Is Your Code at Risk? Understanding the ‘g May Be Used Uninitialized’ Warning
In the world of programming, the phrase “g may be used uninitialized” can send shivers down the spine of even the most seasoned developers. This warning, often encountered in languages like C or C++, serves as a stark reminder of the importance of proper variable initialization. As software systems grow increasingly complex, understanding the implications of uninitialized variables becomes crucial—not just for maintaining code quality but also for ensuring the reliability and security of applications. In this article, we will delve into the nuances of uninitialized variables, explore the potential pitfalls they present, and discuss best practices to avoid these common traps.
Overview
When a variable is declared but not initialized, it holds an indeterminate value, which can lead to unpredictable behavior in a program. This scenario often arises with global variables, such as the variable ‘g’, which may be inadvertently used before it has been assigned a meaningful value. Such situations can lead to bugs that are notoriously difficult to trace, as the program may produce erratic outputs or crash without warning. Understanding how and when this warning appears is essential for developers who wish to write robust and maintainable code.
Moreover, the implications of using uninitialized variables extend beyond mere functionality. They can introduce security vulnerabilities, enabling malicious actors to exploit these weaknesses in
Understanding Uninitialized Variables
In programming, particularly in languages like C and C++, the concept of uninitialized variables can lead to unexpected behavior. An uninitialized variable is one that has been declared but has not been assigned a specific value. This can result in the variable containing a garbage value, which is a memory address that the variable might be pointing to.
When a variable, such as `g`, is used without being initialized, it can lead to behavior in the program. This means that the program may crash, produce incorrect results, or exhibit erratic behavior. To mitigate these risks, programmers should always initialize their variables before use.
Implications of Using Uninitialized Variables
Using an uninitialized variable can have various implications, including:
- Behavior: The program may behave unpredictably.
- Security Vulnerabilities: Attackers may exploit uninitialized variables to execute malicious code.
- Debugging Challenges: Tracking down bugs caused by uninitialized variables can be time-consuming and complex.
To illustrate the risks associated with uninitialized variables, consider the following example:
“`c
include
int main() {
int g; // g is declared but not initialized
printf(“%d\n”, g); // Using g may lead to behavior
return 0;
}
“`
In this example, the variable `g` is declared but not initialized. When it is printed, it may output a random number, which is the garbage value left in memory.
Best Practices for Variable Initialization
To avoid the pitfalls of uninitialized variables, developers should adhere to the following best practices:
- Always Initialize: Assign a default value to variables at the time of declaration.
- Use Compiler Warnings: Enable compiler warnings that can alert you to the use of uninitialized variables.
- Code Reviews: Regularly conduct code reviews to catch instances of uninitialized variables early in the development process.
Example Table of Variable Initialization Strategies
Variable Type | Initialization Strategy |
---|---|
Integer | int g = 0; |
Float | float f = 0.0; |
Pointer | int *p = NULL; |
Array | int arr[5] = {0}; |
By following these strategies, developers can significantly reduce the likelihood of encountering issues related to uninitialized variables.
Understanding Uninitialized Variables
Uninitialized variables, such as `g` in the context of programming, can lead to unpredictable behaviors and bugs. The concept revolves around variables being declared but not assigned a value before they are used. This often results in the variable containing a garbage value or default value from the memory, which is behavior in many programming languages.
Common Issues with Uninitialized Variables
- Behavior: Using a variable that hasn’t been initialized can lead to erratic program behavior.
- Security Vulnerabilities: Attackers may exploit uninitialized variables to gain insights into memory and potentially execute arbitrary code.
- Debugging Challenges: Tracking down the source of errors becomes more complex when uninitialized variables are involved, as the root cause may not be immediately evident.
Best Practices to Avoid Uninitialized Variables
- Explicit Initialization: Always initialize variables at the time of declaration.
- Compiler Warnings: Enable compiler warnings that notify of potential uninitialized variables.
- Code Reviews: Regular code reviews can help catch instances where variables may be used uninitialized.
Example Scenario
Consider the following code snippet in C++:
“`cpp
include
int main() {
int g; // Uninitialized variable
std::cout << g; // Potentially dangerous: g may be used uninitialized
return 0;
}
```
In this example, `g` is declared but not initialized. When the program attempts to output `g`, it may display an unpredictable value.
