How Can You Extract the Body from a SOAP XML Message?
In the world of web services, SOAP (Simple Object Access Protocol) plays a pivotal role in enabling communication between applications over the internet. While SOAP messages are structured in XML, extracting meaningful information from these messages can often be a daunting task, especially for those new to the technology. If you’ve ever found yourself sifting through complex XML structures, trying to locate the body of a SOAP message, you’re not alone. Understanding how to effectively retrieve the body from SOAP XML is essential for developers and IT professionals who work with web services, as it allows for seamless data exchange and integration between disparate systems.
At its core, a SOAP message is composed of a header and a body, with the body containing the actual data being transmitted. However, the intricacies of XML formatting can obscure this information, making it challenging to access the content you need. Fortunately, there are various methods and tools available that simplify this process, allowing users to parse SOAP messages efficiently. Whether you’re working with programming languages like Python, Java, or utilizing specific libraries designed for XML manipulation, knowing how to extract the body from SOAP XML can enhance your ability to interact with web services effectively.
As we delve deeper into this topic, we’ll explore the different approaches to accessing the body of a SOAP message, including practical examples
Extracting the Body from SOAP XML
To extract the body from a SOAP XML message, one must understand the structure of SOAP messages, which typically consist of an envelope, header, and body. The body is where the actual message content resides, often formatted as XML. Below is a step-by-step guide on how to programmatically access the body of a SOAP message using various programming languages.
SOAP Message Structure
A typical SOAP message structure includes:
- Envelope: The root element that defines the XML document as a SOAP message.
- Header: Contains optional attributes of the message, such as authentication information.
- Body: Contains the actual message intended for the recipient.
Here is an example of a SOAP message:
“`xml
“`
Accessing the Body in Different Programming Languages
Extracting the body from a SOAP message varies depending on the programming language used. Below are examples for common languages.
Using Python
In Python, the `xml.etree.ElementTree` module can be utilized to parse the SOAP XML and extract the body.
“`python
import xml.etree.ElementTree as ET
soap_message = “””
root = ET.fromstring(soap_message)
body = root.find(‘{http://schemas.xmlsoap.org/soap/envelope/}Body’)
print(ET.tostring(body, encoding=’unicode’))
“`
Using Java
In Java, you can use the `DocumentBuilder` and `XPath` classes to extract the body.
“`java
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import javax.xml.xpath.XPath;
import javax.xml.xpath.XPathFactory;
import org.w3c.dom.Document;
import org.w3c.dom.Node;
String soapMessage = “
+ “
+ “
+ “
+ “
+ “
+ “
+ “
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document doc = builder.parse(new InputSource(new StringReader(soapMessage)));
XPath xpath = XPathFactory.newInstance().newXPath();
Node body = (Node) xpath.evaluate(“/soapenv:Envelope/soapenv:Body”, doc, XPathConstants.NODE);
“`
Using C
In C, you can use the `System.Xml` namespace to parse the XML.
“`csharp
using System;
using System.Xml;
string soapMessage = @”
XmlDocument doc = new XmlDocument();
doc.LoadXml(soapMessage);
XmlNode body = doc.SelectSingleNode(“//soapenv:Body”);
Console.WriteLine(body.OuterXml);
“`
Common Challenges
While extracting the body from SOAP XML is generally straightforward, some challenges may arise:
- Namespaces: SOAP messages often use namespaces that must be correctly referenced in XPath queries.
- Error Handling: Proper error handling should be implemented to manage parsing issues or unexpected message formats.
To summarize, extracting the body from SOAP XML can be effectively accomplished using various programming languages by understanding the message structure and employing appropriate parsing techniques.
Extracting the Body from SOAP XML
To extract the body from a SOAP XML message, it is essential to understand the structure of the SOAP envelope. The SOAP message typically consists of the following components:
- Envelope: The root element that defines the XML document as a SOAP message.
- Header: An optional element that contains application-specific information.
- Body: A mandatory element that contains the actual message intended for the recipient.
The body is usually where the main content or data is found, and it is critical for processing the request or response. Below is a breakdown of how to extract the body from a SOAP XML.
SOAP XML Structure
A typical SOAP message structure looks like this:
“`xml
“`
Methods to Extract the Body
There are various methods to extract the body from a SOAP XML document, depending on the programming language and libraries in use. Below are examples in popular languages.
