How Can I Retrieve the Maximum Date in SQL?
In the world of database management, the ability to retrieve specific data efficiently is paramount. One common requirement that arises in various applications is the need to identify the maximum date from a dataset. Whether you’re tracking user registrations, sales transactions, or event timestamps, knowing how to extract the latest date can provide critical insights into trends and performance. In this article, we will delve into the methods and best practices for obtaining the maximum date in SQL, empowering you to enhance your data querying skills.
SQL, or Structured Query Language, is the standard language for managing and manipulating relational databases. Among its many powerful functions, the ability to aggregate data is particularly useful. When it comes to dates, SQL offers straightforward functions that allow users to pinpoint the most recent entry in a dataset. This capability not only aids in reporting but also plays a crucial role in data analysis, enabling businesses to make informed decisions based on the latest information available.
As we explore the various techniques for fetching the maximum date, we will cover essential SQL functions, syntax variations, and practical examples. Whether you’re a novice looking to understand the basics or an experienced developer seeking to refine your skills, this guide will equip you with the knowledge needed to navigate date queries with confidence and precision. Get ready to unlock the potential of your SQL queries and
Using MAX Function to Get Maximum Date
To retrieve the maximum date from a date column in SQL, the `MAX()` function is commonly used. This function aggregates the values in a specified column and returns the highest value. When working with date data types, `MAX()` will return the latest date.
The basic syntax for using the `MAX()` function is as follows:
“`sql
SELECT MAX(date_column) AS MaxDate
FROM table_name;
“`
Here, `date_column` refers to the column containing date values, and `table_name` is the name of the table from which data is being retrieved.
Example of MAX Function with GROUP BY
In scenarios where you want to find the maximum date for each group of records, the `MAX()` function can be combined with the `GROUP BY` clause. This allows you to aggregate data based on specific criteria.
For instance, consider a sales table that records transactions with dates and regions. To find the latest transaction date for each region, you would use:
“`sql
SELECT region, MAX(transaction_date) AS LatestTransactionDate
FROM sales
GROUP BY region;
“`
This query will return the most recent transaction date for each region.
Combining MAX with Other Clauses
The `MAX()` function can also be utilized alongside other SQL clauses such as `JOIN`, `WHERE`, and `ORDER BY` to refine your data retrieval.
- WHERE Clause: Filter records before applying the `MAX()` function.
- JOIN Clause: Retrieve maximum dates from related tables.
- ORDER BY Clause: Sort results based on specific criteria.
For example, to find the maximum transaction date for a specific customer, you could write:
“`sql
SELECT MAX(transaction_date) AS MaxTransactionDate
FROM sales
WHERE customer_id = 123;
“`
Table Example of MAX Function in Use
Below is a sample table illustrating how the `MAX()` function retrieves the latest dates:
Region | Transaction Date |
---|---|
North | 2023-01-15 |
South | 2023-03-22 |
East | 2023-02-10 |
West | 2023-04-05 |
Using the previous `GROUP BY` example, the output would be:
Region | Latest Transaction Date |
---|---|
North | 2023-01-15 |
South | 2023-03-22 |
East | 2023-02-10 |
West | 2023-04-05 |
In this example, the `MAX()` function would return the latest transaction date for each region, showcasing the power of SQL in data analysis and retrieval.
Using SQL to Get the Maximum Date
To retrieve the maximum date from a column in SQL, the `MAX()` function is utilized. This function is designed to return the largest value from a specified column, which can include date fields. The basic syntax is as follows:
“`sql
SELECT MAX(date_column) AS max_date
FROM table_name;
“`
Example Query
Consider a table named `orders` that contains a column `order_date`. To find the latest order date, the SQL query would be:
“`sql
SELECT MAX(order_date) AS max_order_date
FROM orders;
“`
Grouping Results
If you wish to find the maximum date for different groups, the `GROUP BY` clause can be employed. For example, if you want to find the latest order date for each customer, you would use:
“`sql
SELECT customer_id, MAX(order_date) AS max_order_date
FROM orders
GROUP BY customer_id;
“`
Handling Null Values
When dealing with dates, it is essential to consider null values. The `MAX()` function will ignore nulls, but if you need to ensure that the query returns a result even if all values are null, you can use the `COALESCE()` function:
“`sql
SELECT COALESCE(MAX(order_date), ‘No orders found’) AS max_order_date
FROM orders;
“`
Using `ORDER BY` for Maximum Dates
If you need to retrieve additional information along with the maximum date, you can combine `ORDER BY` with a `LIMIT` clause to get the entire row corresponding to the maximum date:
“`sql
SELECT *
FROM orders
ORDER BY order_date DESC
LIMIT 1;
“`
Example with Multiple Tables
In scenarios where you need to join tables to get the maximum date, the following example demonstrates how to do this. If there is a `customers` table linked to the `orders` table, you can find the latest order date for each customer:
“`sql
SELECT c.customer_id, MAX(o.order_date) AS max_order_date
FROM customers c
JOIN orders o ON c.customer_id = o.customer_id
GROUP BY c.customer_id;
“`
Performance Considerations
When querying large datasets, consider indexing the date column to improve performance. An index can speed up the retrieval of maximum values significantly, especially in large tables.
