How Can You Convert a Byte Array to a String in Golang?
In the world of programming, data manipulation often requires seamless transitions between different data types. One common scenario developers encounter is the need to convert byte arrays to strings, particularly in languages like Go (Golang). Understanding how to effectively handle these conversions is not just a matter of syntax; it’s a crucial skill that can enhance your coding efficiency and improve your application’s performance. Whether you’re dealing with data from network communications, file I/O, or simply transforming data for display, mastering this conversion can unlock new possibilities in your projects.
Golang, known for its simplicity and efficiency, provides robust tools for handling byte arrays and strings. At first glance, these two data types might seem interchangeable, but they serve different purposes and come with unique characteristics. A byte array, for instance, is a sequence of bytes that can represent raw binary data, while a string is a more abstract representation designed for human-readable text. This distinction is vital when you’re working with data that requires precise manipulation or when you’re interfacing with external systems.
In this article, we will explore the various methods available in Go for converting byte arrays to strings, examining the nuances of each approach and the scenarios in which they are most effective. By the end, you’ll not only understand the mechanics behind the conversion process but also appreciate the
Understanding Byte Arrays in Go
In Go, byte arrays are a sequence of bytes and are often used for handling raw data or binary formats. The `[]byte` type is commonly employed when dealing with file I/O, network communication, or encoding and decoding operations. Converting a byte array to a string is a frequent operation, especially when you need to manipulate or display textual data.
Conversion Techniques
There are several methods to convert a byte array to a string in Go, each suited for different scenarios. The most common techniques include:
- Using the string() Conversion: The simplest and most direct way to convert a byte array to a string is by using the built-in `string()` function. This method creates a new string from the byte slice.
- Using the bytes Package: The `bytes` package provides functions that can be utilized for more complex operations, such as concatenating byte slices or manipulating them before conversion.
Here’s a simple comparison of these methods:
Method | Example | Use Case |
---|---|---|
string() | str := string(byteArray) | Basic conversion with no additional processing |
bytes.NewBuffer | str := buf.String() | When building strings from multiple byte slices |
Example Code Snippets
To illustrate these techniques, consider the following example code:
“`go
package main
import (
“bytes”
“fmt”
)
func main() {
byteArray := []byte{72, 101, 108, 108, 111} // Represents “Hello”
// Method 1: Using string() conversion
str1 := string(byteArray)
fmt.Println(“Using string():”, str1)
// Method 2: Using bytes.NewBuffer
var buf bytes.Buffer
buf.Write(byteArray)
str2 := buf.String()
fmt.Println(“Using bytes.NewBuffer:”, str2)
}
“`
This code demonstrates both methods of conversion, with `byteArray` containing the byte values that correspond to the ASCII characters of “Hello”. The output will be:
“`
Using string(): Hello
Using bytes.NewBuffer: Hello
“`
Considerations When Converting
When converting byte arrays to strings, consider the following:
- Encoding: The byte array should represent valid UTF-8 encoded text. If the byte slice contains invalid sequences, the resulting string may not be as expected.
- Performance: The `string()` conversion creates a new string based on the byte slice, which might involve memory allocation. For large data sets or performance-critical applications, evaluate the impact of conversion methods.
- Immutability: Strings in Go are immutable. Once a string is created from a byte array, any modifications require creating a new string.
By understanding these techniques and considerations, developers can effectively manage data transformations between byte arrays and strings in Go.
Converting a Byte Array to String in Go
In Go, converting a byte array to a string is a straightforward process. The primary method involves using the built-in `string()` function, which allows for an efficient conversion. Below are examples and explanations of this conversion.
Using the String Constructor
The most common and simplest method is to use the `string()` conversion. This method directly converts a byte slice (`[]byte`) to a string. The syntax is as follows:
“`go
byteArray := []byte{72, 101, 108, 108, 111}
str := string(byteArray)
“`
In this example, the byte array represents the ASCII values for the characters “Hello”. The resulting string `str` will contain “Hello”.
Considerations for Conversion
When converting a byte array to a string in Go, keep the following considerations in mind:
- Encoding: The byte array should represent valid UTF-8 encoded data. If it does not, the resulting string may contain invalid characters or lead to unexpected behavior.
- Memory Efficiency: The conversion creates a new string, which is a copy of the data in the byte array. This means that modifications to the original byte array will not affect the string.
Example with UTF-8 Encoding
Here’s an example that demonstrates converting a byte array with UTF-8 encoded characters:
“`go
byteArray := []byte{228, 189, 160, 229, 165, 189} // “你好” in UTF-8
str := string(byteArray)
fmt.Println(str) // Output: 你好
“`
This conversion accurately represents the string “你好”.
Performance Considerations
Using the `string()` conversion for byte arrays is generally efficient, but there are scenarios where performance may be impacted:
- Large Byte Arrays: For very large byte arrays, consider the implications of creating a large string in memory. If the byte array is large, the conversion may lead to increased memory usage.
- Repeated Conversions: If conversions are required repeatedly in performance-critical code, cache the result or use more efficient data structures if applicable.
