How Can I Retrieve Residuals Information in Statsmodels Using Python?
In the realm of statistical modeling, understanding the nuances of your model’s performance is crucial for drawing accurate conclusions. One of the most insightful ways to evaluate a model is by analyzing its residuals—the differences between observed and predicted values. In Python, the `statsmodels` library offers robust tools for not only fitting various statistical models but also for extracting and interpreting residuals. Whether you’re a seasoned data scientist or a budding statistician, mastering how to get residuals information using `statsmodels` can significantly enhance your analytical capabilities.
Residuals serve as a window into the effectiveness of your model, revealing patterns that may indicate whether your assumptions hold true. With `statsmodels`, you can easily access residuals after fitting a model, allowing you to perform diagnostic checks and validate the underlying assumptions of linearity, homoscedasticity, and normality. This article will guide you through the process of obtaining and interpreting residuals, empowering you to make informed decisions based on your model’s performance.
As we delve deeper, we will explore the various methods available in `statsmodels` for retrieving residuals, along with visualizations that can help illuminate potential issues within your model. By the end of this journey, you’ll not only know how to extract residuals but also
Accessing Residuals in Statsmodels
In Statsmodels, residuals can be easily accessed after fitting a model. Residuals represent the difference between the observed values and the values predicted by the model. This information is crucial for diagnosing the fit of the model and understanding the underlying data. After fitting a model using the `fit()` method, you can retrieve residuals through the `resid` attribute.
For example:
“`python
import statsmodels.api as sm
Sample data
X = sm.add_constant(data[‘independent_variable’])
y = data[‘dependent_variable’]
Fit the model
model = sm.OLS(y, X).fit()
Access residuals
residuals = model.resid
“`
Analyzing Residuals
Analyzing residuals is vital for validating the assumptions of your regression model. The analysis can reveal whether the model is appropriate for the data. Key aspects to consider include:
- Normality: Residuals should be normally distributed.
- Homoscedasticity: The variance of residuals should be constant across all levels of the independent variables.
- Independence: Residuals should be independent of each other.
To assess these characteristics, you can use various graphical techniques such as:
- Residuals vs. Fitted plot
- Q-Q plot for normality
- Scale-Location plot for homoscedasticity
Visualizing Residuals
Visualizing residuals can provide insights into the model’s performance. Below is a simple example of how to create a residual plot using Matplotlib:
“`python
import matplotlib.pyplot as plt
plt.scatter(model.fittedvalues, residuals)
plt.axhline(0, color=’red’, linestyle=’–‘)
plt.xlabel(‘Fitted values’)
plt.ylabel(‘Residuals’)
plt.title(‘Residuals vs Fitted’)
plt.show()
“`
Summary Statistics of Residuals
It’s beneficial to compute summary statistics of the residuals to better understand their distribution. Here’s a table summarizing the key statistics:
Statistic | Value |
---|---|
Mean | {:.4f} |
Standard Deviation | {:.4f} |
Min | {:.4f} |
Max | {:.4f} |
You can compute these statistics using NumPy:
“`python
import numpy as np
mean_residuals = np.mean(residuals)
std_residuals = np.std(residuals)
min_residuals = np.min(residuals)
max_residuals = np.max(residuals)
print(f’Mean: {mean_residuals:.4f}, Std: {std_residuals:.4f}, Min: {min_residuals:.4f}, Max: {max_residuals:.4f}’)
“`
This summary gives a clearer picture of the residuals, helping to assess model adequacy and potential improvements.
Accessing Residuals in Statsmodels
In Statsmodels, obtaining residuals from a fitted model is straightforward. After fitting a model, you can access the residuals directly through the model’s attributes. The residuals are defined as the difference between the observed values and the predicted values.
To extract residuals:
- Fit a model using a suitable method (e.g., Ordinary Least Squares).
- Use the `resid` attribute of the fitted model.
Example code snippet:
“`python
import statsmodels.api as sm
import numpy as np
import pandas as pd
Example data
data = pd.DataFrame({
‘x’: np.random.rand(100),
‘y’: np.random.rand(100)
})
Fit the model
X = sm.add_constant(data[‘x’]) Adding a constant for intercept
model = sm.OLS(data[‘y’], X).fit()
Accessing residuals
residuals = model.resid
“`
Analyzing Residuals
Residuals can provide valuable insights into the model’s performance. Analyzing residuals helps identify patterns that suggest model inadequacies. Key aspects to consider include:
- Normality: Residuals should ideally be normally distributed.
- Homoscedasticity: The spread of residuals should remain constant across all levels of the independent variable.
- Independence: Residuals should not exhibit autocorrelation.
To visually inspect these properties, common diagnostic plots include:
- Residuals vs. Fitted Plot: To check for homoscedasticity.
- QQ Plot: To assess normality of residuals.
- Histogram of Residuals: Another method to check the distribution.
