How Can I Resolve the ‘java.security.InvalidKeyException: Failed to Unwrap Key’ Error in Flutter?
In the rapidly evolving world of mobile application development, Flutter has emerged as a powerhouse framework, allowing developers to create beautiful, natively compiled applications for multiple platforms from a single codebase. However, as with any technology, developers often encounter challenges that can hinder their progress. One such challenge is the `java.security.InvalidKeyException: failed to unwrap key` error, which can arise during cryptographic operations in Flutter applications. This error not only disrupts the development workflow but also raises critical questions about security, data protection, and the proper implementation of encryption techniques.
Understanding the intricacies of encryption in Flutter, especially when interfacing with Java-based libraries, is essential for any developer looking to safeguard user data. The `InvalidKeyException` typically indicates that there is a mismatch in key specifications or an issue with how keys are being handled during encryption and decryption processes. As developers delve into the world of cryptography, they must navigate various complexities, including key management, algorithm compatibility, and the nuances of platform-specific implementations.
In this article, we will explore the common pitfalls that lead to the `InvalidKeyException`, offering insights into best practices for managing encryption keys within Flutter applications. By addressing these challenges head-on, developers can enhance their understanding of secure coding practices and ensure that their
Understanding the `InvalidKeyException` in Flutter Encryption
When working with encryption in Flutter, developers might encounter the `java.security.InvalidKeyException: failed to unwrap key` error. This exception typically arises when there is an issue with the cryptographic key being used for the decryption process. Understanding the causes of this exception is crucial for effective troubleshooting.
Common causes of the `InvalidKeyException` include:
- Key Mismatch: The key used for encryption does not match the key being used for decryption.
- Incorrect Key Format: The key format may not be compatible with the expected format by the decryption algorithm.
- Algorithm Discrepancy: The algorithm used for encryption does not align with the algorithm specified for decryption.
- Corrupted Key Data: The key data may have been altered or corrupted during storage or transmission.
Key Management Strategies
Proper key management is essential to prevent issues related to key exceptions. Here are some strategies to manage keys effectively:
- Consistent Key Generation: Ensure that the keys are generated consistently using the same algorithm and parameters.
- Key Storage: Use secure storage solutions such as the secure enclave or key management services (KMS) to store keys securely.
- Key Rotation: Regularly rotate keys to enhance security and mitigate the impact of a potential key compromise.
- Version Control: Maintain versions of keys to manage changes and ensure backward compatibility.
Debugging the `InvalidKeyException`
Debugging the `InvalidKeyException` can involve several steps. Follow this checklist to identify the root cause:
- Verify Key Pair: Ensure that the public and private keys are correctly paired.
- Check Key Encoding: Validate the encoding of the key (e.g., Base64) used in both encryption and decryption processes.
- Review Cipher Configuration: Double-check that the cipher’s configuration matches on both ends (encryption and decryption).
- Log Key Details: Implement logging to capture key details at various points in the encryption and decryption processes.
Cause | Description |
---|---|
Key Mismatch | The encryption key does not match the decryption key. |
Incorrect Key Format | The key format is incompatible with the decryption algorithm. |
Algorithm Discrepancy | The algorithms used for encryption and decryption differ. |
Corrupted Key Data | The key has been altered or corrupted during storage or transmission. |
By systematically addressing each potential cause and implementing robust key management practices, developers can significantly reduce the likelihood of encountering the `java.security.InvalidKeyException` in their Flutter applications.
Understanding java.security.InvalidKeyException
The `java.security.InvalidKeyException` is a critical exception in Java that indicates a problem with the cryptographic key being used. This exception typically arises when the key format is incorrect or the key itself is not suitable for the intended cryptographic operation. In the context of Flutter applications that utilize encryption, this error often surfaces during key unwrapping processes.
Common Causes of InvalidKeyException
Several factors can contribute to encountering a `java.security.InvalidKeyException` during key unwrapping:
- Incorrect Key Format: The key must be in the expected format (e.g., AES keys must be of a specific size).
- Mismatched Algorithms: The algorithm used for wrapping and unwrapping must be the same.
- Expired or Revoked Keys: Keys may become invalid if they are not current or have been revoked.
- Corrupted Key Data: Any alteration or corruption of key data can lead to this exception.
- Unsupported Key Type: The key type must be compatible with the operation being performed.
Troubleshooting Steps
When faced with a `java.security.InvalidKeyException`, consider the following troubleshooting steps:
- Verify Key Format:
- Ensure that the key is in the correct format and size.
- Use appropriate encoding (e.g., Base64) when transferring keys between systems.
- Check Algorithm Compatibility:
- Confirm that the same algorithm is used for both key wrapping and unwrapping.
- Ensure that the encryption and decryption methods match in terms of algorithm and padding.
- Inspect Key Source:
- Validate the origin of the key. If it’s generated or shared from another system, ensure it is correctly formatted and not corrupted.
- Review Security Provider:
- Check if the security provider supports the required key size and type. Some providers may have restrictions.
- Debugging:
- Use logging to capture the state of the key before the unwrapping process.
- Utilize exception handling to gain more insights into the failure.
