Why Does Python Throw ‘Float Object is Not Subscriptable’ Error?
When diving into the world of Python programming, encountering errors is an inevitable part of the learning journey. One common error that can leave both novice and seasoned developers scratching their heads is the notorious “float object is not subscriptable” message. This seemingly cryptic warning often arises in the midst of coding, hinting at a fundamental misunderstanding of how data types function within the language. In this article, we will unravel the mystery behind this error, exploring its causes, implications, and how to effectively troubleshoot it.
At its core, the “float object is not subscriptable” error occurs when a programmer attempts to access an element of a float variable as if it were a list or a dictionary. In Python, certain data types, like lists and dictionaries, allow for indexing and slicing, enabling developers to retrieve specific elements. However, floats, which represent decimal numbers, do not possess this capability. Understanding the distinction between these data types is crucial for writing efficient and error-free code.
As we delve deeper into this topic, we will examine common scenarios that lead to this error, providing practical examples and solutions. By grasping the underlying principles of data types and their limitations, you will not only learn how to resolve this specific error but also enhance your overall programming skills. So,
Understanding the Error Message
The error message “float object is not subscriptable” typically arises in Python when a programmer attempts to access an index or a key from a float data type as though it were a list, tuple, or dictionary. In Python, subscriptable objects are those that can be indexed, such as lists, strings, and dictionaries. Floats, however, represent numerical values and do not support indexing.
Common scenarios leading to this error include:
- Attempting to access a specific index of a float variable.
- Mistaking a float for a list or array.
- Returning a float from a function instead of a list and then trying to index it.
Examples of the Error
To illustrate, consider the following code snippets that generate this error:
“`python
Example 1: Accessing a float as if it were a list
number = 3.14
print(number[0]) Raises TypeError: ‘float’ object is not subscriptable
“`
“`python
Example 2: Function returning a float
def calculate_area(radius):
return 3.14 * radius ** 2
area = calculate_area(5)
print(area[0]) Raises TypeError: ‘float’ object is not subscriptable
“`
In both examples, the attempt to access an index of a float leads to the error.
How to Resolve the Issue
To resolve the “float object is not subscriptable” error, consider the following strategies:
- Check Variable Types: Ensure that the variable you are trying to index is indeed a list, string, or dictionary. Use the `type()` function to verify the data type.
“`python
print(type(number)) Output:
“`
- Correct Data Structure: If you need to store multiple values, use an appropriate data structure such as a list or a dictionary instead of a float.
- Modify Function Returns: If a function is expected to return a list or another subscriptable type, ensure that it does not return a float.
Here’s a table summarizing common causes and resolutions:
Cause | Resolution |
---|---|
Accessing index of a float | Change variable to a list or string |
Function returning float instead of list | Modify function to return a list |
Mistakenly treating a float as a collection | Refactor code to use correct types |
By implementing these resolutions, programmers can avoid the “float object is not subscriptable” error and ensure their code functions as intended.
Understanding the Error
The error message “float object is not subscriptable” typically arises in Python when an attempt is made to access an element of a float variable as if it were a list or a dictionary. In Python, only certain data types are subscriptable, such as lists, tuples, and dictionaries.
Common Causes
- Direct Indexing: Trying to access a specific index of a float, e.g., `my_float[0]`.
- Function Return Values: Using a function that returns a float but expecting a list or similar structure.
- Incorrect Variable Assignment: Accidentally assigning a float to a variable that was intended to hold a list or dictionary.
Examples of the Error
Consider the following code snippets illustrating how this error can occur:
“`python
my_float = 3.14
print(my_float[0]) Error: ‘float’ object is not subscriptable
“`
In this example, `my_float` is a float, and attempting to index it raises the error.
Another example:
“`python
def get_value():
return 5.0
value = get_value()
print(value[0]) Error: ‘float’ object is not subscriptable
“`
Here, the function `get_value()` returns a float, but the code attempts to access it as if it were a list.
How to Fix the Error
To resolve this error, it is essential to ensure that you are not treating float variables as subscriptable objects. Here are some strategies:
- Check Variable Types: Use the `type()` function to confirm the type of the variable.