- Create an Index:
“`sql
CREATE INDEX idx_order_date ON orders(order_date);
“`
- Check Execution Plan: Always analyze the execution plan to ensure that the query is optimized.
Conclusion
The `MAX()` function in SQL provides a straightforward method to find the maximum date within a dataset. By leveraging this function along with grouping, null handling, and indexing strategies, you can efficiently retrieve the latest dates tailored to your specific requirements.
Expert Insights on Retrieving Maximum Dates in SQL
Maria Chen (Database Administrator, Tech Solutions Inc.). “To effectively retrieve the maximum date from a dataset in SQL, utilizing the MAX() function is essential. This function aggregates date values, ensuring that the latest entry is returned efficiently, which is crucial for time-sensitive applications.”
James Patel (Senior SQL Developer, Data Insights Group). “When querying for the maximum date, it is important to consider the context of your dataset. Using GROUP BY in conjunction with MAX() can yield more meaningful results, especially when dealing with multiple categories or groups within your data.”
Linda Garcia (Data Analyst, Analytics Hub). “In scenarios where duplicate maximum dates may exist, employing a subquery can help isolate unique records. This approach not only retrieves the maximum date but also provides the associated data, enhancing the overall analysis.”
Frequently Asked Questions (FAQs)
How can I retrieve the maximum date from a SQL table?
To retrieve the maximum date from a SQL table, use the `MAX()` function in your query. For example: `SELECT MAX(date_column) FROM table_name;`. This will return the latest date in the specified column.
What SQL function is used to find the maximum date?
The `MAX()` function is used to find the maximum date in SQL. It can be applied to date columns to return the most recent date entry.
Can I use the MAX() function with other conditions?
Yes, you can use the `MAX()` function with conditions by incorporating a `WHERE` clause in your query. For example: `SELECT MAX(date_column) FROM table_name WHERE condition;`.
Is it possible to get the maximum date grouped by another column?
Yes, you can group results by another column while retrieving the maximum date. Use the `GROUP BY` clause, like this: `SELECT another_column, MAX(date_column) FROM table_name GROUP BY another_column;`.
What happens if there are NULL values in the date column?
The `MAX()` function ignores NULL values when calculating the maximum date. It only considers non-NULL entries in the specified column.
Can I find the maximum date in a specific range?
Yes, you can find the maximum date within a specific range by using a `WHERE` clause to filter the dates. For example: `SELECT MAX(date_column) FROM table_name WHERE date_column BETWEEN ‘start_date’ AND ‘end_date’;`.
In SQL, retrieving the maximum date from a dataset is a common operation that can be accomplished using the `MAX()` aggregate function. This function is designed to return the highest value from a specified column, which is particularly useful when working with date fields. By applying `MAX()` to a date column within a `SELECT` statement, users can efficiently identify the most recent date in their dataset, which can be critical for reporting and analysis purposes.
To effectively utilize the `MAX()` function, it is important to structure the SQL query correctly. A typical query would look like `SELECT MAX(date_column) FROM table_name;`, where `date_column` represents the column containing the date values, and `table_name` is the name of the table being queried. Additionally, it is possible to combine this function with other SQL clauses, such as `GROUP BY`, to find maximum dates within specific groups or categories.
Understanding how to retrieve maximum dates in SQL not only enhances data analysis capabilities but also improves decision-making processes. By leveraging this function, users can quickly ascertain timelines, track progress, and make informed predictions based on the most current data available. Overall, mastering the use of `MAX()` in SQL is an essential skill for anyone working
Author Profile
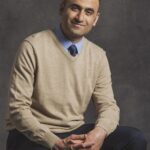
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?