Example Code Snippet
Here is a simplified example of how to handle key wrapping and unwrapping in Java that may help to avoid `InvalidKeyException`:
“`java
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import javax.crypto.spec.SecretKeySpec;
public class KeyManagement {
public static void main(String[] args) throws Exception {
// Generate a key
KeyGenerator keyGen = KeyGenerator.getInstance(“AES”);
keyGen.init(256); // Key size
SecretKey secretKey = keyGen.generateKey();
// Wrap the key
Cipher cipher = Cipher.getInstance(“AES”);
cipher.init(Cipher.WRAP_MODE, secretKey);
byte[] wrappedKey = cipher.wrap(secretKey);
// Unwrap the key
cipher.init(Cipher.UNWRAP_MODE, secretKey);
SecretKey unwrappedKey = (SecretKey) cipher.unwrap(wrappedKey, “AES”, Cipher.SECRET_KEY);
}
}
“`
This example illustrates the correct process of wrapping and unwrapping keys while ensuring the key format and algorithm are consistent.
Conclusion on Exception Handling
Incorporating robust exception handling while processing encryption keys is crucial. Implement `try-catch` blocks to manage `InvalidKeyException` effectively and provide informative error messages to aid in debugging. By following best practices for key management and understanding the nuances of cryptographic operations, developers can mitigate the risk of encountering this exception in Flutter applications.
Understanding Key Management Issues in Flutter Encryption
Dr. Emily Chen (Cryptography Researcher, SecureTech Labs). “The `java.security.InvalidKeyException: failed to unwrap key` error typically arises from mismatched key specifications or incorrect padding during the decryption process. Ensuring that both the encryption and decryption keys align in terms of algorithm and format is crucial for successful key unwrapping.”
Michael Thompson (Mobile Security Architect, AppGuard Solutions). “In Flutter applications, developers often overlook the importance of consistent key management practices across platforms. When using Java for backend services, it’s essential to validate that the keys generated in Flutter are compatible with the Java security framework to avoid such exceptions.”
Sarah Patel (Lead Software Engineer, CryptoSecure Innovations). “The `InvalidKeyException` can also indicate that the key being used has been corrupted or improperly formatted. Developers should implement robust error handling and logging mechanisms to capture the context of the error, which will facilitate quicker debugging and resolution.”
Frequently Asked Questions (FAQs)
What does the error “java.security.InvalidKeyException: failed to unwrap key” mean?
This error indicates that there is an issue with the cryptographic key being used in the encryption or decryption process. It typically arises when the key format is incorrect, the key is not compatible with the algorithm, or the key has been corrupted.
What are common causes of the “failed to unwrap key” error in Flutter applications?
Common causes include using an incorrect key size, mismatched encryption algorithms, or attempting to unwrap a key that was not properly encrypted. Additionally, issues with key storage or retrieval can also lead to this error.
How can I resolve the “java.security.InvalidKeyException” in my Flutter project?
To resolve this issue, ensure that the key being used for unwrapping matches the key used for wrapping. Verify the key length and algorithm compatibility. Additionally, check that the key is correctly formatted and has not been altered or corrupted.
Is there a specific Flutter package that can help prevent this error?
Yes, using well-maintained packages such as `encrypt` or `flutter_secure_storage` can help manage encryption and key storage more effectively, reducing the likelihood of encountering this error. Ensure you follow the documentation for proper key management.
Can the “failed to unwrap key” error occur due to version mismatches in dependencies?
Yes, version mismatches between cryptographic libraries can lead to compatibility issues, which may result in the “failed to unwrap key” error. It is essential to ensure that all dependencies are compatible and up to date.
What best practices should I follow to avoid key-related errors in Flutter applications?
Best practices include using standardized key management protocols, ensuring proper key generation and storage, avoiding hard-coded keys, and regularly updating cryptographic libraries. Additionally, thorough testing of encryption and decryption processes is crucial.
The error message “java.security.InvalidKeyException: failed to unwrap key” typically arises in the context of cryptographic operations in Java, particularly when dealing with key management and encryption processes. This exception indicates that the system encountered an issue while attempting to decrypt or unwrap a key, often due to an invalid or incompatible key format, algorithm, or padding scheme. Understanding the root causes of this exception is crucial for developers working with encryption in Flutter applications, especially when integrating with Java-based back-end services.
One of the primary reasons for this error is the mismatch between the encryption and decryption processes. Developers must ensure that the keys used for wrapping and unwrapping are compatible and that the correct algorithms and modes are applied consistently. Additionally, it is essential to verify that the cryptographic keys are properly generated, stored, and retrieved, as any discrepancies can lead to failures in unwrapping the key.
Another important insight is the necessity of thorough testing and debugging when implementing encryption features in applications. Developers should utilize logging and error-handling mechanisms to capture detailed information about the cryptographic operations being performed. This practice can help identify the exact point of failure and facilitate quicker resolutions. Furthermore, leveraging established libraries and frameworks for encryption can mitigate common pitfalls associated
Author Profile
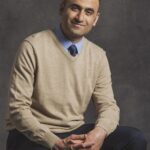
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?