“`python
print(type(my_float)) This will confirm if it is indeed a float
“`
- Convert Floats to Strings: If you need to manipulate the float as a sequence, convert it to a string first.
“`python
my_float_str = str(my_float)
print(my_float_str[0]) This will output ‘3’
“`
- Use Lists or Tuples: If you intend to work with multiple values, consider using a list or tuple instead of a float.
“`python
my_values = [3.14, 2.71, 1.41]
print(my_values[0]) This will correctly output 3.14
“`
Best Practices
To avoid encountering the “float object is not subscriptable” error in the future, adhere to the following best practices:
- Explicit Type Checking: Always check the type of your variables, especially when dealing with functions that may return different types.
- Use Descriptive Variable Names: Name variables clearly to indicate their type and intended use, reducing confusion.
- Implement Exception Handling: Use try-except blocks to catch potential errors gracefully.
“`python
try:
print(my_float[0])
except TypeError:
print(“Cannot index into a float.”)
“`
By following these guidelines, you can minimize the occurrence of this error and ensure smoother code execution.
Understanding the ‘Float Object is Not Subscriptable’ Error
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “The error message ‘float object is not subscriptable’ typically arises when a programmer attempts to access an index of a float variable as if it were a list or a dictionary. This indicates a fundamental misunderstanding of data types in Python, where floats are singular numeric values and do not support indexing.”
Mark Thompson (Python Developer and Educator, CodeLearn Academy). “In Python, subscripting is a feature of iterable types such as lists and tuples. Encountering this error means that the developer is likely trying to manipulate a float as an iterable. It is crucial to ensure that the variable type aligns with the intended operations to avoid such errors.”
Linda Garcia (Data Scientist, Analytics World). “This error can often be a result of variable mismanagement, where a float is mistakenly assigned when a list or array was intended. It serves as a reminder to validate data types throughout the coding process, especially when dealing with complex data structures.”
Frequently Asked Questions (FAQs)
What does “float object is not subscriptable” mean?
This error indicates that you are attempting to access an index or key on a float object, which is not allowed. Float objects represent numeric values and do not support indexing like lists or dictionaries.
When does the “float object is not subscriptable” error occur?
This error typically occurs when you mistakenly try to use square brackets to access elements of a float variable, treating it as a list or similar data structure.
How can I fix the “float object is not subscriptable” error?
To resolve this error, ensure that you are not using indexing on a float variable. Check your code to confirm that you are accessing the correct data type, such as a list or dictionary, instead of a float.
Can I convert a float to a list to avoid this error?
While you can convert a float to a list, it will not resolve the error directly. Instead, focus on understanding the data types you are working with and ensure that you are using indexing only with subscriptable types.
What are examples of subscriptable objects in Python?
Subscriptable objects in Python include lists, tuples, strings, and dictionaries. These data types allow for indexing and key access, unlike float objects.
Is this error specific to Python, or can it occur in other programming languages?
The “float object is not subscriptable” error is specific to Python. Other programming languages may have similar concepts but will use different error messages or handling mechanisms for type-related issues.
The error message “float object is not subscriptable” typically arises in Python programming when an attempt is made to index or access elements of a float variable as if it were a list, tuple, or dictionary. In Python, subscripting is a feature that allows the retrieval of elements from data structures that support indexing. However, since float is a primitive data type representing a numerical value, it does not support this operation, resulting in the error. Understanding the nature of data types and their corresponding operations is crucial for effective programming and debugging.
This error often occurs due to a misunderstanding of variable types or a programming oversight, such as mistakenly assigning a float to a variable that is expected to be a list or another subscriptable type. To resolve this issue, developers should carefully check their code to ensure that they are not attempting to access elements of a float variable. It is advisable to use print statements or debugging tools to trace the variable types and values throughout the code execution.
In summary, the “float object is not subscriptable” error serves as a reminder of the importance of data type awareness in Python. By maintaining clarity about the types of variables being used and their capabilities, programmers can avoid common pitfalls associated with subscripting. This
Author Profile
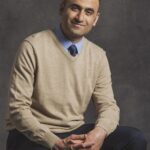
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?