How Can You Store Output to a File in Flink?
In the world of big data processing, Apache Flink stands out as a powerful framework that enables real-time stream processing and batch data analysis. As organizations increasingly rely on data-driven insights, the ability to efficiently store and manage output data becomes crucial. One common requirement in data processing workflows is the need to store the results of computations into files for further analysis, reporting, or archival purposes. This article explores the various methods and best practices for outputting data from Flink jobs to files, ensuring that your data is not only processed effectively but also stored in a manner that meets your operational needs.
When working with Apache Flink, understanding how to write output data to files is essential for any data engineer or developer. Flink provides a variety of connectors and file formats that allow users to easily manage their output data. Whether you’re dealing with large-scale batch processing or real-time streaming applications, knowing how to configure your Flink jobs to write results to files can significantly enhance your data pipeline’s efficiency and reliability.
In this article, we will delve into the different options available for storing Flink output to files, including supported file formats, configuration settings, and performance considerations. We will also discuss common use cases and scenarios where file output is not just beneficial but necessary for effective
Flink File Sink Configuration
To store output in files using Apache Flink, you need to configure a file sink. Flink provides a variety of sinks, but the File Sink is particularly useful for writing data to files in a distributed manner. This configuration generally involves specifying the file path, format, and other parameters.
Key components to consider when configuring a File Sink in Flink include:
- File Path: The location where output files will be written.
- File Format: The format of the output files (e.g., Text, Parquet, Avro).
- Rolling Policy: Determines how and when to roll over to a new file.
- Partitioning: Allows output data to be organized into directories based on certain criteria.
Implementing a File Sink
To implement a File Sink in your Flink job, you can follow these steps:
- Import Required Libraries: Ensure you have the necessary Flink libraries in your project.
- Create a StreamExecutionEnvironment: This is the context in which your data processing is performed.
- Define Your Data Stream: Set up the data stream that you want to write to a file.
- Configure the File Sink: Specify the output path, file format, and other parameters.
Here’s an example code snippet illustrating how to configure a File Sink in Flink:
“`java
import org.apache.flink.api.common.serialization.SimpleStringEncoder;
import org.apache.flink.streaming.api.environment.StreamExecutionEnvironment;
import org.apache.flink.streaming.api.datastream.DataStream;
import org.apache.flink.streaming.api.functions.sink.filesystem.StreamingFileSink;
import org.apache.flink.streaming.api.functions.sink.filesystem.OutputFileConfig;
public class FlinkFileSinkExample {
public static void main(String[] args) throws Exception {
final StreamExecutionEnvironment env = StreamExecutionEnvironment.getExecutionEnvironment();
DataStream
StreamingFileSink
.forRowFormat(new Path(“output/path”), new SimpleStringEncoder
.withOutputFileConfig(OutputFileConfig.builder().withPartPrefix(“prefix”).withPartSuffix(“.txt”).build())
.build();
stream.addSink(sink);
env.execute(“Flink File Sink Example”);
}
}
“`
File Sink Features and Best Practices
When using a File Sink in Flink, it’s essential to understand some of its features and best practices:
- Checkpointing: Use Flink’s checkpointing feature to ensure fault tolerance. This helps in recovering the sink state in case of failures.
- Scaling: You can scale the sink to handle increased data volume by partitioning the output files based on keys.
- File Rolling: Set up a rolling policy to manage how often files are closed and new ones are created. This can be based on size, time, or a combination of both.
Parameter | Description |
---|---|
Output Path | Path to the directory where output files will be stored |
File Format | Format in which the data will be written (e.g., CSV, Parquet) |
Rolling Policy | Conditions under which new files are created |
Partitioning Strategy | Method to organize data into subdirectories |
By adhering to these guidelines and leveraging the built-in capabilities of Flink, you can efficiently store output data to files while ensuring reliability and performance.
Writing Output to Files in Apache Flink
Apache Flink provides several ways to write output data to files, allowing users to store the results of stream and batch processing jobs efficiently. Below are the common approaches to achieve file output in Flink.
File Sink Options
Flink supports various file formats through its File Sink API, which allows users to write data in a flexible manner. The primary file formats include:
- Text Files: Simple, line-oriented text output.
- CSV Files: Comma-separated values for easy tabular data representation.
- Parquet: A columnar storage file format optimized for large-scale data processing.
- Avro: A row-oriented storage format that supports schema evolution.
Implementing File Output in Flink
To write output to files in Flink, you can follow these general steps:
- Set up the execution environment.
- Create a DataStream or DataSet with the desired input data.
- Apply transformations as necessary.
- Use a File Sink to write the output.
