How Can You Efficiently Find Text in SQL Server Stored Procedures?
In the world of data management, SQL Server stands as a powerful ally for businesses and developers alike. As organizations increasingly rely on vast amounts of data, the ability to efficiently locate specific information within databases becomes paramount. Whether you’re troubleshooting an application, analyzing user behavior, or simply seeking to enhance your data retrieval skills, mastering the art of finding text in SQL Server is an invaluable asset. This article delves into the various methods and best practices for searching text within stored procedures, offering insights that will empower you to extract the information you need with precision and ease.
When it comes to searching for text in SQL Server, the task can range from straightforward to complex, depending on the structure of your database and the nature of the text you’re seeking. SQL Server provides a variety of tools and functions designed to streamline this process, allowing users to perform searches within tables, views, and even the intricate layers of stored procedures. Understanding these tools not only enhances your querying capabilities but also equips you with the knowledge to optimize performance and ensure accurate results.
Moreover, as you navigate through the intricacies of SQL Server, you’ll discover that effective text searching involves more than just executing queries. It requires a strategic approach that considers factors such as indexing, data types, and the specific syntax of SQL
Using CHARINDEX to Find Text
In SQL Server, the `CHARINDEX` function is a fundamental tool for locating the position of a substring within a string. It returns the starting position of the first occurrence of the specified substring. If the substring is not found, it returns zero. The syntax is as follows:
“`sql
CHARINDEX ( expressionToFind , stringExpression [ , start_location ] )
“`
- `expressionToFind`: The substring you want to search for.
- `stringExpression`: The string in which you want to search.
- `start_location`: Optional. The position in the string from which to start the search.
Example usage:
“`sql
SELECT CHARINDEX(‘SQL’, ‘Learn SQL Server Programming’) AS Position;
“`
This query will return `7`, indicating that ‘SQL’ starts at the seventh character of the string.
Using PATINDEX for Pattern Matching
The `PATINDEX` function is similar to `CHARINDEX`, but it allows for wildcard characters, thus enabling more flexible pattern matching. The syntax is:
“`sql
PATINDEX ( ‘%pattern%’ , stringExpression )
“`
- `%pattern%`: The pattern that may include wildcard characters (`%` represents any sequence of characters).
- `stringExpression`: The string in which to search for the pattern.
Example usage:
“`sql
SELECT PATINDEX(‘%Server%’, ‘Learn SQL Server Programming’) AS Position;
“`
This will return `7`, as ‘Server’ is found starting at the seventh character.
Finding Text in a Table
To find specific text within a column in a table, you can use the `WHERE` clause combined with either `CHARINDEX` or `PATINDEX`. Here’s an example of how to do this with a table named `Employees` that contains a column `FullName`:
“`sql
SELECT *
FROM Employees
WHERE CHARINDEX(‘John’, FullName) > 0;
“`
This query retrieves all records where the `FullName` contains ‘John’.
Combining Conditions
You can also combine multiple conditions to refine your search. For example, to find names containing ‘John’ and ‘Doe’, you can use:
“`sql
SELECT *
FROM Employees
WHERE CHARINDEX(‘John’, FullName) > 0
AND CHARINDEX(‘Doe’, FullName) > 0;
“`
This ensures that both substrings are present in the `FullName` column.
Performance Considerations
When searching for text in SQL Server, be aware of performance implications, especially with large datasets. Here are some considerations:
- Indexing: If possible, index the columns you are searching in to improve performance.
- Full-Text Search: For complex searches and larger datasets, consider implementing SQL Server’s full-text search capabilities, which can significantly enhance performance and provide more advanced querying options.
Function | Description | Use Case |
---|---|---|
CHARINDEX | Finds the position of a substring. | Simple substring search. |
PATINDEX | Finds the position of a pattern using wildcards. | Pattern matching with wildcards. |
Full-Text Search | Advanced searching capabilities across large text. | Complex queries and searching large datasets. |
Finding Text in SQL Server
In SQL Server, locating specific text within your data can be accomplished using a variety of methods. Below are some of the primary techniques employed to search for text within tables.
Using the `LIKE` Operator
The `LIKE` operator is a fundamental SQL command used to search for a specified pattern in a column. It is particularly useful for performing wildcard searches.
Syntax:
“`sql
SELECT column1, column2
FROM table_name
WHERE column_name LIKE pattern;
“`
Wildcards:
- `%` – Represents zero or more characters.
- `_` – Represents a single character.
Example:
“`sql
SELECT *
FROM Employees
WHERE FirstName LIKE ‘J%’;
“`
This query retrieves all records from the Employees table where the FirstName starts with ‘J’.
Using the `CHARINDEX` Function
`CHARINDEX` is a function that returns the starting position of a specified expression in a character string. If the expression is not found, it returns zero.
**Syntax:**
“`sql
CHARINDEX(substring, string, start_position);
“`
**Example:**
“`sql
SELECT FirstName, LastName
FROM Employees
WHERE CHARINDEX(‘Smith’, LastName) > 0;
“`
This query finds all employees whose last name contains ‘Smith’.
Using the `PATINDEX` Function
`PATINDEX` is similar to `CHARINDEX` but allows for pattern matching using wildcards.
**Syntax:**
“`sql
PATINDEX(‘%pattern%’, string);
“`
**Example:**
“`sql
SELECT FirstName, LastName
FROM Employees
WHERE PATINDEX(‘%a%’, FirstName) > 0;
“`
This retrieves all employees whose first name contains the letter ‘a’.
