Why Am I Getting a ‘float’ Object Is Not Subscriptable Error in Python?
Have you ever encountered the perplexing error message, “`float` object is not subscriptable” while coding in Python? If so, you’re not alone. This common pitfall can leave both novice and seasoned programmers scratching their heads in confusion. Understanding this error is crucial for anyone looking to refine their coding skills and enhance their problem-solving abilities. In this article, we will unravel the mystery behind this error, explore its causes, and equip you with the knowledge to prevent it in your future coding endeavors.
Overview
At its core, the “`float` object is not subscriptable” error arises when a programmer attempts to access elements of a floating-point number as if it were a list or a dictionary. This misstep often occurs when there’s a misunderstanding of data types and their respective properties in Python. As you navigate through the intricacies of Python’s type system, recognizing the difference between mutable and immutable objects becomes essential.
In addition to clarifying the nature of this error, we’ll delve into common scenarios that lead to its occurrence. By examining typical coding mistakes and providing practical examples, you will gain a clearer understanding of how to avoid this error in your own projects. Whether you’re debugging a complex algorithm or simply experimenting with new code, knowing how to handle
Understanding the Error
The error message `’float’ object is not subscriptable` occurs in Python when an attempt is made to access an index or a key on a float object, which is not a subscriptable type. Subscriptable types, such as lists, tuples, and dictionaries, allow for indexing or key-based access, while float variables represent numeric values that cannot be indexed.
This error typically arises in scenarios such as:
- Attempting to access an element of a float as if it were a list or similar collection.
- Misinterpretation of variable types, where a float is expected to be a more complex data structure.
For example, consider the following code:
“`python
num = 3.14
print(num[0]) Raises TypeError
“`
In this case, the code tries to access the first element of `num`, which is a float, leading to the error.
Common Causes
There are several common scenarios that can lead to this error:
- Incorrect Variable Assignment: Assigning a float to a variable that is expected to be a list or dictionary.
- Function Return Values: A function that is supposed to return a list but instead returns a float.
- Data Parsing: When parsing data from a source (like a CSV file), a float might be misinterpreted.
Here is a brief overview of potential causes:
Cause | Description |
---|---|
Variable Misassignment | Assigning a float to a variable meant for a list or dictionary. |
Function Errors | Functions returning unexpected float values instead of lists. |
Data Type Confusion | Parsing errors where floats are treated as lists. |
Troubleshooting Steps
To resolve the `’float’ object is not subscriptable` error, follow these troubleshooting steps:
- Check Variable Types: Use the `type()` function to verify the data type of your variables.
“`python
print(type(num)) Should return
“`
- Inspect Function Outputs: Ensure that functions return the expected types. If a function is supposed to return a list but returns a float, modify it accordingly.
- Review Data Parsing Logic: When dealing with external data, confirm the format and types being read. Use appropriate conversion techniques to ensure that data is in the expected format.
- Debugging: Use debugging tools or print statements to trace variable assignments and function outputs to identify where the float is being incorrectly accessed.
By systematically addressing these areas, you can effectively resolve the `’float’ object is not subscriptable` error and improve the robustness of your code.
Understanding the Error
The error message `’float’ object is not subscriptable` typically occurs in Python when there is an attempt to access an index or a key of a float variable as if it were a list, tuple, dictionary, or another subscriptable type. This can occur in various scenarios, most commonly when mistakenly treating a numeric value as a collection.
Common Causes
Several situations can lead to this error:
- Incorrect Variable Assignment: Assigning a float to a variable that should hold a collection.
- Function Return Values: Functions returning a float instead of a list or other subscriptable type.
- Indexing Mistakes: Attempting to index directly into a float value.
Example Scenarios
Here are some illustrative examples of this error:
“`python
Example 1: Incorrect assignment
my_value = 3.14
print(my_value[0]) Raises TypeError: ‘float’ object is not subscriptable
Example 2: Function returning a float
def calculate_area(radius):
return 3.14 * radius ** 2
area = calculate_area(5)
print(area[0]) Raises TypeError
Example 3: Indexing mistake
data = [1.0, 2.0, 3.0]
result = data[1] Correct, returns 2.0
print(result[0]) Raises TypeError
“`
Debugging Steps
To resolve the issue, consider the following debugging steps:
- Check Variable Types: Use `type()` to confirm the type of the variable you are attempting to index.