Alternative Methods
While the `string()` conversion is the most common method, there are alternative approaches depending on the context:
- Using `fmt.Sprintf`: This approach can be used for formatted output but is less efficient for simple conversions.
“`go
str := fmt.Sprintf(“%s”, byteArray)
“`
- Using `bytes.Buffer`: This can be useful for building strings from multiple byte arrays or appending data.
“`go
var buffer bytes.Buffer
buffer.Write(byteArray)
str := buffer.String()
“`
Common Use Cases
Byte-to-string conversions are frequently used in various scenarios:
- Data Transmission: When receiving byte data from network operations.
- File Operations: Reading data from files that return byte arrays.
- Interfacing with APIs: Handling binary data returned from web services.
Utilizing the correct method for conversion can enhance code readability and performance, making it essential to choose the right approach based on the context of your application.
Expert Insights on Converting Byte Arrays to Strings in Golang
Julia Chen (Senior Software Engineer, GoLang Innovations). “Converting a byte array to a string in Go is straightforward using the built-in string conversion. Simply use `string(byteArray)` to achieve this, which is efficient and idiomatic in Go programming.”
Michael Thompson (Go Language Specialist, Tech Solutions Inc.). “It is crucial to understand that when converting byte arrays to strings, you should ensure that the byte array contains valid UTF-8 encoded data. Otherwise, you may encounter unexpected behavior or errors in your application.”
Sarah Patel (Lead Developer, Cloud Native Applications). “For performance-sensitive applications, consider using `bytes.Buffer` for concatenating byte slices before converting to a string. This approach can help optimize memory allocations and improve efficiency in scenarios involving large data sets.”
Frequently Asked Questions (FAQs)
How can I convert a byte array to a string in Go?
You can convert a byte array to a string in Go using the `string()` function. For example, `str := string(byteArray)` will convert the `byteArray` to a string.
What is the difference between converting a byte slice and a byte array to a string in Go?
In Go, a byte slice is dynamically sized and can be converted to a string using the `string()` function, while a byte array has a fixed size. Both can be converted using the same method, but the syntax differs slightly.
Are there any performance considerations when converting byte arrays to strings in Go?
Yes, converting a byte array to a string creates a new string value, which involves copying the data. For large byte arrays, this can impact performance. If you need to perform multiple conversions, consider using `bytes.Buffer` for efficiency.
Can I convert a string back to a byte array in Go?
Yes, you can convert a string back to a byte array using the `[]byte()` conversion. For example, `byteArray := []byte(str)` will convert the string `str` back to a byte array.
Is it safe to convert a byte array containing non-UTF-8 bytes to a string in Go?
Yes, it is safe to convert a byte array with non-UTF-8 bytes to a string, but the resulting string may contain invalid characters. It is advisable to handle such cases appropriately, especially when dealing with text data.
What happens if I convert an empty byte array to a string in Go?
Converting an empty byte array to a string in Go will result in an empty string. For example, `emptyByteArray := []byte{}` will yield `emptyString := string(emptyByteArray)` as an empty string.
In Go (Golang), converting a byte array to a string is a straightforward process that leverages the language’s built-in capabilities. The primary method to achieve this conversion is by using the `string()` function, which takes a byte slice as an argument and returns the corresponding string representation. This approach is efficient and commonly used in various applications, especially when handling data that originates from network communications or file I/O operations.
It is important to note that the conversion from a byte array to a string creates a new string instance. This means that any modifications to the original byte array will not affect the string, as strings in Go are immutable. Understanding this behavior is crucial for developers to avoid unintended side effects in their applications. Additionally, developers should be aware of the encoding of the byte array to ensure that the resulting string accurately represents the intended characters, especially when dealing with non-ASCII data.
In summary, converting a byte array to a string in Golang is a simple yet essential operation that can be performed using the `string()` function. Developers should consider the immutability of strings and the importance of encoding when performing this conversion. By adhering to these principles, developers can effectively manage data transformations within their Go applications.
Author Profile
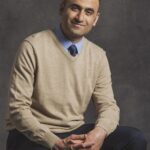
-
Dr. Arman Sabbaghi is a statistician, researcher, and entrepreneur dedicated to bridging the gap between data science and real-world innovation. With a Ph.D. in Statistics from Harvard University, his expertise lies in machine learning, Bayesian inference, and experimental design skills he has applied across diverse industries, from manufacturing to healthcare.
Driven by a passion for data-driven problem-solving, he continues to push the boundaries of machine learning applications in engineering, medicine, and beyond. Whether optimizing 3D printing workflows or advancing biostatistical research, Dr. Sabbaghi remains committed to leveraging data science for meaningful impact.
Latest entries
- March 22, 2025Kubernetes ManagementDo I Really Need Kubernetes for My Application: A Comprehensive Guide?
- March 22, 2025Kubernetes ManagementHow Can You Effectively Restart a Kubernetes Pod?
- March 22, 2025Kubernetes ManagementHow Can You Install Calico in Kubernetes: A Step-by-Step Guide?
- March 22, 2025TroubleshootingHow Can You Fix a CrashLoopBackOff in Your Kubernetes Pod?