How Can You Retrieve Ad Group Members Using PowerShell?
In the ever-evolving landscape of IT management, PowerShell has emerged as an indispensable tool for system administrators and developers alike. Its powerful scripting capabilities allow users to automate complex tasks, streamline workflows, and manage resources efficiently. One common task that many administrators face is retrieving information about Active Directory (AD) groups and their members. Whether you’re looking to audit group memberships, manage permissions, or simply gather data for reporting, knowing how to effectively get ad group members in PowerShell can save you time and enhance your productivity.
Understanding how to interact with Active Directory through PowerShell can seem daunting at first, but it opens up a world of possibilities for managing user accounts and group memberships. PowerShell provides a variety of cmdlets specifically designed for querying and manipulating AD objects, making it easier than ever to extract the information you need. From identifying group members to filtering results based on specific criteria, the flexibility of PowerShell allows for tailored solutions that meet your organization’s unique requirements.
As we delve deeper into the intricacies of retrieving AD group members using PowerShell, you’ll discover practical techniques and best practices that can enhance your scripting skills. Whether you’re a seasoned PowerShell user or just starting your journey, this guide will equip you with the knowledge and tools necessary to navigate the complexities of Active Directory management with
Retrieving Ad Group Members with PowerShell
To get members of an Active Directory (AD) group using PowerShell, you can utilize the `Get-ADGroupMember` cmdlet. This cmdlet is part of the Active Directory module, which is available when you have the Remote Server Administration Tools (RSAT) installed or on a Windows Server where the AD DS role is installed.
The basic syntax to retrieve group members is as follows:
“`powershell
Get-ADGroupMember -Identity “GroupName”
“`
In this command, replace `”GroupName”` with the name of the AD group whose members you want to retrieve.
Filtering Results
You can filter the results to display specific properties or types of members. For instance, if you are only interested in user accounts, you can pipe the results to the `Where-Object` cmdlet.
Example:
“`powershell
Get-ADGroupMember -Identity “GroupName” | Where-Object { $_.objectClass -eq “user” }
“`
This command retrieves members of the specified group and filters the output to show only users.
Displaying Specific Properties
To display specific properties of the members, you can use the `Select-Object` cmdlet. For example, if you want to retrieve the names and email addresses of the group members, you can run:
“`powershell
Get-ADGroupMember -Identity “GroupName” | Get-ADUser -Property EmailAddress | Select-Object Name, EmailAddress
“`
This command first gets the group members, retrieves their user properties, and then selects the desired fields for display.
Exporting Results
In many scenarios, you might want to export the list of group members to a file for reporting or auditing purposes. PowerShell makes this easy with the `Export-Csv` cmdlet. For example:
“`powershell
Get-ADGroupMember -Identity “GroupName” | Get-ADUser -Property EmailAddress | Select-Object Name, EmailAddress | Export-Csv -Path “C:\GroupMembers.csv” -NoTypeInformation
“`
This command will create a CSV file containing the names and email addresses of the group members.
Example of Group Member Retrieval
Here’s a concise example demonstrating the entire process:
“`powershell
Define the group name
$groupName = “Marketing”
Get members of the group and export to CSV
Get-ADGroupMember -Identity $groupName | Get-ADUser -Property EmailAddress | Select-Object Name, EmailAddress | Export-Csv -Path “C:\MarketingGroupMembers.csv” -NoTypeInformation
“`
This script retrieves the members of the “Marketing” group and exports their names and email addresses to a CSV file.
Property | Description |
---|---|
Name | The display name of the user or group member. |
EmailAddress | The email address associated with the user account. |
objectClass | The type of the AD object (e.g., user, group). |
These commands and techniques provide a robust framework for managing and retrieving information about AD group memberships through PowerShell.
PowerShell Command to Retrieve Ad Group Members
To retrieve Active Directory (AD) group members using PowerShell, the `Get-ADGroupMember` cmdlet is employed. This cmdlet allows administrators to extract a list of members from a specified AD group. The following outlines the syntax and examples of usage.
Basic Syntax
“`powershell
Get-ADGroupMember -Identity
“`
- `-Identity`: This parameter specifies the group from which you want to retrieve members. You can provide either the group name or its distinguished name (DN).
Examples
- To get members of a group by name:
“`powershell
Get-ADGroupMember -Identity “Sales Team”
“`
- To get members using the group’s distinguished name:
“`powershell
Get-ADGroupMember -Identity “CN=Sales Team,OU=Groups,DC=example,DC=com”
“`
Filtering Results
You might want to filter the output to only show specific types of members, such as users or computers. The `Where-Object` cmdlet can be used for this purpose.
“`powershell
Get-ADGroupMember -Identity “Sales Team” | Where-Object { $_.objectClass -eq “user” }
“`
- This command retrieves only user accounts from the “Sales Team” group.
Output Formatting
To format the output, you can use `Select-Object` to display specific properties of the members.