- Review Function Outputs: Ensure functions return the expected types, and verify if they need to return a collection.
- Add Print Statements: Insert print statements before the line of code that raises the error to examine the values and types being used.
Best Practices
To prevent this error from occurring in the future, adhere to these best practices:
- Use Descriptive Variable Names: Clearly differentiate between numeric values and collections.
- Type Annotations: Utilize type hints in function definitions to clarify expected return types.
- Unit Testing: Implement unit tests that check for type consistency in functions.
Type Checking with Assertions
Incorporating type checking can be beneficial:
“`python
def process_data(data):
assert isinstance(data, (list, tuple)), “Input must be a list or tuple.”
Process data…
“`
This approach helps ensure that the function receives the correct type, and it can provide immediate feedback if not.
Understanding the ‘float’ Object is Not Subscriptable Error
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error message ‘float’ object is not subscriptable typically arises when a programmer attempts to index or slice a float type, which is inherently not a sequence. This misunderstanding often stems from a failure to recognize the data type being manipulated, underscoring the importance of type checking in Python.”
Mark Thompson (Python Developer Advocate, CodeMaster Solutions). “In Python, the ‘float’ type represents a single numeric value, and thus does not support indexing. This error serves as a reminder for developers to ensure they are working with the correct data structures, particularly when transitioning between different types such as lists or dictionaries.”
Lisa Nguyen (Data Scientist, Analytics Hub). “When encountering the ‘float’ object is not subscriptable error, it is crucial to trace back the code to identify where a float is being mistakenly treated as a list or dictionary. Proper debugging practices, including print statements or using debugging tools, can significantly aid in resolving this common pitfall.”
Frequently Asked Questions (FAQs)
What does the error ‘float’ object is not subscriptable mean?
The error ‘float’ object is not subscriptable occurs when you attempt to access an index or key on a float variable, which does not support indexing. This typically happens when you mistakenly treat a float as a list or dictionary.
How can I fix the ‘float’ object is not subscriptable error?
To fix this error, ensure that you are not trying to index or slice a float variable. Check your code for any instances where you might be using a float in a way that implies it is a list or dictionary.
What are common scenarios that lead to this error?
Common scenarios include mistakenly assigning a float to a variable that was intended to hold a list or dictionary, or attempting to access elements of a float variable due to a logic error in the code.
Can this error occur in mathematical operations?
Yes, this error can occur if the result of a mathematical operation is mistakenly treated as a list or dictionary. For example, if you perform an operation that results in a float and then try to index it, the error will be raised.
Is there a way to prevent this error from occurring in my code?
To prevent this error, always verify the data types of your variables before performing indexing operations. Utilize type-checking functions like `isinstance()` to ensure that you are working with the correct data types.
What should I do if I encounter this error in a large codebase?
In a large codebase, systematically trace back the variable assignments leading to the error. Use debugging tools or print statements to identify the type of the variable at various stages, ensuring that you are not unintentionally assigning a float where a list or dictionary is expected.
The error message “‘float’ object is not subscriptable” typically arises in Python programming when a developer attempts to access an index or a key of a float object as if it were a list, tuple, or dictionary. This indicates a fundamental misunderstanding of data types in Python, where only certain data structures support indexing. Understanding the nature of the float data type is crucial, as it represents a numerical value and does not possess the characteristics necessary for subscriptable operations.
To resolve this error, it is essential to review the code where the float is being accessed. Developers should ensure that they are not mistakenly using a float where a list or another subscriptable object is expected. This often involves checking variable assignments and the data types being manipulated. Additionally, debugging techniques such as printing variable types can help identify where the issue originates.
Key takeaways include the importance of understanding data types in Python and the need for careful variable management. Developers should familiarize themselves with the properties of different data structures to avoid similar errors in the future. By implementing thorough testing and validation of inputs, programmers can enhance their code’s robustness and prevent runtime errors related to subscriptability.
Author Profile
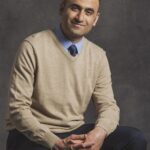
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?