Using Python with `xml.etree.ElementTree`
“`python
import xml.etree.ElementTree as ET
Sample SOAP XML
soap_xml = ”’
Parse the XML
root = ET.fromstring(soap_xml)
Extract the Body
body = root.find(‘{http://schemas.xmlsoap.org/soap/envelope/}Body’)
print(ET.tostring(body, encoding=’unicode’))
“`
Using Java with `javax.xml.parsers`
“`java
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import org.w3c.dom.Document;
import org.w3c.dom.NodeList;
public class SOAPExtractor {
public static void main(String[] args) throws Exception {
String soapXml = “
DocumentBuilderFactory factory = DocumentBuilderFactory.newInstance();
DocumentBuilder builder = factory.newDocumentBuilder();
Document doc = builder.parse(new InputSource(new StringReader(soapXml)));
NodeList body = doc.getElementsByTagNameNS(“http://schemas.xmlsoap.org/soap/envelope/”, “Body”);
System.out.println(body.item(0).toString());
}
}
“`
Using JavaScript with `DOMParser`
“`javascript
const soapXml = `
const parser = new DOMParser();
const xmlDoc = parser.parseFromString(soapXml, “text/xml”);
const body = xmlDoc.getElementsByTagName(“Body”)[0];
console.log(new XMLSerializer().serializeToString(body));
“`
Common Pitfalls
When extracting the body from SOAP XML, consider the following:
- Namespaces: Be aware of the XML namespaces; they must be correctly referenced in the extraction logic.
- Error Handling: Implement error handling to manage malformed XML or missing elements.
- Encoding Issues: Ensure that the encoding of the XML string is compatible with the parser used.
By using the above methods and considerations, the extraction of the body from a SOAP XML message can be efficiently accomplished.
Extracting the Body from SOAP XML: Expert Insights
Dr. Emily Carter (Lead Software Engineer, Web Services Innovations). “To effectively retrieve the body from a SOAP XML message, one must utilize an XML parser that can navigate the structured elements of the SOAP envelope. This process typically involves accessing the ‘Body’ node within the XML hierarchy, which contains the actual payload of the message.”
Michael Thompson (Senior Integration Specialist, Tech Solutions Group). “When working with SOAP XML, it is crucial to understand the namespaces and structure defined in the WSDL. By leveraging libraries such as JAXB in Java or lxml in Python, developers can easily extract the body content for further processing, ensuring compliance with the SOAP protocol.”
Sarah Patel (XML Data Analyst, Data Insights Corp). “The extraction of the body from a SOAP XML document is not merely a technical task; it requires a comprehensive understanding of the underlying data model. Using XPath expressions can greatly simplify the extraction process, allowing for precise targeting of the desired elements within the SOAP body.”
Frequently Asked Questions (FAQs)
What is the structure of a SOAP XML message?
A SOAP XML message consists of an Envelope, Header, and Body. The Envelope defines the message structure, the Header contains optional metadata, and the Body contains the actual message content or data being transmitted.
How can I extract the body from a SOAP XML message?
To extract the body from a SOAP XML message, you can use an XML parser to navigate to the `
What programming languages can be used to parse SOAP XML?
Common programming languages for parsing SOAP XML include Python, Java, C, and PHP. Each language offers libraries and tools that facilitate XML parsing and manipulation.
Are there any tools available for working with SOAP XML?
Yes, several tools are available for working with SOAP XML, including SoapUI for testing, Postman for API requests, and various IDE plugins that assist in generating and parsing SOAP messages.
What are common issues when extracting the body from SOAP XML?
Common issues include improperly formatted XML, namespaces that complicate element access, and variations in SOAP versions (1.1 vs. 1.2) that may affect parsing logic.
Can I use XPath to retrieve the body from a SOAP XML message?
Yes, XPath can be effectively used to retrieve the body from a SOAP XML message. You can use an XPath expression like `//soap:Body` to directly access the Body element, considering the appropriate namespace.
In summary, extracting the body from a SOAP XML message is a fundamental task in web services communication. SOAP, which stands for Simple Object Access Protocol, utilizes XML to structure its messages, consisting of an envelope, header, and body. The body of the SOAP message contains the actual data or the payload intended for the recipient, making it crucial for the processing of requests and responses in service-oriented architectures.
To effectively retrieve the body from a SOAP XML message, developers often utilize various programming languages and libraries that facilitate XML parsing. For instance, in languages like Java, Python, or C, there are built-in libraries and third-party tools that can simplify the extraction process. Understanding the structure of the SOAP message and employing the right parsing techniques are essential for ensuring accurate data retrieval and manipulation.
Key takeaways include the importance of familiarizing oneself with the SOAP message structure and utilizing appropriate XML parsing methods. Additionally, being aware of the nuances in different programming environments can enhance efficiency in handling SOAP messages. Ultimately, mastering the extraction of the body from SOAP XML is vital for developers working with web services, as it directly impacts the functionality and reliability of the applications they build.
Author Profile
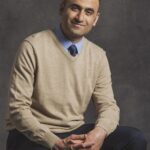
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?