How Can I Retrieve Product Attributes in catalog-add-to-cart.js in Magento 2?
In the ever-evolving world of eCommerce, Magento 2 stands out as a powerful platform that empowers businesses to create dynamic online stores. Among its many features, the ability to manage product attributes effectively can significantly enhance the shopping experience for customers. One critical aspect of this is understanding how to manipulate product attributes within the `catalog-add-to-cart.js` file. This JavaScript file plays a vital role in the functionality of the shopping cart, ensuring that products are added seamlessly while retaining all necessary attribute data.
Navigating the intricacies of Magento 2 can be daunting, especially when it comes to customizing the add-to-cart functionality. Product attributes, which include vital information such as size, color, and other specifications, are essential for providing customers with the details they need to make informed purchasing decisions. By delving into the `catalog-add-to-cart.js` file, developers can unlock the potential to tailor the shopping experience, ensuring that the right attributes are captured and processed during the add-to-cart action.
Understanding how to retrieve and utilize product attributes in this context not only enhances the functionality of your Magento store but also contributes to a more personalized customer experience. As we explore the methods and best practices for accessing these attributes, you’ll gain valuable insights into optimizing your store’s performance and improving
Understanding Product Attributes in Magento 2’s JavaScript
In Magento 2, the `catalog-add-to-cart.js` file plays a crucial role in handling the add-to-cart functionality for products within the catalog. To effectively manage product attributes during this process, developers need to access these attributes through JavaScript. This can be achieved by utilizing Magento’s built-in data structures and methods, ensuring that the correct attributes are captured and processed.
To retrieve product attributes, you typically interact with the `product` object, which contains all necessary information about a product. Here’s how you can effectively get product attributes in `catalog-add-to-cart.js`:
- Accessing the Product Object: Ensure that the product data is available on the page, usually injected via a Magento block or a JSON data structure. The product object will typically look like this:
“`javascript
var product = {
id: 123,
sku: ‘ABC-123’,
name: ‘Sample Product’,
attributes: {
color: ‘Red’,
size: ‘M’
}
};
“`
- Retrieving Specific Attributes: You can access specific attributes directly from the product object. For example, to get the color and size:
“`javascript
var productColor = product.attributes.color;
var productSize = product.attributes.size;
“`
Example: Extracting Attributes in Add-to-Cart Functionality
When implementing the add-to-cart functionality, it’s essential to collect the necessary product attributes to ensure the correct product variant is added to the cart. Below is a simplified example of how to extract and use these attributes:
“`javascript
var addToCart = function(product) {
var productAttributes = {
color: product.attributes.color,
size: product.attributes.size
};
// Logic to add the product to the cart
console.log(“Adding to cart:”, product.name, “with attributes:”, productAttributes);
};
“`
This function illustrates how to gather attributes before executing the add-to-cart action.
Handling Multiple Attributes
In scenarios where products have multiple attributes, it’s beneficial to iterate over the attributes dynamically. This can be accomplished using a loop to ensure that you capture all relevant data.
“`javascript
var extractAttributes = function(attributes) {
var extractedAttributes = {};
for (var key in attributes) {
if (attributes.hasOwnProperty(key)) {
extractedAttributes[key] = attributes[key];
}
}
return extractedAttributes;
};
var productAttributes = extractAttributes(product.attributes);
console.log(productAttributes);
“`
This approach allows for flexibility, accommodating any number of attributes without hardcoding them.
Table of Common Product Attributes
Below is a table summarizing common product attributes that may be useful when implementing the add-to-cart functionality.
Attribute | Description |
---|---|
SKU | Stock Keeping Unit, a unique identifier for each product. |
Name | The name of the product displayed to customers. |
Price | The selling price of the product. |
Color | The color attribute representing the product’s color. |
Size | The size attribute representing the product’s size. |
By understanding how to access and utilize product attributes within `catalog-add-to-cart.js`, developers can enhance the user experience by ensuring that customers can add the correct product variants to their cart seamlessly.
Accessing Product Attributes in catalog-add-to-cart.js
To retrieve product attributes in the `catalog-add-to-cart.js` file within Magento 2, you will need to utilize the existing JavaScript mechanisms provided by the framework. Magento 2 uses a combination of Knockout.js and RequireJS for handling frontend functionalities, including retrieving product attributes.
Understanding the Context
The `catalog-add-to-cart.js` file typically handles the logic for adding products to the cart. When dealing with product attributes, it is crucial to understand where and how these attributes are stored and how they can be accessed during the add-to-cart process.
Key Steps to Retrieve Attributes
- Loading the Product Data:
- Ensure that the product data is loaded into the view model. This can be done using the `Magento_Catalog/js/view/product/view.js` file, where product attributes are often initialized.
- Accessing the Product Object:
- Inside `catalog-add-to-cart.js`, you can access the product object, which contains all the necessary attributes. Look for a method that initializes or fetches product details.
