How Can You Get the Current User ID in React?
In the ever-evolving landscape of web development, React has emerged as a powerful library for building dynamic user interfaces. As developers strive to create personalized experiences, one of the fundamental tasks is to retrieve and manage user-specific data. Understanding how to get the current user ID in a React application is crucial for implementing features such as user authentication, personalized content, and secure data handling. Whether you’re building a social media platform, an e-commerce site, or a simple blog, knowing how to effectively access the current user’s information can significantly enhance the functionality and user experience of your application.
When working with user authentication in React, developers often rely on various methods to obtain the current user ID. This process typically involves integrating with authentication services or managing state effectively within the application. By leveraging tools such as context providers, state management libraries, or even local storage, developers can seamlessly access user information and ensure that their applications respond dynamically to user interactions.
Moreover, the approach to retrieving the current user ID can vary depending on the architecture of your application, whether it’s a single-page application (SPA) or a more complex structure involving server-side rendering. Understanding the underlying principles and best practices for managing user data not only streamlines development but also enhances security and user satisfaction. As we delve deeper
Using Context to Access User ID
React Context provides a way to share values (such as a user ID) between components without having to pass props manually through every level of the component tree. By setting up a context, you can access the current user ID from any component that is a descendant of the context provider.
To implement this, follow these steps:
- Create a User Context.
- Wrap your application in the User Provider.
- Access the User ID in any component using the useContext hook.
Here’s a basic example:
“`javascript
import React, { createContext, useContext, useState } from ‘react’;
// Create User Context
const UserContext = createContext();
// User Provider component
const UserProvider = ({ children }) => {
const [userId, setUserId] = useState(null); // Replace null with actual user ID logic
return (
{children}
);
};
// Hook to use User Context
const useUser = () => {
return useContext(UserContext);
};
// Component example using the User ID
const UserProfile = () => {
const { userId } = useUser();
return
;
};
“`
In this example, any component wrapped in the `UserProvider` can access the `userId` using the `useUser` hook.
Retrieving User ID from API
If your application requires fetching the user ID from an API, you can do so by implementing asynchronous logic within your component. The following example demonstrates how to fetch the user ID when the component mounts:
“`javascript
import React, { useEffect, useState } from ‘react’;
const UserProfile = () => {
const [userId, setUserId] = useState(null);
useEffect(() => {
const fetchUserId = async () => {
const response = await fetch(‘/api/user’); // Replace with actual API endpoint
const data = await response.json();
setUserId(data.id); // Assuming the API returns an object with an id property
};
fetchUserId();
}, []);
return
;
};
“`
This method ensures that you retrieve the current user ID dynamically when the component mounts.
Storing User ID in State Management
Using a state management library like Redux or Zustand can be beneficial for larger applications. This allows you to store the user ID globally, making it accessible from any component. Below is an example using Redux:
- **Set Up Redux Store**:
- Create actions and reducers for managing user ID.
- **Accessing User ID in Components**:
- Use `useSelector` to get the current user ID from the store.
Here’s a simplified version:
“`javascript
// actions.js
export const setUserId = (userId) => ({
type: ‘SET_USER_ID’,
payload: userId
});
// reducer.js
const userReducer = (state = { id: null }, action) => {
switch (action.type) {
case ‘SET_USER_ID’:
return { …state, id: action.payload };
default:
return state;
}
};
// Component example
import { useSelector } from ‘react-redux’;
const UserProfile = () => {
const userId = useSelector((state) => state.user.id);
return
;
};
“`
Using a state management solution provides a structured way to manage user data across your application, ensuring that the current user ID is readily available wherever needed.
Method | Pros | Cons |
---|---|---|
React Context | Simple setup, no external libraries | Can become cumbersome with deeply nested components |
API Fetch | Dynamic retrieval of user ID | Requires network calls, potential latency |
State Management (Redux) | Centralized state, easy access | Additional complexity, requires setup |
Accessing the Current User ID in React
In React applications, obtaining the current user ID typically involves managing authentication state. This state can be derived from various sources such as context providers, Redux stores, or local storage, depending on the architecture of the application.
Using Context API
The Context API provides a way to share values like user authentication state across the component tree without passing props down manually at every level.
“`jsx
import React, { createContext, useContext, useState } from ‘react’;
// Create a Context
const AuthContext = createContext();
// Provider Component
export const AuthProvider = ({ children }) => {
const [user, setUser] = useState({ id: ‘12345’, name: ‘John Doe’ });
return (
{children}
);
};
// Custom hook to use the AuthContext
export const useAuth = () => {
return useContext(AuthContext);
};
// Component to display user ID
const UserProfile = () => {
const user = useAuth();
return
;
};
“`
Using Redux for State Management
Redux is another popular method for managing global state in React applications. If your application utilizes Redux, you can access the current user ID from the Redux store.