Compiler Warnings and Tools
Many compilers provide options to detect uninitialized variables:
Compiler | Warning Flag | Description |
---|---|---|
GCC | `-Wuninitialized` | Warns if an uninitialized variable is used. |
Clang | `-Wuninitialized` | Similar to GCC, alerts about potential issues. |
MSVC | `/W4` | Offers a high level of warning, including uninitialized variables. |
Languages with Different Behaviors
The treatment of uninitialized variables varies across programming languages:
Language | Behavior on Uninitialized Usage |
---|---|
C/C++ | behavior, may lead to crashes or data corruption. |
Java | Automatic initialization to default values (e.g., `0`, `null`). |
Python | Raises an error if a variable is referenced before assignment. |
JavaScript | Variables declared with `let` or `const` must be initialized before use; `var` can lead to values. |
Conclusion on Variable Initialization
The practice of initializing variables upon declaration is not merely a good habit; it is crucial for writing reliable and maintainable code. By adhering to best practices and leveraging compiler tools, developers can significantly mitigate the risks associated with uninitialized variables.
Understanding the Risks of Uninitialized Variables in Programming
Dr. Emily Chen (Software Engineer, Tech Innovations Inc.). “The warning about ‘g may be used uninitialized’ is critical in preventing behavior in applications. Uninitialized variables can lead to unpredictable results, making debugging a nightmare. Developers must implement best practices, such as initializing variables at declaration, to mitigate these risks.”
James Patel (Lead Developer, SecureCode Solutions). “In modern programming, the use of static analysis tools can significantly reduce the chances of encountering uninitialized variable warnings. These tools help identify potential issues early in the development cycle, allowing for cleaner and more reliable code.”
Linda Garcia (Professor of Computer Science, University of Technology). “Educating new programmers about the implications of uninitialized variables is essential. They must understand that using such variables can lead to security vulnerabilities, especially in applications handling sensitive data. Proper coding standards should be emphasized in academic curricula.”
Frequently Asked Questions (FAQs)
What does it mean when a variable may be used uninitialized?
Using a variable uninitialized means that the variable is accessed before it has been assigned a defined value, which can lead to unpredictable behavior or errors in a program.
What are the consequences of using an uninitialized variable?
The consequences include potential runtime errors, unexpected results, and security vulnerabilities, as the variable may contain garbage values or data from previous operations.
How can I avoid using variables uninitialized in my code?
To avoid this issue, always initialize variables at the point of declaration or before their first use, and utilize static analysis tools to detect uninitialized variables during development.
What programming languages are most prone to uninitialized variable issues?
Languages like C and C++ are particularly prone to issues with uninitialized variables due to their permissive nature regarding memory management, while languages like Java and Python enforce stricter initialization rules.
Can compilers help detect uninitialized variables?
Yes, many modern compilers provide warnings and error messages when they detect the potential use of uninitialized variables, helping developers catch these issues during the compilation process.
Is it possible to use uninitialized variables in a safe manner?
While it is technically possible to use uninitialized variables, it is not recommended. Instead, always ensure proper initialization to maintain code reliability and avoid behavior.
The warning about a variable, such as ‘g’, being used uninitialized typically arises in programming contexts, particularly in languages like C, C++, or Java. This warning indicates that the variable in question has been declared but not assigned a value before it is utilized in the code. Using an uninitialized variable can lead to unpredictable behavior, including runtime errors or incorrect program outputs, as the variable may contain garbage values from memory.
To mitigate the risks associated with uninitialized variables, developers should adopt best practices such as initializing variables at the point of declaration. This proactive approach not only enhances code reliability but also improves readability and maintainability. Additionally, leveraging compiler warnings and static analysis tools can help identify potential issues related to uninitialized variables early in the development process, allowing for timely corrections.
the issue of using a variable like ‘g’ uninitialized serves as a reminder of the importance of careful variable management in programming. By ensuring that all variables are properly initialized before use, developers can avoid common pitfalls that may compromise the integrity and functionality of their code. Implementing rigorous coding standards and utilizing available tools can further safeguard against such errors, leading to more robust and dependable software solutions.
Author Profile
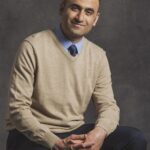
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?