Full-Text Search
For more advanced text search capabilities, SQL Server provides full-text search functionality. This is particularly effective for searching large text fields.
Steps to Enable Full-Text Search:
- Ensure the full-text feature is installed.
- Create a full-text index on the desired column.
Creating a Full-Text Index:
“`sql
CREATE FULLTEXT INDEX ON TableName(ColumnName)
KEY INDEX IndexName;
“`
Example of Full-Text Query:
“`sql
SELECT *
FROM Documents
WHERE CONTAINS(TextColumn, ‘search_term’);
“`
This retrieves documents containing ‘search_term’ in the TextColumn.
Using Regular Expressions in SQL Server
While SQL Server does not natively support regular expressions, you can utilize the `LIKE` operator and other string functions to achieve similar results.
Example with `LIKE`:
“`sql
SELECT *
FROM Employees
WHERE FirstName LIKE ‘[A-C]%’
“`
This retrieves employees whose first names start with letters A, B, or C.
Third-party solutions or CLR integration can also provide regex capabilities if necessary.
Combining Search Methods
You may often need to combine different search methods for comprehensive results. Using `AND` or `OR` operators can enhance the search capabilities.
**Example:**
“`sql
SELECT *
FROM Employees
WHERE FirstName LIKE ‘J%’
OR CHARINDEX(‘Smith’, LastName) > 0;
“`
This query fetches all employees whose first name starts with ‘J’ or whose last name contains ‘Smith’.
Performance Considerations
When searching for text within SQL Server, consider the following tips to optimize performance:
- Use indexes where applicable to speed up searches.
- Limit the number of records processed by filtering results early.
- Avoid wildcard searches at the beginning of a string, as they can lead to full table scans.
By employing these techniques, you can effectively find text in SQL Server databases while maintaining optimal performance.
Expert Insights on Finding Text in SQL Server Stored Procedures
Dr. Emily Carter (Database Architect, Tech Innovations Inc.). “When searching for specific text within stored procedures in SQL Server, utilizing the `sys.sql_modules` system view is essential. This view allows you to access the definition of stored procedures, enabling you to filter results using the `LIKE` operator to pinpoint the exact text you’re looking for.”
Michael Chen (Senior SQL Developer, Data Solutions Group). “To efficiently find text in stored procedures, I recommend writing a custom script that iterates through the `sys.sql_modules` and `sys.objects` tables. This approach not only enhances performance but also provides a more comprehensive search capability across multiple databases if needed.”
Laura Simmons (Database Management Consultant, SQL Insights). “In addition to querying `sys.sql_modules`, consider using SQL Server Management Studio’s built-in search functionality. This tool can quickly locate text across all stored procedures and functions, making it a user-friendly option for developers who prefer a graphical interface.”
Frequently Asked Questions (FAQs)
How can I find specific text within a column in SQL Server?
You can use the `LIKE` operator in a `SELECT` statement to find specific text within a column. For example: `SELECT * FROM TableName WHERE ColumnName LIKE ‘%searchText%’;` This query retrieves all rows where `ColumnName` contains `searchText`.
What is the difference between CHARINDEX and PATINDEX in SQL Server?
`CHARINDEX` returns the starting position of a specified substring within a string, while `PATINDEX` works similarly but allows for wildcard characters. Use `CHARINDEX` for exact matches and `PATINDEX` for pattern matching.
Can I search for text in multiple columns in SQL Server?
Yes, you can search multiple columns by combining conditions with the `OR` operator. For example: `SELECT * FROM TableName WHERE Column1 LIKE ‘%searchText%’ OR Column2 LIKE ‘%searchText%’;` This retrieves rows where either column contains the specified text.
Is it possible to perform a case-insensitive search in SQL Server?
Yes, SQL Server performs case-insensitive searches by default if the collation of the column is set to a case-insensitive collation. You can also specify a case-insensitive collation in your query, such as `COLLATE SQL_Latin1_General_CP1_CI_AS`.
How can I find text in a stored procedure in SQL Server?
To find text in a stored procedure, you can query the `sys.sql_modules` system view. Use the following query: `SELECT OBJECT_NAME(object_id), definition FROM sys.sql_modules WHERE definition LIKE ‘%searchText%’;` This retrieves the names and definitions of stored procedures containing the specified text.
What functions can I use for more complex text searches in SQL Server?
For more complex text searches, consider using `FULLTEXT` search capabilities in SQL Server. This allows for advanced querying, including searching for words or phrases, and supports features like proximity searching and ranking of results.
In SQL Server, finding specific text within a database can be accomplished through various methods, including the use of the `LIKE` operator, full-text search capabilities, and string functions. The `LIKE` operator is commonly employed for pattern matching in queries, allowing users to search for specific substrings within text fields. However, this method may not be efficient for large datasets or complex queries.
For more advanced text search requirements, SQL Server offers full-text search functionality. This feature enables users to perform sophisticated searches on large volumes of text data, including the ability to search for words, phrases, and even proximity searches. Implementing full-text indexing can significantly enhance search performance and accuracy, making it a valuable tool for applications that require robust text retrieval capabilities.
Additionally, SQL Server provides various string functions, such as `CHARINDEX`, `PATINDEX`, and `SUBSTRING`, which can be utilized to locate and manipulate text within strings. These functions allow for more granular control over text processing, enabling users to extract specific portions of text or determine the position of a substring within a larger string.
In summary, SQL Server offers multiple approaches to finding text within databases, each suited to different scenarios and performance considerations. By leveraging the
Author Profile
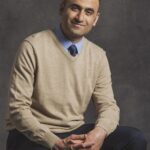
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?