Here’s an example of writing to a text file using the DataStream API:
“`java
import org.apache.flink.streaming.api.environment.StreamExecutionEnvironment;
import org.apache.flink.streaming.api.datastream.DataStream;
public class FileOutputExample {
public static void main(String[] args) throws Exception {
final StreamExecutionEnvironment env = StreamExecutionEnvironment.getExecutionEnvironment();
DataStream
inputData.writeAsText(“output/output.txt”);
env.execute(“Flink File Output Example”);
}
}
“`
Configuration of File Sink
When configuring a File Sink, consider the following parameters:
Parameter | Description |
---|---|
Path | Specifies the file path where the output will be stored. |
Format | Defines the format of the output file (e.g., Text, CSV, Parquet). |
Rolling Policy | Determines when to roll over to a new file (e.g., based on size, time). |
File Compression | Option to compress files (e.g., gzip, snappy). |
Example of Configuring File Sink with Parquet
Below is a code snippet that demonstrates how to configure a File Sink to write output in Parquet format:
“`java
import org.apache.flink.streaming.api.environment.StreamExecutionEnvironment;
import org.apache.flink.connector.file.sink.FileSink;
import org.apache.flink.connector.file.sink.rollingpolicies.DefaultRollingPolicy;
import org.apache.flink.formats.parquet.ParquetAvroWriters;
public class ParquetFileOutputExample {
public static void main(String[] args) throws Exception {
final StreamExecutionEnvironment env = StreamExecutionEnvironment.getExecutionEnvironment();
DataStream
FileSink
.forBulkFormat(new Path(“output/mydata.parquet”), ParquetAvroWriters.forSpecificRecord(MyDataType.class))
.withRollingPolicy(DefaultRollingPolicy.builder()
.withRolloverInterval(TimeUnit.MINUTES.toMillis(10))
.withInactivityInterval(TimeUnit.MINUTES.toMillis(5))
.withMaxPartSize(1024 * 1024 * 128)
.build())
.build();
inputData.sinkTo(sink);
env.execute(“Flink Parquet File Output Example”);
}
}
“`
Best Practices for File Output
When writing output to files in Flink, consider the following best practices:
- Batch Size: Optimize the batch size for performance and resource utilization.
- Error Handling: Implement proper error handling to manage write failures.
- Data Partitioning: Utilize data partitioning to enhance parallelism and performance.
- Monitoring: Monitor the file output process to ensure timely completion and data integrity.
By following these guidelines, you can effectively manage output file writing in your Flink applications.
Expert Insights on Flink Output File Storage
Dr. Emily Chen (Data Engineering Specialist, Big Data Insights). “Apache Flink provides several options for outputting data to files, including the use of the FileSink API. This allows for efficient writing of streaming data to various file formats such as Parquet and Avro, enabling seamless integration with data lakes and analytical tools.”
Mark Thompson (Senior Software Architect, StreamTech Solutions). “When configuring Flink to store output to files, it is crucial to consider the partitioning strategy. Proper partitioning can significantly enhance read performance and data management, especially in large-scale applications where data volume is substantial.”
Lisa Patel (Lead Data Scientist, Analytics Innovations). “Incorporating checkpointing and state management when writing output to files in Flink is essential for ensuring data consistency and fault tolerance. This practice mitigates the risk of data loss during processing interruptions, providing a robust solution for real-time data applications.”
Frequently Asked Questions (FAQs)
How can I store output to a file in Apache Flink?
You can store output to a file in Apache Flink by using the `writeAsText()` method for text files or `writeAsCsv()` for CSV files within a DataStream or DataSet. This method allows you to specify the output path where the results will be saved.
What formats can I use to write output files in Flink?
Flink supports various output formats including text, CSV, Parquet, Avro, and JSON. You can choose the format based on your requirements and the libraries available in your Flink environment.
Can I specify the output file name when writing to a file in Flink?
Flink does not allow you to specify the output file name directly. Instead, it generates output files with a unique identifier based on the job execution. You can control the output path but not the individual file names.
Is it possible to write output to multiple files in Flink?
Yes, Flink can write output to multiple files by using partitioning or bucketing strategies. You can achieve this by configuring the output format to partition data based on a key or using a specific number of output files.
How do I handle file output in Flink when dealing with large datasets?
For large datasets, it is advisable to use a distributed file system like HDFS or S3. Flink’s output formats are designed to handle large volumes of data efficiently, allowing for parallel writes and optimized storage.
What are the best practices for writing output files in Flink?
Best practices include using appropriate output formats based on your data type, ensuring fault tolerance by configuring checkpointing, and managing output paths to avoid conflicts. Additionally, consider performance optimizations like batch writing and using partitioning strategies.
Apache Flink is a powerful stream processing framework that enables users to process large volumes of data in real-time. One of the common requirements when working with Flink is the ability to store output data to files for further analysis or archival purposes. Flink provides several connectors and sink options that facilitate the writing of processed data to various file formats, including text, CSV, and Parquet, among others. This capability is crucial for applications that require persistent storage or integration with other data processing workflows.
When configuring Flink to write output to files, users can leverage the FileSink API, which allows for efficient and fault-tolerant writing of data streams to the file system. This API supports various options, such as specifying the output format, partitioning data, and managing file rotation and cleanup. Additionally, users can choose between batch and streaming modes, depending on their specific use case and performance requirements. The flexibility of Flink’s file output options empowers developers to tailor their data storage solutions effectively.
In summary, storing output to files in Apache Flink is a straightforward process that can be customized to meet diverse needs. By utilizing the FileSink API and understanding the various configurations available, users can ensure that their data is stored reliably and efficiently
Author Profile
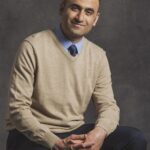
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?