“`jsx
import { useSelector } from ‘react-redux’;
// Component to display user ID
const UserProfile = () => {
const userId = useSelector(state => state.auth.user.id);
return
;
};
“`
Accessing User ID from Local Storage
In scenarios where user authentication information is stored in local storage, you can easily retrieve the current user ID from there.
“`jsx
// Component to display user ID
const UserProfile = () => {
const userId = localStorage.getItem(‘userId’);
return
;
};
“`
Example of a Complete User Context Implementation
“`jsx
import React, { createContext, useContext, useState } from ‘react’;
// Create User Context
const UserContext = createContext();
// User Provider
export const UserProvider = ({ children }) => {
const [user, setUser] = useState({ id: null, name: ” });
const login = (userId, userName) => {
setUser({ id: userId, name: userName });
localStorage.setItem(‘userId’, userId);
};
return (
{children}
);
};
// Custom hook
export const useUser = () => {
return useContext(UserContext);
};
// Component to login and display user ID
const LoginComponent = () => {
const { login } = useUser();
const handleLogin = () => {
login(‘12345’, ‘John Doe’);
};
return ;
};
const UserProfile = () => {
const { user } = useUser();
return
;
};
“`
Best Practices for Managing User ID
- State Management: Choose a state management solution that suits the complexity of your application. For simpler apps, Context API may suffice, whereas Redux is better for larger applications.
- Security: Avoid storing sensitive user information in local storage. Consider using secure HTTP-only cookies for managing authentication tokens.
- Reactivity: Ensure that components reactively update when the user ID changes, leveraging hooks or state management libraries.
Summary of Approaches
Approach | Description | When to Use |
---|---|---|
Context API | Shares state across components easily | Simpler applications |
Redux | Centralized state management | Larger applications |
Local Storage | Persistent storage for user data | When needing to retain state on refresh |
Expert Insights on Retrieving Current User ID in React
Dr. Emily Carter (Senior Software Engineer, Tech Innovations Inc.). “To effectively get the current user ID in a React application, developers should leverage context APIs or state management libraries like Redux. This approach ensures that the user ID is accessible throughout the component tree without prop drilling.”
Michael Chen (Lead Frontend Developer, Web Solutions Co.). “Using hooks such as useContext or custom hooks can simplify the process of retrieving the current user ID. This method promotes cleaner code and enhances maintainability, especially in large-scale applications.”
Sarah Johnson (React Specialist, CodeCraft Academy). “It’s crucial to handle user authentication properly to ensure that the current user ID is securely stored and retrieved. Implementing libraries like Firebase or Auth0 can streamline this process and provide robust security measures.”
Frequently Asked Questions (FAQs)
How can I retrieve the current user ID in a React application?
You can retrieve the current user ID in a React application by accessing the user state from your context or state management solution (like Redux). For example, if you’re using React Context, you can use the `useContext` hook to access the user object and extract the ID.
Is there a built-in method to get the current user ID in React?
React does not provide a built-in method to get the current user ID. You typically need to implement your own logic using state management or context to store and retrieve the user information.
What libraries can help manage user authentication in React?
Libraries such as Firebase, Auth0, and Redux Toolkit can help manage user authentication in React applications. These libraries often provide methods to access user information, including the user ID.
How do I access user ID from a REST API in React?
To access the user ID from a REST API in React, you can make an HTTP request using libraries like Axios or Fetch. Once you receive the user data, you can extract the user ID from the response.
Can I store the current user ID in local storage?
Yes, you can store the current user ID in local storage for persistent access across sessions. Use `localStorage.setItem(‘userId’, userId)` to store and `localStorage.getItem(‘userId’)` to retrieve it.
What should I do if the user ID is not available?
If the user ID is not available, ensure that the user is authenticated and that the user data is being correctly fetched from your authentication provider or API. Implement error handling to manage scenarios where the user ID cannot be retrieved.
In React applications, obtaining the current user ID is a crucial aspect, especially when managing user-specific data or implementing features that require user authentication. Typically, this process involves integrating a state management solution, such as Context API or Redux, to store user information after authentication. Once the user is authenticated, their ID can be accessed from the global state, allowing components to reactively render user-specific content.
Moreover, utilizing hooks like `useContext` or `useSelector` can streamline the process of retrieving the current user ID from the application state. This approach not only promotes cleaner code but also enhances the maintainability of the application. Additionally, leveraging libraries such as Firebase or Auth0 can simplify user authentication and provide built-in methods to access user data, including user IDs.
effectively managing the current user ID in a React application involves a combination of proper state management and authentication practices. By following these methodologies, developers can ensure that their applications are both user-friendly and efficient, ultimately leading to a better user experience. Understanding these concepts is essential for any developer looking to build robust and scalable React applications.
Author Profile
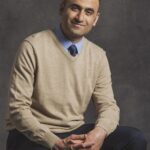
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- April 13, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- April 13, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- April 13, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- April 13, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?