Example code for diagnostic plots:
“`python
import matplotlib.pyplot as plt
import seaborn as sns
import statsmodels.api as sm
Residuals vs. Fitted
plt.figure(figsize=(12, 6))
plt.subplot(1, 2, 1)
plt.scatter(model.fittedvalues, residuals)
plt.axhline(0, linestyle=’–‘, color=’red’)
plt.title(‘Residuals vs. Fitted’)
plt.xlabel(‘Fitted Values’)
plt.ylabel(‘Residuals’)
QQ Plot
plt.subplot(1, 2, 2)
sm.qqplot(residuals, line=’s’, ax=plt.gca())
plt.title(‘QQ Plot of Residuals’)
plt.tight_layout()
plt.show()
“`
Statistical Information on Residuals
Statsmodels provides detailed statistical information about the residuals through various attributes and methods. Important statistics include:
- Mean of Residuals: Should be close to zero.
- Standard Deviation of Residuals: Indicates variability.
- Skewness and Kurtosis: To assess the distribution shape.
You can compute these statistics using:
“`python
Statistical information on residuals
mean_residuals = np.mean(residuals)
std_residuals = np.std(residuals)
skewness = pd.Series(residuals).skew()
kurtosis = pd.Series(residuals).kurt()
Display results
residuals_stats = pd.DataFrame({
‘Statistic’: [‘Mean’, ‘Standard Deviation’, ‘Skewness’, ‘Kurtosis’],
‘Value’: [mean_residuals, std_residuals, skewness, kurtosis]
})
print(residuals_stats)
“`
This table summarizes the key statistics related to residuals, enabling a deeper understanding of model behavior and potential areas for improvement.
Understanding Residuals in Statsmodels: Expert Insights
Dr. Emily Carter (Data Scientist, Analytics Innovations). “Residuals are a crucial component in regression analysis, as they provide insights into the model’s accuracy. In Python’s Statsmodels, you can easily access residuals using the `resid` attribute after fitting a model, which allows for effective diagnostic checks and model refinement.”
Michael Chen (Statistical Analyst, Quantitative Research Group). “When working with residuals in Statsmodels, it’s essential to visualize them to assess the assumptions of linear regression. Utilizing plots such as residuals vs. fitted values can reveal patterns indicating potential issues with the model, which is vital for ensuring robust statistical inference.”
Dr. Sarah Thompson (Professor of Statistics, University of Data Science). “Interpreting residuals correctly is fundamental to understanding model performance. In Python, Statsmodels not only allows you to extract residuals but also provides tools to conduct further statistical tests, enhancing the reliability of your conclusions drawn from the model.”
Frequently Asked Questions (FAQs)
How can I obtain residuals from a statsmodels regression model in Python?
You can obtain residuals by accessing the `resid` attribute of the fitted model object. For example, after fitting a model using `OLS`, you can retrieve residuals with `model.resid`.
What do residuals represent in a regression analysis?
Residuals represent the difference between the observed values and the values predicted by the regression model. They indicate how well the model fits the data.
Can I visualize residuals in statsmodels, and if so, how?
Yes, you can visualize residuals using various plotting functions. A common method is to use `matplotlib` to create a scatter plot of residuals versus fitted values, or to generate a Q-Q plot to assess normality.
What is the significance of analyzing residuals?
Analyzing residuals helps identify patterns that may indicate model inadequacies, such as non-linearity, heteroscedasticity, or the presence of outliers, which can affect the validity of the regression results.
Are there built-in functions in statsmodels for residual analysis?
Yes, statsmodels provides several built-in functions for residual analysis, including `sm.graphics.tsa.plot_acf` for autocorrelation and `sm.qqplot` for assessing normality of residuals.
How do I interpret the residuals in terms of model performance?
Interpreting residuals involves checking for randomness and homoscedasticity. Ideally, residuals should be randomly distributed around zero without patterns, indicating a good model fit.
In the context of using the Statsmodels library in Python, obtaining residuals from statistical models is a crucial step in evaluating model performance. Residuals, which are the differences between observed and predicted values, provide insights into the model’s accuracy and can help identify patterns that the model may not have captured. Statsmodels offers straightforward methods to extract residuals from various types of models, including Ordinary Least Squares (OLS) regression, Generalized Linear Models (GLM), and others.
To retrieve residuals in Statsmodels, users typically fit a model using the appropriate function, such as `OLS` or `GLM`, and then access the residuals through the model’s results object. For instance, after fitting an OLS model, one can simply call the `resid` attribute of the results object to obtain the residuals. This functionality is essential for diagnostic checks, such as assessing homoscedasticity and normality of residuals, which are fundamental assumptions in regression analysis.
Moreover, analyzing residuals can provide valuable insights into potential model improvements. For example, if residuals exhibit a non-random pattern, it may indicate that the model is missing important predictors or that a transformation of the response variable is necessary.
Author Profile
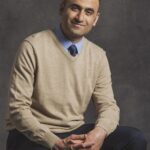
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?