- Using Observables:
- Magento 2 utilizes Knockout.js for data binding. Make sure to use observables to properly bind the product attributes to the UI. For example:
“`javascript
var productAttributes = this.product().customAttributes;
“`
Example Code Snippet
Here is an example of how you might retrieve a specific product attribute:
“`javascript
define([
‘jquery’,
‘Magento_Catalog/js/model/product/add-to-cart’,
‘Magento_Catalog/js/model/product/view’
], function ($, addToCart, productView) {
‘use strict’;
return function (product) {
var productAttributes = product.customAttributes;
if (productAttributes) {
// Example of accessing a specific attribute
var attributeValue = productAttributes.find(attr => attr.attribute_code === ‘color’).value;
console.log(‘Selected Color: ‘ + attributeValue);
}
// Add logic to handle adding the product to the cart
addToCart(product);
};
});
“`
Commonly Used Attributes
When working with product attributes, you might frequently access the following:
- SKU: Stock Keeping Unit of the product.
- Name: The product name.
- Price: The product price.
- Custom Attributes: Any additional attributes defined in the product configuration.
Attribute Code | Description |
---|---|
sku | Unique identifier for the product |
name | Display name of the product |
price | Product price |
color | Color attribute |
size | Size attribute |
Handling Different Product Types
Different product types (simple, configurable, grouped) may have different ways of handling attributes. Ensure that your code checks the product type before attempting to access attributes.
- Configurable Products: May require fetching selected attribute values based on user selection.
- Simple Products: Attributes can be directly accessed since they are less complex.
Utilizing these practices will help you effectively retrieve product attributes in the `catalog-add-to-cart.js` file and ensure that your add-to-cart functionality operates smoothly within Magento 2’s architecture.
Expert Insights on Accessing Product Attributes in Magento 2
Emily Chen (Magento Developer, E-Commerce Solutions Inc.). “To effectively retrieve product attributes in the catalog-add-to-cart.js file, developers should utilize Magento’s built-in JavaScript components. Specifically, leveraging the `Magento_Catalog/js/model/product` module allows for seamless access to product data, ensuring that attributes are dynamically pulled based on the selected product.”
James Patel (Senior E-Commerce Architect, Digital Commerce Agency). “Understanding the structure of the product object in Magento 2 is crucial. By referencing the `data` attribute within the `add-to-cart` function, developers can extract specific product attributes such as SKU, price, and custom options, which can then be utilized for enhancing the user experience during the checkout process.”
Lisa Tran (Magento Certified Solution Specialist, E-Store Innovations). “When working with catalog-add-to-cart.js, it’s essential to ensure that the product attributes are properly configured in the backend. This guarantees that the JavaScript can access and manipulate these attributes without encountering issues, ultimately leading to a smoother integration of product data into the shopping cart functionality.”
Frequently Asked Questions (FAQs)
How can I access product attributes in catalog-add-to-cart.js in Magento 2?
You can access product attributes by using the `product` object available in the `catalog-add-to-cart.js` file. The attributes can be retrieved through `product.custom_attributes` or directly from `product` if they are standard attributes.
What are the common product attributes available in Magento 2?
Common product attributes in Magento 2 include `name`, `sku`, `price`, `description`, `short_description`, and `custom_attributes` which may contain additional data defined in the product settings.
Can I add custom attributes to the add-to-cart functionality?
Yes, you can add custom attributes by extending the product model and ensuring that these attributes are included in the product data sent to the front end. Modify the `catalog-add-to-cart.js` to handle these attributes accordingly.
Where can I find the catalog-add-to-cart.js file in Magento 2?
The `catalog-add-to-cart.js` file is located in the `vendor/magento/module-catalog/view/frontend/web/js` directory. You can also find it under your theme’s directory if it has been overridden.
How do I modify the add-to-cart functionality to include additional product attributes?
To modify the functionality, extend the `Magento_Catalog/js/catalog-add-to-cart` module, and override the necessary methods to include your custom attributes in the data being processed during the add-to-cart action.
Is it necessary to use a custom module to modify product attributes in catalog-add-to-cart.js?
While it is not strictly necessary, creating a custom module is recommended for maintainability and to avoid conflicts with future updates. This approach allows for cleaner code management and easier updates.
In Magento 2, the `catalog-add-to-cart.js` file plays a crucial role in managing the add-to-cart functionality for products within the catalog. This JavaScript file is responsible for handling user interactions and AJAX requests related to adding products to the shopping cart. Understanding how to retrieve product attributes in this context is essential for developers looking to customize or enhance the shopping experience.
To get product attributes in `catalog-add-to-cart.js`, developers typically utilize Magento’s JavaScript components and the product’s data structure. Attributes can be accessed through the product object, which is usually populated with relevant data when the product is rendered on the page. By leveraging the Magento 2 framework’s built-in methods and observables, developers can efficiently retrieve and manipulate product attributes as needed.
Key takeaways include the importance of understanding the Magento 2 architecture, particularly how JavaScript interacts with PHP backend processes. Developers should familiarize themselves with the product data structure and the various methods available for accessing and modifying product attributes. This knowledge not only aids in customizing the add-to-cart functionality but also enhances the overall user experience by providing relevant product information dynamically.
Author Profile
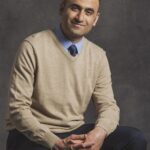
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?