattern and sample text to see matches and replacements in real-time, ensuring accuracy before implementation.
In summary, the find and replace functionality using regular expressions is a powerful tool for text manipulation across various programming languages and text editors. Regular expressions (regex) allow users to define complex search patterns, enabling them to locate specific strings or formats within larger bodies of text. This capability is particularly useful for tasks such as data cleaning, code refactoring, and content editing, where precision and efficiency are paramount.
One of the main advantages of using regex for find and replace operations is its flexibility. Users can craft intricate patterns that can match a wide range of text variations, including optional characters, repetitions, and specific character classes. This flexibility makes it possible to perform bulk edits that would be time-consuming or impossible with simple string matching methods.
Another key takeaway is the importance of understanding the syntax and semantics of regular expressions. Mastery of regex can significantly enhance a user’s ability to manipulate text effectively. While the learning curve may be steep for beginners, the investment in time and effort can yield substantial returns in productivity and accuracy in text processing tasks.
the find and replace functionality powered by regular expressions is an essential skill for anyone involved in programming, data analysis, or content management. By leveraging the capabilities of regex,
Author Profile
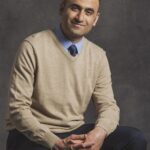
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?