“`powershell
Get-ADGroupMember -Identity “Sales Team” | Select-Object Name, SamAccountName, objectClass
“`
This will output a list of members with their names, SAM account names, and object classes.
Exporting to CSV
For reporting purposes, you may need to export the list of group members to a CSV file. This can be done easily with the `Export-Csv` cmdlet.
“`powershell
Get-ADGroupMember -Identity “Sales Team” | Select-Object Name, SamAccountName | Export-Csv -Path “C:\Reports\SalesTeamMembers.csv” -NoTypeInformation
“`
- `-NoTypeInformation`: This parameter prevents PowerShell from adding type information to the CSV file.
Recursive Group Membership
To retrieve members of a group, including nested group members, you can use the `-Recursive` switch.
“`powershell
Get-ADGroupMember -Identity “Sales Team” -Recursive
“`
This command provides a comprehensive list of all members within the “Sales Team” group and any sub-groups.
Handling Errors
When working with AD groups, it is essential to handle potential errors. You can use `Try-Catch` blocks to manage exceptions gracefully.
“`powershell
Try {
Get-ADGroupMember -Identity “Sales Team”
} Catch {
Write-Host “An error occurred: $_”
}
“`
This structure ensures that if there is an error in retrieving group members, it will be caught and displayed without terminating the script abruptly.
Utilizing the `Get-ADGroupMember` cmdlet in PowerShell provides a powerful means to manage and retrieve information about Active Directory group memberships effectively.
Expert Insights on Retrieving Ad Group Members in PowerShell
Dr. Emily Carter (Senior IT Consultant, Cloud Solutions Inc.). “Utilizing PowerShell to get ad group members is an efficient method for managing user accounts. The command ‘Get-ADGroupMember’ is essential for extracting user details, and understanding its parameters can enhance your scripting capabilities significantly.”
Mark Thompson (PowerShell Automation Specialist, Tech Innovations). “When working with Active Directory, leveraging PowerShell not only streamlines workflows but also minimizes errors. The ‘Get-ADGroupMember’ cmdlet allows administrators to easily list group members, which is crucial for maintaining security and compliance within an organization.”
Linda Zhang (Cybersecurity Analyst, SecureTech Solutions). “In today’s security landscape, managing group memberships effectively is vital. PowerShell provides a robust framework for querying Active Directory, and mastering the ‘Get-ADGroupMember’ command can significantly aid in auditing and monitoring user access within your network.”
Frequently Asked Questions (FAQs)
How can I get ad group members using PowerShell?
You can retrieve Active Directory group members by using the `Get-ADGroupMember` cmdlet in PowerShell. For example, use the command `Get-ADGroupMember -Identity “GroupName”` to list all members of the specified group.
Do I need special permissions to run the Get-ADGroupMember cmdlet?
Yes, you must have sufficient permissions in Active Directory to query group members. Typically, being a member of the Domain Users group is sufficient, but specific permissions may vary based on your organization’s policies.
Can I filter the results of Get-ADGroupMember?
Yes, you can filter results using the `Where-Object` cmdlet. For example, `Get-ADGroupMember -Identity “GroupName” | Where-Object { $_.ObjectClass -eq “user” }` will return only user accounts from the group.
Is it possible to export the list of group members to a CSV file?
Yes, you can export the list using the `Export-Csv` cmdlet. For example, `Get-ADGroupMember -Identity “GroupName” | Export-Csv -Path “GroupMembers.csv” -NoTypeInformation` will save the output to a CSV file.
Can I get additional details about each group member?
Yes, you can retrieve additional properties by using the `Get-ADUser` cmdlet in combination with `Get-ADGroupMember`. For example, `Get-ADGroupMember -Identity “GroupName” | Get-ADUser -Properties DisplayName, EmailAddress` will provide more detailed information about each member.
What if I want to get members from a nested group?
To retrieve members from nested groups, you can use the `-Recursive` parameter with the `Get-ADGroupMember` cmdlet. For instance, `Get-ADGroupMember -Identity “GroupName” -Recursive` will include members from any nested groups.
In summary, retrieving ad group members using PowerShell is a straightforward process that can significantly enhance administrative efficiency in managing Active Directory environments. By utilizing the appropriate cmdlets, such as `Get-ADGroupMember`, administrators can easily list all members of a specified Active Directory group. This capability is essential for maintaining accurate records and ensuring that group memberships align with organizational policies.
Moreover, leveraging PowerShell for this task allows for greater flexibility and automation. Administrators can filter results, export data to various formats, and integrate these commands into larger scripts to automate routine tasks. This not only saves time but also reduces the likelihood of human error in managing group memberships.
mastering the use of PowerShell to get ad group members is a valuable skill for IT professionals. It empowers them to efficiently manage user accounts and group memberships, thereby enhancing overall organizational security and compliance. As organizations continue to rely on Active Directory for user management, proficiency in these PowerShell commands will remain an essential asset for system administrators.
Author Profile
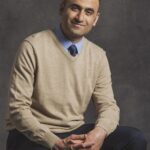
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?